Are Unit Tests for Data Access Code Really Worth It?
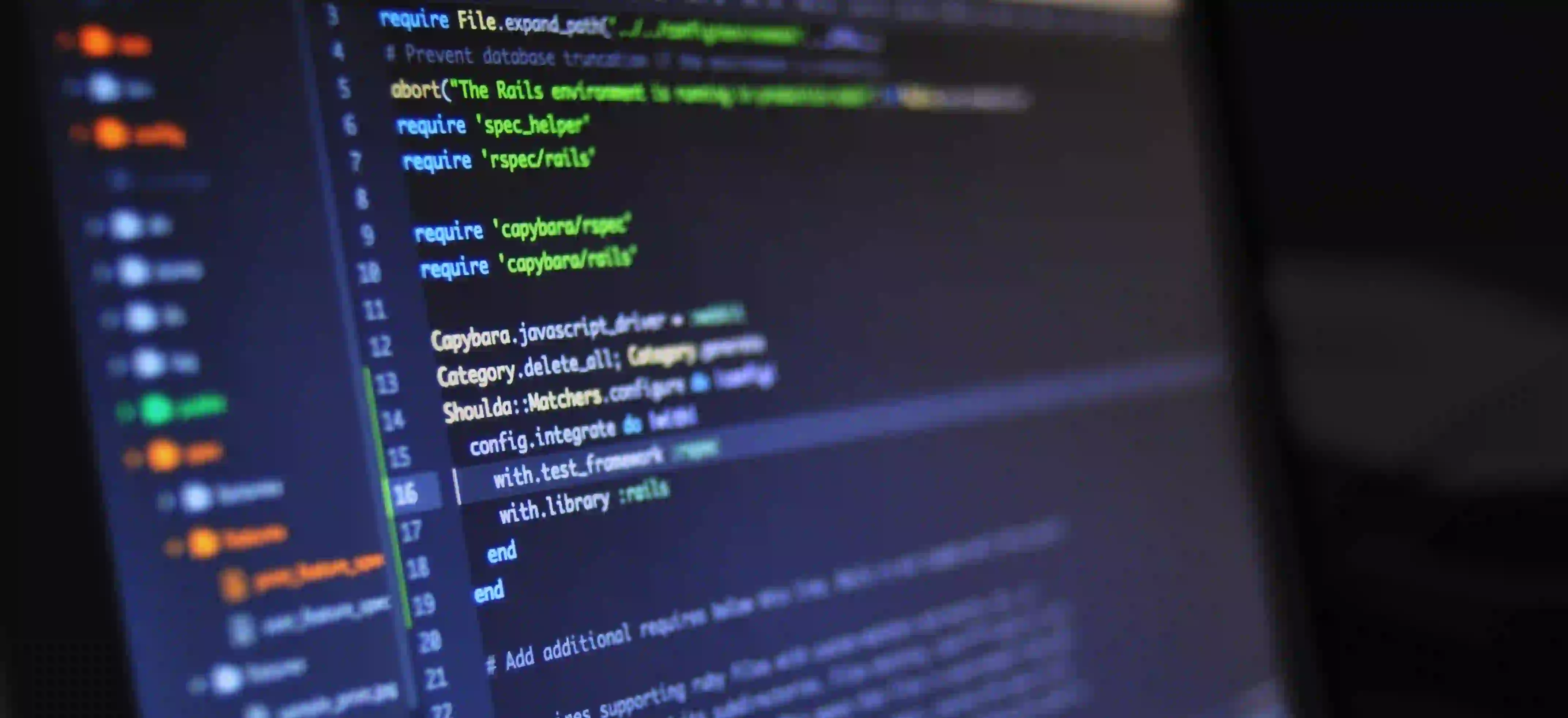
Are Unit Tests for Data Access Code Really Worth It?
When developing software applications, the question of whether to write unit tests for data access code can spark a heated debate among developers. On one side, you have proponents who argue that unit tests are essential for maintaining code quality and ensuring reliability. On the other hand, some see it as unnecessary overhead. This blog post will delve into the pros and cons of unit testing data access code, while providing you with real-world examples and best practices in Java.
Understanding Data Access Code
Before we jump into the discussion on unit tests, let's briefly define what data access code entails. Data access code primarily includes the methods and functions that interact with a database to perform operations such as creating, reading, updating, or deleting data—commonly referred to as CRUD operations.
In Java, data access code is often managed using data access objects (DAOs) or repositories. These abstractions help separate database operations from business logic, promoting a clean architecture. As such, ensuring that this code is reliable is critical for the overall stability of your application.
Importance of Unit Testing
Unit tests are written to validate individual units of source code, ensuring that each function or method behaves as expected. Their significance can be summarized in five key points:
-
Catch Bugs Early: Writing unit tests enables you to identify bugs at an early stage, thus reducing the cost and effort involved down the line.
-
Facilitate Refactoring: With a solid suite of unit tests, you can refactor your data access code with confidence, knowing that your tests will catch any errors introduced during the process.
-
Document Code Behavior: Unit tests serve as documentation for future developers, making it easier to understand what the code is supposed to do.
-
Ensure Code Quality: Regularly running unit tests helps maintain code quality by enforcing discipline in your coding practices.
-
Improve Design: When writing unit tests, you're often forced to write more modular, decoupled code, which leads to better design overall.
