Resolving Sticky Layout Issues in Java-based Web Apps
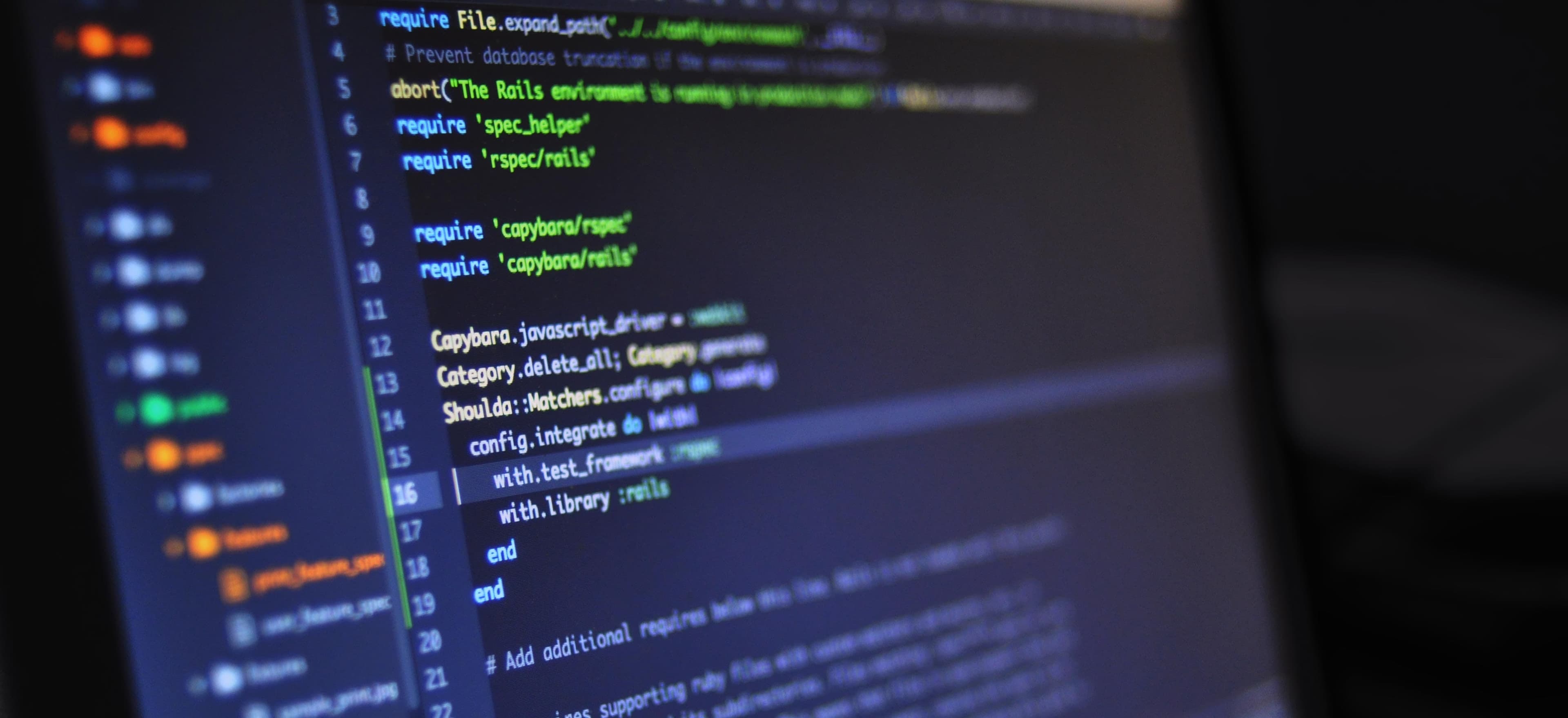
- Published on
Resolving Sticky Layout Issues in Java-based Web Apps
Creating web applications using Java frameworks such as Spring or JavaServer Faces (JSF) can sometimes lead to unique layout challenges, including the infamous "sticky" layout issue. A sticky layout means that certain elements remain fixed at a certain position even while scrolling, leading to potential confusion for users if not implemented correctly.
This blog post will delve into sticky layouts, explore common pitfalls, and discuss how to effectively troubleshoot these issues in Java-based web applications. Moreover, we will examine how CSS plays a vital role in resolving these layout concerns and link to external resources for further reading.
