Why JDK 9's Release Features Were Underappreciated
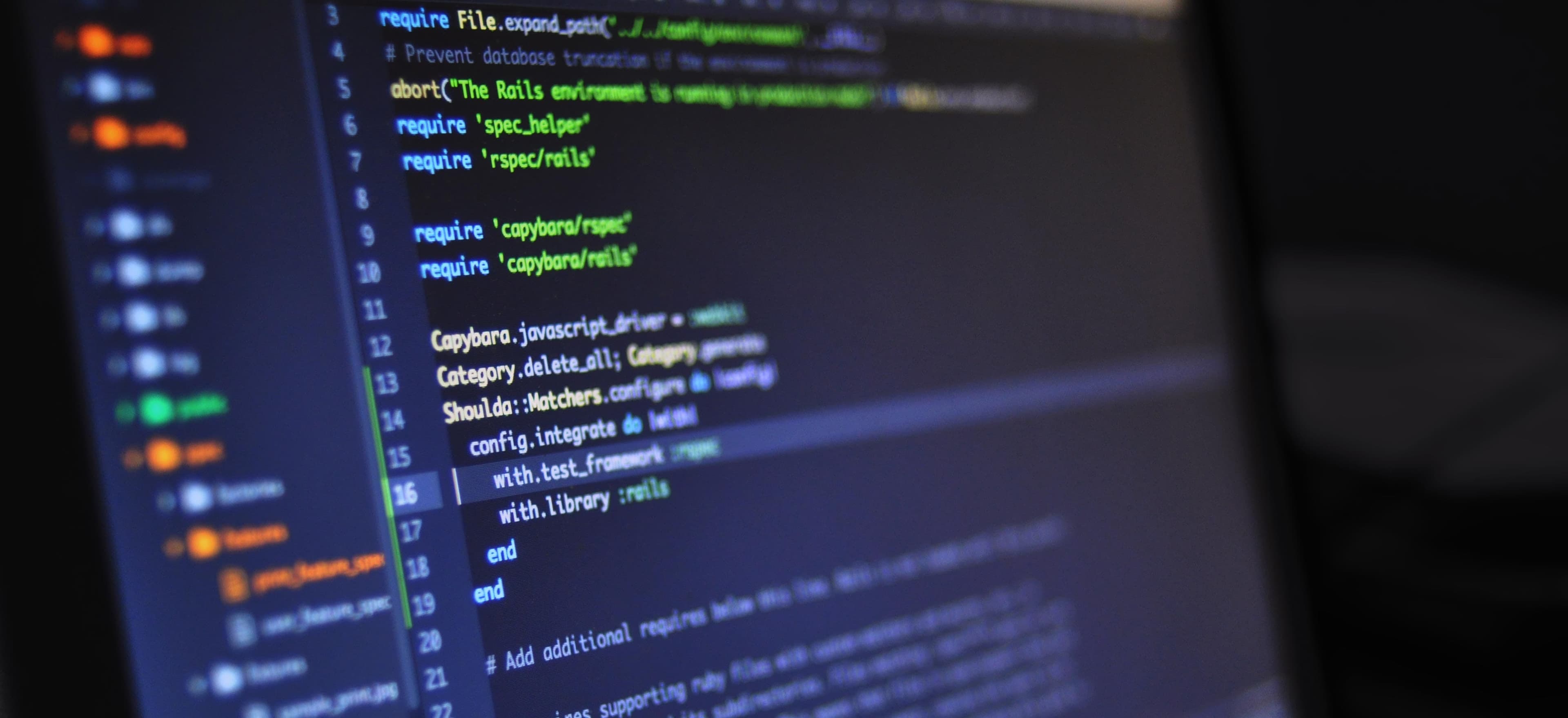
- Published on
Why JDK 9's Release Features Were Underappreciated
Java Development Kit (JDK) 9 marked a pivotal shift in the Java programming ecosystem. Released in September 2017, it introduced significant features and improvements that, while powerful, often fell under the radar of many developers. This blog post delves into the notable features of JDK 9, why they might not have received the appreciation they deserve, and their implications for developers moving forward.
