Streamlining User Management: Save Application Users to a Table
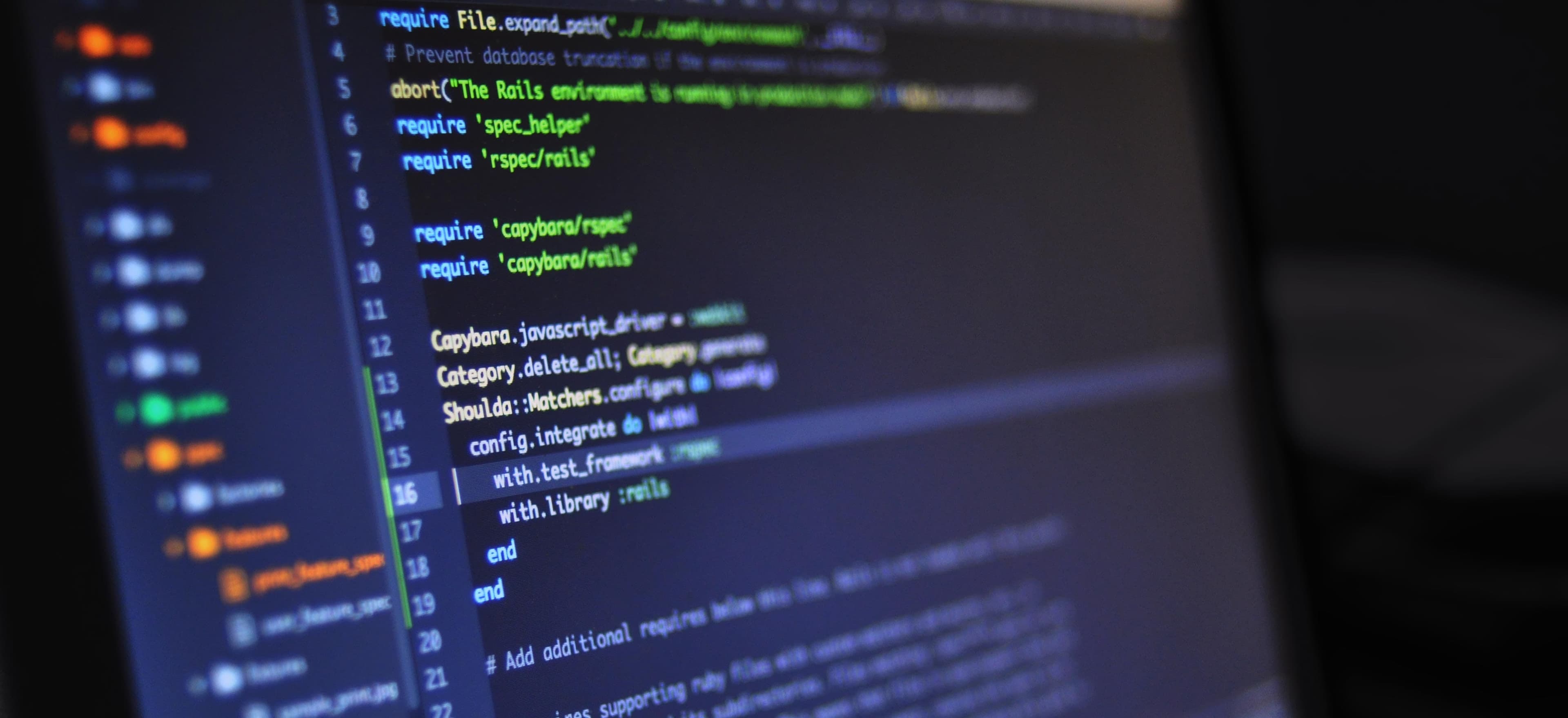
- Published on
Streamlining User Management: Save Application Users to a Table
User management is a crucial aspect of any application. Maintaining user data effectively not only enhances the user experience but also streamlines application performance. This blog post will guide you through the process of implementing a simple user management system in Java, using relational databases to store users efficiently.
In this article, we will cover:
- The significance of user management
- A brief introduction to databases
- The Java Database Connectivity (JDBC) API
- Code snippets for saving users to a database
- Best practices for user data storage
- Additional resources for further learning
Importance of User Management
User management is the backbone of many applications. Whether you are developing a simple web application or a complex enterprise system, efficient user data handling can lead to a more robust application. Why is it important?
