Understanding Functors: Simple Java Examples Explained
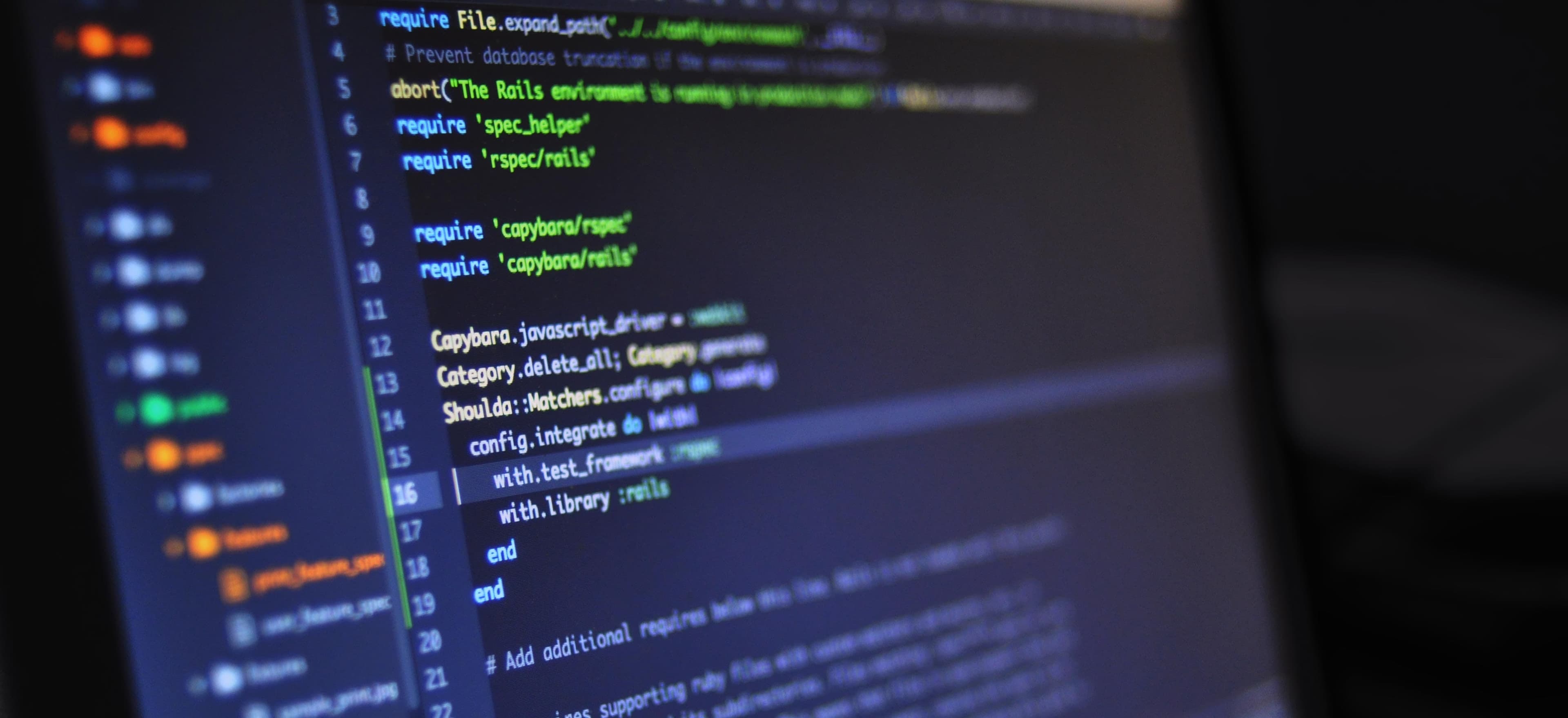
- Published on
Understanding Functors: Simple Java Examples Explained
In functional programming, the concept of functors plays an essential role in abstracting and managing computations. Although the term originates from category theory, it has found a niche in languages like Java, contributing to better code organization and readability. This blog post will demystify functors in Java, providing clear explanations and practical examples along the way.
What is a Functor?
