Java-Based Solutions for Emergency Preparedness Apps
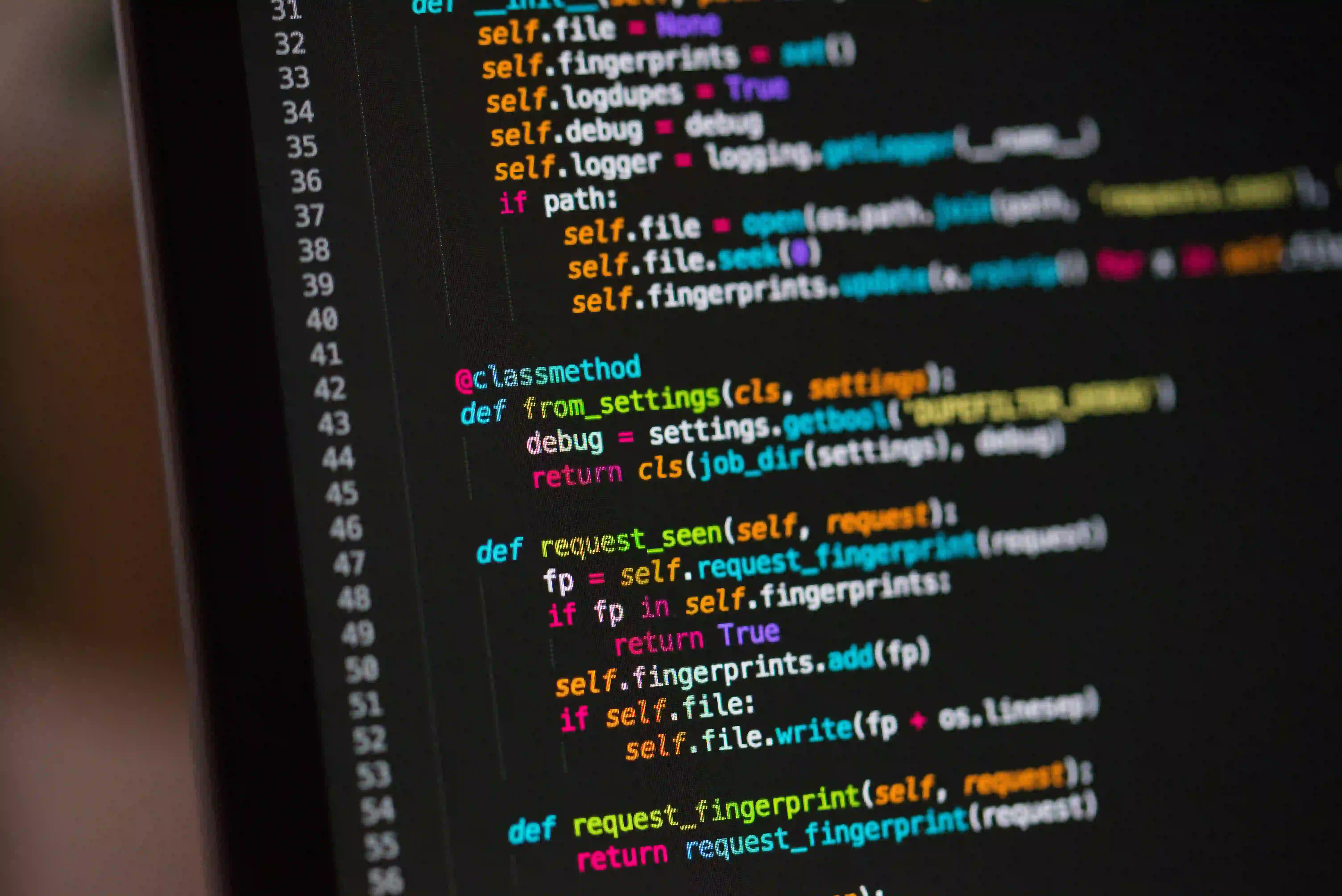
Java-Based Solutions for Emergency Preparedness Apps
In an increasingly unpredictable world, emergency preparedness has evolved into a movement imbued with creativity, innovation, and, dare we say, necessity. To adapt efficiently, many individuals are turning towards technology for solutions that address their preparedness needs. One effective way to engage in this tech-savvy approach is through Java programming to develop emergency preparedness applications. In this article, we'll delve into the fundamentals of creating a Java-based emergency preparedness app, explore best practices in coding, and draw inspiration from the "Survival and Faith: Navigating the Mormon Prepper Movement" youvswild.com/blog/survival-faith-mormon-prepper-movement.
The Necessity of Emergency Preparedness Apps
Before we dive into coding, let’s consider why emergency preparedness apps are essential. Natural disasters, pandemics, and other emergencies can occur without warning, making it crucial for individuals to have the right information and tools at their fingertips. An effective app can provide:
- Real-time Updates: Notifications regarding alerts and situation changes.
