Navigating 'this' and 'delegate' in Groovy Closures
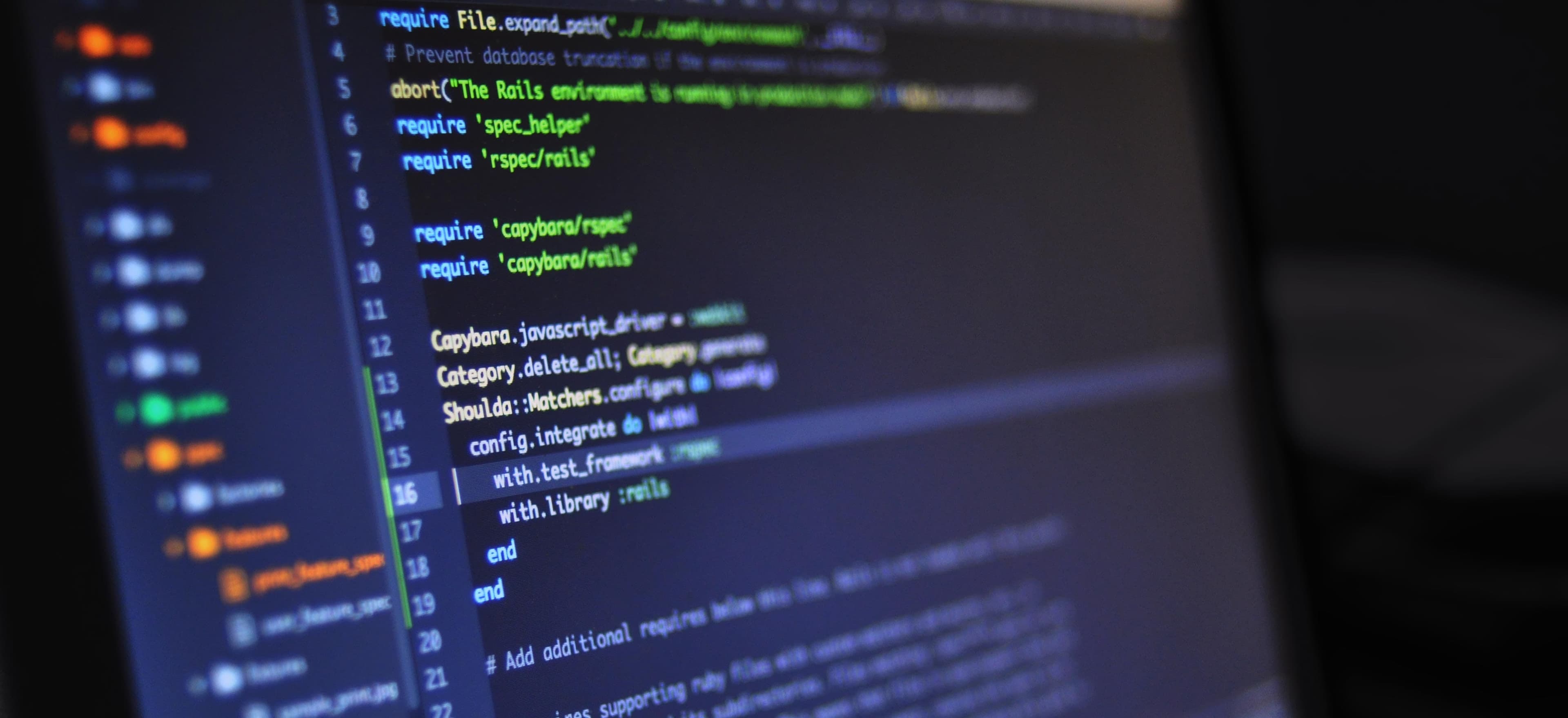
- Published on
Navigating 'this' and 'delegate' in Groovy Closures
When it comes to dynamic languages on the Java Virtual Machine, Groovy stands out for its expressiveness and ease of use. One of the most powerful features of Groovy is the use of closures, which are essentially code blocks that can be assigned to variables, passed around as parameters, or even returned from methods. Understanding how this
and delegate
work within closures can enhance your Groovy programming skills significantly. In this blog post, we will explore the intricacies of this
and delegate
to help you navigate Groovy closures effectively.
