Common Issues When Setting Up Spring Boot CI Pipelines
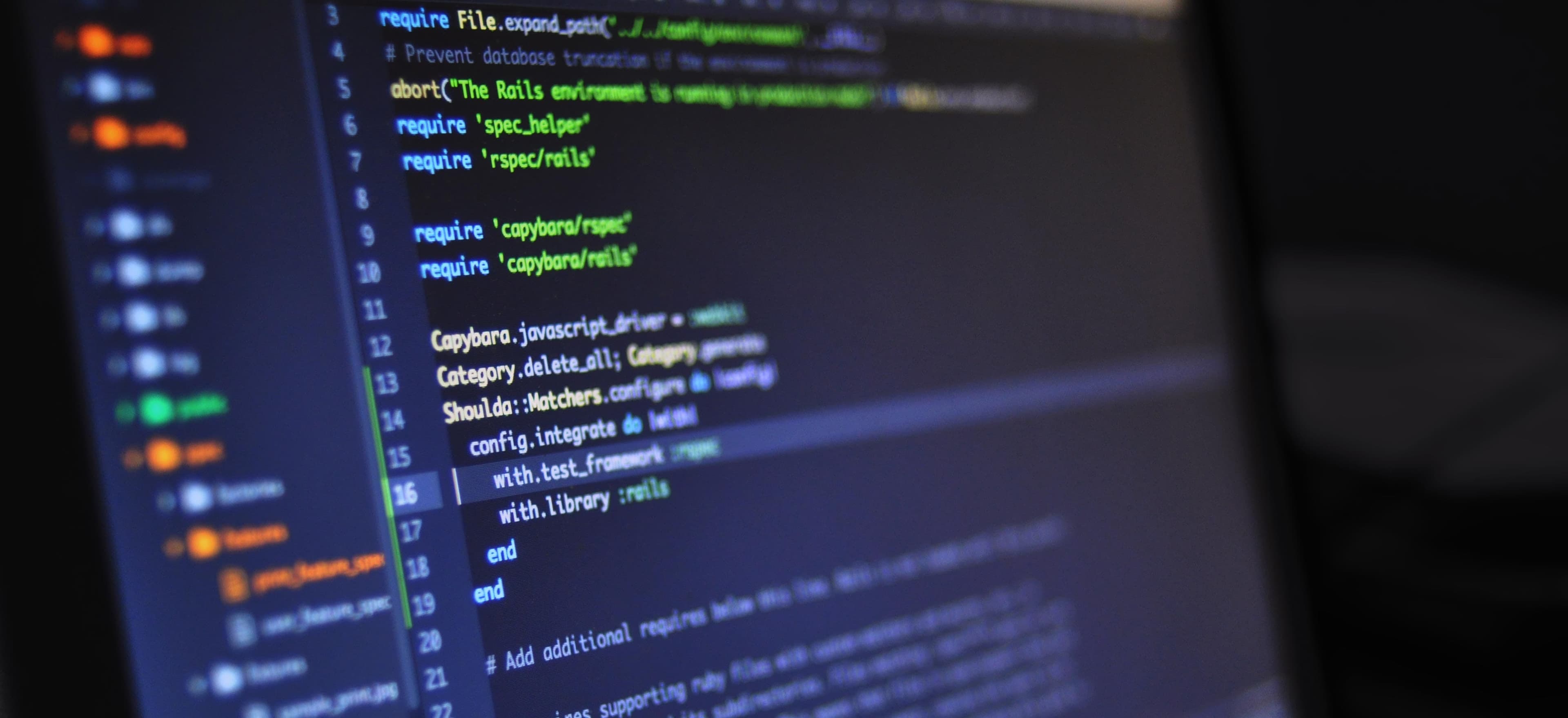
- Published on
Common Issues When Setting Up Spring Boot CI Pipelines
Continuous Integration (CI) is a fundamental practice in modern software development that helps ensure code quality and reliability through automated testing and deployment. When working with Spring Boot, a popular Java framework for building web applications, setting up CI pipelines can present several challenges. In this article, we will discuss common issues developers encounter when configuring Spring Boot CI pipelines and how to resolve them effectively.
Table of Contents
Understanding Spring Boot CI Pipelines
A Spring Boot CI pipeline automates the process of building, testing, and deploying your application whenever code changes are detected. Utilizing tools like Jenkins, GitLab CI/CD, Travis CI, or GitHub Actions enables teams to streamline this workflow and minimize human error. A well-configured pipeline supports rapid development cycles and ensures that your code remains in a deployable state.
