Mastering CDI Disposer Methods in Java EE: A How-To Guide
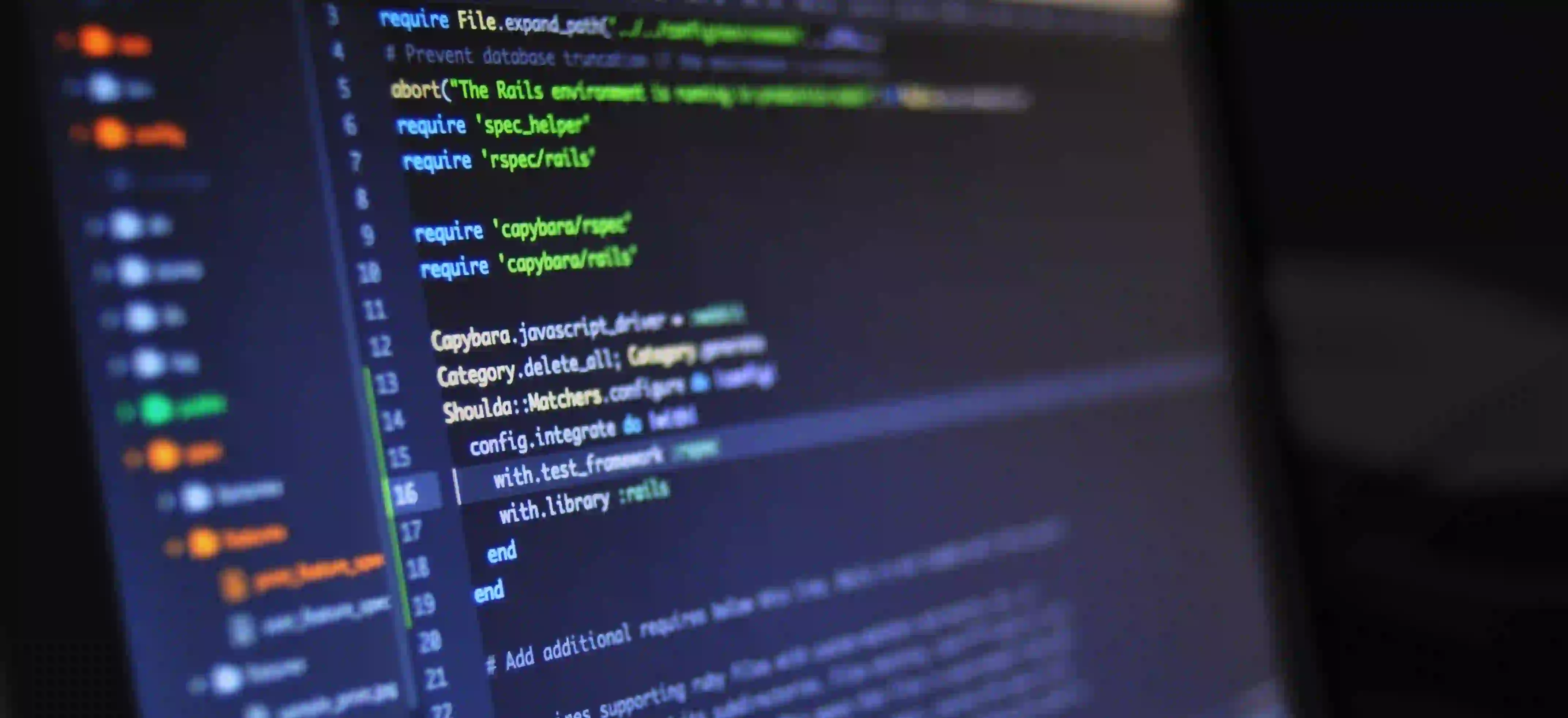
Mastering CDI Disposer Methods in Java EE: A How-To Guide
In the evolving landscape of Java Enterprise Edition (Java EE), Contexts and Dependency Injection (CDI) stands out as a critical API that enhances the modularity and manageability of applications. Among its various features, CDI's disposer methods play a pivotal role in resource management by clearing up managed resources. This blog post will guide you through the concept of CDI disposer methods, providing you with clear examples and code snippets that elucidate their usage and benefits.
