Boost Your Java Testing Efficiency: Automate with Ease!
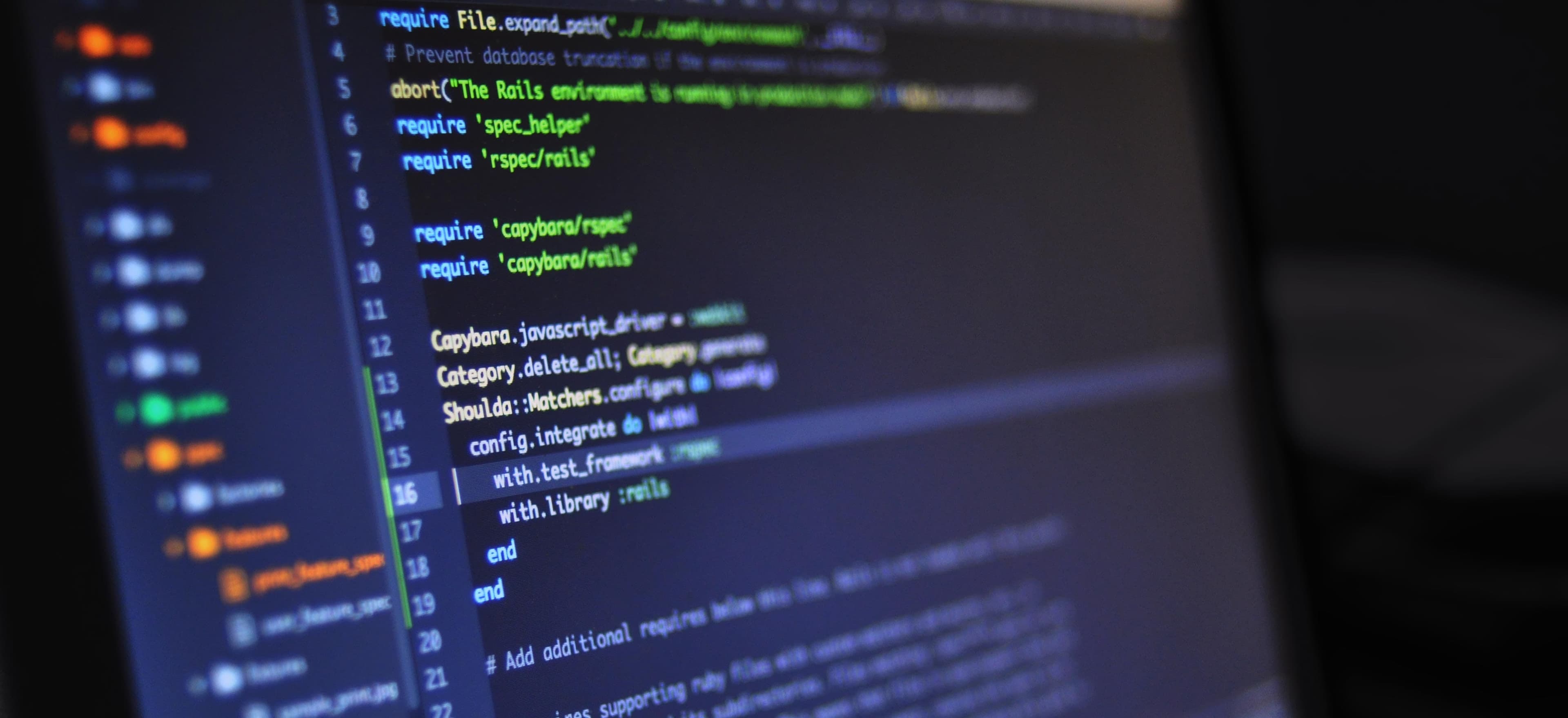
- Published on
Boost Your Java Testing Efficiency: Automate with Ease!
In an era where rapid development cycles are the norm, fostering robust testing is vital. For Java developers, automated testing presents a unique opportunity to enhance efficiency without compromising code quality. A recent article titled Mastering Quick Automation: 150 Tests in 30 Minutes! provides insights into how you can streamline your testing process. In this blog post, we will dive into strategies, frameworks, and code snippets that can help you optimize your Java testing efforts.
Understanding the Importance of Automated Testing
Automated testing allows developers to run tests consistently to identify issues early in development. It reduces the manual overhead associated with testing, enabling more time for other critical tasks. Key benefits include:
- Speed: Automated tests reduce test execution time, enabling quick feedback.
