Mastering Gradle: Run Just One Test Effortlessly
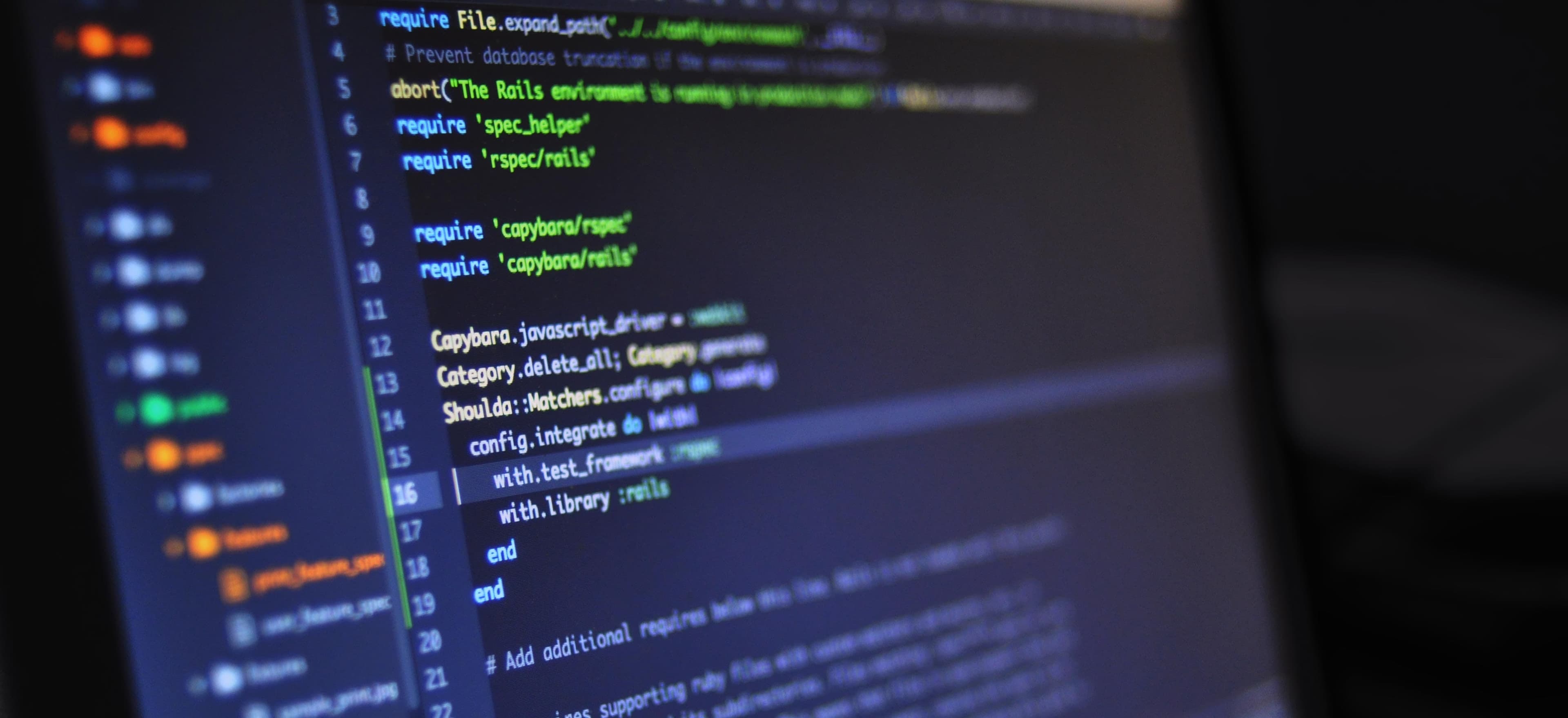
- Published on
Mastering Gradle: Run Just One Test Effortlessly
Gradle has become a staple in the world of JVM projects, especially when it comes to building Java applications. Its flexibility and powerful features allow developers to streamline their builds significantly. One common requirement that arises during development is the need to run a single test instead of executing the entire test suite. This blog post will cover how to efficiently run just one test using Gradle, along with some practical insights and code snippets.
