Troubleshooting Ehcache Integration in OpenXava Applications
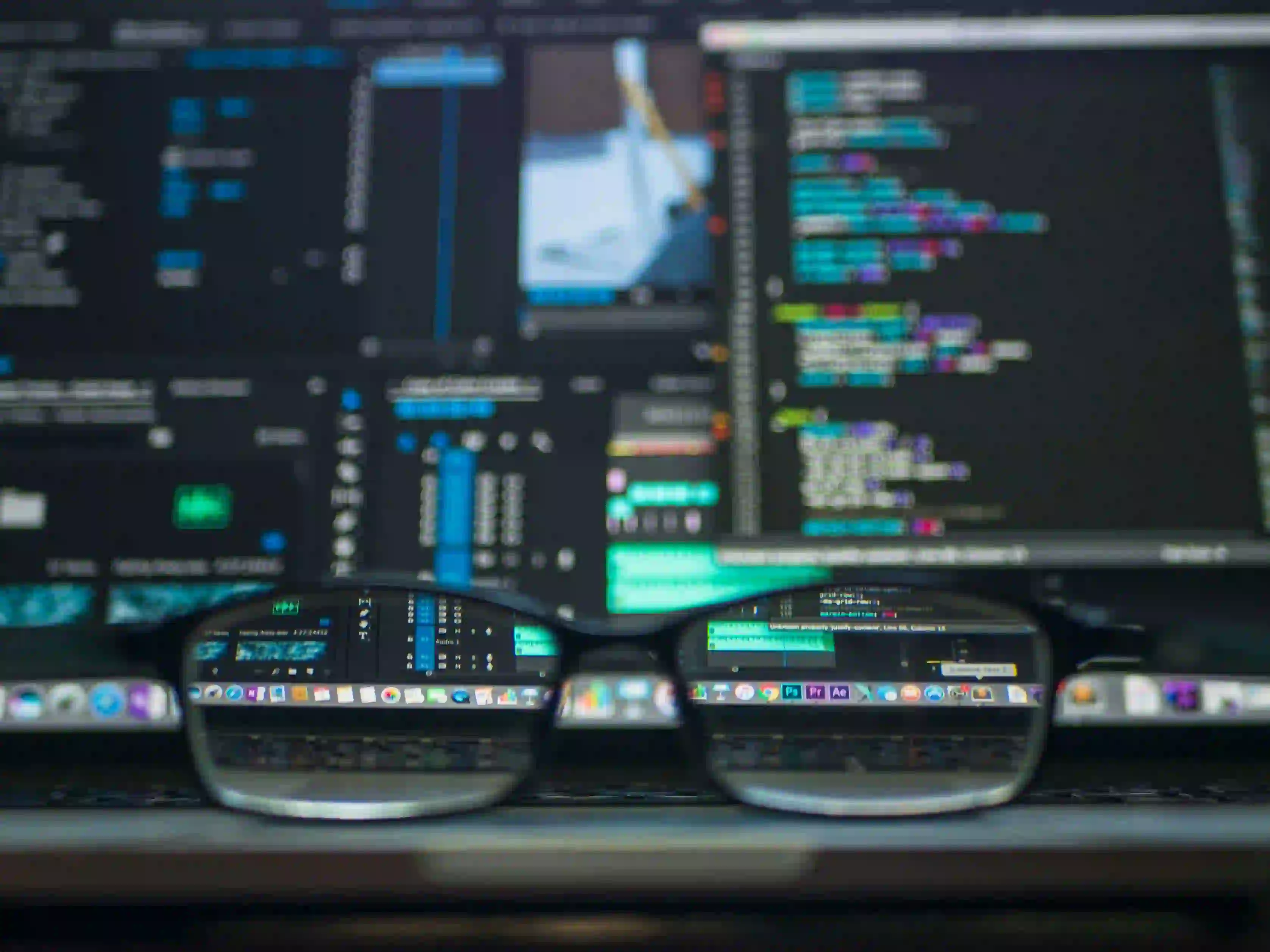
Troubleshooting Ehcache Integration in OpenXava Applications
OpenXava is a powerful framework for developing Java-based business applications with minimal effort. One common aspect of application performance optimization is the effective use of caching. Ehcache, a widely used caching library, can significantly enhance the performance of an OpenXava application by reducing database load and speeding up response times. However, like many integrations, implementing Ehcache with OpenXava can present challenges. This blog post aims to provide you with a comprehensive guide for troubleshooting Ehcache integration issues in OpenXava applications.
