Unlocking Fluent APIs: The Challenges of Builder AST in Groovy
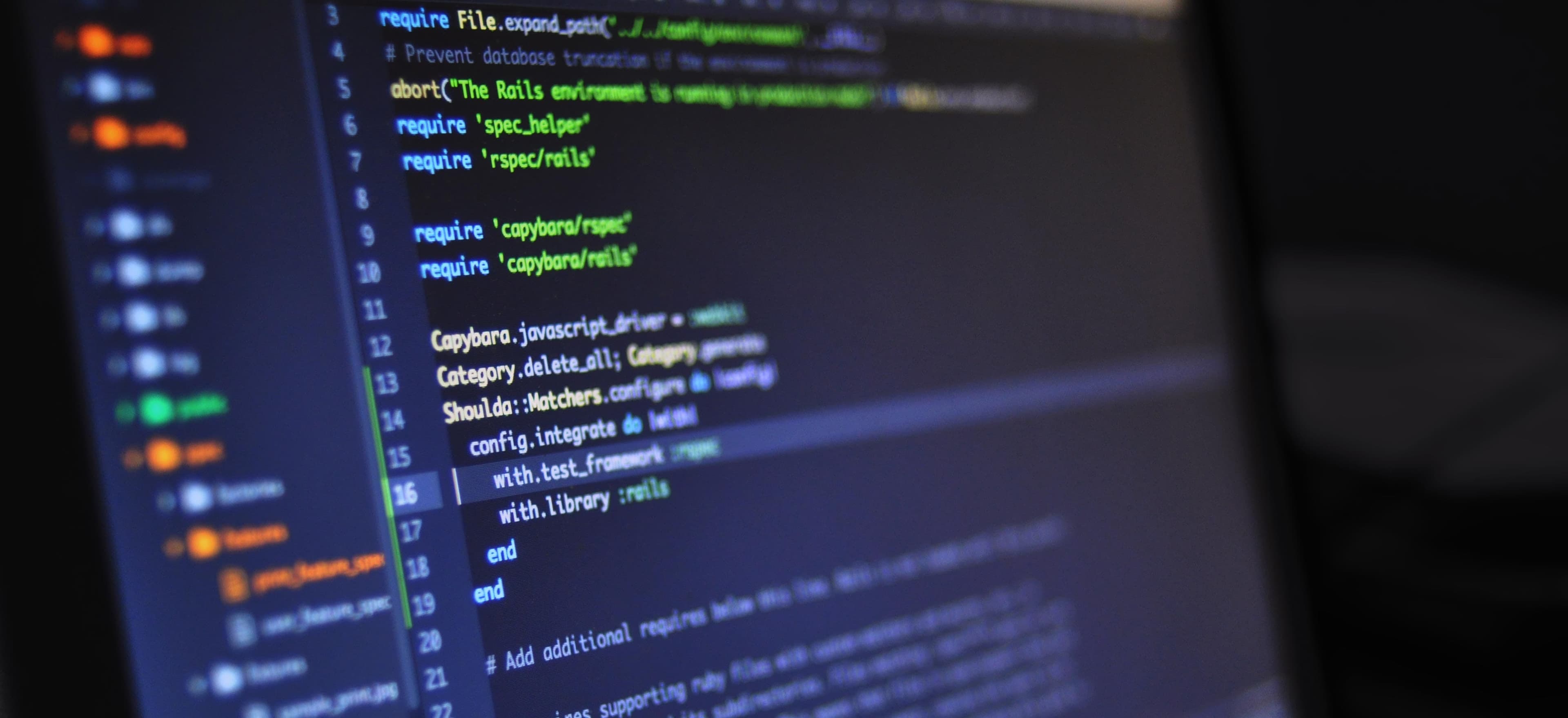
- Published on
Unlocking Fluent APIs: The Challenges of Builder AST in Groovy
In software development, the ability to create clear, concise, and expressive APIs is essential. One of the noteworthy techniques in Groovy for achieving this is through the use of Builder AST (Abstract Syntax Tree). Although Builders in Groovy can create DSLs (Domain Specific Languages) and facilitate the construction of complex objects fluently, they come with their own set of challenges. This blog post will explore these challenges and provide guidance on how you can tackle them effectively.