Table of Contents
- Overview of JDK 9 Features
- The Java Platform Module System
- JShell - The Java Shell
- Improved Stream API
- Other Notable Features
- Conclusion
Overview of JDK 9 Features
When Java 9 was released, it brought several enhancements that aimed to improve the Java developer experience. Some of the most noteworthy features included:
- Java Platform Module System (JPMS)
- JShell: The Interactive Java Shell
- Stream API Improvements
- New HTTP Client
- Stack-Walking API
These features not only enhance productivity but also enable more efficient development practices. However, many developers did not fully embrace them.
The Java Platform Module System
One of the most significant introductions in JDK 9 is the Java Platform Module System (JPMS), also known as Project Jigsaw. JPMS allows developers to modularize their applications, improving maintainability and scalability.
Understanding JPMS
Before JPMS, Java applications were typically built as monolithic units. This approach can lead to complications as the codebase grows. With JPMS, you can encapsulate code in modules, making it more manageable.
Example: Defining a Simple Module
Here’s a simple example of how to create a module in Java:
module com.example.myapp {
exports com.example.myapp.services;
}
Why This Matters:
By defining modules, you control what parts of your code are exposed to other modules. This containment helps avoid classpath pollution and enhances security. The encapsulation fosters cleaner architecture, a concept often overlooked by developers still accustomed to older Java practices.
The Future of Modular Programming
Despite its advantages, JPMS received mixed reviews. Some developers saw it as a complex addition to the Java ecosystem. However, as large-scale applications become the norm, modular programming will increasingly be the best practice.
For an excellent resource on modular programming, check Oracle’s official documentation on JPMS.
JShell - The Interactive Java Shell
Another significant feature in JDK 9 is JShell, which enables a more interactive way to write Java code. It allows developers to execute Java code snippets on the fly without needing a full Java class.
How JShell Enhances Learning and Development
JShell is particularly beneficial for beginners and experienced developers alike. It provides instant feedback, which can accelerate the learning process.
Example: Running Code in JShell
You can simply type a command in the JShell environment:
jshell> int sum(int a, int b) { return a + b; }
Why This Matters:
JShell reduces the overhead of creating full projects for testing small snippets. Instead of dealing with a complex environment, you can focus on learning and exploring new APIs with ease. This feature is also handy for rapid prototyping.
The Diminished Recognition of JShell
Despite its practical benefits, many developers either have not adopted JShell or are unaware of its capabilities. Some might perceive it as a novelty rather than a tool for productivity and learning.
Improved Stream API
The Stream API, introduced in JDK 8, provided developers with a new way of working with collections. JDK 9 makes substantial enhancements to this framework.
New Methods in Stream API
JDK 9 introduces new methods, including takeWhile()
, dropWhile()
, and ofNullable()
.
Example: Using takeWhile()
Method
You can use takeWhile()
to retrieve elements that match a specific condition until the condition fails:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6);
Stream<Integer> filteredNumbers = numbers.stream()
.takeWhile(n -> n < 4);
filteredNumbers.forEach(System.out::println); // Outputs: 1, 2, 3
Why This Matters:
This functionality leads to cleaner, more readable code. Instead of writing complex loops, a simple stream operation achieves the same goal.
Other Notable Features
JDK 9 came loaded with additional features:
- New HTTP Client: A more efficient way to handle HTTP requests.
- Stack-Walking API: Improved tools for debugging by allowing stack trace iterations.
- Enhanced Collections: Factory methods for easier collection creation.
Each of these advancements enhances productivity, but they were overshadowed by the larger conversation around modularity and tooling.
Final Thoughts
JDK 9 introduced numerous features aimed at modernizing and simplifying Java development. While some developers were quick to embrace the new modular system, others failed to recognize the value of enhancements like JShell and the improved Stream API.
As the Java landscape continues to evolve, embracing these tools will be critical. JDK 9 may not have received the fanfare it deserved, but as developers seek efficiency and performance, its features will become increasingly relevant.
In summary, understanding and integrating the advancements from JDK 9 into your development workflow can lead to a more organized and productive coding experience. For those looking to deepen their knowledge of Java, consider exploring the links provided for further insights.
The appreciation of JDK 9’s features may not have been universal, but their potential to transform the Java programming approach is undeniable. Embrace these innovations, and bolster your Java programming strategies!
Checkout our other articles