Mastering JavaFX Animation: Common Pitfalls and Solutions
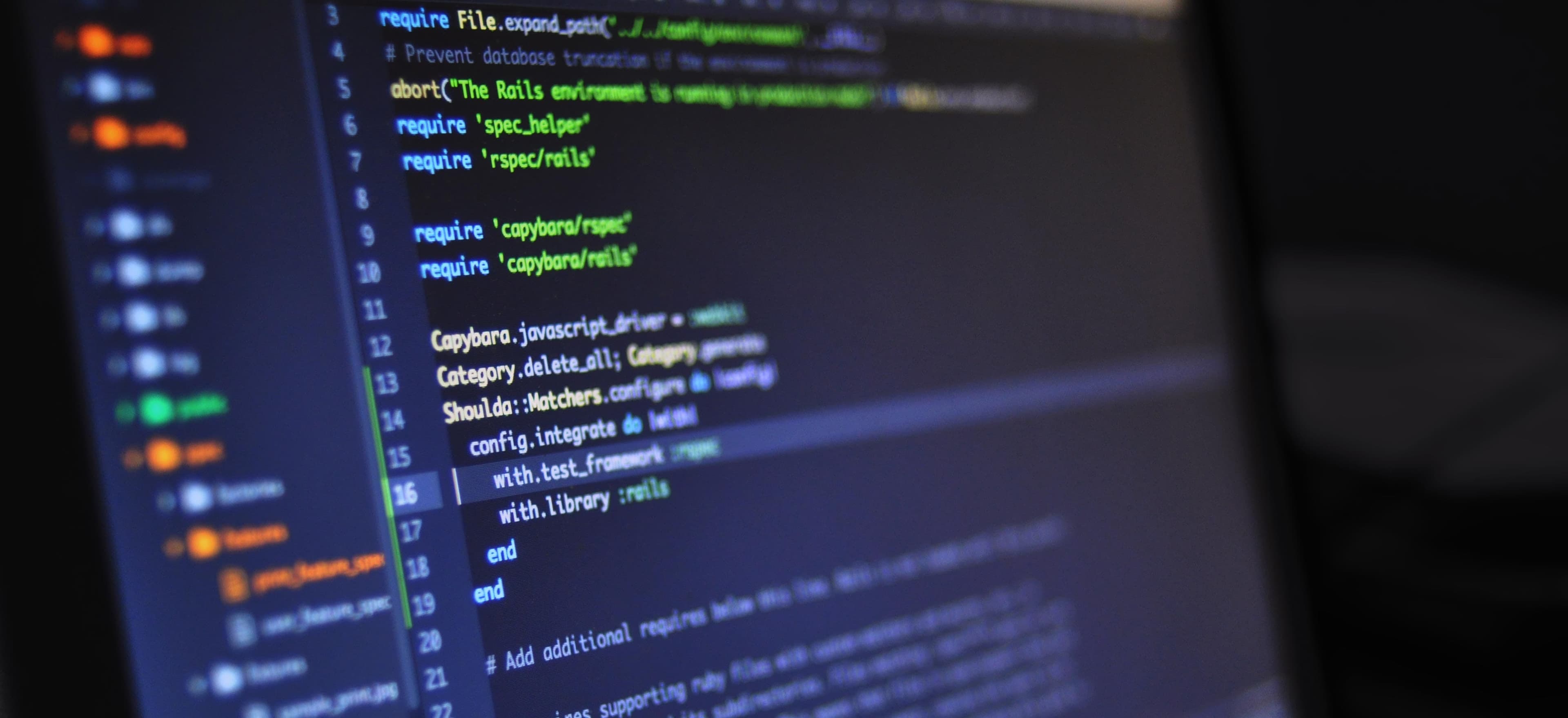
- Published on
Mastering JavaFX Animation: Common Pitfalls and Solutions
JavaFX is a powerful framework for developing rich desktop applications in Java. One of its standout features is the ability to create smooth animations that enhance user experiences. Animations can make your applications more visually appealing and engaging. However, newcomers and even seasoned developers can stumble upon common pitfalls when working with JavaFX animations. In this blog post, we’ll discuss these common pitfalls and provide solutions to help you master JavaFX animation.
