Common Pitfalls When Transferring InputStream to OutputStream in JDK 9
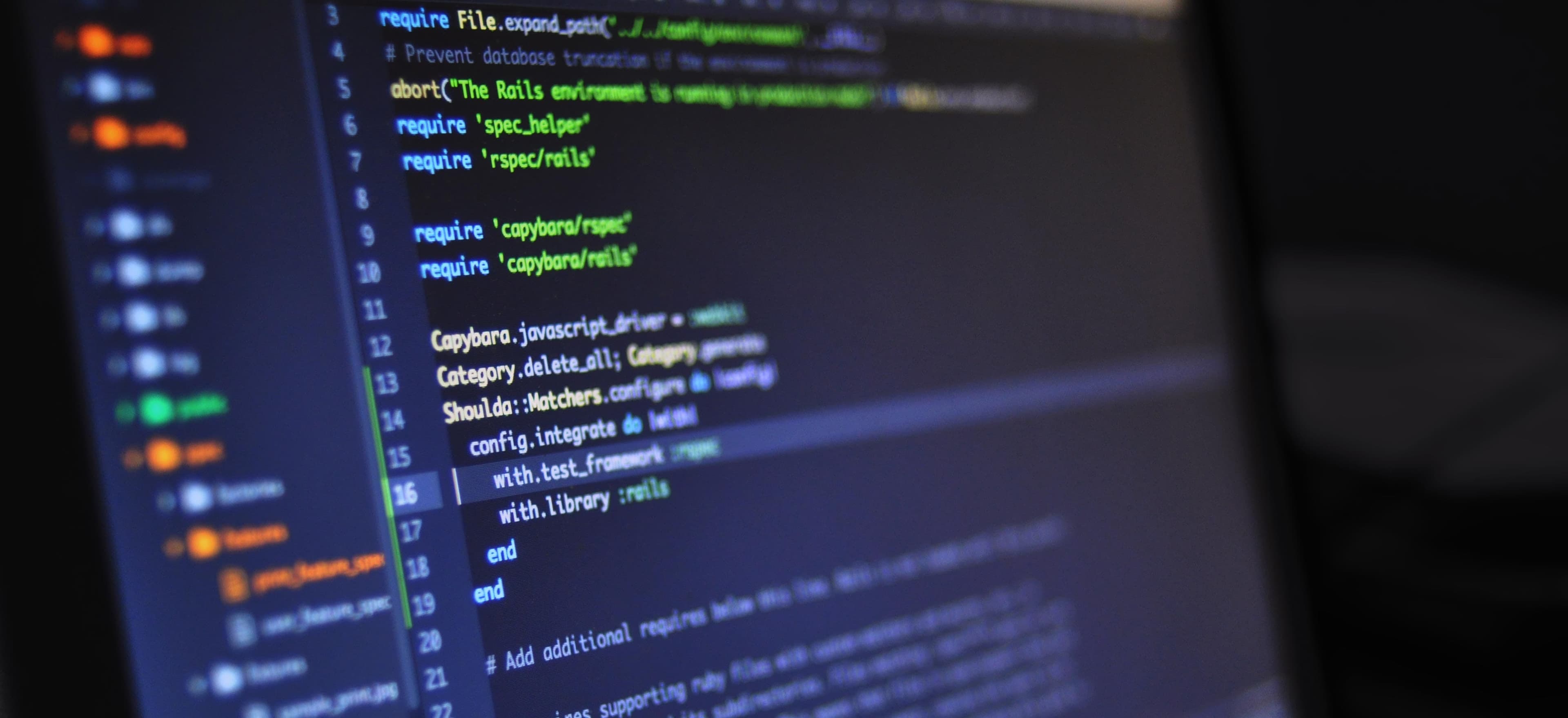
- Published on
Common Pitfalls When Transferring InputStream to OutputStream in JDK 9
In Java, transferring data from an InputStream
to an OutputStream
is a fundamental task often encountered in file handling, network communication, and other input-output operations. While this may seem straightforward, developers can run into several common pitfalls, especially when using Java Development Kit (JDK) 9 and its new features. In this blog post, we will explore these challenges, provide solutions, and offer best practices to ensure smooth data transfer.
Understanding the Basics
Before diving into the pitfalls, it's essential to understand the basics of InputStream
and OutputStream
.
- InputStream is designed for reading bytes from a source, such as a file or network stream.
- OutputStream, on the other hand, is used for writing bytes to a destination.
