Streamlining Ajax Calls in Java: Managing Multiple Status Updates
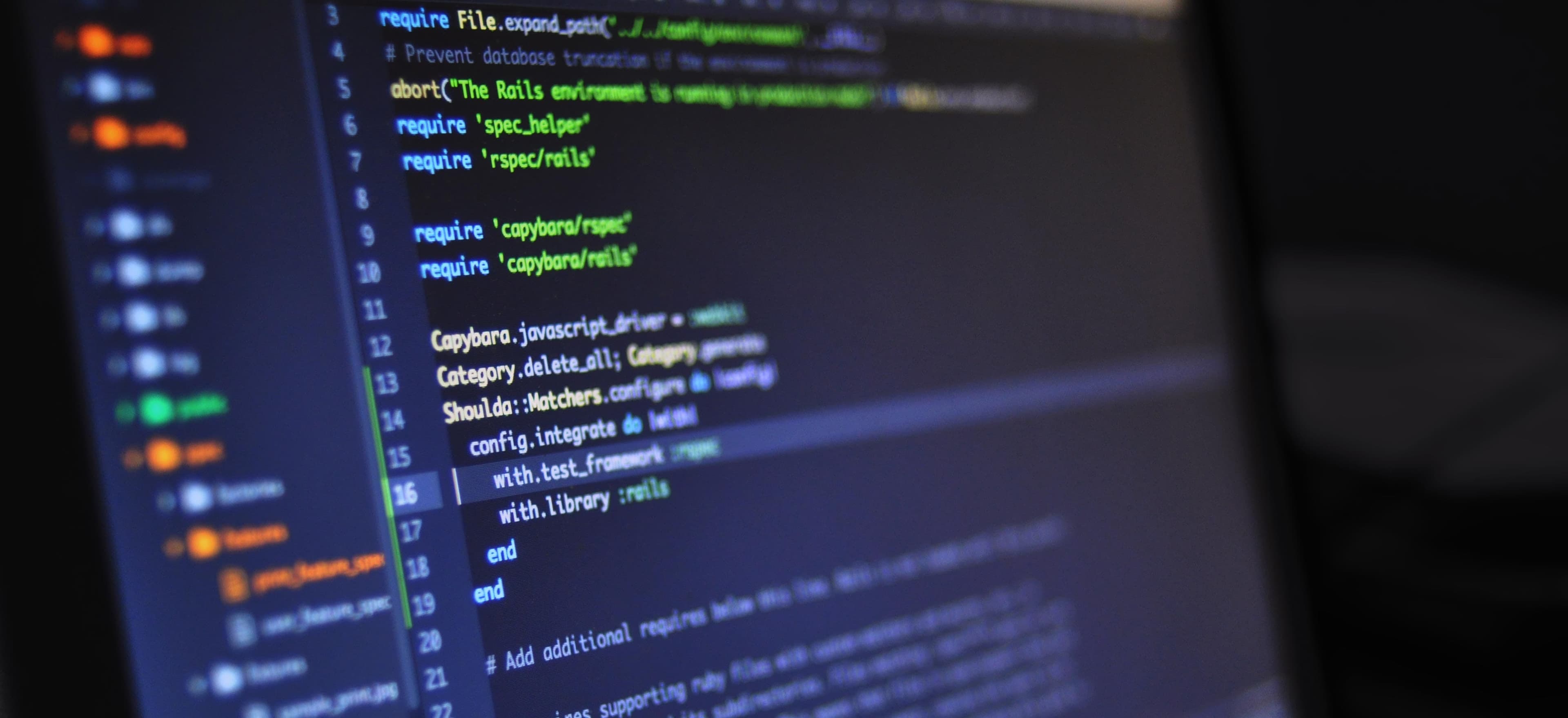
- Published on
Streamlining Ajax Calls in Java: Managing Multiple Status Updates
In modern web applications, managing server interactions through Ajax calls is increasingly important. These calls allow dynamic updates to a webpage without requiring a full page refresh, enhancing user experience significantly. However, handling multiple Ajax status updates can sometimes lead to complications. In this blog post, we will discuss best practices for streamlining Ajax calls in Java, ensuring efficient handling of multiple status updates while maintaining a responsive user interface.