Understanding JavaFX Animation Basics
Before diving into pitfalls, it’s essential to understand some core concepts of JavaFX animation. JavaFX provides several classes for animation, including:
Timeline
: For creating a sequence of keyframes.Transition
: For simple animations that involve transformations or property changes.FadeTransition
: Specifically designed for fade-in and fade-out effects.
Here's a basic example of using a FadeTransition
:
import javafx.animation.FadeTransition;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
import javafx.util.Duration;
public class FadeExample extends Application {
@Override
public void start(Stage primaryStage) {
Button button = new Button("Click to Fade");
FadeTransition fadeTransition = new FadeTransition(Duration.seconds(1), button);
fadeTransition.setFromValue(1.0);
fadeTransition.setToValue(0.0);
button.setOnAction(e -> fadeTransition.play());
StackPane root = new StackPane(button);
Scene scene = new Scene(root, 300, 200);
primaryStage.setTitle("Fade Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why FadeTransition?
FadeTransition
is simple to implement and gives feedback to the user, which is crucial for an engaging UI.- Using the
Duration
class allows you to precisely control how long the transition takes.
Common Pitfalls in JavaFX Animation
While JavaFX animations are powerful, there are several pitfalls developers frequently encounter:
1. Not Updating UI Components on the JavaFX Application Thread
Explanation
JavaFX is single-threaded, meaning all UI updates must occur on the JavaFX Application Thread. Attempting to modify UI components from background threads will lead to exceptions and unpredictable behavior.
Solution
Always ensure that you are on the JavaFX Application Thread using Platform.runLater()
. Here’s a snippet:
Platform.runLater(() -> {
// Update UI components
myLabel.setText("Updated Text");
});
2. Overusing Animation Loops
Explanation
Creating animations that loop indefinitely without purpose can lead to performance issues. It’s vital to strike a balance between aesthetics and performance.
Solution
Use .setCycleCount()
wisely. If an animation should repeat, set it to Animation.INDEFINITE
only when necessary.
Timeline timeline = new Timeline();
timeline.setCycleCount(Animation.INDEFINITE);
3. Failing to Define Keyframes Properly
Explanation
Defining keyframes is essential for smooth animations. Poorly defined keyframes can lead to jerky animations or unintended effects.
Solution
Use at least two keyframes, from and to. Here’s a code example:
Timeline timeline = new Timeline();
KeyFrame keyFrame1 = new KeyFrame(Duration.seconds(0), new KeyValue(myNode.opacityProperty(), 1));
KeyFrame keyFrame2 = new KeyFrame(Duration.seconds(2), new KeyValue(myNode.opacityProperty(), 0));
timeline.getKeyFrames().addAll(keyFrame1, keyFrame2);
timeline.play();
4. Ignoring Thread Safety
Explanation
With animations, we can be tempted to access shared resources without proper synchronization. This can lead to race conditions and inconsistent states.
Solution
Consider using javafx.beans.property
to help manage state and automatically notify listeners.
5. Lack of Performance Optimization
Explanation
Animations running without optimization can degrade the user experience. Considerations like frame rate and hardware acceleration can significantly affect performance.
Solution
Use the JavaFX Scene Graph effectively. Limit the number of nodes being animated at once, and utilize Node
features such as caching and translate
properties to reduce Redrawing.
6. Not Using Animation Events
Explanation
JavaFX animations come with a range of events such as onFinished
, which can provide opportunities to perform actions after an animation completes.
Solution
Utilize events properly to improve interactivity and usability:
fadeTransition.setOnFinished(event -> {
myLabel.setText("Fade completed!");
});
7. Ignoring User Preferences
Explanation
Some users may have preferences regarding animations for accessibility reasons. Ignoring these can alienate users.
Solution
Consider providing options in your application settings to enable or disable animations.
Enhancing Your JavaFX Animation Skills
To further improve your JavaFX animation skills, consider exploring these resources:
- JavaFX Animation Documentation
- Oracle's JavaFX Tutorials
- JavaFX GitHub Repository
Key Takeaways
Mastering JavaFX animation requires an understanding of its fundamentals, along with awareness of common pitfalls. By following the solutions outlined in this post, you can create seamless, engaging animations that enhance your Java applications. Always remember to test your animations thoroughly and pay attention to user experience.
Continue practicing, exploring, and engaging with the vibrant JavaFX community. This journey will only enhance your skills and open doors to innovative application designs.
Feel free to share any common pitfalls you've encountered and how you've overcome them in the comments below. Happy coding!