Troubleshooting Common Apache Ignite Spring Data Issues
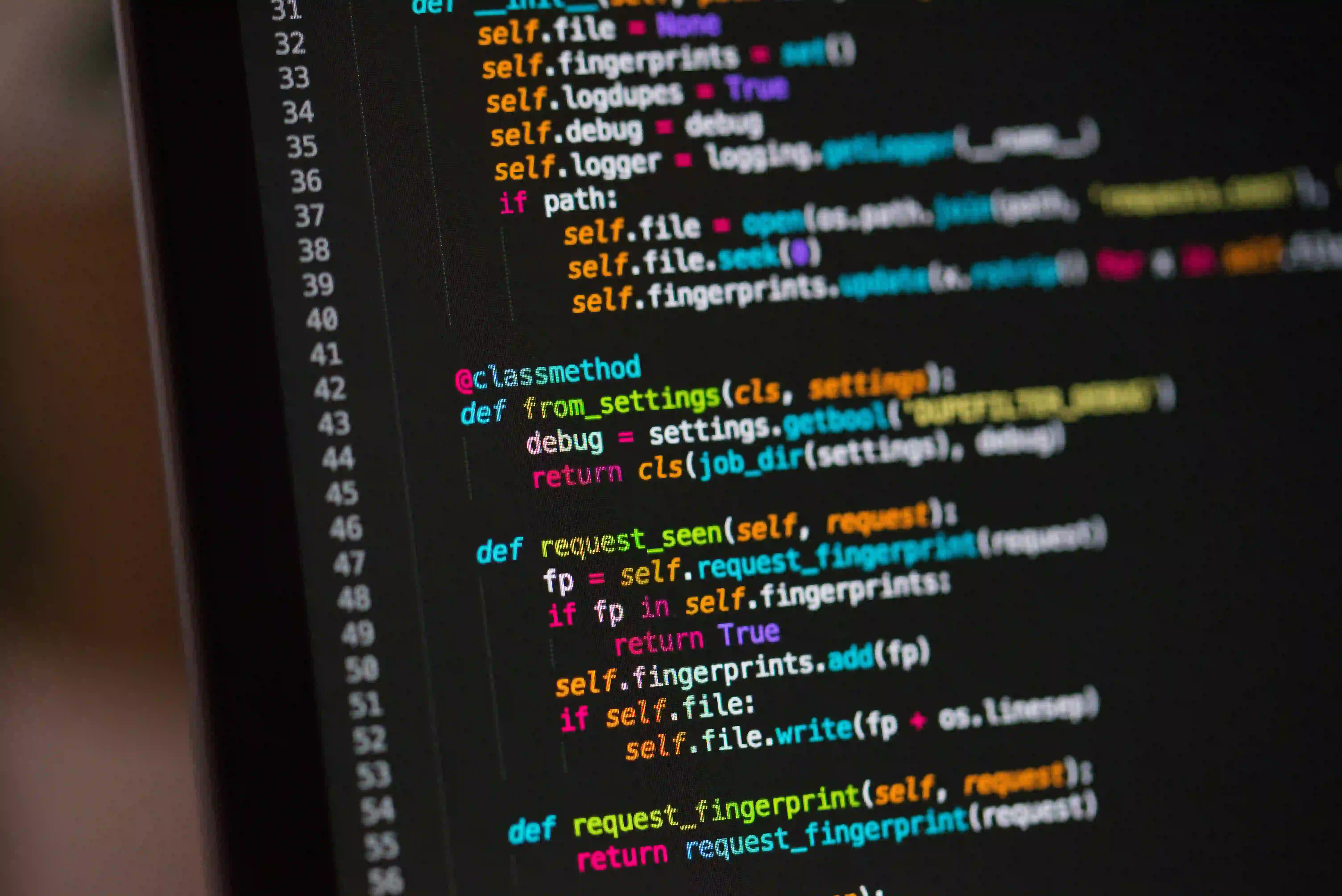
Troubleshooting Common Apache Ignite Spring Data Issues
Apache Ignite is an in-memory computing platform that can provide huge performance improvements over traditional database systems. When working with Apache Ignite alongside Spring Data, developers may run into various issues that can hinder development and application performance. This blog post will help you troubleshoot common problems encountered when working with Apache Ignite and Spring Data.
Why Use Apache Ignite with Spring Data?
Apache Ignite is widely appreciated for its speed, scalability, and versatility. By combining it with Spring Data, developers can benefit from a seamless integration that simplifies interactions with Ignite. Below are some key benefits of using Apache Ignite and Spring Data together:
- Speed: In-memory data storage allows for quick data retrieval.
- Scalability: Ignite can be clustered to handle varying loads.
