Mastering State Machines: Avoiding Common Design Pitfalls
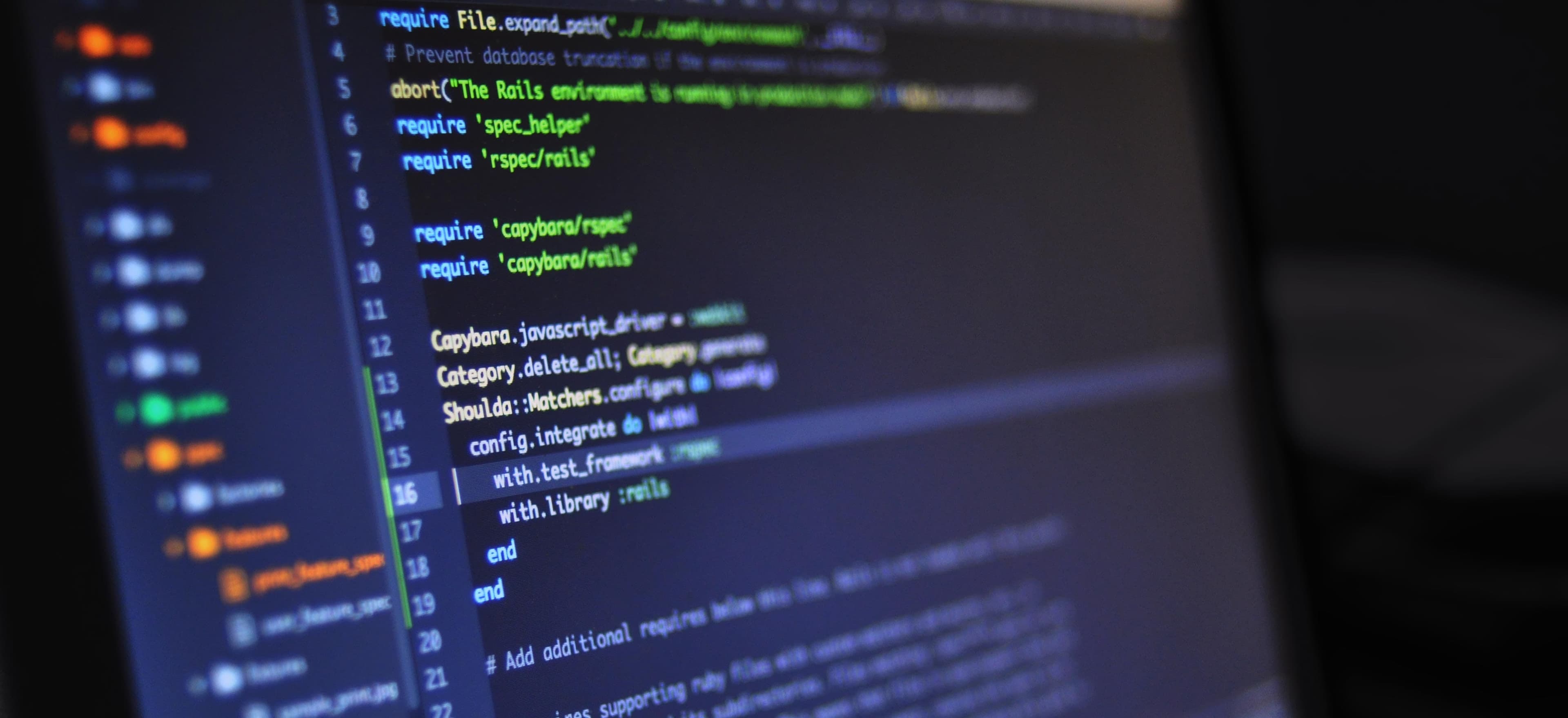
- Published on
Mastering State Machines: Avoiding Common Design Pitfalls
State machines are powerful tools in software design, especially for managing system states and transitions. Yet, despite their utility, developers can stumble into common design pitfalls that hinder performance and maintainability. In this blog post, we will explore the fundamentals of state machines, common missteps, and best practices to help you master this vital design pattern.
What is a State Machine?
A state machine is a design pattern used to define a system in terms of its states and the transitions between those states. It operates on a finite number of conditions:
- States: The distinct stages in which the system can exist.
- Transitions: The rules that dictate how states change in response to inputs or events.
Why Use State Machines?
State machines make it easier to model complex behavior. They provide:
- Clarity: States and transitions are well-defined, simplifying the understanding of a system's logic.
