Struggling with Custom Thread Pools in Parallel Database Streams?
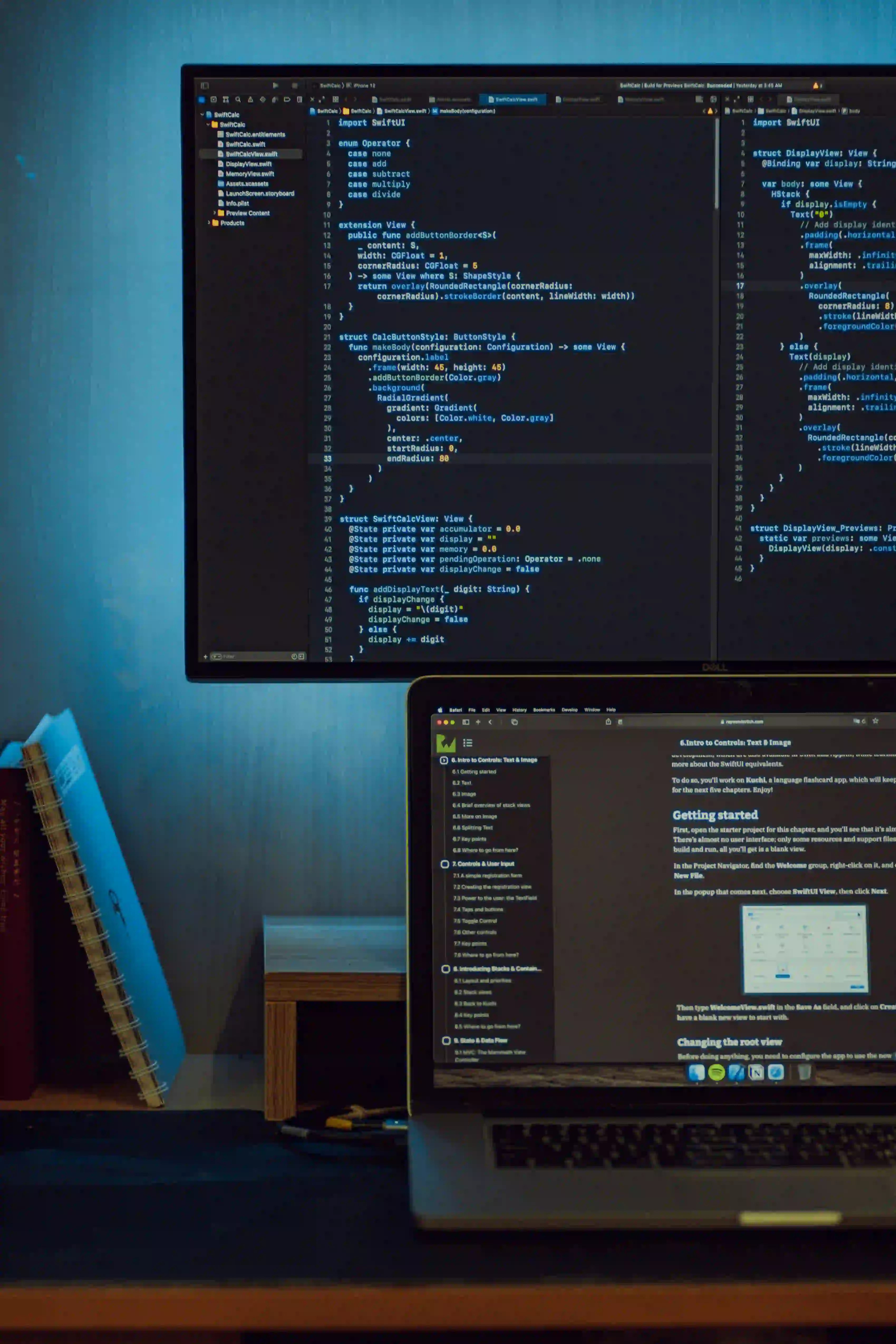
Struggling with Custom Thread Pools in Parallel Database Streams?
When dealing with concurrent programming in Java, especially in the context of managing database interactions, custom thread pools can be a game-changer. They enhance performance, control resource utilization, and help manage complex tasks efficiently. In this blog post, we'll explore how to create and use custom thread pools in conjunction with Java's parallel streams, especially when working with databases.
Understanding the Basics of Parallel Streams
Parallel streams were introduced in Java 8 to process sequences of elements in parallel. This means that operations on a stream can be executed in multiple threads. Parallel streams can improve application performance by maximizing the use of available CPU cores.
