Mastering BTrace: Debugging Java With Ease
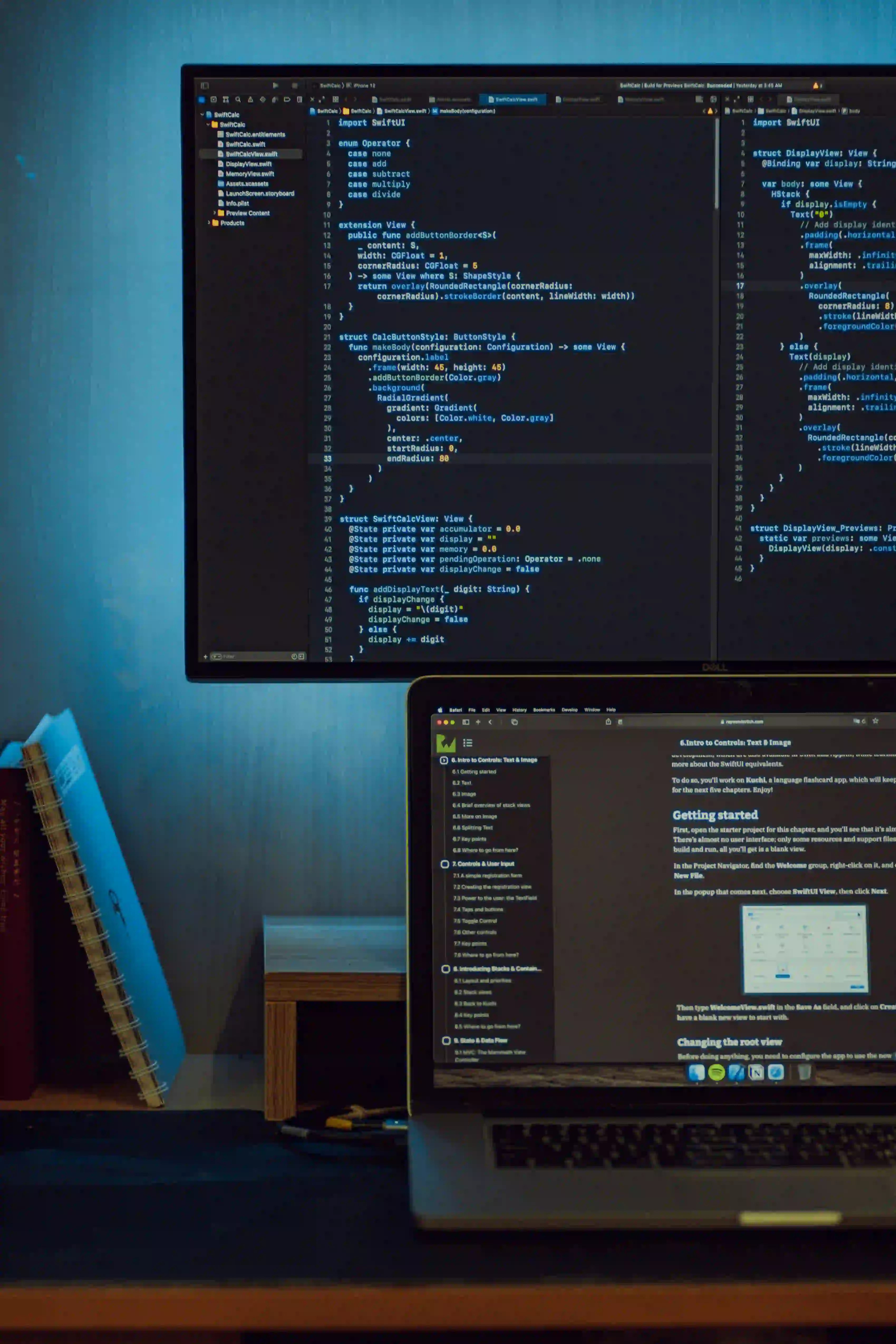
Mastering BTrace: Debugging Java With Ease
Debugging is an essential part of software development, especially in complex applications such as those built with Java. With increasing codebases, traditional debugging methods may prove cumbersome and ineffective. In this blog post, we will explore BTrace, a powerful tool designed for dynamic tracing of Java programs, which allows developers to probe running Java applications without altering their source code. We'll delve into its features, usage, and how it can make debugging a breeze.
