Managing Backpressure When Integrating Java with Node.js Streams
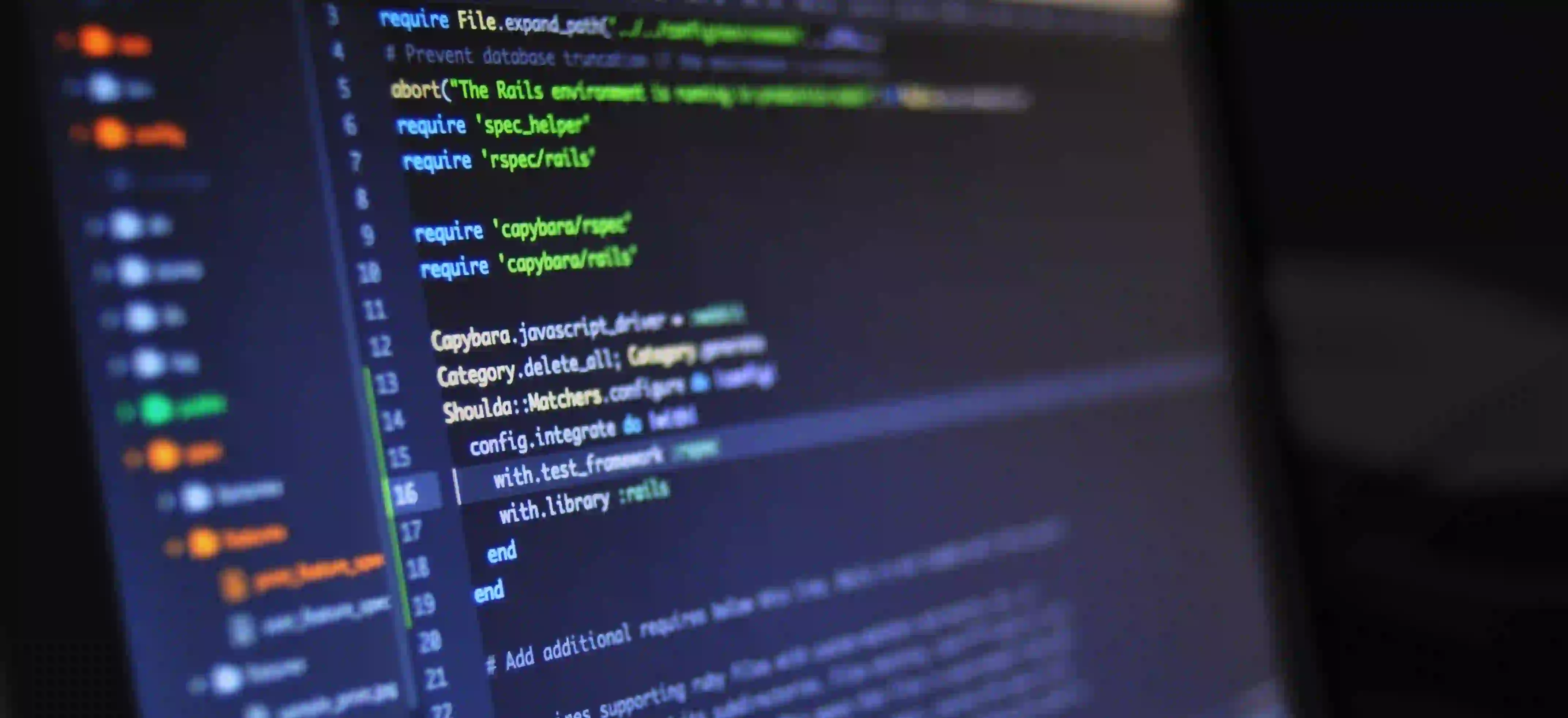
Managing Backpressure When Integrating Java with Node.js Streams
In today's fast-paced application development environment, seamless integration of different technology stacks is crucial. Many applications leverage both Java and Node.js, utilizing their individual strengths to offer robust and scalable solutions. However, when it comes to managing data flow between these two languages—especially with Node.js streams—developers often encounter a challenge known as backpressure.
For a comprehensive understanding of this concept, you might want to check out “Conquering Backpressure in Node.js Streams: A Guide” at infinitejs.com. This article delves into how Node.js handles backpressure within its ecosystem. In this blog post, we will explore how to effectively manage backpressure when integrating Java with Node.js streams.
