Extracting Date and Time from Java DateTime Strings
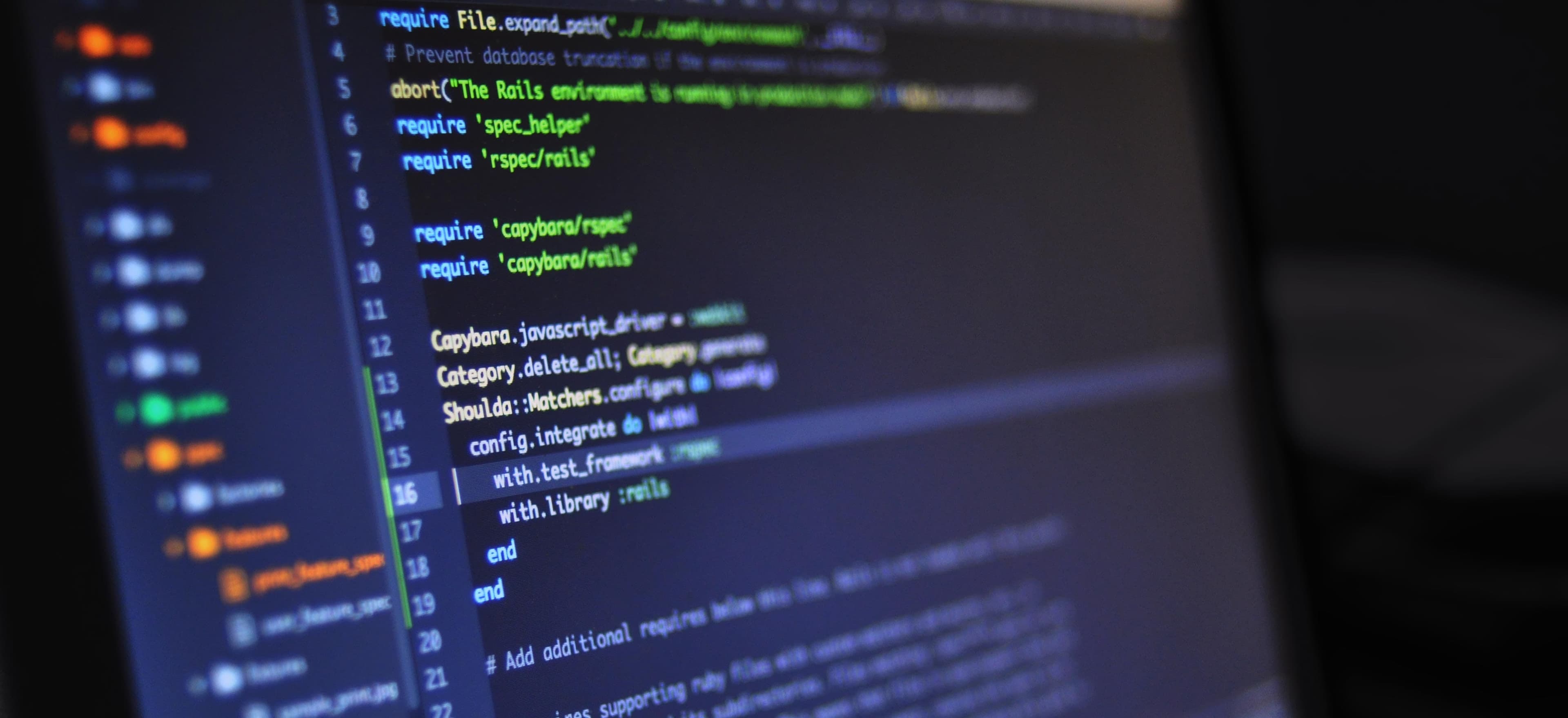
- Published on
Extracting Date and Time from Java DateTime Strings
In the modern world, working with date and time is an essential part of development, especially in enterprise applications. Java provides a robust API for managing dates and times starting in Java 8 with the introduction of the java.time
package. This post will guide you through various methods of extracting date and time from DateTime strings in Java, highlighting code samples and best practices.
Getting Started to DateTime Strings
A DateTime string typically represents a specific point in time, often formatted in accordance with ISO-8601 standards. For example:
2023-10-12T15:30:00
This string specifies the year, month, day, and time. Parsing such strings can sometimes be complicated due to various formats. Fortunately, Java's java.time
package provides a seamless way to handle these variations.
