Unraveling Hoisting Confusion in Java with Practical Tips
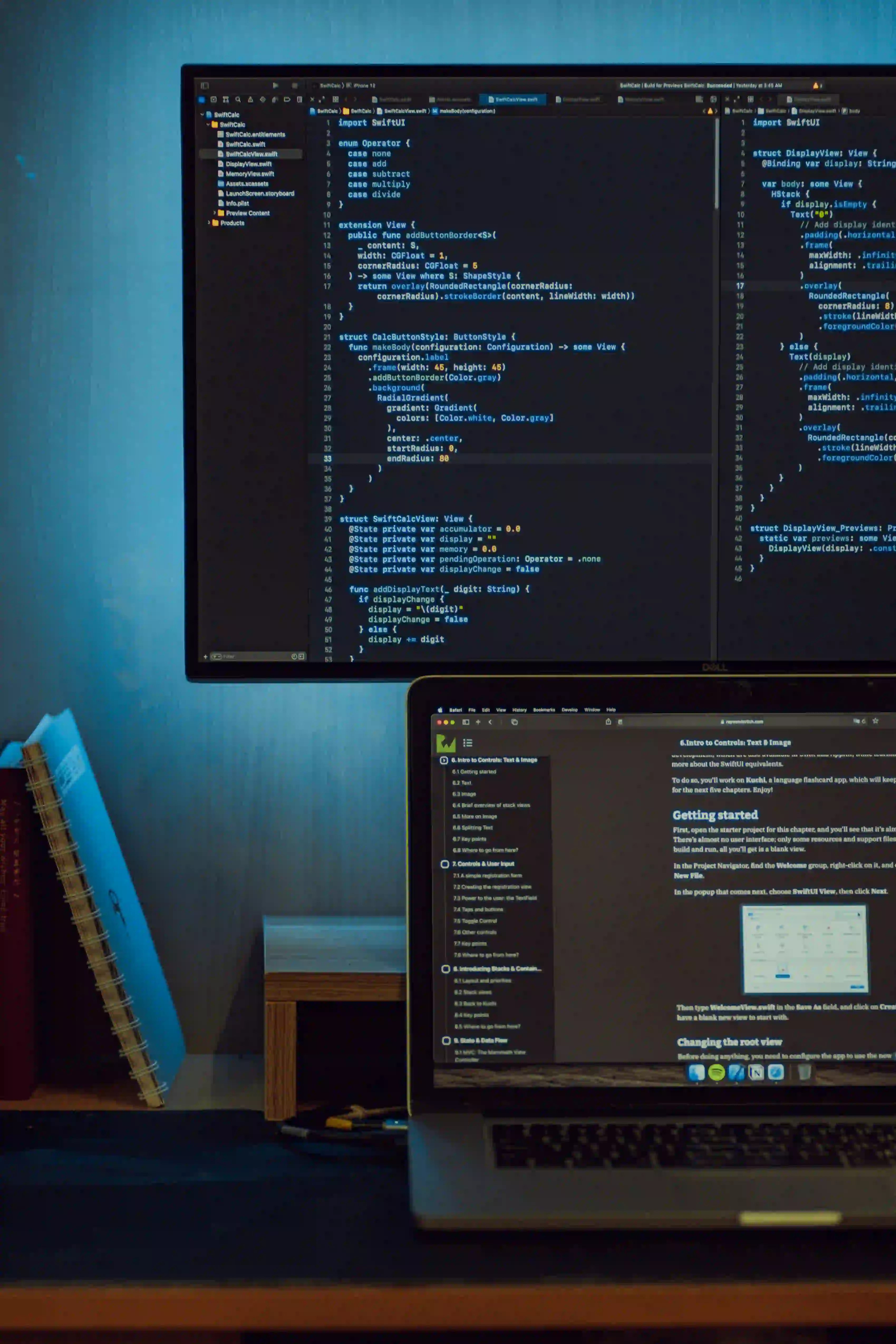
Unraveling Hoisting Confusion in Java with Practical Tips
Java, a pivotal player in the realm of programming languages, has its own intricacies that can spell confusion for both novice and seasoned developers. One such topic is the concept of hoisting. While hoisting is predominantly associated with JavaScript, the principles can also be relevant when working with Java. Join us as we explore hoisting, its implications in Java, and share practical tips to navigate through this seemingly perplexing concept.
