Common Pitfalls When Building SOAP Proxies in Spring
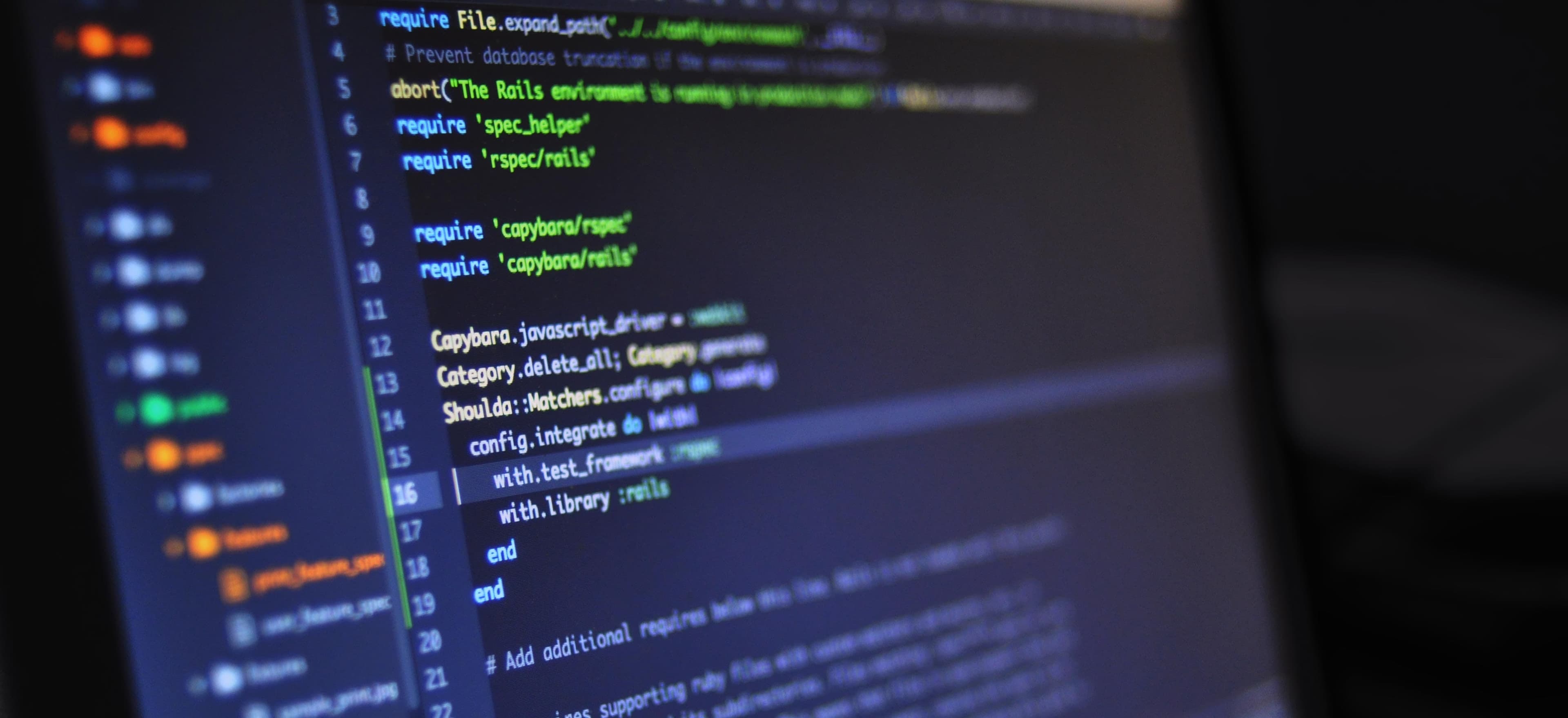
- Published on
Common Pitfalls When Building SOAP Proxies in Spring
In today’s world of microservices and integration, SOAP (Simple Object Access Protocol) remains an essential technology in many enterprise environments. When integrating SOAP services into your Spring applications, crafting a SOAP proxy can seem straightforward. However, several common pitfalls may jeopardize the integrity and performance of your integration. This blog post will discuss these pitfalls and provide best practices, ensuring that you avoid common traps when building SOAP proxies in Spring.
Understanding SOAP and Its Relevance
SOAP is a protocol for exchanging structured information in web services. Its extensibility and robustness make it suitable for enterprise scenarios. While REST has gained in popularity, SOAP is still heavily utilized for enterprise deployments due to its support for complex transactions and reliable messaging.
If you're new to SOAP and want to understand its inner workings, I recommend reading the SOAP Web Services Documentation for a foundational understanding.
Setting Up your Spring SOAP Proxy
Before diving into the pitfalls, let’s set up a basic SOAP proxy in Spring. You need to have the following dependencies in your pom.xml
file if you are using Maven:
<dependency>
<groupId>org.springframework.ws</groupId>
<artifactId>spring-ws-core</artifactId>
<version>3.0.10</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web-services</artifactId>
<version>3.0.10</version>
</dependency>
With these dependencies in place, you can create your service.
