How Automated Tests Can Slow Down Your Development Process
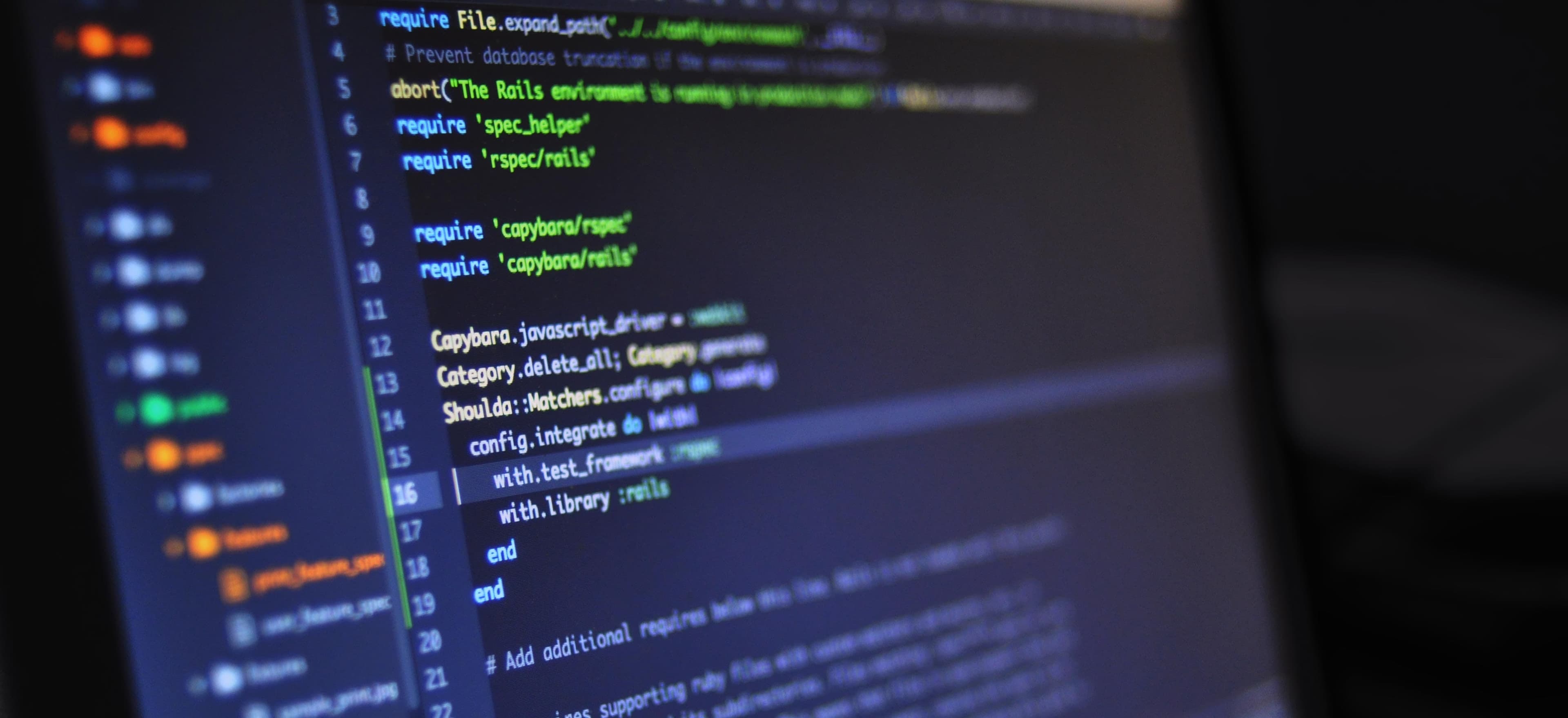
- Published on
How Automated Tests Can Slow Down Your Development Process
Automated testing is often seen as a cornerstone of modern software development. It offers numerous benefits, such as reducing manual testing time, increasing test coverage, and fostering confidence in code changes. However, the relationship between automated tests and the development process can be more complex than it appears at first glance. In this article, we will explore how automated tests can inadvertently slow down your development process and what you can do to mitigate these effects.
Understanding Automated Testing
Automated testing encompasses a variety of testing practices, including unit tests, integration tests, and end-to-end tests. These tests can be performed using tools like JUnit for Java applications or Selenium for web applications. The primary goal of automated testing is to ensure code quality and functionality while minimizing human error.
The Good: Benefits of Automated Testing
Before delving into the downsides, it’s essential to understand the positives:
- Increased Coverage: Automated tests can cover a vast array of scenarios that manual tests might miss.