Understanding Closures in Groovy
Before we dive into this
and delegate
, let's take a moment to understand what a closure is. A closure in Groovy is defined using curly braces {}
and can take parameters and return values, much like a method. Closures can access variables defined in their surrounding context, which further adds to their flexibility.
A Simple Closure Example
def greetPerson = { String name ->
"Hello, $name!"
}
println greetPerson("Alice") // Output: Hello, Alice!
In this snippet, we define a closure greetPerson
that takes a name and returns a greeting. The beauty of closures lies in their ability to capture the surrounding lexical scope.
The Role of 'this' in Closures
What is 'this'?
In Groovy, this
refers to the current object instance. Within a closure, this
can point to different objects depending on where the closure is defined. Understanding how this
behaves is crucial to comprehending closures effectively.
Example of 'this' in Closure
class Person {
String name
Person(String name) {
this.name = name
}
def introduce() {
return {
"My name is ${this.name}."
}
}
}
def alice = new Person("Alice")
def introduction = alice.introduce()
println introduction() // Output: My name is Alice.
In this example, the closure defined in the introduce
method uses this
to refer to the Person
object. Therefore, when we call introduction()
, it successfully accesses the name
property of the alice
object.
When to be Cautious
Using this
can sometimes create confusion, especially for developers coming from other programming languages. If you define a closure inside another closure or method and expect this
to refer to the containing object, it might not behave as expected. Always keep in mind where the closure is defined.
The Role of 'delegate' in Closures
Understanding 'delegate'
Every closure in Groovy has a delegate
property, which allows you to set an alternative object for the closure to operate on. The delegate
can come in handy when you want to create DSLs (Domain Specific Languages) or APIs where the closure needs access to specific properties of another object.
Example of 'delegate' in Closure
class DSL {
String message = "Default Message"
def render(Closure closure) {
closure.delegate = this // Set delegate to the current DSL instance
closure()
}
}
def dsl = new DSL()
dsl.render {
println message // Accesses the `message` property of the DSL class
}
In this code snippet, we have a DSL class with a method render
. By assigning this
to the closure's delegate
, the closure can directly access properties of the DSL
instance, allowing for a more fluent and expressive way to define behavior.
Mixing 'this' and 'delegate'
Understanding the interplay between this
and delegate
is crucial. While this
always refers to the object where the closure is defined, delegate
can change based on your requirements. Here is an example to illustrate:
class OuterClass {
String outerProperty = "Outer Property"
def closureWithDelegate() {
def innerClosure = {
// This will access the `outerProperty` of `OuterClass`
println "From Outer: ${this.outerProperty}"
// This will access the property of the new delegate, if set
println "From Delegate: ${delegate.innerProperty}"
}
innerClosure.delegate = new InnerClass()
innerClosure()
}
}
class InnerClass {
String innerProperty = "Inner Property"
}
def outer = new OuterClass()
outer.closureWithDelegate()
Output:
From Outer: Outer Property
From Delegate: Inner Property
In this example, innerClosure
uses both this
and delegate
. It can access properties of OuterClass
through this
, while delegate
offers access to properties of InnerClass
.
Best Practices for Using 'this' and 'delegate'
-
Clarity First: Always prioritize clarity when using
this
anddelegate
. If you find yourself confused, refactor the code for better readability. -
Consistency: Maintain consistency in your use of
this
anddelegate
. This can help you and your team understand the flow of your code. -
Explicit Delegate: Use explicit delegate assignments when closures are intended to operate on different objects. This makes your intentions clear to anyone reading the code.
The Closing Argument
Understanding how this
and delegate
work within Groovy closures can elevate your coding skills and ability to write expressive, maintainable code. By taking advantage of closures, you unlock powerful patterns that can lead to more succinct and readable programs. Whether you are working on a small script or a larger Groovy application, these concepts will help you navigate the unique advantages Groovy offers.
For deeper insights into Groovy and closures, feel free to visit the official Groovy documentation and Groovy in Action for more comprehensive examples. Happy coding!