What Are CDI Disposer Methods?
CDI features a powerful mechanism for managing the lifecycle of beans. Specifically, disposer methods are defined for cleaning up resources when beans are no longer needed. They are particularly useful for managing scope, ensuring that resources are released properly and avoiding memory leaks.
A disposer method is simply a method annotated with @Disposes
that receives the managed bean that is about to be destroyed. This allows you to perform any necessary clean-up tasks.
Why Use Disposer Methods?
-
Resource Management: They help facilitate proper resource management. For example, if you open a database connection, you can ensure that the connection is closed when the bean is no longer in use.
-
Lifecycle Control: Disposer methods give you fine-grained control over the lifecycle of beans and ensure that the application is efficient and responsive.
-
Code Clarity: It helps keep your code clean and easy to understand, as all the resource management is neatly encapsulated.
Creating a Simple CDI Example
Let’s craft a simple example to demonstrate how to use CDI disposer methods effectively. This example will include a managed bean that opens a resource and a disposer method that ensures proper cleanup when the resource is no longer needed.
Step 1: Set Up a Managed Bean
Create a class that manages a hypothetical resource, say a database connection.
import javax.annotation.PostConstruct;
import javax.annotation.PreDestroy;
import javax.enterprise.context.RequestScoped;
@RequestScoped
public class DatabaseConnection {
private String connection;
@PostConstruct
public void init() {
this.connection = "Opened database connection";
System.out.println(this.connection);
}
public String getConnection() {
return connection;
}
@PreDestroy
public void cleanup() {
this.connection = null;
System.out.println("Closed database connection");
}
}
Explanation:
@RequestScoped
specifies that this bean's lifecycle is tied to a single HTTP request.- The
@PostConstruct
method initializes the resource (simulating opening a database connection). - The
@PreDestroy
annotated method cleans up when the bean is about to be destroyed.
Step 2: Create a Disposer Method
Next, you will need a method to act as the disposer for the DatabaseConnection
bean. Here’s how you can implement it.
import javax.enterprise.inject.Disposes;
public class ConnectionManager {
public void dispose(@Disposes DatabaseConnection connection) {
connection.cleanup();
System.out.println("Disposing DatabaseConnection bean.");
}
}
Explanation:
- The
dispose
method is annotated with@Disposes
, signifying that it will be called when theDatabaseConnection
bean goes out of scope. - It will call the
cleanup()
method of theDatabaseConnection
to ensure that the resource is released.
Step 3: Integrating It All Together
You might want to use the CDI beans in a service or controller. Here’s how you’d typically inject the DatabaseConnection
.
import javax.inject.Inject;
import javax.enterprise.context.RequestScoped;
@RequestScoped
public class UserService {
@Inject
private DatabaseConnection databaseConnection;
public void performDatabaseOperations() {
System.out.println("Performing operations with: " + databaseConnection.getConnection());
// Implement actual database logic here.
}
}
Explanation:
- The
UserService
class uses the@Inject
annotation to get an instance ofDatabaseConnection
. This approach promotes loose coupling and easy testing.
Step 4: Testing the CDI Setup
To test the code:
- Set up your Java EE environment (e.g., using a server like Payara or WildFly).
- Deploy your application and observe the console logs to see the order of operations and confirm that the connections open and close as expected.
Best Practices for Using CDI Disposer Methods
- Always Clean Up: Ensure that every resource that is opened has a corresponding cleanup mechanism.
- Keep Logic Simple: The disposing methods should be straightforward. If you find that it is becoming complex, consider refactoring into smaller methods.
- Monitor Usage: Employ performance monitoring to ensure that the CDI is managing resources effectively.
Wrapping Up
Understanding and utilizing CDI disposer methods thoughtfully can lead to robust applications that manage their resources effectively. By encapsulating cleanup logic, you not only prevent resource leaks but also enhance the maintainability of your code. Experiment with these examples, and don’t shy away from extending them to fit your application’s unique requirements.
For more on Java EE and CDI, consider exploring the official Java EE Documentation.
Further Reading
- Introduction to Contexts and Dependency Injection (CDI)
- Understanding the Java EE Lifecycle
Feel free to reach out with any questions or shared experiences regarding CDI. Happy coding!