Understanding Test Execution in Gradle
Before diving into the specifics of running a single test, let's briefly discuss how Gradle handles test execution. By default, Gradle utilizes the JUnit framework for running tests. Gradle performs unit tests with the help of a dedicated source set, and executing all tests can sometimes be time-consuming. Thus, being able to run just one test can significantly enhance productivity, especially in large codebases.
Why Run Just One Test?
- Speed: Running all tests can take a considerable amount of time depending on the size of the codebase. When you’re correcting a single function or feature, running a single test can save time.
- Debugging: You can focus on a particular failing test case without the distraction of seeing hundreds of tests run.
- Resource Management: Running fewer tests consumes fewer system resources, making it ideal for local development on under-spec hardware.
Prerequisites
Ensure you have Gradle installed on your machine and your Java project is set up with Gradle correctly. Confirm you have JUnit integrated into your project. If you haven't set it up yet, refer to the official Gradle documentation for guidance.
Command to Run a Single Test
To run just one test, you can leverage the simple command line instruction:
./gradlew test --tests "com.example.MyClassTest.myTestMethod"
Breaking Down the Command
-
./gradlew
: This part executes your Gradle wrapper script, which is crucial for ensuring the correct version of Gradle is used. If you are on Windows, you might want to usegradlew.bat
. -
test
: This keyword tells Gradle that you intend to execute tests. -
--tests
: This option specifies that you want to filter your test execution. Gradle will only run tests matching the provided pattern. -
"com.example.MyClassTest.myTestMethod"
: This is the fully qualified name of the test method you wish to execute. Make sure to replace this with the actual path and name of your test method.
Example Code Snippet
Let’s put the command in context with a simple JUnit test case:
package com.example;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class MyClassTest {
@Test
public void myTestMethod() {
assertEquals(5, add(2, 3), "Should return the sum of two numbers");
}
public int add(int a, int b) {
return a + b;
}
}
In this example, myTestMethod
tests a simple addition. To run just this test method, use the Gradle command provided above.
Running Tests with Gradle from IntelliJ IDEA
If you are using IntelliJ IDEA, you can also run a specific test directly from the IDE:
- Navigate to the test method in the editor.
- Right-click on the method name.
- Select "Run 'myTestMethod'".
This method utilizes Gradle under the hood, offering a visual and interactive way to run your tests.
More Fine-Grained Control
Using Test Task Configuration
Sometimes, you may need even more control over your tests. Here’s how to configure the Gradle task for running tests programmatically:
tasks.named('test') {
useJUnitPlatform()
testLogging {
events "passed", "skipped", "failed"
}
}
By modifying the test
task, you can control how the test results are logged and even set up various test execution parameters. This can be extremely helpful to tailor the output to your needs.
Running Tests with Tags
In versions of JUnit that support tagging (JUnit 5 and above), you can run tests based on specific tags. Here’s how:
./gradlew test --tags "slow"
This command will run all tests marked with the tag "slow". Ensure you have applied tags to your tests as shown below:
import org.junit.jupiter.api.Tag;
import org.junit.jupiter.api.Test;
public class MyClassTest {
@Test
@Tag("slow")
public void mySlowTestMethod() {
// Test implementation
}
}
This feature aids in organizing your tests based on their execution time, ensuring you only run necessary tests during certain phases of your development.
To Wrap Things Up
Mastering Gradle’s capability to run a single test efficiently can significantly improve your development workflow. By understanding the commands and configurations available to you, you can enhance your productivity and focus on what really matters: building robust applications. Whether you are executing tests through the CLI or from your IDE, the power of Gradle ensures you have the tools at your disposal to manage your Java test suite effectively.
For further reading on testing in Gradle, check out the Gradle documentation to enhance your understanding and capabilities with this powerful build tool.
Call to Action
If you found this guide helpful, please share it with your development peers. Stay tuned for more posts on advanced Gradle functionalities, and don’t hesitate to leave a comment if you have any questions or suggestions!
Happy coding!