Understanding Ehcache Integration
What is Ehcache?
Ehcache is an open-source caching solution for Java applications. It provides a simple and flexible API for managing caching with various features such as caching of persistent data, in-memory caching, and distributed caching capabilities.
OpenXava Overview
OpenXava is a framework that simplifies Java EE web applications through a variety of built-in features, including automatic UI generation, validation, and entity management. It leverages Java Persistence API (JPA) for data access, which can benefit from caching mechanisms like Ehcache to improve performance.
Benefits of Caching
- Reduced Database Load: Caching repetitive database queries reduces the number of calls to the database.
- Improved Performance: Faster data retrieval means users experience less latency.
- Scalability: Applications can handle higher loads without necessitating heavier database infrastructure.
Integrating Ehcache with OpenXava
Step 1: Adding Dependencies
First, confirm that you have the required dependencies in your pom.xml
if you are using Maven. Here's how to add Ehcache:
<dependency>
<groupId>net.sf.ehcache</groupId>
<artifactId>ehcache</artifactId>
<version>2.10.6</version>
</dependency>
Adding this dependency allows you to use Ehcache features in your application.
Step 2: Configuration of Ehcache
Next, create an ehcache.xml
configuration file in your resources directory. This file defines how cache is created, managed, and utilized. Here is a sample configuration:
<ehcache xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="http://ehcache.org/ehcache.xsd"
updateCheck="false">
<defaultCache
maxEntriesLocalHeap="1000"
eternal="false"
timeToIdleSeconds="120"
timeToLiveSeconds="120"
memoryStoreEvictionPolicy="LRU" />
<cache name="myEntityCache"
maxEntriesLocalHeap="5000"
eternal="true"
memoryStoreEvictionPolicy="LRU" />
</ehcache>
In this configuration:
- maxEntriesLocalHeap specifies the maximum number of entries the cache can hold.
- eternal means the cache entry never expires.
- timeToIdleSeconds defines the number of seconds an entry can remain in cache without being accessed.
Step 3: Using Ehcache with OpenXava
Once configured, you can integrate Ehcache into your OpenXava application by annotating your entity classes. For instance:
@Entity
@Cacheable
@Cache(usage = CacheConcurrencyStrategy.READ_WRITE)
public class MyEntity {
@Id
private Long id;
private String name;
// Getters and Setters
}
Using annotations like @Cacheable
and @Cache
informs the framework to utilize caching for this entity.
Troubleshooting Common Issues
Despite thorough implementation, you may encounter issues during the integration of Ehcache with OpenXava. Here are some typical problems and their solutions.
1. Cache Not Being Used
Possible Causes:
- Incorrect cache configuration.
- Not annotating the entities properly.
Solution:
Double-check your ehcache.xml
file for correct configuration. Ensure that the cache names you’re referencing in annotations match those defined in your XML.
2. Stale Data
Possible Causes:
- Cache entries not updated after data changes.
Solution:
Implement cache invalidation strategies. When updating or deleting entities, ensure you clear the cache:
public void updateMyEntity(MyEntity entity) {
myEntityRepository.save(entity);
cacheManager.getCache("myEntityCache").remove(entity.getId());
}
This ensures users always access the most current data.
3. Cache Performance Issues
Possible Causes:
- Poor specification of cache size.
- Eager fetching of data instead of lazy loading.
Solution:
Increase the size of your cache in ehcache.xml
if memory allows. Investigate eager vs. lazy loading based on the application's data access patterns.
4. Class Not Found Exception
Possible Causes:
- Missing Ehcache dependency in your classpath.
Solution:
Ensure your module's classpath includes the Ehcache library. If you're using Maven, do a mvn clean install
to refresh your dependencies.
5. Exception Handling
Capture and log exceptions, as they can provide insight into underlying issues. Efficient logging is critical during debugging:
try {
// try to access cache or database
} catch (Exception e) {
Logger.error("Cache access failed", e);
}
Wrapping Up
Integrating Ehcache into your OpenXava applications can be a straightforward process, as long as you understand the framework and its caching capabilities. By following the steps outlined and keeping an eye out for common pitfalls, you can take full advantage of caching. This optimization can serve to significantly enhance your application's performance and user satisfaction.
Should you need more detailed guidance or community support, resources such as the OpenXava Forum or the Ehcache Documentation are excellent starting points.
Implementing caching successfully will not only improve user experience but also pave the way to scaling your application efficiently. Happy coding!