Boost Your Java Testing Efficiency with Quick Automation Techniques
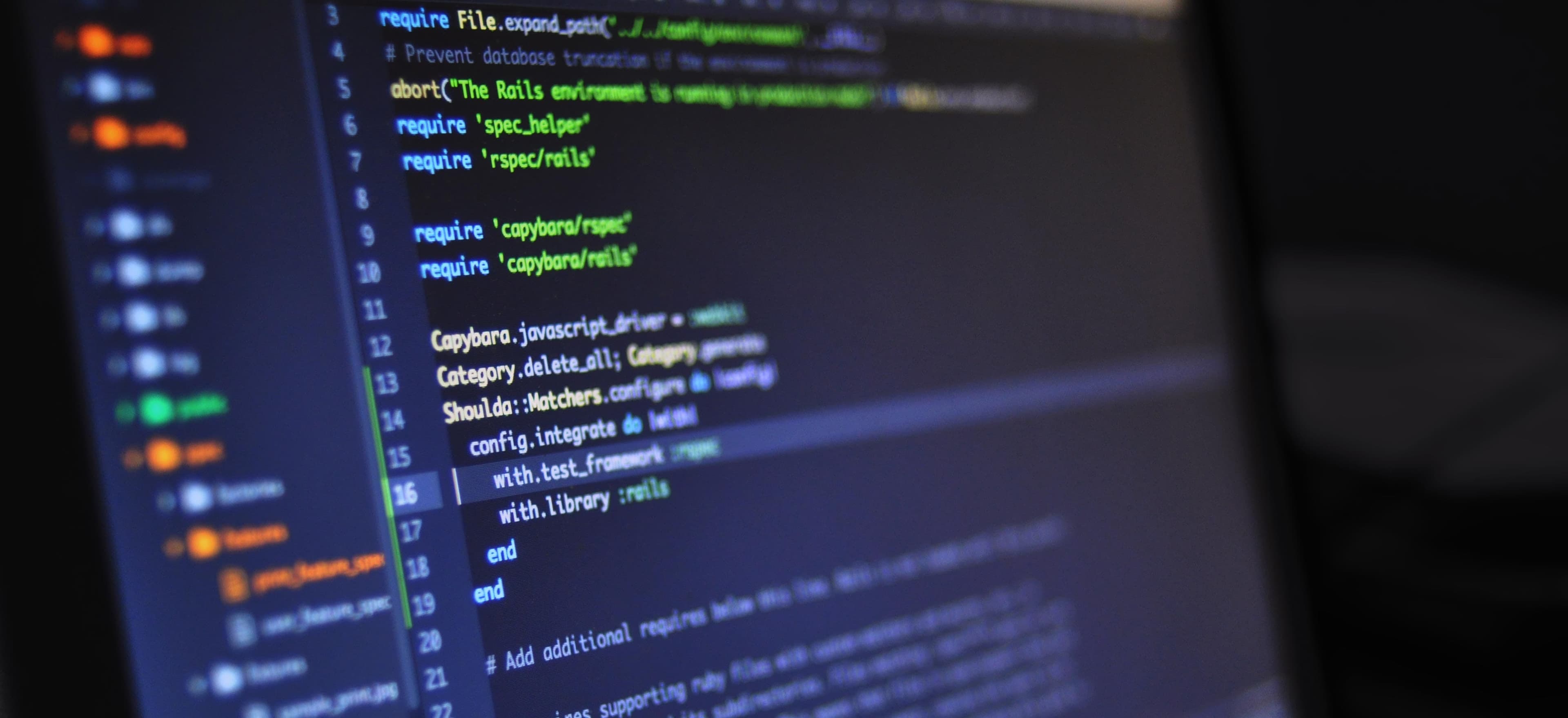
- Published on
Boost Your Java Testing Efficiency with Quick Automation Techniques
Java has long been a cornerstone of software development, and its testing frameworks are key to ensuring code quality. With the growing complexity of applications, manual testing is often insufficient. To meet the demand for speed and reliability, automation techniques come into play. This blog post focuses on optimizing your Java testing process using quick automation techniques, enabling you to conduct efficient tests while maintaining high quality.
Why Automation in Testing?
Automation in software testing reduces the time spent on repetitive tasks, ensures consistency, and enhances test accuracy. As mentioned in the existing article, Mastering Quick Automation: 150 Tests in 30 Minutes! (read the article here), leveraging automation can significantly ramp up your testing capacities.
In this post, we'll explore practical strategies you can employ to streamline your Java testing efforts.
Selecting the Right Framework
Choosing the right testing framework is crucial to successful automation. There are several options available within the Java ecosystem, including:
- JUnit - Framework for unit testing.
- TestNG - Framework that supports data-driven testing; provides powerful test configuration.