Understanding Builders in Groovy
Groovy's Builder is a powerful construct that allows developers to create complex structures using simple, readable syntax. They enable a fluent API that is easy to understand and use. The Builder AST in Groovy utilizes a distinguished syntactic sugar that simplifies the instantiation of complex objects.
Example of a Simple Builder
Here's a straightforward example illustrating a Groovy Builder in action:
class Person {
String name
int age
}
def personBuilder = new groovy.util.BuilderSupport()
def person = personBuilder.person {
name 'John Doe'
age 30
}
println "Name: ${person.name}, Age: ${person.age}"
In the snippet above, a Person
object is constructed using a Groovy builder. It provides a clean and expressive way to instantiate an object.
The Power of Fluent APIs
Fluent APIs allow developers to chain method calls succinctly, making the code more readable and easier to write. This is a significant advantage in scenarios where multiple properties need to be set.
Code Example
You can define a fluent API for the Person
class like this:
class FluentPerson {
String name
int age
FluentPerson setName(String name) {
this.name = name
return this
}
FluentPerson setAge(int age) {
this.age = age
return this
}
}
// Usage
def person = new FluentPerson().setName('Jane Doe').setAge(25)
println "Name: ${person.name}, Age: ${person.age}"
In this code snippet, methods return the instance itself, permitting method chaining, which enhances code clarity.
Challenges with Builder AST
While Building ASTs can be highly beneficial, several challenges typically arise when using them in Groovy:
-
Complexity of Understanding: Novice developers may find the Builder and AST concepts challenging to grasp initially. Understanding how Builders translate into ASTs can be daunting.
-
Performance Overhead: Groovy's dynamic nature and the additional processing for Builders may lead to performance issues, especially in critical performance scenarios.
-
Error Handling: Since Builders provide a flexible structure, poorly defined syntax can often lead to hard-to-debug errors. Ensuring that errors are caught and handled effectively is paramount.
-
Maintainability: With the power of builders comes the risk of creating overly complex structures that can become challenging to maintain. Ensuring that the built code is clear and maintainable is essential.
-
Compatibility Issues: As languages evolve, certain patterns may become outdated or less supported. Relying heavily on Builders can lead to compatibility issues, particularly with upgrades to the Groovy framework itself.
Tackling the Challenges
Overcoming these challenges requires thoughtful approaches:
1. Code Readability
To keep code maintainable, it is crucial to maintain high readability throughout your applications. Use clear naming conventions and consistent formatting. For example:
class FluentCar {
String model
String color
FluentCar withModel(String model) {
this.model = model
return this
}
FluentCar withColor(String color) {
this.color = color
return this
}
}
// Usage
def car = new FluentCar().withModel('Toyota').withColor('Red')
2. Performance Monitoring
Keep an eye on performance metrics especially when using dynamic features of Groovy like Builders. If you notice performance drops, profile your application to identify bottlenecks. Consider alternative design patterns or reduce the complexity of Builders if necessary.
3. Comprehensive Error Handling
Implement comprehensive error handling within your Builders to capture runtime exceptions or incorrect input:
class EnhancedFluentPerson {
String name
int age
EnhancedFluentPerson setName(String name) {
if (name == null || name.isEmpty()) {
throw new IllegalArgumentException("Name must not be null or empty")
}
this.name = name
return this
}
EnhancedFluentPerson setAge(int age) {
if (age < 0) {
throw new IllegalArgumentException("Age must be non-negative")
}
this.age = age
return this
}
}
4. Documenting Your API
Documentation is vital for both internal teams and external users. Provide clear and consistent documentation, including examples that show how to use Builders effectively.
5. Compatibility Management
Stay updated with the Groovy language evolution. Regularly check the Groovy website for updates and best practices that can help you prepare for changes in the API landscape.
Closing Remarks
The implementation of Builder ASTs in Groovy can significantly enhance your ability to write readable and maintainable code. However, as we've explored, developers must navigate challenges, including understanding complexity, performance concerns, and maintainability. By applying best practices, implementing error handling, and maintaining clear documentation, you can unlock the potential of fluent APIs while keeping your code clean and effective.
Explore more about Groovy here to expand your understanding and begin leveraging its features in your projects. Embrace the power of Fluent APIs, and let them guide you to create better software solutions.