Understanding the Transition from Drools Planner to OptaPlanner
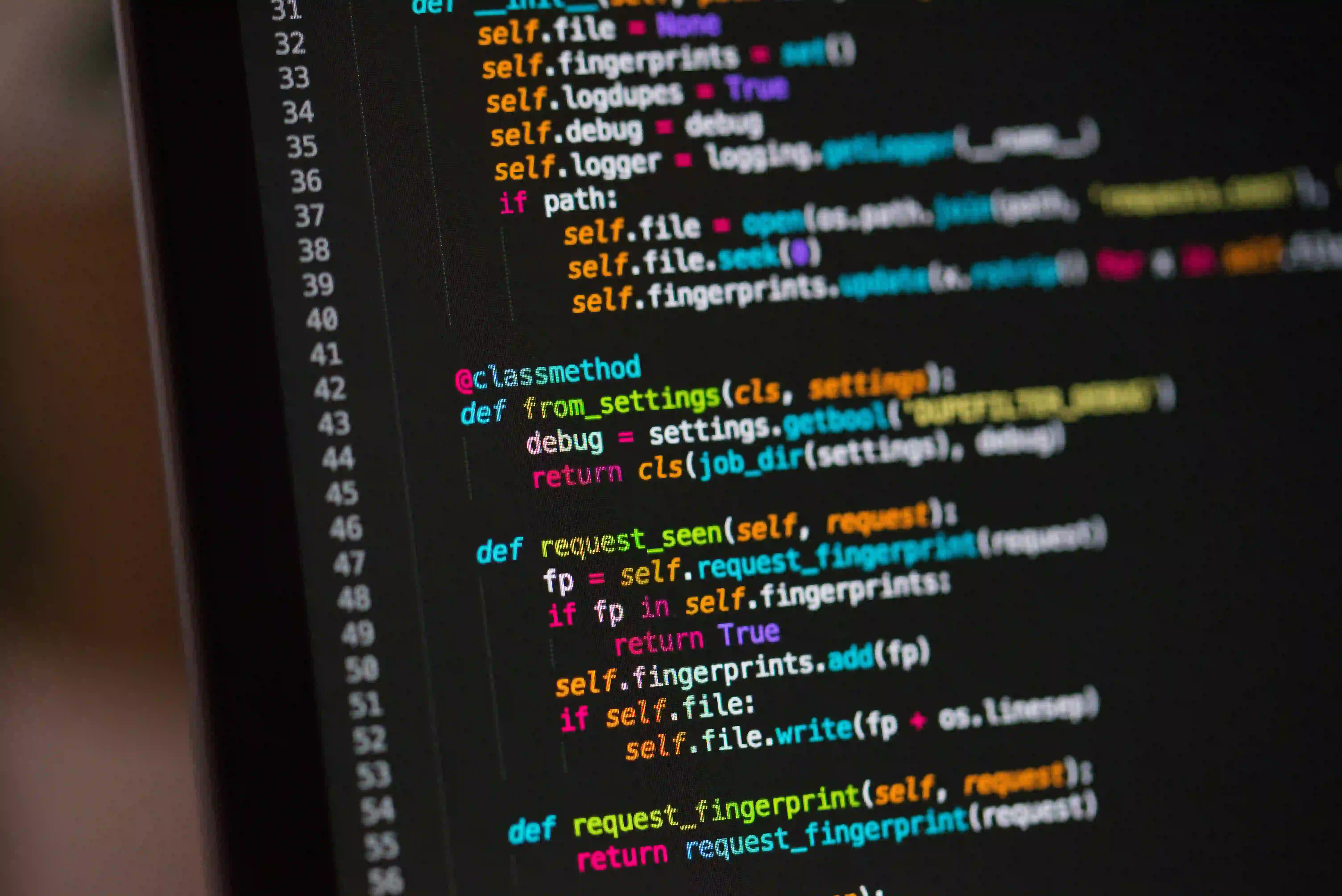
Understanding the Transition from Drools Planner to OptaPlanner
In the world of business process management and decision automation, optimization becomes a critical necessity. For those familiar with the Java ecosystem and the complexities of optimization, the transition from Drools Planner to OptaPlanner is a significant evolution worth discussing. This article will navigate through the nuances of this transition, exploring reasons for the change, key features, and how to make the most of OptaPlanner.
What is OptaPlanner?
OptaPlanner is an open-source constraint solver developed in Java. Its primary role is to optimize planning and scheduling problems, addressing issues where resources need to be efficiently allocated to tasks. It builds on the foundational principles of Drools Planner but advances them to provide a more robust, versatile framework.
Why the Shift?
The shift from Drools Planner to OptaPlanner stemmed from the need for enhanced features and a stronger community backing. Drools Planner provided essential functionalities, but as the demand for optimization increased, it became necessary to develop a more scalable and user-friendly solution. OptaPlanner is focused on usability, performance, and extensive testing, making it a leading choice in the optimization domain.
Key Features of OptaPlanner
-
Enhanced Scalability: OptaPlanner can effortlessly handle larger datasets than its predecessor, allowing for the optimization of more complex problems.
