Mastering Search and Sort: Common Interview Pitfalls
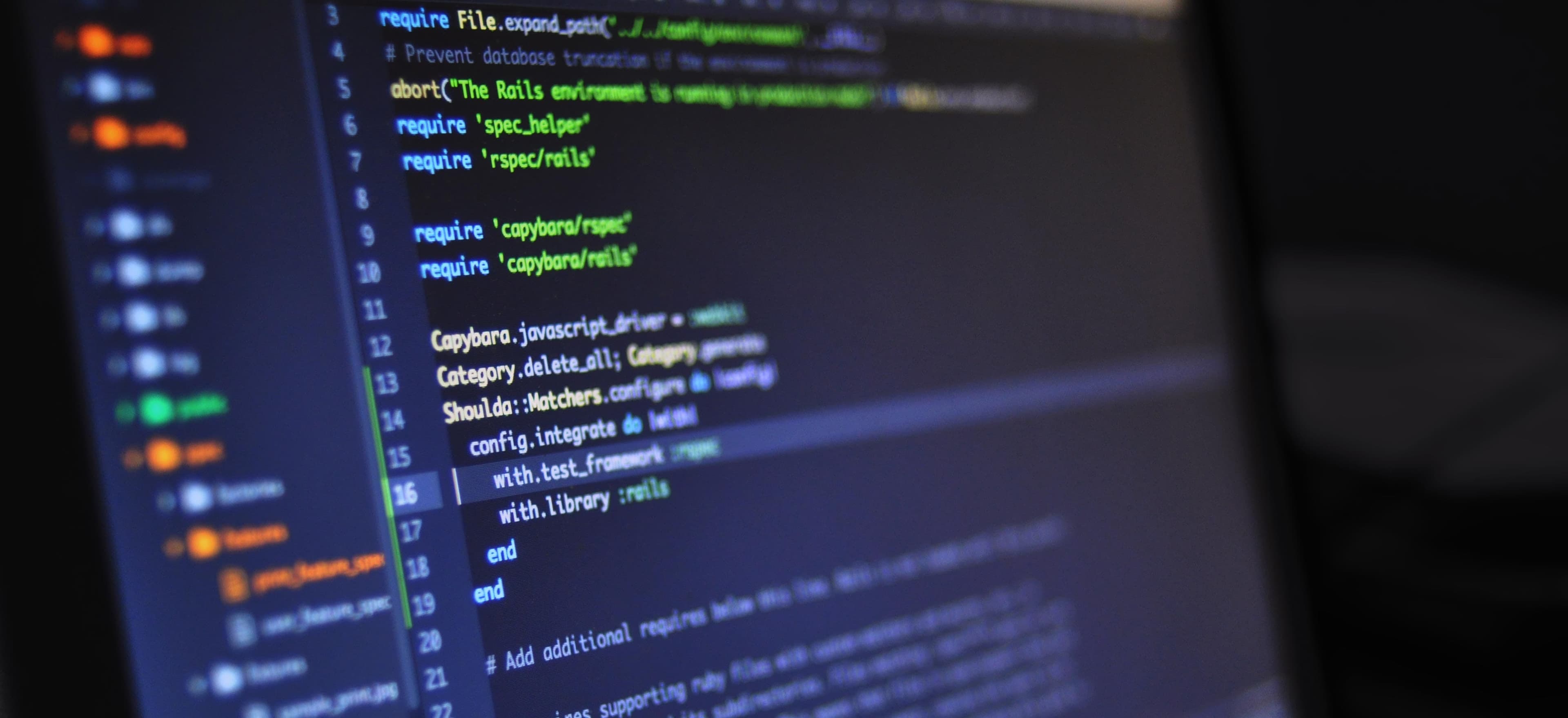
- Published on
Mastering Search and Sort: Common Interview Pitfalls
When it comes to technical interviews, two topics that often come into play are searching and sorting algorithms. These fundamental concepts not only form the building blocks of computer science but also serve as indicators of a candidate's problem-solving abilities. Mastering these topics is crucial, as many candidates fall into common pitfalls that can hinder their performance. In this blog post, we'll explore the search and sort algorithms, highlight typical interview mistakes, and discuss strategies to rise above them.
Why Search and Sort Matter
Understanding how to implement and optimize search and sort algorithms is crucial for various applications — from simple tasks like organizing a list of names to complex data retrieval tasks in databases. Given the competitive nature of the tech industry, demonstrating a solid understanding of these concepts can set you apart from other candidates.
Searching Algorithms
Searching algorithms help find an item from a data structure. The most common searching algorithms include Linear Search and Binary Search.
Linear Search
Linear search is the most straightforward search algorithm. It scans each element in a list until it finds the target value or reaches the end of the list.
public class LinearSearch {
public static int linearSearch(int[] arr, int target) {
// Iterates through the array to find the target
for (int i = 0; i < arr.length; i++) {
if (arr[i] == target) {
return i; // Returns the index if the target is found
}
}
return -1; // Returns -1 if the target is not found
}
}
Why Use Linear Search?
