Debugging Ehcache: Solving Spring Test Context Caching Issues
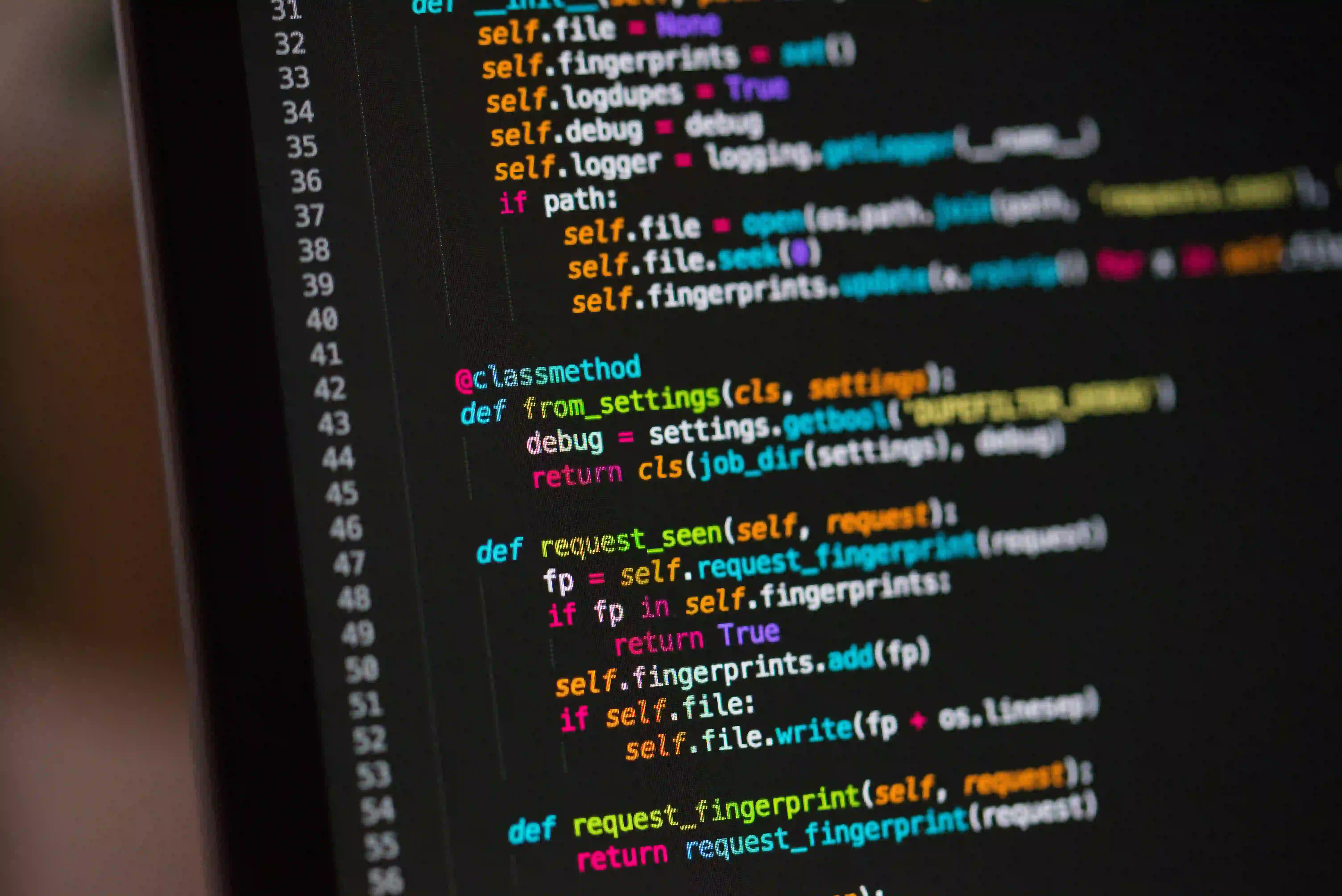
Debugging Ehcache: Solving Spring Test Context Caching Issues
In modern Java applications, Spring is one of the most widely used frameworks for building robust and maintainable applications. One of the powerful features of Spring is its testing capabilities. However, when it comes to testing, caching behaviors can introduce unexpected quirks and elusive bugs. This post will delve into debugging Ehcache related issues within the Spring test context, providing insights and actionable solutions.
