Harnessing Java to Unlock Your Zodiac Energy Dynamics
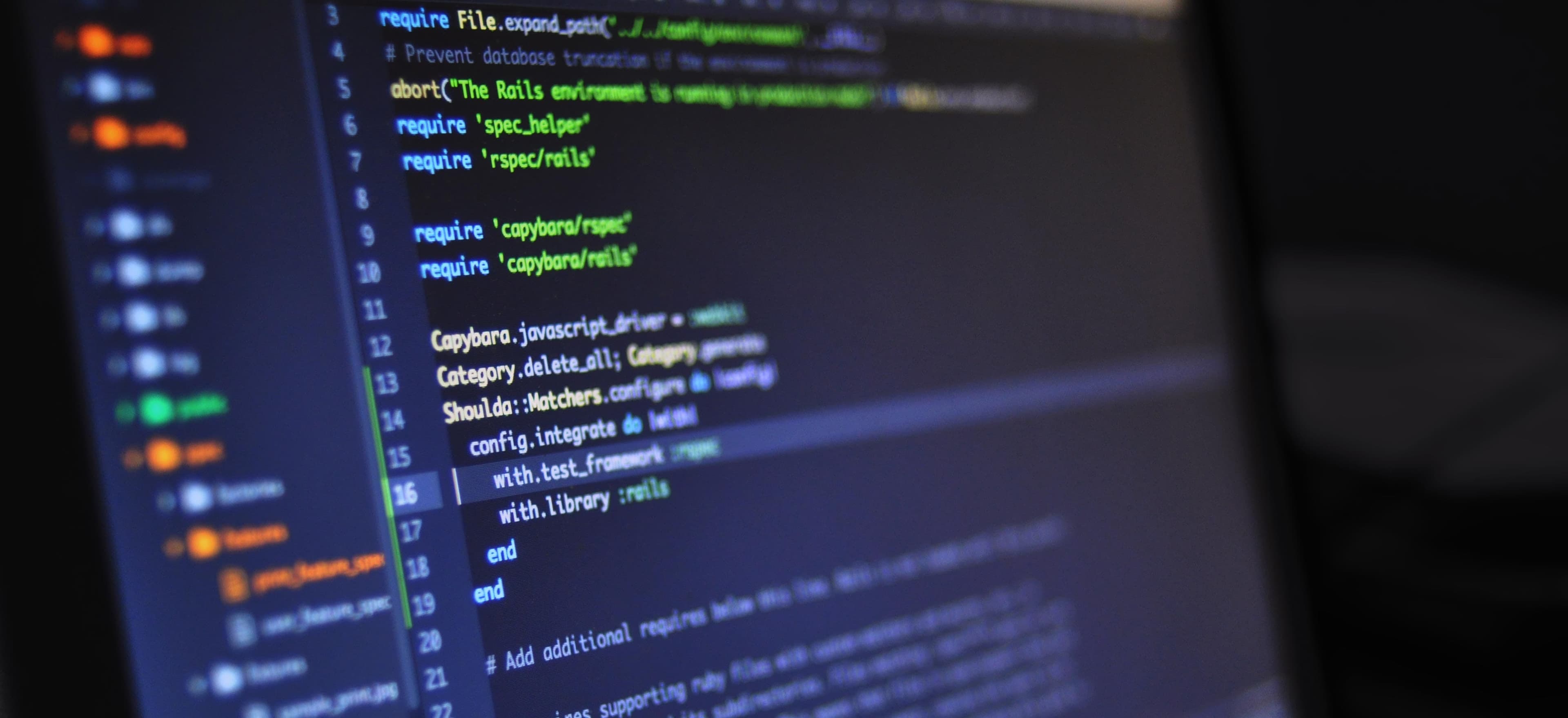
- Published on
Harnessing Java to Unlock Your Zodiac Energy Dynamics
Astrology enthusiasts often seek ways to connect their zodiac signs with various aspects of their lives. One particularly interesting approach is using Java programming to reveal patterns and insights that correlate with astrological beliefs. This article will explore how Java can be leveraged to unlock the dynamics of zodiac energies, steering our lives in positive directions.
For those who have an interest in astrology, you may want to check out the article titled “Widder: Entfessel die Kraft deiner Sternzeichen-Energie!” which highlights the importance of understanding zodiac energies. In this article, we'll bridge that discussion with practical Java programming concepts.
Understanding Zodiac Signs
Before we dive into coding, let’s take a moment to understand what zodiac signs represent. Each of the twelve zodiac signs is linked to specific traits, characteristics, and energies. For instance, Aries is often seen as dynamic and energetic, while Taurus is more about stability and patience.
By identifying these traits, we can programmatically associate different actions or responses within our Java applications. Let's explore some fundamental Java techniques to represent and manipulate zodiac attributes.
Java Classes for Zodiac Signs
First, we will create a class to encapsulate the properties of a zodiac sign. This approach promotes encapsulation and modular coding:
public class ZodiacSign {
private String name;
private String element;
private String modality;
private String strengths;
private String weaknesses;
public ZodiacSign(String name, String element, String modality, String strengths, String weaknesses) {
this.name = name;
this.element = element;
this.modality = modality;
this.strengths = strengths;
this.weaknesses = weaknesses;
}
public String getName() {
return name;
}
public String getElement() {
return element;
}
public String getModality() {
return modality;
}
public String getStrengths() {
return strengths;
}
public String getWeaknesses() {
return weaknesses;
}
}
Explanation
This ZodiacSign
class encapsulates vital information about each zodiac sign:
- Attributes: Each zodiac sign holds properties such as its name, element, modality (cardinal, fixed, mutable), strengths, and weaknesses.
