Improving Java Class Loading Performance in Large Applications
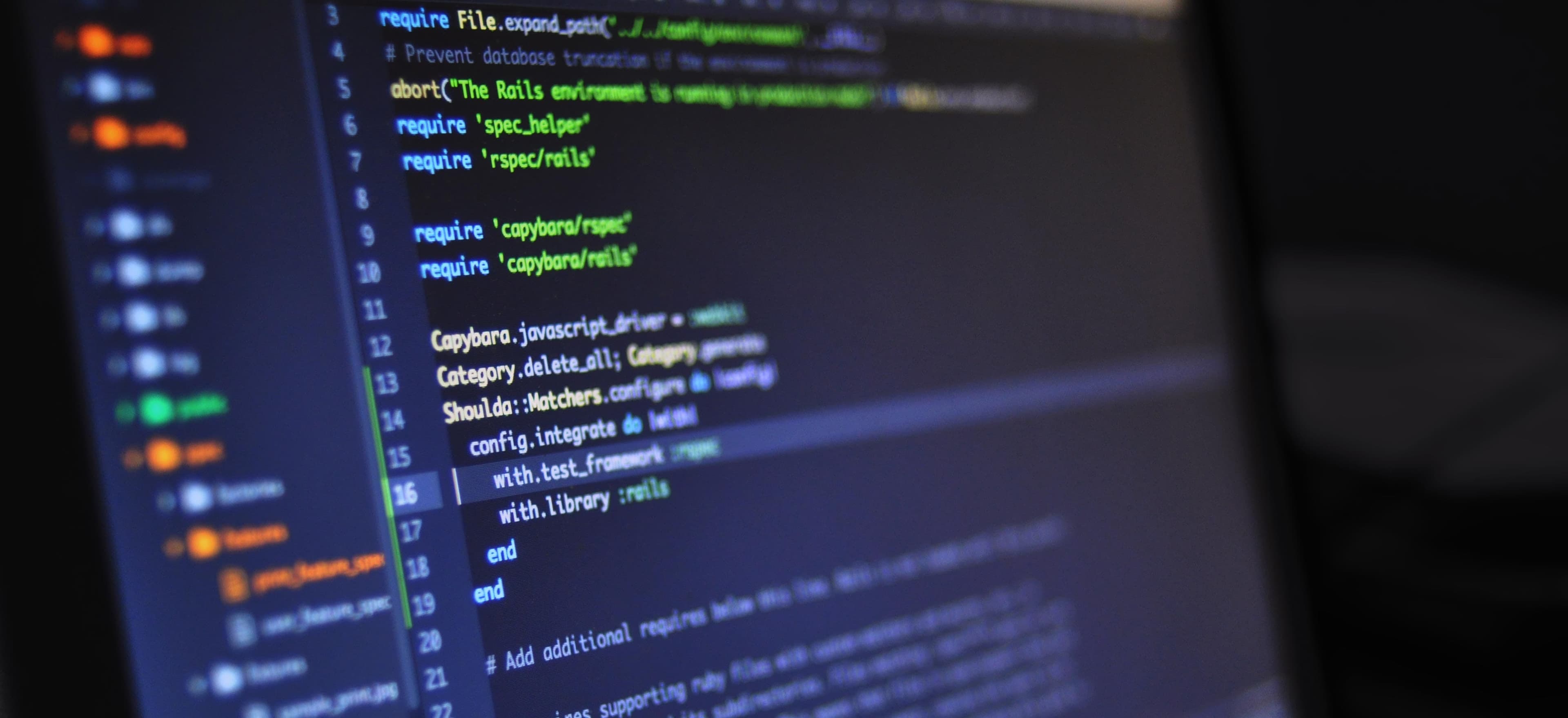
- Published on
Improving Java Class Loading Performance in Large Applications
Java is an object-oriented programming language that emphasizes portability, security, and performance. One core aspect of Java performance is class loading. In large-scale applications, understanding and optimizing class loading can significantly enhance performance. In this blog post, we will explore how Java accomplishes class loading, why performance matters, and practical ways to improve class loading performance.
Understanding Java Class Loading
Before we dive into performance optimization, let's clarify the Java class loading mechanism. When a Java application runs, the Java Virtual Machine (JVM) loads classes into memory. This process involves several steps:
- Loading: The JVM locates and loads the
.class
file using a ClassLoader. - Linking: This stage includes verification, preparation, and resolution of symbols.
- Initialization: The JVM initializes static variables and executes static blocks.
The process can be complex, especially in large applications with numerous classes and dependencies. Improper management of class loading can lead to performance bottlenecks.