1. Creating the Service Endpoint
Here’s an example of how to create a SOAP proxy for a fictional currency conversion service. The end goal is to enable our Spring application to communicate with this SOAP service.
import org.springframework.ws.client.core.support.WebServiceGatewaySupport;
import org.springframework.ws.soap.client.core.SoapActionCallback;
public class CurrencyConversionClient extends WebServiceGatewaySupport {
public CurrencyConversionResponse convertCurrency(String from, String to, double amount) {
ConvertCurrency request = new ConvertCurrency();
request.setFrom(from);
request.setTo(to);
request.setAmount(amount);
CurrencyConversionResponse response = (CurrencyConversionResponse) getWebServiceTemplate()
.marshalSendAndReceive("http://www.example.com/ws/currency", request,
SoapActionCallback.create("http://www.example.com/ws/convertCurrency"));
return response;
}
}
Commentary on Code
In this code snippet, we extend the WebServiceGatewaySupport
class to use Spring's WebServiceTemplate
. The convertCurrency
method crafts a request and sends it to the specified endpoint. The SoapActionCallback
indicates the action in the XML payload. This encapsulation is critical as it abstracts the complexities of working with SOAP while streamlining the communication process.
Common Pitfalls
1. Not Handling WSDL Changes
Problem: SOAP services often evolve, and the WSDL (Web Services Description Language) might change. Not continuously validating against the WSDL can lead to runtime errors or mismatched data structures.
Solution: Always regenerate your client stubs whenever there are WSDL changes. Leveraging tools like Apache CXF or JAX-WS ensures your generated classes stay updated with the latest service description.
2. Ignoring Fault Handling
Problem: SOAP services may fail due to various reasons, such as network issues or service downtime. Not properly catching SOAP faults can lead to application crashes.
Solution: Wrap your service calls in try-catch blocks to handle exceptions gracefully. You can extend WebServiceGatewaySupport
and create a custom error handler for better logging and debugging.
try {
CurrencyConversionResponse response = convertCurrency("USD", "EUR", 100);
// process the response
} catch (SoapFaultClientException e) {
// Handle SOAP Fault
System.err.println("SOAP Fault occurred: " + e.getMessage());
}
3. Neglecting Security Aspects
Problem: Security is often an afterthought. Services may need credentials or specific headers for validation.
Solution: Use Spring Security with your WebServiceTemplate
to enforce WS-Security. This ensures that the tokens are automatically included with your requests.
@Bean
public WebServiceTemplate webServiceTemplate() {
WebServiceTemplate webServiceTemplate = new WebServiceTemplate();
webServiceTemplate.setInterceptors(new ClientInterceptor[]{new WsSecurityInterceptor()});
return webServiceTemplate;
}
4. Performance and Timeout Issues
Problem: Improper configuration for timeouts and buffer sizes may lead your application to hang indefinitely.
Solution: Configure your WebServiceTemplate
with appropriate timeout settings:
webServiceTemplate.setRequestFactory(new HttpComponentsMessageFactory());
HttpComponentsMessageFactory messageFactory = (HttpComponentsMessageFactory) webServiceTemplate.getMessageFactory();
messageFactory.setConnectionTimeout(5000); // 5 seconds
messageFactory.setReadTimeout(5000); // 5 seconds
5. Log Management
Problem: SOAP services can produce verbose logs, especially during debugging. Without proper log management, these logs can get overwhelming and unmanageable.
Solution: Leverage SLF4J with Logback or Log4j. Implement structured logging to clarify log outputs.
logger.info("Request for currency conversion from {} to {} for amount {}", from, to, amount);
6. Ignoring XML Namespace Issues
Problem: XML namespaces often lead to serialization issues if not managed properly.
Solution: Make sure to set your XStream or JAXB context with the correct namespaces. This is particularly apparent when dealing with complex XML responses.
Testing Your SOAP Proxy
Testing SOAP proxies is crucial for ensuring they work correctly. Tools like SoapUI or Postman allow you to send requests and view responses effectively. Additionally, unit tests in conjunction with tools like Mockito can validate your proxy behaviors.
Example Test Case
import static org.mockito.Mockito.*;
import org.junit.jupiter.api.Test;
public class CurrencyConversionClientTest {
@Test
public void testConvertCurrency() {
CurrencyConversionClient client = mock(CurrencyConversionClient.class);
CurrencyConversionResponse mockResponse = new CurrencyConversionResponse();
mockResponse.setConvertedAmount(85);
when(client.convertCurrency("USD", "EUR", 100)).thenReturn(mockResponse);
CurrencyConversionResponse response = client.convertCurrency("USD", "EUR", 100);
assertEquals(85, response.getConvertedAmount());
}
}
Commentary on Testing
This test uses Mockito to simulate the behavior of our CurrencyConversionClient
. By isolating the service layer, we can verify our logic without making actual SOAP calls. This boosts performance and reliability in our testing suite.
Closing the Chapter
While building SOAP proxies in Spring can be straightforward, ignoring these common pitfalls can lead to issues that compromise your system's effectiveness. Always remember to keep your WSDL updated, handle faults gracefully, prioritize security, manage performance, maintain logging, and deal with XML namespaces effectively.
By adhering to these best practices, you will build robust and maintainable SOAP proxies in Spring that can handle the complexities of enterprise-level applications while ensuring a smooth and error-free integration experience. For further reading on building SOAP web services, explore the official Spring Web Services Documentation.
Following these guidelines will not only help you avoid pitfalls but also arm you with the knowledge required for developing reliable SOAP integrations. Happy coding!
Checkout our other articles