Understanding Ajax
Ajax (Asynchronous JavaScript and XML) is not a programming language but a technique used in web development. It allows web applications to send and retrieve data asynchronously without interfering with the display and behavior of the existing page. This approach leads to a better user experience, as it minimizes waiting times and makes applications more dynamic.
Common uses of Ajax include fetching data from an API, submitting forms without reloading the page, or reorganizing content based on user interactions.
The Challenges of Managing Multiple Ajax Calls
When an application makes multiple Ajax calls, it can encounter various issues:
- Overlapping Requests: Multiple Ajax requests sent simultaneously may cause collisions or inconsistent data responses.
- Race Conditions: The order of completing Ajax calls can lead to unexpected application behavior.
- UI Updates: Managing the display of updates from multiple Ajax responses can overwhelm the UI if not handled carefully.
To mitigate these challenges, it’s crucial to adopt a robust approach that incorporates best practices in your Java backend.
Best Practices for Handling Ajax Calls
1. Use Promises and Async/Await
Using constructs like Promises and async/await
creates a clear, manageable flow of asynchronous code. This approach prevents the issues related to callback hell and makes the code more readable.
Example of Using Promises in Java
In Java, we can simulate promise-like behavior using CompletableFuture. Here’s an example:
import java.util.concurrent.CompletableFuture;
public class AjaxHandler {
public CompletableFuture<String> fetchData(String url) {
return CompletableFuture.supplyAsync(() -> {
// Simulating an Ajax request with a delay
try {
Thread.sleep(2000); // Simulates network latency
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
// Returning mock data
return "Data from " + url;
});
}
}
Why use CompletableFuture? This approach allows non-blocking execution, meaning your application can handle other tasks while waiting for data to return. This optimizes performance and responsiveness.
2. Batch Your Requests
When multiple requests are needed simultaneously, batching them can reduce overhead. Instead of sending several individual requests, combine them into a single request.
Example of Batch Requests
Consider a situation where you need to fetch user data and their posts. Instead of two separate calls, combine them.
public class BatchRequestHandler {
public CompletableFuture<List<String>> fetchBatchData(List<String> urls) {
return CompletableFuture.supplyAsync(() -> {
return urls.stream()
.map(url -> fetchData(url)) // Applying the fetchData method
.collect(Collectors.toList());
});
}
}
Why batch requests? Batching minimizes the number of HTTP requests, reducing latency and improving the overall user experience.
3. Implement Cancellation Logic
Sometimes, users may trigger multiple requests when they are not needed anymore. Implementing cancellation logic helps prevent unnecessary processing.
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.atomic.AtomicReference;
public class CancelableRequest {
private final AtomicReference<CompletableFuture<String>> currentRequest = new AtomicReference<>();
public void fetchData(String url) {
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
// Simulated delay for fetching data
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return "Data from " + url;
});
CompletableFuture<String> previousRequest = currentRequest.getAndSet(future);
if (previousRequest != null) {
previousRequest.cancel(false); // Cancel the previous request if it's still running
}
future.thenAccept(data -> {
System.out.println(data);
currentRequest.set(null); // Clear the reference
});
}
}
Why implement cancellation logic? This feature conserves resources and improves application performance by avoiding unnecessary processing of requests that the user may no longer require.
4. Efficiently Handle Server Responses
When receiving multiple updates, it’s important to manage the responses intelligently to prevent overwhelming the client. Grouping or prioritizing responses will help in creating a smoother user experience.
5. Use WebSockets for Real-time Updates
For applications that require real-time updates, consider using WebSockets instead of Ajax. WebSockets establish a long-lived connection allowing bidirectional communication without multiple requests.
To Wrap Things Up
Managing multiple Ajax status updates in your Java applications does not have to be complicated. By implementing the best practices outlined above, including using promises, batching requests, implementing cancellation logic, and leveraging real-time technologies like WebSockets, developers can ensure smooth and efficient handling of Ajax calls.
For additional insights, refer to the article titled Handling Multiple Ajax Status Updates on Ready State.
This guidance will help you create a more responsive application while leveraging the full power of Ajax in your Java environment. Streamlining Ajax calls is not merely about faster execution; it's about enhancing the user experience through smarter, cleaner, and well-managed interactions.
Checkout our other articles