-
Performance Improvements: With features such as multi-threading capabilities and various solving algorithms, OptaPlanner offers an optimized experience for developers and end-users alike.
-
Community-Driven Development: OptaPlanner has an active community that contributes to continuous improvements, ensuring that it stays relevant with emerging trends and needs.
-
Compatibility with Modern Technologies: OptaPlanner integrates seamlessly with frameworks such as Quarkus and Spring, making it suitable for modern application stacks.
Setting Up OptaPlanner
Before diving into code examples, you need to get OptaPlanner set up in your development environment. You can install it using Maven, Gradle, or directly through JAR files.
Maven Dependency
Include the following dependency in your pom.xml
:
<dependency>
<groupId>org.optaplanner</groupId>
<artifactId>optaplanner-spring-boot-starter</artifactId>
<version>8.16.0.Final</version>
</dependency>
Gradle Dependency
If you are using Gradle, include it in your build.gradle
like this:
implementation 'org.optaplanner:optaplanner-spring-boot-starter:8.16.0.Final'
The Simple Example: Solving a Traveling Salesperson Problem
Let’s illustrate how to define and solve an optimization problem using OptaPlanner. The Traveling Salesperson Problem (TSP) is a classic example that will help in understanding OptaPlanner's capabilities.
Step 1: Model the Problem
First, we need to define our data model. In our case, we are looking to optimize the route taken by a salesperson to visit a number of cities.
import org.optaplanner.core.api.score.buildin.hardsoft.HardSoftScore;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import java.util.List;
@Entity
public class SalesTour {
@Id
@GeneratedValue
private Long id;
private List<City> cityList;
// Constructors, Getters, and Setters
}
Commentary
In the above code, we declare a SalesTour
entity. Each SalesTour
contains a list of City
objects. The HardSoftScore
type enables us to define scoring rules explicitly, distinguishing between hard constraints (unbreakable) and soft constraints (desirable but flexible).
Step 2: Define The Score Calculation
We need to create a score calculation to guide the optimization process, encapsulated within a ScoreCalculator implementation.
import org.optaplanner.core.api.score.calculator.ScoreCalculator;
public class SalesTourScoreCalculator implements ScoreCalculator<SalesTour> {
@Override
public HardSoftScore calculateScore(SalesTour salesTour) {
int hardScore = calculateHardScore(salesTour);
int softScore = calculateSoftScore(salesTour);
return HardSoftScore.of(hardScore, softScore);
}
private int calculateHardScore(SalesTour salesTour) {
// Your hard constraint logic here.
return 0; // Example return
}
private int calculateSoftScore(SalesTour salesTour) {
// Your soft constraint logic here.
return 0; // Example return
}
}
Commentary
In this part, we define the scoring rules for our problem. The calculateHardScore
and calculateSoftScore
methods are placeholders for your specific business logic. Keep in mind that the scoring mechanism is crucial; it directly impacts the quality of the optimization solution.
Step 3: Solution Approaching Algorithm Configuration
OptaPlanner supports various algorithms. Let's configure a simple solver configuration via XML.
<solver>
<solutionClass>com.example.SalesTour</solutionClass>
<entityClass>com.example.City</entityClass>
<scoreDirectorFactory>
<scoreCalculatorClass>com.example.SalesTourScoreCalculator</scoreCalculatorClass>
</scoreDirectorFactory>
</solver>
Commentary
This XML configuration defines the relationships between your solution and entity classes while specifying the score calculation method. This approach allows for a clear separation of concerns, enhancing maintainability.
Step 4: Running the Solver
Finally, you need to run the solver to optimize the SalesTour.
import org.optaplanner.core.api.solver.SolverManager;
public void runSolver(SalesTour unsolvedSalesTour) {
SolverManager<SalesTour, Long> solverManager = SolverManager.create();
SalesTour solvedSalesTour = solverManager.solve(unsolvedSalesTour);
System.out.println("Best route: " + solvedSalesTour);
}
Commentary
By running the solve
method, OptaPlanner’s solver engine applies your scoring rules to find the most efficient route. The results showcase how effective and elaborate routing can be achieved simply with OptaPlanner.
The Benefits of Using OptaPlanner
-
Flexibility: OptaPlanner can adapt to a wide range of problems, making it a valuable tool if your business evolves.
-
Reduced Time-to-Solution: OptaPlanner reduces the complexity often associated with optimization challenges, leading to faster and more reliable solutions.
-
Community and Documentation: With an extensive documentation and community behind it, developers can acquire quicker support and maintain a higher code quality.
The Last Word
The transition from Drools Planner to OptaPlanner marks a crucial improvement in the optimization landscape. With its enhanced features, established community, and powerful algorithms, OptaPlanner emerges as a preferred choice for many Java developers.
Whether you are tackling a simple scheduling problem or a multifaceted business optimization challenge, OptaPlanner equips you with the essential tools to guide your solutions effectively. For further reading on optimization strategies, consider checking OptaPlanner Documentation or the GitHub repository, where you can engage with the community and explore code samples.
By embracing OptaPlanner, you're stepping into a more efficient and evolved future of optimization in Java development.