However, by default, parallel streams use the ForkJoinPool, which can lead to problems when interacting with resource-heavy operations like database calls. By tuning the thread pool, you can avoid resource contention and improve efficiency.
Why Custom Thread Pools?
-
Performance Control: You can tailor the thread pool size according to the specific needs of your application and the constraints of your database.
-
Resource Management: Custom thread pools allow you to manage the number of concurrent connections to a database, reducing the risk of overloading your database server.
-
Task-specific Optimizations: You can create different thread pools for different types of tasks, allowing for more efficient handling of your workload.
Creating a Custom Thread Pool
Java offers a rich set of APIs to create custom thread pools. The simplest way to create a thread pool is by using the Executors
class. Below is an example:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ThreadPoolExample {
public static void main(String[] args) {
// Create a custom thread pool with a fixed number of threads
int poolSize = 10;
ExecutorService executorService = Executors.newFixedThreadPool(poolSize);
// Execute tasks
for (int i = 0; i < 20; i++) {
final int taskId = i;
executorService.submit(() -> {
System.out.println("Executing task " + taskId + " by thread " + Thread.currentThread().getName());
// Simulate some work
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
}
// Shutdown the executor service
executorService.shutdown();
}
}
Code Explanation
In this example, we create a fixed thread pool with 10 threads. We submit tasks to the executor service, and each task simulates work by sleeping for 1 second. This simple approach allows you to run multiple tasks concurrently without overwhelming the resources.
Integrating with Parallel Streams
Using a custom thread pool with parallel streams requires a bit more setup. By changing the default ForkJoinPool to a custom one, you can better manage how parallel processing interacts with database operations.
You can achieve this by overriding the common pool with your own. Below is how you can do this:
import java.util.List;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.stream.Collectors;
import java.util.stream.IntStream;
public class CustomParallelStream {
public static void main(String[] args) {
// Create a custom executor service
ExecutorService executorService = Executors.newFixedThreadPool(5);
// Set custom parallelism level
System.setProperty("java.util.concurrent.ForkJoinPool.common.parallelism", "5");
// Generate data (representing database records)
List<Integer> data = IntStream.rangeClosed(1, 20).boxed().collect(Collectors.toList());
// Use the custom executor in a parallel stream
data.parallelStream().forEach(dataItem -> {
// Perform database operations
performDatabaseOperation(dataItem);
});
// Shutdown the executor service
executorService.shutdown();
}
private static void performDatabaseOperation(int dataItem) {
System.out.println("Processing data item " + dataItem + " by thread " + Thread.currentThread().getName());
// Simulate database operation with sleep
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
Code Explanation
In this code snippet, we set up a custom thread pool with 5 threads. By setting the ForkJoinPool.common.parallelism
system property, we control the parallelism level of the application. This way, when we invoke data.parallelStream()
, it uses the defined pool instead of the default one.
The performDatabaseOperation
simulates heavy database transactions, and you can replace the sleep
with actual database calls.
Things to Consider
-
Database Connection Pooling: Using a connection pool library (like HikariCP or Apache DBCP) is highly recommended. It manages a pool of database connections, preventing bottlenecks during high concurrency.
-
Error Handling: Ensure you have proper error handling in your parallel processing, as exceptions thrown in parallel streams can be challenging to manage.
-
Thread Safety: Be cautious about shared resources. If multiple threads manipulate shared state, you need to ensure that the code is thread-safe.
-
Performance Testing: Always perform thorough benchmarking. The number of threads in your custom pool and the database’s capacity may need fine-tuning based on your specific workload.
-
Utilizing ForkJoinPool: For more advanced usage, consider directly creating a
ForkJoinPool
instance to handle more complex concurrency scenarios.
The Closing Argument
Custom thread pools can enhance parallel database operations in Java, leading to better performance and resource management. By following the approach outlined in this blog post, you can easily integrate custom thread pools with parallel streams and manage your database transactions more efficiently.
For further reading on Java concurrency and thread safety, check out resources from Oracle here.
Feel free to test the code provided above and adapt it based on your application's needs. If you have any further questions or need assistance, don't hesitate to reach out!
Happy Coding!
Use this blog post as a guide to enhance your understanding of custom thread pools and parallel stream processing in Java. By leveraging these techniques, you'll be well on your way to creating efficient, concurrent applications that can handle complex workloads with ease.