- Ease of Maintenance: With a structured approach, modifying states or transitions becomes more straightforward.
- Debugging: Tracking behavior through states simplifies issue identification.
Common Design Pitfalls
While designing state machines, developers often encounter certain pitfalls:
1. Overcomplicating State Definitions
Problem
One mistake is creating too many states, leading to convoluted transitions and confusion. For example, consider a simple online order system. You might be tempted to define separate states for every status change, like "Pending Payment," "Payment Confirmed," "Order Processing," etc.
Solution
Minimize state definitions and group similar states when possible. A simplified state machine might just include three major states: "Pending," "Active," and "Completed."
enum OrderState {
PENDING, ACTIVE, COMPLETED
}
class Order {
private OrderState state;
public void setState(OrderState state) {
this.state = state;
}
public OrderState getState() {
return state;
}
}
// Usage
Order order = new Order();
order.setState(OrderState.PENDING);
Why this works: A simpler state machine enhances readability and maintainability, making it easier to follow the order’s lifecycle.
2. Ignoring State Transition Logic
Problem
Another common issue arises when transition logic is buried deep in the code, making it hard to track and manage. When mixing state logic with application logic, you make your code less cohesive.
Solution
Encapsulate transitions in dedicated methods within the state machine. This will separate concerns and improve readability.
class Order {
private OrderState state;
public void pay() {
if (state == OrderState.PENDING) {
state = OrderState.ACTIVE;
System.out.println("Payment confirmed, order is now active.");
}
}
public void complete() {
if (state == OrderState.ACTIVE) {
state = OrderState.COMPLETED;
System.out.println("Order completed.");
}
}
public OrderState getState() {
return state;
}
}
// Usage
Order order = new Order();
order.setState(OrderState.PENDING);
order.pay();
order.complete();
Why this works: This approach keeps the transition logic clear and centralized, making it easier to manage changes in state functionality.
3. Failing to Implement Guards
Problem
If you allow any transition to occur without checks, the state machine can enter an invalid state. For instance, in our order system, you should not allow an order to be marked as "Completed" without being "Active" first.
Solution
Add guard clauses to ensure valid transitions.
public void complete() {
if (state == OrderState.ACTIVE) {
state = OrderState.COMPLETED;
System.out.println("Order completed.");
} else {
throw new IllegalStateException("Cannot complete the order unless it is active.");
}
}
Why this works: Guard clauses help enforce rules within your state transitions, preventing invalid operations that can compromise system integrity.
4. Neglecting to Handle State Entry/Exit
Problem
Overlooking state entry and exit behavior can yield unexpected results. For example, additional setup or cleanup actions might be necessary when entering or exiting a state.
Solution
Implement hooks for entering and exiting states to manage necessary state-specific operations.
class Order {
private OrderState state;
public void pay() {
if (state == OrderState.PENDING) {
enterActiveState();
}
}
private void enterActiveState() {
// Perform necessary actions upon entering the ACTIVE state
state = OrderState.ACTIVE;
System.out.println("Payment confirmed, order is now active.");
}
public void complete() {
if (state == OrderState.ACTIVE) {
exitActiveState();
}
}
private void exitActiveState() {
// Clean up actions when exiting ACTIVE state
state = OrderState.COMPLETED;
System.out.println("Order completed.");
}
}
Why this works: By managing entry and exit actions, you ensure each state properly initiates or concludes operations, keeping the system stable.
5. Not Documenting State Behavior
Problem
Documentation is often deprioritized, leading to confusion for other developers (or future you). If the purpose of each state and transition isn’t evident, it can cause miscommunication.
Solution
Document each state and its transitions, possibly through a state diagram or a state table.
State Table for Order System
| Current State | Event | Next State |
|---------------|---------------|----------------|
| PENDING | pay | ACTIVE |
| ACTIVE | complete | COMPLETED |
| ACTIVE | cancel | CANCELED |
Why this works: Clear documentation acts as both a guide and a reference, facilitating collaboration and reducing the learning curve for other developers.
Bringing It All Together
State machines can significantly improve a system’s architecture, but it’s crucial to avoid common design pitfalls. By focusing on simplicity, maintaining clear transition logic, enforcing valid state changes, managing state entry and exit behaviors, and properly documenting everything, developers can create robust, maintainable state machines that improve the overall quality of the software.
For more information on designing state machines, consider checking out resources such as State Machines for Managers or visit TutorialsPoint for comprehensive explanations and examples.
Feel free to explore state machines further, and remember that clarity and simplicity in design lead to solid software development!
Checkout our other articles