- Ease of Use: Spring Data abstracts many complexities, allowing quicker development.
In this post, we will cover several common issues that may arise during integration and provide solutions to resolve them.
Issue 1: Connection Problems
Symptoms
When you try to start your Spring application, you may encounter connection issues that manifest as exceptions indicating that the Ignite cluster cannot be reached.
Troubleshooting Steps
-
Check Configuration: Ensure your
application.yml
orapplication.properties
file has the correct configuration for connecting to Ignite.⚙️snippet.ymlspring: cache: type: ignite data: ignite: uri: ignite-configuration.xml
The URI points to the configuration file where Ignite's cluster settings are defined.
-
Network Connectivity: Make sure that the Ignite nodes can communicate with each other. If you are running Ignite in a cloud environment, check if the required ports (default 10800 for client connections) are open.
-
Cluster State: Ensure the Ignite cluster is running and healthy. Use the Ignite Web Console or run scripts to verify the status of Ignite nodes.
Example Code Snippet: Configuration Class
Using a custom configuration class might provide better debugging insights.
@Configuration
@EnableIgniteRepositories
public class IgniteConfig {
@Bean
public Ignite igniteInstance() {
IgniteConfiguration cfg = new IgniteConfiguration();
// You can add your Ignite configurations here
return Ignition.start(cfg);
}
}
In this snippet, ensure that your Ignite configuration is properly initialized.
Issue 2: Entity Mapping Problems
Symptoms
You may face exceptions related to entity mapping when Spring Data attempts to read or write data.
Troubleshooting Steps
-
Check Annotations: Verify that your entity classes are annotated correctly. For example, using
@IgniteEntity
is crucial.☕snippet.java@IgniteEntity public class User { @Id private Long id; private String name; // Constructors, Getters, and Setters }
-
Ignite Configuration: Make sure you have set up the Ignite Working Directory with
binaryConfiguration
, which is key for storing metadata about your entities.☕snippet.javaIgniteConfiguration igniteCfg = new IgniteConfiguration(); BinaryConfiguration binaryCfg = new BinaryConfiguration(); binaryCfg.setCompactFooter(true); igniteCfg.setBinaryConfiguration(binaryCfg);
-
Database Compatibility: Validate that your entities are compatible with Ignite's data structure. Unsupported data types could lead to issues.
Example Code Snippet: Repository Definition
public interface UserRepository extends IgniteRepository<User, Long> {
List<User> findByName(String name);
}
This repository leverages Spring Data features to easily fetch entities. Ensure that the queries respect your entity structure.
Issue 3: Performance Issues
Symptoms
You may notice that queries are slow or that data retrieval times are not meeting expectations.
Troubleshooting Steps
-
Use Caching: Ensure that caching is enabled properly. You may also specify cache names in your repositories.
☕snippet.java@Cacheable("UserCache") public User findUserById(Long id) { // Method implementation }
-
Query Optimization: Make sure you are using efficient queries. Utilize Ignite's SQL capabilities to run optimized queries on your data.
☕snippet.javaString sql = "SELECT * FROM User WHERE name = ?"; try (IgniteDataStreamer<Long, User> dataStreamer = ignite.dataStreamer("User")) { dataStreamer.addData(1L, new User("John")); }
-
Monitor Resource Usage: Use Ignite’s management tools to monitor CPU and memory usage thoroughly. Look for bottlenecks and optimize accordingly.
Issue 4: Serialization Problems
Symptoms
You might see exceptions related to serialization or deserialization while persisting or retrieving data.
Troubleshooting Steps
-
Ensure Serializable Classes: Confirm that all your entity classes implement
Serializable
.☕snippet.javapublic class User implements Serializable { private static final long serialVersionUID = 1L; // Class body }
-
Use Binary Objects: Prefer Binary Objects for performance gains and to avoid serialization mismatches.
☕snippet.javaIgniteBiTuple<Long, String> userTuple = new IgniteBiTuple<>(1L, "John Doe");
-
Update Ignite Version: If you’re encountering serialization issues, consider updating to the latest version of Ignite, as improvements and fixes are continually made.
My Closing Thoughts on the Matter
Integrating Apache Ignite with Spring Data can significantly enhance your application's performance, yet it is not without its challenges. We’ve explored common issues surrounding connection problems, entity mapping, performance, and serialization intricacies. By systematically troubleshooting these areas, developers can effectively overcome obstacles and leverage the full power of Ignite.
Additional Resources
- Apache Ignite Documentation
- Spring Data Documentation
- Ignite SQL Queries
With these troubleshooting steps, you're well-equipped to tackle the most frequent issues that arise when working with Apache Ignite and Spring Data. Happy coding!