A functor can be considered as a type that implements a map functionality, allowing you to apply a function over wrapped values. In simpler terms, it's a container over values that enables operations on the contained values without explicitly extracting them. To bring this into context, in Java, functors are represented as objects that encapsulate behavior.
Functors in Java: Why Important?
- Abstraction: Functors abstract operations on values, making it easier to compose operations and manage side effects.
- Reusability: They enable code reusability by applying the same operation to different data structures seamlessly.
- Maintainability: With clear functional abstractions, the codebase becomes easier to maintain and reason about.
Creating a Functor in Java
Let's start by creating a simple functor in Java using generics. Below is a code snippet demonstrating how to implement a basic functor encapsulating an integer value.
Example of a Simple Functor
public class Functor<T> {
private T value;
public Functor(T value) {
this.value = value;
}
public <R> Functor<R> map(Function<T, R> mapper) {
R newValue = mapper.apply(value);
return new Functor<>(newValue);
}
public T getValue() {
return value;
}
public static void main(String[] args) {
Functor<Integer> functor = new Functor<>(5);
Functor<Integer> incrementedFunctor = functor.map(value -> value + 1);
System.out.println(incrementedFunctor.getValue()); // Output: 6
}
}
Code Explanation
- Functor Class: This generic class takes a type parameter
T
, focusing on holding a single value. - Constructor: It initializes the
value
property of the functor. - map Method: This method takes a function as an argument. The function is applied to the contained value, producing a new functor encapsulating the result.
- getValue Method: This provides access to the inner value.
In the main
method, we create an instance of Functor
with an integer value of 5. The map
method is then called with a lambda function that increments the value. As a result, the incrementedFunctor
contains 6, demonstrating how functors allow easy transformations with encapsulated behavior.
Functors with Collections
Let’s explore how we can utilize functors with collections. Collections are a perfect example where functors shine, allowing us to manipulate collections of data more elegantly.
Functor with a List
import java.util.*;
import java.util.function.Function;
public class ListFunctor {
public static <T, R> List<R> map(List<T> list, Function<T, R> mapper) {
List<R> result = new ArrayList<>();
for (T item : list) {
result.add(mapper.apply(item));
}
return result;
}
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
List<Integer> squaredNumbers = map(numbers, value -> value * value);
System.out.println(squaredNumbers); // Output: [1, 4, 9, 16, 25]
}
}
Code Explanation
- map Method: Here, we create a static method that takes a list and a function. It iterates through each item of the list, applies the function, and collects the results in a new list.
- Lambda Function: In
main
, we demonstrate the convenience of ourmap
function through a list of integers that we square.
By encapsulating the mapping behavior in our own function, we gain better code organization and can apply it to any List
.
Benefits of Using Functors
Simplifying Code
Using functors can lead to less cluttered code:
- You avoid manual handling of wrapped values.
- Logic aligns closer to its functional transformations rather than manual iteration.
Emphasizing Immutability
With functors, you promote immutability by returning new instances rather than modifying existing ones. This aspect is particularly beneficial in concurrent programming.
Enhanced Readability
The functional style can be more readable, clearly expressing transformations of values.
Limitations of Functors
It is vital to acknowledge that while functors provide numerous advantages, they also have some limitations:
- Learning Curve: The concept can be initially confusing for those used to imperative programming models.
- Performance: In some scenarios, functors might introduce overhead compared to more direct approaches.
Real-World Applications
Functors come handy in various real-world applications, including:
- Data Transformation: When dealing with APIs or data sources, employing functors to transform response bodies is common.
- Functional Composition: Constructing pipelines for data processing, where several operations follow one another seamlessly.
- Error Handling: Using functors can encapsulate failure handling elegantly, distinguishing between values and error states.
Lessons Learned
Functors in Java are a powerful tool in managing computations and transformations fluidly. By understanding the principles behind them, developers can write more maintainable, reusable, and elegant code.
If you wish to dive deeper into functional programming in Java, consider exploring Java 8 lambdas and functional interfaces.
By embracing concepts like functors, you enrich your Java programming skills, making code more dynamic and expressive. Happy coding!