- It's easy to implement and understand.
- It works on unsorted data.
Common Pitfall: Candidates often overlook the O(n) time complexity of linear search, which can become inefficient with larger datasets. Always recognize when linear search is appropriate by considering the size and order of the data.
Binary Search
Binary search is a much more efficient algorithm, but it requires the data to be sorted beforehand. It reduces the search space in half each time it compares the target value with the middle element of the array.
public class BinarySearch {
public static int binarySearch(int[] arr, int target) {
int left = 0;
int right = arr.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid; // Target found
}
if (arr[mid] < target) {
left = mid + 1; // Search in the right half
} else {
right = mid - 1; // Search in the left half
}
}
return -1; // Target not found
}
}
Why Use Binary Search?
- It has a time complexity of O(log n), making it vastly superior to linear search on large, sorted datasets.
Common Pitfall: Candidates often forget to sort the array before applying binary search. Failing to recognize when this pre-condition applies could lead to incorrect results.
Sorting Algorithms
Sorting algorithms arrange elements in a specified order. Some widely utilized sorting algorithms include Bubble Sort, Insertion Sort, Merge Sort, and Quick Sort.
Bubble Sort
Bubble sort is the simplest sorting algorithm, repeatedly stepping through the list, comparing adjacent elements and swapping them if they are in the wrong order.
public class BubbleSort {
public static void bubbleSort(int[] arr) {
int n = arr.length;
boolean swapped;
do {
swapped = false;
for (int i = 1; i < n; i++) {
if (arr[i - 1] > arr[i]) {
// Swap arr[i - 1] and arr[i]
int temp = arr[i - 1];
arr[i - 1] = arr[i];
arr[i] = temp;
swapped = true;
}
}
n--; // Reduce the size by one after each pass
} while (swapped);
}
}
Why Use Bubble Sort?
- It's simple and easy to understand.
Common Pitfall: Many candidates use bubble sort in environments where performance matters, despite its O(n^2) time complexity. Recognizing the algorithm's limitations is critical.
Quick Sort
Quick sort is a more advanced sorting algorithm that uses a divide-and-conquer approach. It selects a "pivot" element and partitions the array based on this pivot.
public class QuickSort {
public static void quickSort(int[] arr, int low, int high) {
if (low < high) {
// Partition the array
int pivotIndex = partition(arr, low, high);
// Recursively sort elements before and after partition
quickSort(arr, low, pivotIndex - 1);
quickSort(arr, pivotIndex + 1, high);
}
}
private static int partition(int[] arr, int low, int high) {
int pivot = arr[high];
int i = (low - 1); // Index of smaller element
for (int j = low; j < high; j++) {
if (arr[j] <= pivot) {
i++;
// Swap arr[i] and arr[j]
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
// Swap arr[i + 1] and arr[high] (or pivot)
int temp = arr[i + 1];
arr[i + 1] = arr[high];
arr[high] = temp;
return i + 1; // Return the index of the pivot
}
}
Why Use Quick Sort?
- Quick sort has an average time complexity of O(n log n) and is often faster in practice than other O(n log n) algorithms like Merge Sort.
Common Pitfall: Many candidates neglect to consider the worst-case scenario with quick sort (O(n^2)), leading to inefficiencies if the pivot is poorly chosen. Candidates should recognize the benefits of selecting a good pivot and its impact on performance.
Strategies for Success
-
Understand the Algorithms: Don't just memorize the code. Understand each algorithm's mechanics, strengths, and weaknesses.
-
Practice, Practice, Practice: Implement algorithms in various programming languages. Websites like LeetCode and GeeksforGeeks offer great resources for honing your skills.
-
Implement Edge Cases: Make sure to test edge cases (e.g., empty arrays, arrays with one element, arrays with duplicates) to verify the robustness of your implementation.
-
Discuss Your Thought Process: During interviews, communicate your reasoning while solving a problem. It shows your depth of understanding and problem-solving approach.
-
Optimize Your Code: After arriving at a solution, think about ways to make it more efficient. Presenting a better algorithm can demonstrate advanced knowledge.
The Last Word
Mastering search and sort algorithms is key to acing technical interviews. Avoiding common pitfalls like overlooking time complexity, failing to sort before searching, or not considering edge cases will help you present yourself as a well-rounded candidate.
As you prepare, remember that the goal is not only to know the algorithms but to understand their practical applications, limitations, and optimizations. With diligent practice and a focused mindset, you'll be well on your way to mastering these fundamental concepts and excelling in your technical interviews.
By arming yourself with the knowledge of these algorithms, you position yourself as a valuable asset to any tech team. Happy coding!
Checkout our other articles