- User Authentication: Securely storing user credentials ensures only authorized access to your application.
- User Data Integrity: Effectively managing user data minimizes the chance of duplicate or erroneous entries.
- Scalability: A well-structured user table allows your application to handle growth seamlessly.
In short, user management is not just about saving user data; it’s about creating a seamless experience that fosters trust and reliability.
Setting the Stage to Databases
Databases serve as structured repositories for application data. They keep the data organized, enabling quick retrieval and manipulation. Relational databases, like MySQL, PostgreSQL, and SQLite, use tables to store information, making them ideal for user management.
Basic Terminology
- Table: A collection of related data entries consisting of rows and columns.
- Row: A single record in a table.
- Column: A category of data stored in a table.
For instance, a users table might include columns such as user_id
, username
, password
, and email
.
Setting the Stage to JDBC
Java Database Connectivity (JDBC) is an API that allows Java applications to interact with databases. It provides methods to execute SQL queries, manage connections, and process results.
To start using JDBC, you'll need:
- A JDBC driver for your database.
- An understanding of SQL for database manipulation.
Here's a simple example of how to download the necessary JDBC driver for MySQL. You can find it here.
Code Snippet: Saving Users to a Database
Let's explore how to save user information to a database using JDBC. The following code snippets assume the creation of a users table with columns: user_id
(INT), username
(VARCHAR), password
(VARCHAR), and email
(VARCHAR).
Step 1: Setting Up the Database
CREATE TABLE users (
user_id INT AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(255) NOT NULL,
password VARCHAR(255) NOT NULL,
email VARCHAR(255) NOT NULL UNIQUE
);
Step 2: Connecting to the Database
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseConnection {
private static final String URL = "jdbc:mysql://localhost:3306/yourdatabase";
private static final String USER = "yourusername";
private static final String PASSWORD = "yourpassword";
public static Connection getConnection() throws SQLException {
return DriverManager.getConnection(URL, USER, PASSWORD);
}
}
Commentary:
DriverManager.getConnection()
facilitates the connection to the database.- Replace
yourdatabase
,yourusername
, andyourpassword
with your actual database details.
Step 3: Inserting a User
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class UserManager {
public void saveUser(String username, String password, String email) {
String sql = "INSERT INTO users (username, password, email) VALUES (?, ?, ?)";
try (Connection conn = DatabaseConnection.getConnection();
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setString(1, username);
pstmt.setString(2, password); // Consider hashing passwords using a library like BCrypt
pstmt.setString(3, email);
pstmt.executeUpdate();
System.out.println("User saved successfully.");
} catch (SQLException e) {
System.out.println(e.getMessage());
}
}
}
Commentary:
- Using
PreparedStatement
helps prevent SQL injection by precompiling the SQL query. - Make sure to hash passwords before storing them for enhanced security. Consider using libraries such as BCrypt.
Step 4: Testing User Creation
public class Main {
public static void main(String[] args) {
UserManager userManager = new UserManager();
userManager.saveUser("john_doe", "securePass123", "john@example.com");
}
}
Best Practices for User Data Storage
To ensure optimal performance and security, adhere to the following best practices:
- Use of Prepared Statements: Always use prepared statements to avoid SQL injection vulnerabilities.
- Password Hashing: Never store plain text passwords. Use libraries like BCrypt or PBKDF2 to hash sensitive information.
- Regular Backups: Ensure regular data backups to prevent data loss.
- Data Validation: Always validate user inputs to maintain data integrity.
My Closing Thoughts on the Matter
In this post, we've built a simple Java application that connects to a MySQL database to save user information effectively. User management is a complex task, but you can streamline the process by leveraging JDBC and structured data storage.
For those keen on delving deeper into user management systems, consider exploring additional topics such as:
- Implementing user authentication mechanisms
- Using frameworks like Spring Security for advanced features
- Leveraging ORM tools like Hibernate for simplified database interactions
By mastering these techniques, you'll not only enhance your application's robustness but also provide a seamless user experience.
For more information, check out these resources:
- Java JDBC Documentation
- MySQL Connector/J Documentation
- Spring Security Documentation
Happy coding!
Checkout our other articles