Common Issues
Issue 1: Dependency Conflicts
Description: Dependency conflicts arise when different modules rely on incompatible versions of libraries. This is especially prevalent in Spring Boot applications where various dependencies may transitively bring in conflicting libraries.
Solution: To resolve this:
-
Utilize the
maven-enforcer-plugin
in your Maven configuration to enforce dependency convergence. Here's an example configuration:<build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-enforcer-plugin</artifactId> <version>3.0.0-M3</version> <executions> <execution> <id>enforce-dependencies</id> <goals> <goal>enforce</goal> </goals> <configuration> <rules> <requireUpperBoundDeps /> </rules> </configuration> </execution> </executions> </plugin> </plugins> </build>
-
Run
mvn dependency:tree
to visualize your dependencies and troubleshoot conflicts. -
Keep your dependencies updated with tools like Maven Dependency Plugin to avoid older conflicting versions.
Issue 2: Testing Failures
Description: CI pipelines often fail during the testing phase due to flaky tests or environmental discrepancies. This can be frustrating, as tests can pass locally but fail in CI.
Solution: To mitigate testing failures:
-
Use Testcontainers to create a consistent testing environment that closely mirrors production. Example code for a PostgreSQL container:
@Test public void testUserRepository() { try (PostgreSQLContainer<?> postgres = new PostgreSQLContainer<>("postgres:latest")) { postgres.start(); // Your test code here } }
-
Review your test configurations and Mock Behavior carefully when dealing with external services.
-
Isolate tests and run them in parallel where possible. This reduces the risk of side effects between tests.
Issue 3: Environment Configuration
Description: Misconfiguration of environments can lead to issues when the application runs in CI compared to local tests.
Solution: Use Spring Profiles to manage environment-specific configurations:
-
Create
application-{profile}.yml
files for each environment, e.g.,application-ci.yml
for your CI pipeline. -
Load the correct profile by setting the
SPRING_PROFILES_ACTIVE
environment variable in your CI environment. -
Make sure to externalize sensitive configurations using tools like Spring Cloud Config or environment variables to avoid hardcoding values.
Issue 4: Containerization Problems
Description: When deploying containerized applications, issues can arise from incorrect Docker configurations or failed builds.
Solution: Follow best practices for Docker:
-
Use multi-stage builds to keep your images small. A simple example:
FROM openjdk:11-jdk AS build WORKDIR /app COPY . . RUN ./mvnw package FROM openjdk:11-jre WORKDIR /app COPY --from=build /app/target/*.jar app.jar ENTRYPOINT ["java","-jar","/app/app.jar"]
-
Implement health checks in your Docker containers to ensure they are running as expected. This can be done in the
Dockerfile
:HEALTHCHECK --interval=30s --timeout=10s CMD curl -f http://localhost:8080/actuator/health || exit 1
-
Run integration tests against your containers to confirm everything is working in the configured environment.
Issue 5: Security and Credential Management
Description: Storing sensitive information like API keys and database passwords in version control can lead to security breaches.
Solution: Leverage secret management tools:
-
Use Spring Cloud Vault or similar solutions to store secrets securely and access them via environment variables.
-
In your CI/CD configurations, set sensitive values as encrypted environment variables instead of hardcoding them. For example, in GitHub Actions:
secret: ${{ secrets.DB_PASSWORD }}
-
Regularly review and rotate your credentials to limit exposure risk.
Best Practices for Spring Boot CI
-
Keep Your CI/CD Configuration Simple: Avoid complex CI configurations that may introduce more failures. Start simple, then iterate.
-
Use Caching: Take advantage of caching dependencies in your CI to speed up build times and minimize redundant operations.
-
Maintain Observability: Implement logging and monitoring within your applications and CI pipelines to diagnose issues quickly. Consider using tools like ELK Stack or Prometheus for insights.
-
Automate: Automate as many processes as you can within your pipeline, such as deployments, rollbacks, and notifications.
-
Document Everything: Ensure your CI/CD practices and configurations are thoroughly documented. This supports onboarding and troubleshooting.
Lessons Learned
Setting up CI pipelines for Spring Boot applications can introduce various challenges, but understanding these common issues can lead to efficient solutions. By addressing dependency conflicts, ensuring robust testing, managing configurations correctly, and securing credentials, you can enhance the reliability of your CI processes. Implementing best practices can further improve your development workflow, leading to more predictable releases and a better overall developer experience.
For more information on CI/CD practices, check out the official Spring documentation, and explore other resources to enhance your knowledge in continuous integration and delivery in the context of Spring Boot applications.