Understanding Hoisting
In simple terms, hoisting refers to the JavaScript behavior where variable and function declarations are moved to the top of their containing scope during the compile phase. However, in Java, this behavior is significantly different.
Here’s a quick breakdown:
-
Variable Declarations: Unlike JavaScript, where uninitialized variables can lead to undefined results due to hoisting, Java enforces a stricter rule. Variables must be declared and initialized before they're used.
-
Function Declarations: In Java, functions (or methods) must also be defined before being called. While it might seem simple, understanding the order of operations and where hoisting could play a role is crucial.
Why Is Understanding Hoisting Important?
Grasping the concept of hoisting can help prevent errors in your code and ensure that you write more predictable Java applications. For a deep dive into JavaScript's hoisting and its potential pitfalls, check out this insightful article: Why JavaScript Hoisting Can Break Your Code: A Deep Dive.
Hoisting in Java: Key Points to Remember
-
Local Variables: Local variables in a method do not undergo hoisting. Attempting to access them before declaration will lead to a compilation error.
☕snippet.javapublic class HoistingExample { public static void main(String[] args) { System.out.println(value); // This will cause an error int value = 10; } }
In this code snippet, the line
System.out.println(value);
will generate a compilation error stating that it cannot find the variablevalue
because it hasn't been declared yet. -
Static and Instance Variables: On the other hand, static and instance variables, which are class-level variables, are initialized to their default values and hoisted in a sense, making them accessible throughout the class.
☕snippet.javapublic class HoistingDemo { static int staticValue; // Initialized to 0 int instanceValue; // Initialized to 0 public static void main(String[] args) { System.out.println(staticValue); // Prints 0 HoistingDemo demo = new HoistingDemo(); System.out.println(demo.instanceValue); // Also prints 0 } }
Practical Tips to Avoid Hoisting Confusion
Navigating around the confusion of hoisting in Java can seem like a daunting task, but here are some practical tips to ease the process:
1. Declare Before You Use
This is the golden rule in Java. Whether it be variables or methods, always declare them before you attempt to use them.
public class DeclarationExample {
public static void main(String[] args) {
greetUser(); // Valid as `greetUser` is defined before this line
}
static void greetUser() {
System.out.println("Hello User!");
}
}
2. Use a Consistent Naming Convention
Keeping a clear distinction in your naming conventions can assist in eliminating hoisting errors. For instance, using camel case for method names can help differentiate them easily from variables.
3. Utilize Annotations
Annotations like @Override
can help your IDE and compiler catch certain things early on. Leveraging this can make it easier to understand what method in a superclass you are trying to override, thus reducing confusion regarding calls to the parent class methods.
4. Maintain a Clean Code Style
By organizing your methods and variables logically, you promote readability. It is generally a good practice to top-load your methods in the class to avoid any misinterpretation of order.
public class CleanStyleExample {
static void showMessage() {
System.out.println("This is a clear style!");
}
public static void main(String[] args) {
showMessage(); // Easy to follow.
}
}
5. Regular Code Reviews
Regularly reviewing code can help catch potential hoisting-related issues, as well as improve coding standards across your team. Working collaboratively ensures that everyone adheres to best practices.
Final Thoughts
While Java may not encounter hoisting in the same way that JavaScript does, understanding how variable scope and declaration work is critical in preventing errors. By adhering to best practices, declaring variables and methods before use, employing a consistent naming convention, maintaining a clean code style, and conducting regular code reviews, you will mitigate the confusion surrounding hoisting.
As a developer, the better you understand code behavior, the more robust your applications will become. Java offers a plethora of features, but a solid grasp of its foundational concepts is what truly empowers you as a programmer.
For more information on related topics, explore the article linked earlier, Why JavaScript Hoisting Can Break Your Code: A Deep Dive. Understanding these principles not only enhances your skills but also ensures that you write more effective and maintainable code in your future projects.