Understanding the Basics of Ehcache
Ehcache is a widely used caching library in Java. It allows developers to enhance the performance of their applications through the caching of frequently accessed data. Spring integrates seamlessly with Ehcache, enabling you to use it in service layers and controller layers without much overhead.
Why Caching is Important
Caching can speed up an application's performance significantly by reducing database load and response times. However, improper cache handling can lead to inconsistencies, making debugging a challenge.
Spring Test Context Caching Issues
The Spring Test framework provides a way to manage the test context, which includes configurations, beans, and more. However, when caching comes into play, the test context's behavior can lead to some unclear situations.
Issues You Might Encounter
- Stale Data: You may find that your tests are not reflecting the current state of the database due to data being cached.
- Inconsistent Test Results: As tests run, they might exhibit different behaviors on subsequent executions due to context caching.
- Memory Issues: Caches that are not properly evicted may lead to memory bloat during tests.
Debugging Steps
To effectively debug Ehcache-related issues in Spring test contexts, follow these steps:
1. Understanding Default Caching Behavior
Spring applies caching automatically for beans that use the @Cacheable
annotation. Here's a simple code snippet:
@Service
public class UserService {
@Cacheable("users")
public User getUserById(Long id) {
// Simulating a call to the database
return repository.findById(id);
}
}
In the example above, when getUserById
is called with an id
, the result is cached on the first call. On subsequent calls with the same id
, the method will return the cached user instead of hitting the database again.
2. Using @TestInstance to Manage Test Context
To ensure that tests have a fresh context every time, consider using the @TestInstance
annotation:
@TestInstance(TestInstance.Lifecycle.PER_CLASS)
public class UserServiceTest {
@Autowired
private UserService userService;
@Test
public void testGetUser() {
User user = userService.getUserById(1L);
// your assertions here
}
}
With this annotation, each test class gets a single instance. This can help with caching issues in tests, ensuring that the context behaves as expected.
3. Clearing the Cache
Sometimes, you need to manually clear the cache between tests to avoid stale data. You can do this using @CacheEvict
:
@CacheEvict(value = "users", allEntries = true)
@Test
public void resetUserCache() {
// Resets the user cache before tests
}
4. Using Test Profiles
Spring profiles allow you to define different parts of your configuration for different environments. You can load a profile specifically for testing that disables caching:
@Configuration
@Profile("test")
public class TestCachingConfig {
@Bean
public CacheManager cacheManager() {
return new NoOpCacheManager(); // Disables caching
}
}
5. Logging Cache Activity
To understand how caching is affecting your tests, enable logging for Ehcache. Edit your log4j2.xml
or logback.xml
configuration to include:
<logger name="net.sf.ehcache" level="DEBUG" />
With logging enabled, you can see when items are being cached, evicted, or loaded, enabling you to trace issues more efficiently.
Additional Recommendations
- Review Cache Configuration: Ensure your cache settings in your configuration files are appropriate for the testing environment. Sometimes, increasing timeouts or decreasing memory size for test scenarios can alleviate issues.
- Use Mocking: Use tools like Mockito to mock behavior instead of relying on an actual cache to isolate the behavior of the service or repository during tests.
- Read the Documentation: Familiarize yourself with Spring Caching and Ehcache documentation for deeper insights.
The Last Word
Debugging Ehcache issues related to Spring test context can be challenging due to the intricacies of caching behaviors. By following structured debugging steps, like utilizing configurations, clearing caches, and including proper logging, you can efficiently identify and rectify these issues. The goal is always to maintain confidence in your automated tests while ensuring the system remains performant and reliable.
Further Reading
For more in-depth information, consider the following resources:
By understanding the behaviors of Ehcache in a Spring context, you can make the most out of them while minimizing challenges, ensuring your applications run smoothly, even during testing. Happy debugging!