Unlocking the Mystery of Java Streams: Terminal Operation Troubles
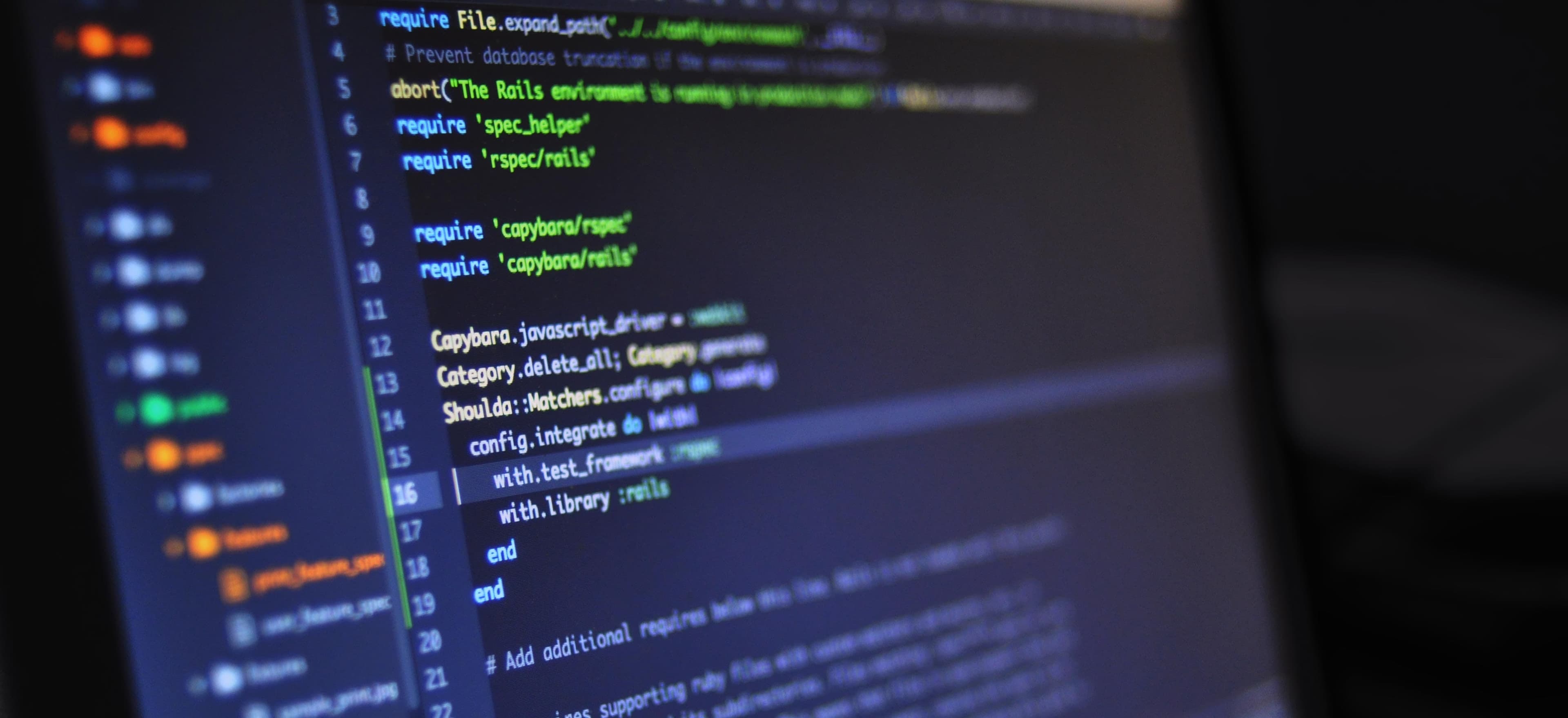
- Published on
Unlocking the Mystery of Java Streams: Terminal Operation Troubles
Java Streams API has revolutionized the way we handle collections in modern Java programming. Introduced in Java 8, it provides a powerful framework for processing sequences of elements in a functional style. However, while Streams offer a concise way to handle data, they come with their own set of challenges—particularly when it comes to terminal operations.