- Resource Management: Inventory tracking for emergency supplies.
- Community Alerts: Communication networks for community members.
By focusing on these areas, we can create impactful applications that serve users well in times of crisis.
Choosing Java for Your Emergency Preparedness Application
Java is a powerful programming language well-suited for building mobile and web applications. Here are several reasons why Java stands out in this context:
-
Platform-Independence: Java applications can run on any device with a Java Virtual Machine (JVM), making your app accessible across different platforms.
-
Robust Libraries: With a rich API and extensive libraries, Java supports various functionalities like data networking and GUI design.
-
Strong Community Support: A large developer community means abundant resources, tutorials, and troubleshooting assistance.
Now, let’s get started on building a simple emergency preparedness application in Java.
Building a Simple Emergency Preparedness App
For this demonstration, we will create an emergency supply tracker. This app will keep track of essential items, their quantities, and expiration dates. The goal is to provide a clear interface and easy-to-use functionalities.
Setting Up the Project
To initiate the project:
- Choose an IDE: Install an Integrated Development Environment (IDE) like IntelliJ IDEA or Eclipse.
- Create a New Java Project: Designate your project as "EmergencySupplyTracker".
- Add Necessary Libraries: For this basic app, we’ll utilize the built-in Java libraries.
Creating the Main Application
Here’s a simple representation of the main application in Java.
import java.util.ArrayList;
import java.util.Scanner;
class Item {
String name;
int quantity;
String expirationDate;
public Item(String name, int quantity, String expirationDate) {
this.name = name;
this.quantity = quantity;
this.expirationDate = expirationDate;
}
@Override
public String toString() {
return "Item: " + name + ", Quantity: " + quantity + ", Expires on: " + expirationDate;
}
}
public class EmergencySupplyTracker {
private ArrayList<Item> supplyList;
public EmergencySupplyTracker() {
supplyList = new ArrayList<>();
}
public void addItem(String name, int quantity, String expirationDate) {
supplyList.add(new Item(name, quantity, expirationDate));
}
public void displaySupplies() {
for (Item item : supplyList) {
System.out.println(item);
}
}
public static void main(String[] args) {
EmergencySupplyTracker tracker = new EmergencySupplyTracker();
Scanner scanner = new Scanner(System.in);
System.out.println("Welcome to the Emergency Supply Tracker!");
boolean running = true;
while (running) {
System.out.println("Enter command: 1) Add Item 2) Show Supplies 3) Exit");
int command = scanner.nextInt();
scanner.nextLine(); // Consume newline
switch (command) {
case 1:
System.out.println("Enter item name:");
String name = scanner.nextLine();
System.out.println("Enter item quantity:");
int quantity = scanner.nextInt();
scanner.nextLine(); // Consume newline
System.out.println("Enter expiration date (YYYY-MM-DD):");
String expirationDate = scanner.nextLine();
tracker.addItem(name, quantity, expirationDate);
break;
case 2:
tracker.displaySupplies();
break;
case 3:
running = false;
break;
default:
System.out.println("Invalid command. Try again.");
}
}
scanner.close();
}
}
Code Explanation
-
Items Class: This object-oriented structure manages individual supply items. Each item has a name, quantity, and expiration date.
-
Main Application: The
EmergencySupplyTracker
acts as the engine for our app. It creates anArrayList
to store multiple items. -
Adding Items: Users are prompted to enter the specifics for each supply item. This data is then captured and stored in the list.
-
Displaying Supplies: Users can view all entered items, utilizing the
toString()
method for formatted output.
Running the Application
Compile and run your project. You will be greeted with a simple command-line interface. Enter items to populate your emergency supply list, or view them with a specific command.
Enhancing Your Application
To turn a basic app into a comprehensive solution, consider integrating additional features, such as:
-
Data Persistence: Store data using a database (like SQLite) or simple file storage to maintain records between sessions.
-
Push Notifications: Implement notifications reminding users of upcoming expiration dates or necessary restocks.
-
Geolocation Services: To provide relevant information, integrate APIs that could give users resources based on their location.
-
Multilingual Support: Considering diverse user bases, allowing multiple languages can make your app more inclusive.
Stay Informed and Connected
As mentioned in the introduction, nature and preparedness are often at the forefront of discourse. A deeper understanding of the cultural and historical contexts behind these movements—like those explored in the article "Survival and Faith: Navigating the Mormon Prepper Movement"—can provide valuable insights into developing more tailored solutions for specific communities. Be sure to check out the article for additional perspectives!
The Closing Argument
Java's versatility combined with a user-centered design approach can lead to powerful emergency preparedness applications. Our simple supply tracker serves as a basic foundation, paving the way for a more robust, functional app. By adding features, ensuring usability, and engaging with the preparedness community, you can create an app that genuinely helps people when they need it most.
Stay prepared, stay safe, and keep coding!