- Consistency: Automated tests are run the same way every time, eliminating the risk of human error.
- Scalability: Easily scalable tests facilitate large codebases and expanding features.
Choosing the Right Testing Framework
When it comes to Java, there are several frameworks available for automated testing. Popular choices include:
- JUnit: Widely used for unit testing.
- Mockito: Excellent for mocking objects.
- Selenium: Great for testing web applications.
- TestNG: A versatile testing framework that supports data-driven testing.
For our examples, we will focus primarily on JUnit and Mockito, as they are foundational tools in the Java testing ecosystem.
Setting Up Your Testing Environment
Before automating your tests, you need to set up your environment. This involves:
- Install JUnit and Mockito: You can add them to your Maven
pom.xml
file:
<dependencies>
<!-- JUnit Dependency -->
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>5.8.1</version>
<scope>test</scope>
</dependency>
<!-- Mockito Dependency -->
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
<version>3.12.4</version>
<scope>test</scope>
</dependency>
</dependencies>
This setup ensures that your project is equipped with the tools needed for efficient automated testing.
Writing Your First Unit Test with JUnit
Code Example
Let’s create a simple Java class called Calculator
that provides basic arithmetic operations.
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public int subtract(int a, int b) {
return a - b;
}
}
Now, we will create a unit test for the Calculator
class using JUnit.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3), "Optional assertion message");
}
@Test
public void testSubtract() {
Calculator calculator = new Calculator();
assertEquals(1, calculator.subtract(3, 2), "Optional assertion message");
}
}
Commentary
- The
@Test
annotation marks each method as a test case. assertEquals
verifies that the expected outcome matches the actual result.- Good practice is to include an optional message in assertions to clarify test failures.
Running the Tests
You can run your tests using an IDE like IntelliJ IDEA or Eclipse. Both provide integrated tools for running JUnit tests seamlessly. The efficiency gained here is substantial—running multiple tests takes a fraction of the time compared to manual testing.
Mocking Dependencies with Mockito
In real-world applications, your code often interacts with external services. That's where Mockito comes in. It allows you to create mock objects to simulate interactions, which is excellent for unit testing.
Code Example
Suppose we have a UserService
class that depends on a UserRepository
.
public interface UserRepository {
User findById(long id);
}
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User getUser(long id) {
return userRepository.findById(id);
}
}
Now let’s write a test for UserService
.
import static org.mockito.Mockito.*;
import org.junit.jupiter.api.Test;
public class UserServiceTest {
@Test
public void testGetUser() {
// Arrange
UserRepository mockRepo = mock(UserRepository.class);
User user = new User(1, "John Doe");
when(mockRepo.findById(1)).thenReturn(user);
UserService userService = new UserService(mockRepo);
// Act
User result = userService.getUser(1);
// Assert
assertEquals("John Doe", result.getName(), "User name should match");
}
}
Commentary
In this example:
- Mocking: We create a mock of
UserRepository
to simulate its behavior. - Stubbing: We use
when...thenReturn
to specify that callingfindById(1)
should return a predefined user object. - This allows us to test
UserService
in isolation without needing a real database connection.
Continuous Integration for Testing
To maximize the benefits of automated testing, incorporate Continuous Integration (CI) tools like Jenkins, Travis CI, or CircleCI. Each time you push code:
- The CI tool will execute all tests to ensure that new changes don't break existing functionality.
- This practice fosters a "fail fast" culture, addressing issues quickly.
For a deeper dive into automation strategies, you might want to check out the aforementioned article on Mastering Quick Automation: 150 Tests in 30 Minutes!.
Final Thoughts
Automating your Java tests significantly increases efficiency and code quality. With frameworks like JUnit and Mockito, writing and maintaining tests is straightforward, allowing you to focus on writing better code. The strategies outlined above can help you optimize your testing workflow, paving the way for successful software delivery.
Key Takeaways
- Automated testing is crucial for fast-paced development.
- Framework selection is paramount; JUnit and Mockito are great starting points.
- Mocking dependencies allows for isolated unit tests without external dependencies.
- Continuous Integration enhances code robustness through regular testing.
By adopting these practices, you can elevate your testing efficiency, ensuring a smoother development process and a more stable product. Happy coding and testing!
Checkout our other articles