Mastering Command Line Argument Parsing in Java
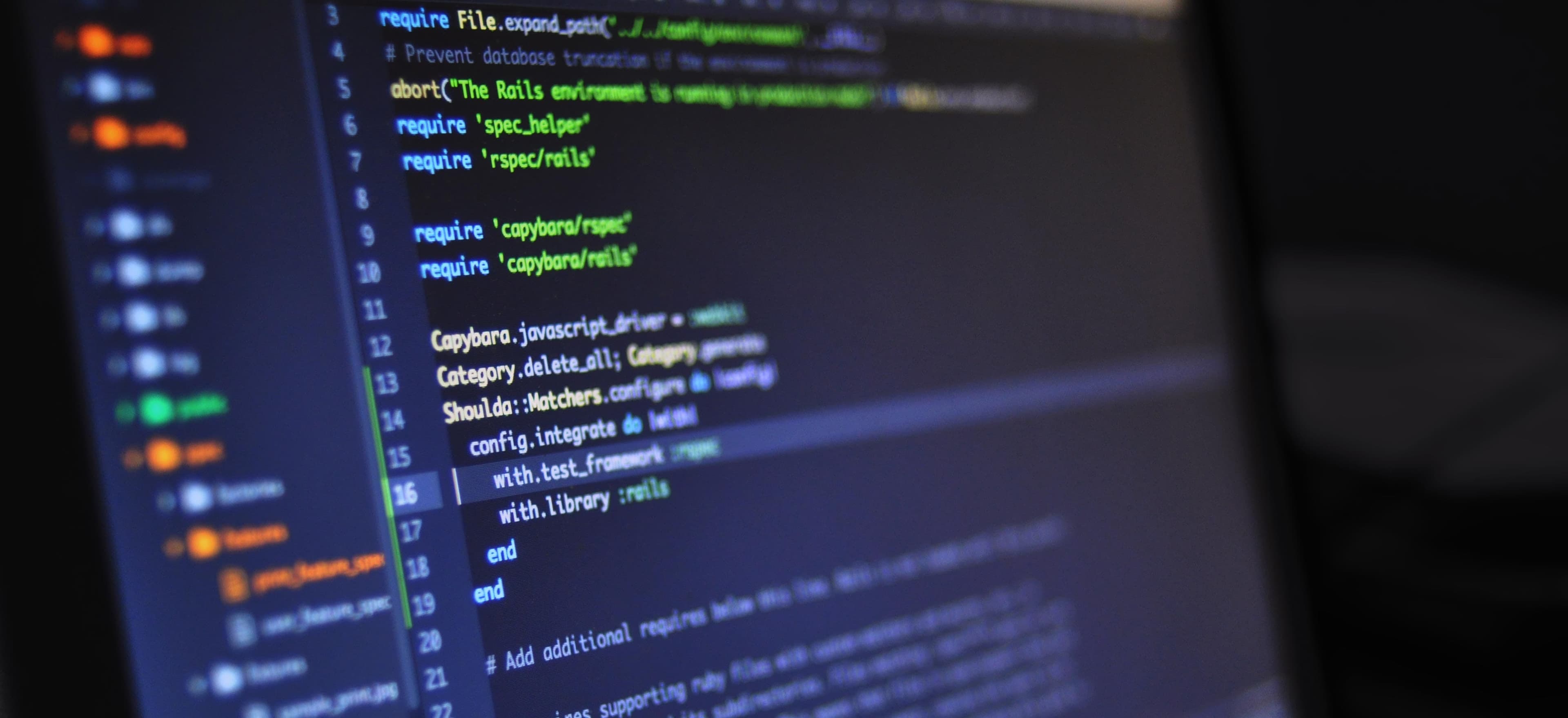
- Published on
Mastering Command Line Argument Parsing in Java
Command line arguments are essential for any Java application that requires user input at runtime. Whether you are building a utility tool, a desktop application, or a server-side application, mastering command line argument parsing in Java is a vital skill. It enables your Java programs to accept user input directly from the command line, enhancing usability and versatility.
In this blog post, we will explore:
- Understanding command line arguments.
- Common techniques for parsing command line arguments in Java.
- Practical examples using Java's built-in capabilities and third-party libraries.
Let’s dive in!