What is BTrace?
BTrace is a dynamic tracing tool developed by the Oracle Corporation for Java applications. It allows developers to attach scripts to running JVMs (Java Virtual Machines) to monitor and analyze application behavior in real-time.
Why BTrace?
- Dynamic Instrumentation: BTrace can inject code into Java applications without the need for restarting them.
- No Source-Code Changes: You can trace applications without modifying the actual source code.
- Performance-Friendly: BTrace is built to have minimal impact on application performance.
- Rich API: It offers a rich set of functions to retrieve data with just a few lines of code.
Setting Up BTrace
Before we can use BTrace, we need to set it up. Follow these steps:
- Download BTrace: You can find it in the BTrace GitHub repository.
- Install Java: Ensure you have Java Development Kit (JDK) installed on your machine. BTrace works with JDK 7 and later versions.
- Configure Environment Variables: Set up your
JAVA_HOME
and ensure the BTracebin
directory is in your system'sPATH
.
Example Code Snippet
Here's a simple example of how to start using BTrace. First, let’s create a Java class that we want to trace.
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
for (int i = 0; i < 10; i++) {
compute(i);
}
}
public static int compute(int x) {
return x * x;
}
}
Now, we'll create a BTrace script to trace the compute
method execution.
The BTrace Script
Create a new BTrace script file called TraceHelloWorld.java
.
import com.sun.btrace.annotations.*;
import static com.sun.btrace.BTraceUtils.*;
@BTrace
public class TraceHelloWorld {
@OnMethod(
clazz = "HelloWorld",
method = "compute"
)
public static void onCompute(@Self Object self, int x) {
println(str("Called compute with argument: ") + str(x));
}
}
Explanation of the Script
- Annotations:
@BTrace
marks the class as a BTrace script. The@OnMethod
annotation specifies the class and method to trace. - Parameters: Using
@Self
gives us access to the instance of the object running the method. Here, we are tracing the argumentx
. - Output: The
println
function logs the argument each time thecompute
method is called.
Running BTrace
To run BTrace, compile the Java program and the BTrace script.
Compile HelloWorld.java
javac HelloWorld.java
Run HelloWorld
java HelloWorld
Attach BTrace to Running Application
In a new terminal window, run the following command to attach BTrace to the HelloWorld
program:
btrace <pid_of_helloworld> TraceHelloWorld.java
You can find the process ID (PID) using the jps
command, which lists all JVM processes currently running.
Output
As the compute
method is called, you should see output like this:
Called compute with argument: 0
Called compute with argument: 1
Called compute with argument: 2
...
Advanced Features of BTrace
Conditional Tracing
BTrace also allows for advanced filtering using conditions. For instance, you can log additional information when a specific condition is met.
@OnMethod(
clazz = "HelloWorld",
method = "compute",
permit = @Permit
)
public static void onComputeWithCondition(int x) {
if (x > 5) {
println(str("Called compute with argument greater than 5: ") + str(x));
}
}
Exception Handling
You can also trace exceptions by using the @OnThrow
annotation. This allows you to capture exceptions thrown in your application.
@OnThrow(
clazz = "HelloWorld",
method = "compute"
)
public static void onException(Throwable t) {
println(str("Exception thrown in compute: ") + str(t.getMessage()));
}
A Final Look
BTrace is a potent tool that can significantly ease the process of debugging Java applications. It stands out due to its ability to trace live applications with minimal performance penalties and without requiring any alteration of the source code.
In this blog post, we’ve explored basic setups, created BTrace scripts, and utilized advanced tracing features to help you master BTrace for Java debugging. By integrating BTrace into your development workflow, you can unlock a more efficient debugging experience.
Further Reading:
- Learn more about Java Performance Optimization
- Understand dynamic tracing tools through Oracle's Documentation
Feel free to share your experiences with BTrace in the comments below—whether it’s tips, code samples, or troubleshooting techniques. Happy debugging!