The Impact of Class Loading on Performance
Class loading directly affects application startup time and runtime performance. If classes are loaded too late or inefficiently, it can cause:
- Increased startup times
- Higher memory consumption
- Sluggish application responsiveness
By improving class loading, developers can enhance the overall efficiency of their Java applications in substantial ways.
Strategies for Improving Class Loading Performance
Let’s discuss effective strategies to optimize class loading and consequently improve the performance of large Java applications.
1. Use the Right ClassLoader
Choosing the appropriate ClassLoader is crucial. The JVM comes with several ClassLoaders, but custom ClassLoaders can be created for specific use cases. Here is how you can implement a custom ClassLoader:
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.lang.reflect.InvocationTargetException;
public class CustomClassLoader extends ClassLoader {
private final String classPath;
public CustomClassLoader(String classPath) {
this.classPath = classPath;
}
@Override
protected Class<?> findClass(String name) throws ClassNotFoundException {
try {
byte[] classData = loadClassData(name);
return defineClass(name, classData, 0, classData.length);
} catch (IOException e) {
throw new ClassNotFoundException("Could not find class", e);
}
}
private byte[] loadClassData(String className) throws IOException {
className = className.replace('.', '/');
FileInputStream fis = new FileInputStream(new File(classPath, className + ".class"));
byte[] buffer = new byte[fis.available()];
fis.read(buffer);
fis.close();
return buffer;
}
public static void main(String[] args) throws Exception {
CustomClassLoader classLoader = new CustomClassLoader("path/to/classes");
Class<?> clazz = classLoader.loadClass("your.package.ClassName");
Object instance = clazz.getDeclaredConstructor().newInstance();
}
}
Why Use a Custom ClassLoader?
Custom ClassLoaders allow you to control how and when classes are loaded. They help avoid premature loading of classes that may not be needed immediately. This can decrease memory usage and improve startup time.
2. Avoid ClassLoader Hell
ClassLoader hell refers to complications that arise from class path conflicts. Duplicate classes or incompatible libraries can lead to unpredictable behavior and slow performance. To mitigate this:
- Stick to consistent versions of libraries.
- Use build tools like Maven or Gradle to manage dependencies effectively.
- Modularize your application to minimize cross-dependencies.
3. Optimize Class Loading Order
Java loads classes as they are required. Consider using lazy loading for non-essential classes. Here's a simplified approach to lazy loading:
public class LazyLoader {
private SomeService someService;
public SomeService getService() {
if (someService == null) {
someService = new SomeService();
}
return someService;
}
}
Why Lazy Loading?
Lazy loading defers the loading of classes until they are needed. This strategy is particularly beneficial for large applications where some components may never be used during a session.
4. Use ClassData Sharing (CDS)
Class Data Sharing (CDS) allows classes to be pre-loaded into the JVM. This capability can shorten application startup times by sharing loaded classes among various Java applications.
You can enable CDS using the -Xshare:on
flag when starting a Java application.
Why Use CDS?
By using CDS, you reduce the overhead of loading classes from disk multiple times, leading to faster application performance. More information on using CDS can be found in the official Java documentation.
5. Profiling and Monitoring
To improve class loading, you must understand precisely what is happening under the hood. Monitoring tools such as VisualVM, JConsole, or Java Mission Control can provide insights into class loading times and memory consumption patterns.
Why Profile?
Profiling allows developers to pinpoint slow-loading classes or ClassLoaders, enabling them to make informed optimization decisions.
6. Minimize Reflection Usage
Reflection can introduce performance overheads, especially when frequently used in large applications. Consider limiting reflection use or caching reflected objects. Here’s an example:
import java.lang.reflect.Method;
import java.util.HashMap;
import java.util.Map;
public class ReflectionCache {
private static final Map<String, Method> methodCache = new HashMap<>();
public static Method getMethod(Class<?> clazz, String methodName) throws NoSuchMethodException {
String key = clazz.getName() + "." + methodName;
return methodCache.computeIfAbsent(key, k -> {
try {
return clazz.getMethod(methodName);
} catch (NoSuchMethodException e) {
throw new RuntimeException(e);
}
});
}
}
Why Cache Reflection Results?
Caching reflection results minimizes the costly reflection calls, improving performance for frequently called methods.
In Conclusion, Here is What Matters
Java class loading is an essential factor that affects the performance of large applications. By using the right ClassLoader, avoiding classpath conflicts, implementing lazy loading, enabling Class Data Sharing, profiling the application, and minimizing reflection usage, developers can significantly enhance their Java application's performance.
By addressing class loading performance, you not only improve the application efficiency but also create a better user experience. Always remember to assess the specific needs of your application and choose the appropriate strategies accordingly.
For more in-depth information regarding Java performance optimization, visit the Java Performance Tuning Guide.
By implementing these strategies, you can rise above the complexities of large applications and harness the full power of Java!
Checkout our other articles