Key Classes in java.time
- LocalDate - Represents a date without a time zone (e.g., 2023-10-12).
- LocalTime - Represents a time without a date or time zone (e.g., 15:30:00).
- LocalDateTime - Combines date and time without a time zone (e.g., 2023-10-12T15:30:00).
- ZonedDateTime - Represents a full date (year, month, day) with time zone information (e.g., 2023-10-12T15:30:00Z).
Extracting Date and Time Using LocalDateTime
Code Example
Here's a basic example showing how to parse a date-time string into a LocalDateTime
object and extract the date and time components.
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class DateTimeExtractor {
public static void main(String[] args) {
// Sample DateTime String
String dateTimeString = "2023-10-12T15:30:00";
// Parse String to LocalDateTime
LocalDateTime dateTime = LocalDateTime.parse(dateTimeString);
// Extract Date
String date = dateTime.toLocalDate().toString();
// Extract Time
String time = dateTime.toLocalTime().toString();
// Output
System.out.println("Extracted Date: " + date);
System.out.println("Extracted Time: " + time);
}
}
Explanation
- Parsing: The
LocalDateTime.parse
method converts the String into aLocalDateTime
object. - Extracting Components:
toLocalDate()
andtoLocalTime()
methods are used to retrieve the date and time parts, respectively. - Output: The program prints the extracted date and time.
Output
Extracted Date: 2023-10-12
Extracted Time: 15:30:00
Custom DateTime Formats
Often, you may encounter non-standard formatting in DateTime strings. The DateTimeFormatter
class is useful for defining custom formats.
Code Example
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class CustomDateTimeExtractor {
public static void main(String[] args) {
String customDateTimeString = "12-10-2023 15:30:00";
// Define the formatter
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd-MM-yyyy HH:mm:ss");
// Parse the string into LocalDateTime
LocalDateTime dateTime = LocalDateTime.parse(customDateTimeString, formatter);
// Extract Date and Time
String date = dateTime.toLocalDate().toString();
String time = dateTime.toLocalTime().toString();
// Output
System.out.println("Extracted Date: " + date);
System.out.println("Extracted Time: " + time);
}
}
Explanation
- Custom Format: By utilizing
DateTimeFormatter.ofPattern
, you can define how to parse the given String. - Flexibility: This makes your code adaptable to various DateTime string formats, ensuring versatile date handling.
Output
Extracted Date: 2023-10-12
Extracted Time: 15:30:00
Handling Time Zones
For applications that involve multiple time zones, ZonedDateTime
becomes invaluable. This class allows you to operate with date and time while considering geographic locations.
Code Example
import java.time.ZonedDateTime;
import java.time.format.DateTimeFormatter;
public class TimeZoneExtractor {
public static void main(String[] args) {
String zonedDateTimeString = "2023-10-12T15:30:00+01:00[Europe/Paris]";
// Parse String to ZonedDateTime
ZonedDateTime zonedDateTime = ZonedDateTime.parse(zonedDateTimeString);
// Extract Date and Time
String date = zonedDateTime.toLocalDate().toString();
String time = zonedDateTime.toLocalTime().toString();
// Output
System.out.println("Extracted Date: " + date);
System.out.println("Extracted Time: " + time);
}
}
Explanation
- Time Zones: The
ZonedDateTime.parse
method can handle strings that include time zone information. - No Additional Formatting Required: The ISO-8601 format is automatically recognized without additional parsing format definitions.
Output
Extracted Date: 2023-10-12
Extracted Time: 15:30:00
Additional Resources
If you wish to dive deeper into Java DateTime and learn about the intricacies involved, you may consider the following resources:
- Java 8 Date and Time Tutorial
- Official Java Documentation for java.time
These links provide comprehensive insights into mastering DateTime handling in your Java applications.
The Closing Argument
Handling DateTime strings in Java need not be complicated with the java.time
package. By understanding the key classes and methods available, you can easily extract and manipulate date and time from various string formats. Whether you're working with LocalDate, LocalTime, LocalDateTime, or ZonedDateTime, the Java API offers a consistent experience.
Leveraging these techniques allows developers to create responsive applications that seamlessly manage time zones, custom formats, and standard DateTime strings. With this newfound knowledge, you're better equipped to implement date and time functionality in your next Java project. Happy coding!