- Constructor: The constructor initializes these attributes, allowing for easy instantiation of ZodiacSign objects.
- Getters: Getters provide access to the properties, promoting good encapsulation.
Extending the Class
Next, we can create instances of this class to represent a few zodiac signs:
public class Main {
public static void main(String[] args) {
ZodiacSign aries = new ZodiacSign("Aries", "Fire", "Cardinal", "Courageous, Determined", "Impulsive, Aggressive");
ZodiacSign taurus = new ZodiacSign("Taurus", "Earth", "Fixed", "Reliable, Patient", "Stubborn, Possessive");
System.out.println("Zodiac: " + aries.getName());
System.out.println("Element: " + aries.getElement());
System.out.println("Strengths: " + aries.getStrengths());
System.out.println("Weaknesses: " + aries.getWeaknesses());
System.out.println();
System.out.println("Zodiac: " + taurus.getName());
System.out.println("Element: " + taurus.getElement());
System.out.println("Strengths: " + taurus.getStrengths());
System.out.println("Weaknesses: " + taurus.getWeaknesses());
}
}
Output
When executing the above code, you will see the details of Aries and Taurus displayed in a structured format. This allows you to quickly reference a sign's attributes for further processing.
Mapping Zodiac Signs to Behavioral Traits
To build further upon our foundational knowledge, let’s create a mapping system that ties zodiac signs to specific behavioral traits. This can help us simulate how a person's zodiac sign might influence their decisions or actions.
Creating a Trait Class
Here's an example Trait
class that can be linked to a zodiac sign:
public class Trait {
private String description;
public Trait(String description) {
this.description = description;
}
public String getDescription() {
return description;
}
}
Linking Traits to Zodiac Signs
You can then modify the ZodiacSign
class to include a list of traits:
import java.util.ArrayList;
import java.util.List;
public class ZodiacSign {
private String name;
private String element;
private String modality;
private String strengths;
private String weaknesses;
private List<Trait> traits;
public ZodiacSign(String name, String element, String modality, String strengths, String weaknesses) {
this.name = name;
this.element = element;
this.modality = modality;
this.strengths = strengths;
this.weaknesses = weaknesses;
this.traits = new ArrayList<>();
}
public void addTrait(Trait trait) {
traits.add(trait);
}
public List<Trait> getTraits() {
return traits;
}
}
Adding Traits
Now you can easily assign various traits to a zodiac sign in your main application:
public class Main {
public static void main(String[] args) {
ZodiacSign aries = new ZodiacSign("Aries", "Fire", "Cardinal", "Courageous, Determined", "Impulsive, Aggressive");
aries.addTrait(new Trait("Leadership"));
aries.addTrait(new Trait("Adventurous"));
for (Trait trait : aries.getTraits()) {
System.out.println("Trait: " + trait.getDescription());
}
}
}
Output
The updated code will now include traits specific to Aries, demonstrating how to link astrological attributes to Java objects effectively.
Creating an Astrological Influencer System
With our foundational classes in place, we can create aspects like an astrological influencer system that predicts outcomes based on a user’s zodiac sign. This prediction can be in terms of daily activities, career choices, or personal growth suggestions.
Example Scenario
Here’s a simple method that generates a personalized suggestion based on the zodiac sign:
public String generateSuggestion(ZodiacSign sign) {
String suggestion;
switch (sign.getName()) {
case "Aries":
suggestion = "Today is a great day for new beginnings. Take a bold step!";
break;
case "Taurus":
suggestion = "Focus on your financial stability, and don’t rush decisions.";
break;
default:
suggestion = "Follow your heart and stay true to your values.";
}
return suggestion;
}
Integrating It All
You can integrate this suggestion mechanism into your main Java application as shown below:
public class Main {
public static void main(String[] args) {
ZodiacSign aries = new ZodiacSign("Aries", "Fire", "Cardinal", "Courageous, Determined", "Impulsive, Aggressive");
// Add some traits
aries.addTrait(new Trait("Leadership"));
aries.addTrait(new Trait("Adventurous"));
System.out.println("Suggestion for " + aries.getName() + ": " + generateSuggestion(aries));
}
public static String generateSuggestion(ZodiacSign sign) {
String suggestion;
switch (sign.getName()) {
case "Aries":
suggestion = "Today is a great day for new beginnings. Take a bold step!";
break;
case "Taurus":
suggestion = "Focus on your financial stability, and don’t rush decisions.";
break;
default:
suggestion = "Follow your heart and stay true to your values.";
}
return suggestion;
}
}
Wrap-Up: Java Meets Astrology
In this article, we demonstrated how to leverage Java programming to bring zodiac energies into a structured coding environment. The concepts we covered allowed us to define zodiac signs, associate traits, and even create personalized suggestions based on these signs.
If you want to further explore the intersection of astrology and programming, consider reading articles like “Widder: Entfessel die Kraft deiner Sternzeichen-Energie!”. Such resources can enhance your understanding and provide deeper insights into how you can harness astrological energies effectively.
Let the coding begin, and may your zodiac sign lead you to new avenues of personal and professional growth!
Checkout our other articles