- Mockito - Framework for mocking objects during unit tests.
Each framework has its strengths and is suited for different types of testing scenarios. For instance, JUnit is excellent for unit tests, while TestNG allows greater flexibility in configuring tests.
Example: Using JUnit
Here's how you can create a simple test case using JUnit:
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calc = new Calculator();
int result = calc.add(5, 5);
assertEquals(10, result);
}
}
Why this code works:
In this example, we test a method add
from the Calculator
class. The @Test
annotation marks the method as a test case, and we use assertEquals
to verify that the outcome is as expected. This makes it easy to identify whether the method is functioning correctly.
Adopting Behavior-Driven Development (BDD)
Behavior-Driven Development (BDD) is a refinement of Test-Driven Development (TDD) that encourages collaboration between developers and non-technical stakeholders. BDD uses natural language constructs to define test cases, making them approachable for all team members.
Example: Implementing BDD with Cucumber
Cucumber is a popular BDD tool that integrates well with Java. Here’s a simple example:
Feature: Calculator
Scenario: Add two numbers
Given the calculator is available
When I add 5 and 3
Then the result should be 8
In your step definitions, you write the Java code that implements the behavior defined in the feature file.
import io.cucumber.java.en.Given;
import io.cucumber.java.en.When;
import io.cucumber.java.en.Then;
import static org.junit.Assert.assertEquals;
public class CalculatorSteps {
private Calculator calculator;
private int result;
@Given("the calculator is available")
public void setUp() {
calculator = new Calculator();
}
@When("I add {int} and {int}")
public void addNumbers(int a, int b) {
result = calculator.add(a, b);
}
@Then("the result should be {int}")
public void checkResult(int expected) {
assertEquals(expected, result);
}
}
Why this code works:
Utilizing Cucumber allows us to define scenarios in human-readable text. The step definitions then translate these steps into executable code, promoting better understanding and participative development.
Parallel Test Execution
Parallel testing allows tests to run simultaneously, significantly speeding up the execution time for your test suites. Tools like TestNG support parallel execution, which makes it easy to leverage multi-core processors effectively.
Example: Configuring TestNG for Parallel Execution
Configure the testng.xml
file to enable parallel testing:
<suite name="Parallel Tests" parallel="methods" thread-count="4">
<test name="Unit Tests">
<classes>
<class name="com.example.CalculatorTest"/>
</classes>
</test>
</suite>
Why this configuration works:
Setting the parallel
attribute to "methods" allows TestNG to run tests in parallel at the method level, and thread-count
specifies the number of threads to use. This approach leads to more efficient test execution times.
Leveraging Test Automation Tools
While frameworks and techniques are important, they often do not encompass the entire testing process. Test automation tools enhance efficiency further. Some popular tools include:
- Selenium - Automates web applications.
- JMeter - For performance testing and load testing.
Example: Implementing Selenium for UI Testing
Here’s a brief Selenium test case that checks if Google can be reached:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class GoogleTest {
private WebDriver driver;
@Before
public void setUp() {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
driver = new ChromeDriver();
}
@Test
public void testGoogleTitle() {
driver.get("https://www.google.com");
assertEquals("Google", driver.getTitle());
}
@After
public void tearDown() {
driver.quit();
}
}
Why this code works:
In this Selenium example, we initialize a ChromeDriver, navigate to Google, and check the title. The @Before
and @After
annotations help establish testing setups and clean-ups. This showcases how automation can extend beyond code to include user interface interactions.
Continuous Integration and Continuous Deployment (CI/CD)
Integrating automation into your CI/CD pipeline helps maintain the application quality more effectively. Tools such as Jenkins, GitLab CI, and Travis CI can be paired with your tests to ensure that every change is verified as it is introduced into the codebase.
Best Practices for Java Testing Automation
- Use Descriptive Test Names - Names should communicate intent and scope clearly.
- Isolate Tests - Ensure tests do not depend on each other, promoting reliability.
- Keep Tests Short - Focus on a single behavior; short, self-contained tests are easier to debug.
- Review Failures - Analyze failed tests promptly to improve the testing process.
- Maintain Your Test Code - Just like production code, your tests should be clean and well-organized.
In Conclusion, Here is What Matters
Automation has transformed Java testing into a streamlined process capable of handling extensive test cases efficiently. Employing the strategies discussed, including selecting the right framework, embracing BDD, leveraging parallel execution, and integrating automation tools into CI/CD pipelines, can help elevate your testing game.
To explore more about enhancing your automation efficiency, dive deeper into the ideas presented in Mastering Quick Automation: 150 Tests in 30 Minutes! available here.
By focusing on creating a solid testing foundation with automation, you pave the way for seamless development cycles, ensuring that your applications remain robust and reliable. Happy testing!
Checkout our other articles