Understanding Command Line Arguments
In Java, command line arguments are passed to the main
method:
public static void main(String[] args) {
// Your code here
}
The args
parameter is an array of String
that contains each argument provided after the class name. For example, running:
java YourClass arg1 arg2 arg3
will give you the array args
containing ["arg1", "arg2", "arg3"]
.
Basic Parsing Techniques
-
Basic Array Indexing: The simplest way to access command line arguments is through direct indexing:
public class ArgParser { public static void main(String[] args) { if (args.length == 0) { System.out.println("No arguments provided"); return; } System.out.println("First argument: " + args[0]); } }
In this code snippet, we check if any arguments are supplied and print the first one. This approach is straightforward but can lead to issues if the input isn't validated correctly.
-
Using a Loop: For applications that need to iterate through arguments, a loop can be used:
public class ArgLoop { public static void main(String[] args) { for (String arg : args) { System.out.println("Argument: " + arg); } } }
This is efficient for retrieving and processing multiple arguments.
Input Validation
It's crucial to validate the input we receive before using it in our applications. You should check for required arguments and ensure they meet necessary criteria:
public class InputValidation {
public static void main(String[] args) {
if (args.length < 2) {
System.out.println("Two arguments required: <name> <age>");
return;
}
String name = args[0];
int age;
try {
age = Integer.parseInt(args[1]);
System.out.println("Name: " + name + ", Age: " + age);
} catch (NumberFormatException e) {
System.out.println("Age must be a number.");
}
}
}
Here, we've introduced validation by checking the number of arguments and ensuring age
is a valid integer.
More Advanced Parsing Techniques
For larger applications, a more structured approach to parsing command line arguments becomes necessary. One popular solution is to use libraries like Apache Commons CLI, which simplifies the process dramatically.
Using Apache Commons CLI
Apache Commons CLI helps organize and structure arguments with a powerful API. First, you need to add the dependency:
For Maven users, add the following to your pom.xml
:
<dependency>
<groupId>commons-cli</groupId>
<artifactId>commons-cli</artifactId>
<version>1.4</version>
</dependency>
Example of Apache Commons CLI
Here’s an example of how to use Apache Commons CLI:
import org.apache.commons.cli.*;
public class CommonsCliExample {
public static void main(String[] args) {
Options options = new Options();
options.addOption("n", "name", true, "Your name");
options.addOption("a", "age", true, "Your age");
CommandLineParser parser = new DefaultParser();
try {
CommandLine cmd = parser.parse(options, args);
if (cmd.hasOption("n") && cmd.hasOption("a")) {
String name = cmd.getOptionValue("n");
int age = Integer.parseInt(cmd.getOptionValue("a"));
System.out.println("Name: " + name + ", Age: " + age);
} else {
HelpFormatter formatter = new HelpFormatter();
formatter.printHelp("CommonsCliExample", options);
}
} catch (ParseException e) {
System.out.println("Failed to parse command line properties: " + e.getMessage());
}
}
}
Explanation of the Code:
- Options Creation: We define our options using the
Options
class. - Parser: We create a parser instance to parse command line arguments.
- Command Line Check: We check if the required options are provided.
- Help Message: If the user needs help, we provide a formatted message.
This structured approach not only simplifies usage but also improves readability.
Using Typer Library
Another advanced library for managing command line arguments in Java is Typer, which is relatively new but gaining popularity for its usability and ease of integration with existing codebases.
Example with Typer
Add Typer dependency:
<dependency>
<groupId>com.github.typer</groupId>
<artifactId>typer-core</artifactId>
<version>1.1.0</version>
</dependency>
Using Typer can create more user-friendly options:
import com.github.typer.core.Command;
import com.github.typer.core.Main;
public class TyperExample {
@Command(name = "TyperExample", description = "Example of argument parsing with Typer")
public void run(String name, int age) {
System.out.println("Name: " + name + ", Age: " + age);
}
public static void main(String[] args) {
new Main().run(new TyperExample(), args);
}
}
Wrapping Up
Understanding command line argument parsing is crucial for developing robust Java applications. Whether you choose basic techniques, third-party libraries like Apache Commons CLI, or a newer solution like Typer, each method has its advantages.
For further reading, consider also checking out the official Java documentation on command-line arguments and Apache Commons CLI documentation.
By using the outlined methods, you can effortlessly create powerful Java applications that accept user input directly from the command line.
Happy coding!
Checkout our other articles