In this blog post, we'll dive deep into the concept of terminal operations, identify common pitfalls, and provide effective solutions to overcome them. If you’ve been wrestling with Java Streams or simply want to elevate your Java skills, you’re in the right place.
What Are Java Streams?
Java Streams are sequences of data that allow you to perform functional-style operations on collections. Streams can be processed in a pipeline, enabling a smooth flow of data from one operation to the next.
Key features of Java Streams:
- Declarative: Focus on what to do rather than how to do it.
- Lazy Evaluation: Operations are not executed until a terminal operation is invoked.
- Internal Iteration: Streams handle iteration, freeing developers from boilerplate code.
Understanding Terminal Operations
Terminal operations are the last step in a Stream pipeline. They produce a result or a side-effect and initiate the processing of the Stream. Some common terminal operations include:
collect()
forEach()
reduce()
count()
anyMatch()
Why Terminal Operations Matter
Understanding terminal operations is critical for several reasons:
- Performance Management: They dictate how data is processed and can impact application performance.
- Side Effects: They can produce side effects that you must manage, especially in parallel streams.
- Data Reduction: They can consolidate data in meaningful ways.
Common Terminal Operation Problems
Many developers run into issues when utilizing terminal operations. Below are a few examples along with solutions.
Problem 1: Performance Hits With forEach()
Using forEach()
as a terminal operation can lead to unexpected performance hits, especially when working with large datasets. This is often due to the fact that forEach()
executes in the order of the underlying source, making it less efficient than other approaches in some scenarios.
Example: Performing a File I/O Operation
List<String> names = Arrays.asList("Alice", "Bob", "Charles");
names.stream()
.forEach(name -> {
// Simulating File I/O
System.out.println(name);
});
This approach could impact performance if the list is large. Instead, consider collecting the results first before processing them:
List<String> collectedNames = names.stream().collect(Collectors.toList());
collectedNames.forEach(name -> /* perform action */);
Why This Matters: Using collect()
prepares data more efficiently for later operations.
Problem 2: Forgetting to Concat Streams
Developers often forget that Streams can be concatenated. When processing multiple collections, they might attempt to process them separately instead of using an efficient approach.
List<String> list1 = Arrays.asList("A", "B", "C");
List<String> list2 = Arrays.asList("D", "E");
Stream<String> combined = Stream.concat(list1.stream(), list2.stream());
combined.forEach(System.out::println);
Why This Matters: This method is streamlined, leading to cleaner code and avoiding unnecessary loops.
Problem 3: Redundant Computation with Reduce()
The reduce()
operation can also present pitfalls, particularly if your lambda expression is computationally intensive.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
Optional<Integer> sum = numbers.stream()
.reduce(0, (a, b) -> a + computeIntensiveOperation(b));
In this case, every number runs through computeIntensiveOperation
, increasing overhead. Optimize by computing values beforehand:
List<Integer> precomputed = numbers.stream()
.map(this::computeIntensiveOperation)
.collect(Collectors.toList());
Optional<Integer> sumOptimized = precomputed.stream().reduce(0, Integer::sum);
Why This Matters: Pre-computation avoids redundant calculations, enhancing performance.
Advanced Concepts: Short-Circuiting Operations
Short-circuiting terminal operations, like anyMatch()
or findFirst()
, allow for early termination of the Stream.
Example Usage
boolean anyEven = numbers.stream()
.anyMatch(n -> n % 2 == 0);
if (anyEven) {
System.out.println("List contains even numbers.");
}
Why This Matters: Utilizing short-circuiting operations can lead to substantial performance gains in large datasets.
Wrapping Up
Java Streams and their terminal operations offer an elegant way to process collections, but they come with their own set of challenges. By understanding common pitfalls and employing best practices, you can leverage Streams effectively while optimizing performance.
To further enhance your understanding, consider reading the Java Streams official documentation and exploring effective Java programming techniques that implement Streams in real-world applications.
Java Streams are a game-changer. They provide a fresh perspective on handling data, but only if you unlock their full potential. Armed with this knowledge, you should be equipped to tackle any terminal operation troubles head-on. Happy coding!