Arguments Against Unit Testing Data Access Code
Despite the advantages of unit testing, some developers argue against writing tests for data access code. Here are the commonly cited reasons:
-
Overhead: Writing tests takes time, and in fast-paced development environments, this can be seen as a burden.
-
Complexity: Setting up mocks and stubs for databases can add complexity to tests and lead to fragility.
-
Integration Testing Sufficiency: Some developers argue that integration tests—which test the interaction between various modules—are sufficient for database code.
-
Cost of Maintenance: Tests must be maintained alongside the code; frequent changes can lead to a bloated test suite.
However, while these arguments hold some merit, they should not overshadow the significant benefits of unit testing data access code.
Key Advantages of Unit Testing Data Access Code
To better understand why unit tests for data access code are worth implementing, let’s explore some specific advantages:
1. Isolation of Database Logic
Unit tests allow you to isolate your database logic from the rest of your application. This separation is particularly advantageous because it reduces the risks of cascading failures in larger applications. For example, suppose you have a method that fetches user information from the database:
public User getUserById(int id) {
String query = "SELECT * FROM users WHERE id = ?";
// Logic to execute query and map result to User entity
}
Without unit tests, if changes in the database schema occur, you may only discover issues during integration testing—resulting in lengthy debugging sessions or unexpected runtime exceptions.
2. Testing Edge Cases
Unit tests allow you to effectively test edge cases that might only rarely occur during production. For instance, consider the following scenario where a user is not found:
@Test
public void testGetUserByIdNotFound() {
assertNull(userDao.getUserById(999)); // Assuming 999 does not exist
}
Unit tests like this enable developers to catch such potential scenarios ahead of time, ensuring robustness in your application.
3. Enhancing Code Quality and Documentation
Unit tests can act as living documentation for your methods. Developers looking at the data access methods can easily understand their expected behavior through the associated tests.
4. Continuous Integration Benefits
As part of a continuous integration pipeline, automated unit tests ensure that new code does not break existing functionality. If a developer changes a data access method, running unit tests can immediately verify the change's impact.
Code Example: Unit Testing Data Access Code
Let’s put what we've discussed into practice with an example of unit testing data access code in Java. We will use JUnit for our tests and Mockito for mocking dependencies.
The DAO Class
Here’s a simple UserDAO class for fetching users from the database:
public class UserDAO {
private DataSource dataSource;
public UserDAO(DataSource dataSource) {
this.dataSource = dataSource;
}
public User getUserById(int id) {
User user = null;
String query = "SELECT * FROM users WHERE id = ?";
try (Connection conn = dataSource.getConnection();
PreparedStatement stmt = conn.prepareStatement(query)) {
stmt.setInt(1, id);
ResultSet rs = stmt.executeQuery();
if (rs.next()) {
user = new User(rs.getInt("id"), rs.getString("name"));
}
} catch (SQLException e) {
e.printStackTrace(); // In real code, you'd handle this properly
}
return user;
}
}
The Unit Test Class
Now, let’s write a unit test for the getUserById
method in the UserDAO
class:
import static org.mockito.Mockito.*;
import static org.junit.Assert.*;
import org.junit.Before;
import org.junit.Test;
import org.mockito.Mock;
import org.mockito.MockitoAnnotations;
import javax.sql.DataSource;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
public class UserDAOTest {
@Mock
private DataSource dataSource;
@Mock
private Connection connection;
@Mock
private PreparedStatement preparedStatement;
@Mock
private ResultSet resultSet;
private UserDAO userDAO;
@Before
public void setUp() throws Exception {
MockitoAnnotations.openMocks(this);
userDAO = new UserDAO(dataSource);
}
@Test
public void testGetUserById() throws Exception {
when(dataSource.getConnection()).thenReturn(connection);
when(connection.prepareStatement(any())).thenReturn(preparedStatement);
when(preparedStatement.executeQuery()).thenReturn(resultSet);
when(resultSet.next()).thenReturn(true);
when(resultSet.getInt("id")).thenReturn(1);
when(resultSet.getString("name")).thenReturn("John Doe");
User user = userDAO.getUserById(1);
assertNotNull(user);
assertEquals(1, user.getId());
assertEquals("John Doe", user.getName());
}
}
Commentary on the Test
In this test case, we are leveraging Mockito to create mock objects for DataSource
, Connection
, PreparedStatement
, and ResultSet
. This allows us to simulate interaction with the database without the need for an actual database connection.
This method promotes the idea of testing behavior rather than implementation. If we later decide to change the internal workings of our getUserById
method, our tests would continue to validate that the method still adheres to the expected behavior.
The Bottom Line
While there are valid arguments against unit testing data access code, the benefits far outweigh the drawbacks. Incorporating unit tests into your data access layer can lead to better code quality, improved documentation, and help avoid costly bugs.
In software development, the goal is not merely to write code but to write robust, maintainable code. Unit tests for data access code serve as an essential instrument in achieving this aim.
Whether you are part of a large team or a solo developer, consider integrating unit tests into your coding practices. The initial investment in time will pay off exponentially in the longevity and reliability of your software.
For further reading on unit testing in Java, consider checking out the following resources:
By adopting good unit testing practices, you will foster a healthier codebase and a smoother development process. Test it, trust it!