Understanding Sticky Layouts
A sticky layout occurs when an element "sticks" to the viewport as a user scrolls through a webpage. This feature is especially useful for navigation bars or headers. However, if not implemented correctly in a Java-based application, you could face various layout challenges. Let's examine some core principles before jumping into the coding aspects.
CSS and Positioning Properties
The primary CSS properties involved in creating a sticky layout are:
position: sticky;
top, bottom, left, right
offsets
These properties determine how an element behaves as the user scrolls. However, issues may arise related to parent containers, z-index, and overflow settings.
Java-based Web Applications
In Java-based web applications, frameworks such as Spring MVC or JSF handle view rendering through server-side Java code. Thus, understanding the interplay between Java and CSS is crucial.
Sticky Layout Pitfalls
Before diving into code, let’s summarize some common pitfalls when implementing a sticky layout:
-
Parent Overflow Issues: If a parent container has an overflow property set to anything other than visible, the sticky element can behave unexpectedly.
-
Incorrect z-index Settings: A sticky element may become hidden behind other elements if z-index values are not appropriately assigned.
-
Misunderstanding Sticky Context: The sticky position will only activate when the element reaches its designated offset within its nearest scrolling ancestor.
-
Browser Compatibility: Ensure compatibility across different browsers, as some CSS properties may not behave identically.
For a detailed overview of sticky CSS pitfalls, consider checking out the article titled Troubleshooting Sticky CSS: Common Pitfalls Explained.
Implementing a Sticky Layout in Java
Let’s explore how to implement a sticky layout using Java frameworks and CSS effectively. Here is a snippet illustrating the concept of a sticky header:
HTML Structure
<div class="container">
<header class="sticky-header">
<h1>My Sticky Header</h1>
</header>
<main>
<p>Your main content goes here...</p>
<p>More content...</p>
<p>Keep scrolling!</p>
</main>
</div>
CSS Implementation
.container {
width: 100%;
max-width: 1200px;
margin: 0 auto;
}
.sticky-header {
position: sticky; /* Activates sticky positioning */
top: 0; /* Header starts sticking when it reaches top */
z-index: 1000; /* Higher z-index to ensure visibility */
background: #fff; /* Background to avoid transparency issues */
padding: 10px;
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.1); /* Adds some depth */
}
main {
height: 2000px; /* For demonstration purposes */
padding: 20px;
background-color: #f4f4f4;
}
Explanation of Code
-
position: sticky;: This CSS property enables the "sticky" behavior. The header will stick to the top of the viewport once you scroll past it.
-
top: 0;: This tells the browser that the top of the sticky header should remain at the top of the viewport.
-
z-index: 1000;: Ensures the sticky header overlays other content, preventing overlap issues.
-
background: #fff;: Defines a solid background to ensure readability against various other content.
Integrating with Java Frameworks
In a Spring MVC application, you would typically return a view that contains this HTML structure from your controller. For example:
@Controller
public class MyController {
@GetMapping("/home")
public String displayHomePage(Model model) {
// Additional logic can be placed here
return "home"; // Returns the 'home' view
}
}
Here, within the resources/templates/home.html
, one would embed our sticky header structure and relevant CSS.
Debugging Sticky Issues
After implementing the code, you may still run into some sticky layout issues. Here are some debugging tips:
-
Use Developer Tools: Inspect the element using browser developer tools (F12) to see if the
position: sticky;
is being applied as expected. -
Check Parent Styles: Ensure no parent containers have overflow properties that would disrupt the sticky behavior.
-
Test in Multiple Browsers: Variety in browser interpretation can lead to differing results, so always test across popular browsers—Chrome, Firefox, and Safari.
Final Considerations
Creating effective sticky layouts in Java-based web applications involves understanding both CSS and the Java framework you are using. Remember, sticking to a clean design not only enhances user experience but also helps prevent user confusion.
As we've discussed, proper setup and debugging can simplify your layout issues. By understanding how these elements interact, you ensure a seamless experience for users, which is the goal of any web application. For more granular details on sticky CSS pitfalls, do visit Troubleshooting Sticky CSS: Common Pitfalls Explained.
By mastering sticky layouts in Java web applications, you can create intuitive interfaces that remain user-friendly and engaging. Keep experimenting, and your applications will flourish!
In this blog post, we've examined sticky layouts from multiple angles, provided code snippets, and considered debugging techniques—all aligned with SEO best practices to ensure your content is discoverable and relevant. Happy coding!
Checkout our other articles