Here’s a simple example of using InputStream
and OutputStream
:
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class StreamTransfer {
public static void main(String[] args) {
String inputFile = "source.txt";
String outputFile = "destination.txt";
try (InputStream in = new FileInputStream(inputFile);
OutputStream out = new FileOutputStream(outputFile)) {
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = in.read(buffer)) != -1) {
out.write(buffer, 0, bytesRead);
}
} catch (IOException e) {
e.printStackTrace(); // Handle exceptions appropriately
}
}
}
This code reads bytes from "source.txt" and writes them to "destination.txt". The use of try-with-resources automatically closes the streams, preventing resource leaks.
Common Pitfalls
1. Not Closing Streams Properly
One of the most common mistakes is failing to close streams properly. While using try-with-resources as shown above is the recommended practice, not all developers do so.
Issue
If the InputStream
or OutputStream
isn’t closed, it can lead to resource leaks, which eventually cause memory issues.
Solution Always use try-with-resources to automate closing streams. This ensures that your streams are closed properly, even if an exception occurs.
2. Choosing the Wrong Buffer Size
The buffer size can significantly affect the performance of your I/O operation. A buffer that's too small may lead to too many read/write operations, while a very large buffer can waste memory and cause inefficiency.
Issue Using a very small buffer may slow down the transfer drastically.
Solution Typically, a buffer size of 4KB (4096 bytes) is a good starting point. Always measure performance and adjust according to your application's needs.
byte[] buffer = new byte[4096]; // More efficient buffer size
3. Ignoring Exceptions
Another frequent pitfall is ignoring exceptions, especially IOException
. While it is easy to suppress errors or log them without handling them correctly, doing so can create debugging nightmares.
Issue Ignoring exceptions can lead to silent failures which are challenging to trace.
Solution Always handle exceptions properly. Propagate exceptions if necessary or log them meaningfully.
catch (IOException e) {
System.err.println("Error during stream transfer: " + e.getMessage());
e.printStackTrace();
}
4. Not Flushing OutputStream
When writing data to an OutputStream
, it’s crucial to flush it, particularly when working with buffered streams. If you forget to flush, data may not be written immediately to the destination.
Issue Data may remain in the buffer and not actually get written, leading to data loss.
Solution If you're using a buffered output stream, manually flush it after writing data, or ensure that it gets flushed in the close method.
out.flush(); // Ensure data is written out
5. Using BufferedStream inappropriately
JDK 9 introduced enhancements, but using BufferedInputStream
or BufferedOutputStream
incorrectly can still create issues.
Issue Not wrapping streams appropriately can lead to inefficient reads/writes.
Solution Always wrap your streams as necessary:
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
// Buffered streams for better efficiency
try (BufferedInputStream in = new BufferedInputStream(new FileInputStream(inputFile));
BufferedOutputStream out = new BufferedOutputStream(new FileOutputStream(outputFile))) {
// Data transfer code
}
6. Assuming Stream Lengths Are Valid
There can be confusion around the lengths of streams. When transferring an InputStream
, the length is not always known, especially if working with ByteArrayInputStream
or a stream from a network.
Issue Assuming a fixed length can lead to incomplete data transfers.
Solution Always read until the end of the stream (EOF) is reached as shown in the initial example:
while ((bytesRead = in.read(buffer)) != -1) {
out.write(buffer, 0, bytesRead);
}
7. Not Handling Charset in Character Streams
When working with character streams (like Reader
and Writer
), it’s crucial to pay attention to character encoding.
Issue If the character encoding is not specified, it may lead to data corruption, especially when transferring text.
Solution Specify the encoding explicitly when creating input and output streams. For instance, using UTF-8:
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
// Example with character streams
try (Reader in = new InputStreamReader(new FileInputStream(inputFile), "UTF-8");
Writer out = new OutputStreamWriter(new FileOutputStream(outputFile), "UTF-8")) {
// Data transfer code
}
Best Practices for Efficient Stream Handling
- Use try-with-resources: Always use this structure to handle your streams and free up resources quickly.
- Choose Appropriate Buffer Sizes: Test to find the optimal size for your data transfer scenario.
- Handle Exceptions Carefully: Provide meaningful error messages and consider allowing exceptions to propagate where necessary.
- Utilize Buffering: Always wrap your streams in buffered wrappers to enhance performance.
- Specify Charset: Always specify your desired charset for character streams to avoid data corruption.
- Test & Measure Performance: Regularly profile your I/O operations to identify bottlenecks.
Final Considerations
Transferring data between InputStream
and OutputStream
in Java is a routine task, but a result of negligence can lead to significant issues. By following the best practices and being mindful of common pitfalls discussed in this article, you will ensure that your applications run smoothly and efficiently.
For further reading, you might find these resources helpful:
By understanding the intricacies of stream handling in JDK 9, you're better prepared to write robust and efficient Java applications. Happy coding!