- Immediate Feedback: Developers gain quick feedback on their code, helping identify bugs early.
- Cost-Effective in the Long Term: Although the initial setup can be time-consuming, automated tests can save significant time and effort in the long run.
For more details, check out Testing Strategies in Software Development.
The Downside: How Automated Tests Can Slow Down Development
While automated testing brings many advantages, it can also impede development speed in several ways:
1. Time-Consuming Setup and Maintenance
Creating an automated test suite requires a considerable time investment. Writing test cases, setting up the testing framework, and maintaining these tests as the code evolves can consume a lot of resources.
Example: Setting Up a JUnit Test
Here's a simple example of setting up a JUnit test.
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3), "2 + 3 should equal 5");
}
}
In this code:
- The
Calculator
class needs to exist before the test can be written. If it isn't properly defined, the test is worthless. - The initial setup might take time, especially for complex systems involving databases or external APIs.
2. False Sense of Security
Automated tests can create a false sense of security, leading developers to overlook manual testing practices. This could mean bugs slipping through the cracks when the automated tests don't cover certain edge cases.
Example: Lack of Edge Case Testing
Suppose we have a method that completes a user input. The automated tests might check for general user inputs but fail to account for peculiar inputs such as special characters or large volumes of data.
public void registerUser(String username, int age) {
// Simplified example
if (username.isEmpty() || age < 0) {
throw new IllegalArgumentException("Invalid user info");
}
// proceed with registration...
}
In automated tests, if you forget to test peculiar edge cases such as:
- Empty usernames
- Negative age values
The application could fail under real-world conditions, leaving a gap in your testing strategy.
3. Extended Feedback Loop
While automated tests often provide quick feedback, there are scenarios where the feedback loop can be lengthy, especially for integration and end-to-end tests. If your test suite takes longer to execute:
- Developers may end up waiting for tests to pass before continuing with their work.
- The overall agile flow is disrupted, slowing down the pace of development.
4. Complexity in Test Design
Writing insightful tests requires an understanding of both the functionality being tested and the testing framework.
Example: Using Mocks and Stubs
Consider the scenario where you're testing a service that relies on external resources. Using tools like Mockito to create mocks and stubs can complicate your test design.
import static org.mockito.Mockito.*;
import org.junit.jupiter.api.Test;
public class UserServiceTest {
@Test
void testGetUserById() {
UserRepository mockRepo = mock(UserRepository.class);
UserService userService = new UserService(mockRepo);
when(mockRepo.findById(1)).thenReturn(new User("Alice"));
User user = userService.getUserById(1);
assertEquals("Alice", user.getName());
}
}
In this case:
- Additional libraries complicate the testing process.
- Mocks can lead to convoluted setups that muddy the intention of the test.
5. Continuous Refactoring Needs
As the application evolves, the tests can become obsolete or fail due to changes in implementation. Regular refactoring of tests is essential, which can distract developers from new features or critical fixes.
Example: Adapting to New APIs
If the API changes, the tests that rely on it must also be updated. Otherwise, they could cause false positives or negatives during the deployment pipeline.
Mitigating the Risks
To address the potential slowdown caused by automated tests, consider adopting the following strategies:
-
Prioritize Critical Tests Focus on writing high-quality tests for critical functionalities first. This will ensure that your essential features are adequately validated.
-
Embrace Testing Pyramid Follow the testing pyramid approach, where you have a larger number of unit tests, fewer integration tests, and an even lesser number of end-to-end tests. This balances speed with coverage effectively.
-
Continuous Improvement of Test Suites Regularly review and refactor your tests to keep them relevant and efficient. Remove deprecated tests and merge redundant ones.
-
Utilize CI/CD Best Practices Implement Continuous Integration/Continuous Deployment practices to run your automated tests in a controlled environment, ensuring they do not block developer progress unnecessarily.
-
Realistic Testing Timeframes Plan testing cycles realistically while taking into account the time automated tests will consume. Allocate dedicated sprints for writing and refactoring tests.
The Closing Argument
Automated tests play a crucial role in maintaining software quality, but they can also create bottlenecks in the development process if not managed correctly. By being aware of the ways automated tests can slow down your development, you can implement strategies to effectively balance testing and development speed.
Through thoughtful test design, prioritizing your tests, and continuous improvement, you can ensure that your automated tests support rather than hinder your development process. The goal is not to eliminate automated testing altogether, but to integrate it seamlessly into your workflow, fostering both quality software and an agile development environment.
For further insights, you can refer to The Testing Pyramid Explained.
Happy coding!
Checkout our other articles