Understanding Backpressure
Backpressure occurs when a system is overwhelmed by more data than it can handle at any given time. In Node.js, streams provide a way to read and write data in chunks, and backpressure occurs when a writable stream cannot accept more data.
Java and Node.js: A Brief Overview
- Java: Known for its performance and scalability, Java is commonly used in enterprise applications, providing solid multithreading capabilities.
- Node.js: With its non-blocking, asynchronous architecture, Node.js is favored for handling I/O operations and real-time applications such as chat servers and streaming services.
When these two technologies come together, particularly with data streams, challenges related to backpressure must be addressed.
Why Backpressure Matters
Ignoring backpressure can lead to memory leaks, application crashes, and erratic data processing. It's essential to implement mechanisms that gracefully handle scenarios where the data producer outpaces the data consumer.
Techniques for Managing Backpressure
1. Buffering Data in Java
One fundamental strategy for combating backpressure is to use a buffering mechanism on the Java side. Below is a simple example of how to implement a buffering strategy with a BlockingQueue
.
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.BlockingQueue;
public class DataBuffer {
private BlockingQueue<String> queue = new ArrayBlockingQueue<>(100);
public void produce(String data) throws InterruptedException {
// Waits if the queue is full (backpressure management)
queue.put(data);
System.out.println("Produced: " + data);
}
public String consume() throws InterruptedException {
// Waits if the queue is empty
String data = queue.take();
System.out.println("Consumed: " + data);
return data;
}
}
Code Explanation
Using BlockingQueue
allows for simple backpressure handling. If the producer (your method that adds data) tries to add data to a full queue, it will wait (block) until space becomes available. Conversely, the consumer will also block if it tries to remove an item from an empty queue.
2. Flow Control Mechanism
If you're dealing with a high-throughput data scenario, consider implementing a flow control mechanism. Here's a simple example of how that might work.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class FlowControl {
private static final int MAX_CONCURRENT_THREADS = 5;
private ExecutorService executor = Executors.newFixedThreadPool(MAX_CONCURRENT_THREADS);
public void processData(String data) {
executor.submit(() -> {
// Simulate data processing
System.out.println("Processing: " + data);
// Simulate some I/O delay.
try {
Thread.sleep(500);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
System.err.println("Task interrupted.");
}
});
}
public void shutdown() {
executor.shutdown();
}
}
Code Explanation
This flow control mechanism pools a limited number of threads. By limiting concurrent processing, it inherently manages backpressure by controlling the rate at which tasks are executed. You can adjust the MAX_CONCURRENT_THREADS
constant according to your specific use case.
3. Integrating with Node.js Streams
When integrating Java with Node.js streams, it's essential to adopt event-driven paradigms. An example implementation could involve using an HTTP client in Java to pipe the data from a Node.js server.
Here’s a skeleton of how you might handle this:
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class HttpClient {
public void fetchData() {
try {
URL url = new URL("http://localhost:3000/data");
HttpURLConnection con = (HttpURLConnection) url.openConnection();
con.setRequestMethod("GET");
try (BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()))) {
String inputLine;
while ((inputLine = in.readLine()) != null) {
// Here, we would handle the incoming data
processData(inputLine);
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
private void processData(String data) {
// Implement backpressure handling logic
System.out.println("Received: " + data);
}
}
Code Explanation
This Java code uses HTTP to request data from the Node.js server. As data is received, the processData
method is invoked. This example is simplistic and does not manage backpressure yet, but forms a foundation to build upon.
In practice, you would want to include a mechanism to rate-limit or buffer incoming data from the response, similar to the previous techniques discussed.
The Last Word
Navigating the complexities of backpressure when integrating Java and Node.js can be challenging, but with thoughtful implementation of buffering, flow control, and event-driven processing, you can create a robust solution that maintains data integrity and application performance.
As you advance in your project, consider exploring additional resources like the article "Conquering Backpressure in Node.js Streams: A Guide" found at infinitejs.com for strategies that pertain specifically to Node.js.
By implementing best practices for backpressure management, you can ensure smooth data flow between Java applications and Node.js streams. Happy coding!