Understanding JavaScript Hoisting Impact on Java Integrations
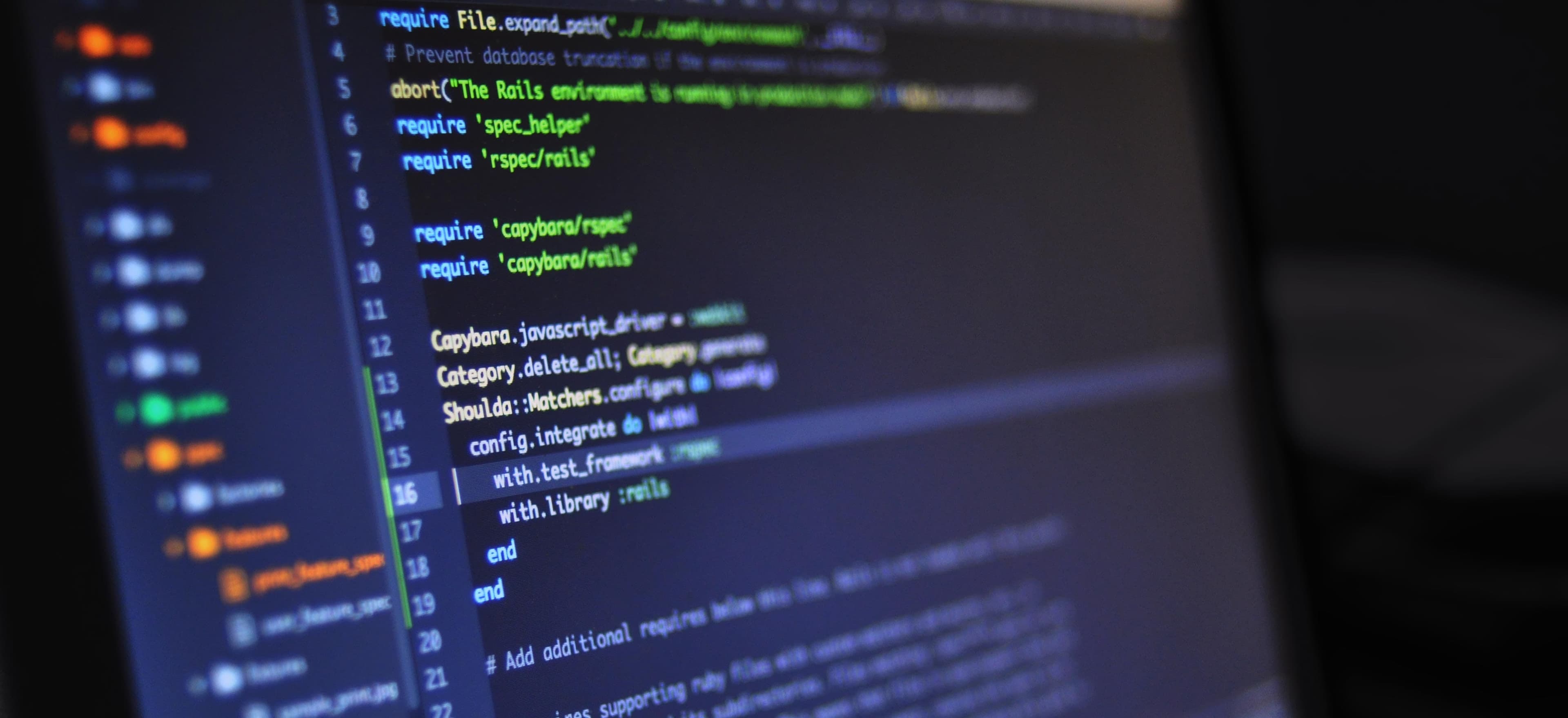
- Published on
Understanding JavaScript Hoisting Impact on Java Integrations
In the world of web development, Java and JavaScript often play complementary roles. Java powers the backend, offering robust capabilities for server-side logic, while JavaScript dominates the frontend, providing dynamic interactions on websites. However, when these two languages interact, especially in situations where JavaScript is embedded in Java applications, understanding JavaScript concepts like hoisting becomes crucial.
In this blog post, we'll delve into the concept of hoisting in JavaScript, referencing the informative article, Why JavaScript Hoisting Can Break Your Code: A Deep Dive. We will explore how JavaScript hoisting affects Java integrations and what developers need to be cautious about.
What is Hoisting?
The Basics of Hoisting
Hoisting in JavaScript is a mechanism where variables and function declarations are moved (or "hoisted") to the top of their containing scope during the compilation phase. While you may assume that the following code would log undefined
due to the declaration being below the console log statement, it actually logs undefined
because of hoisting.
console.log(myVar); // This logs undefined
var myVar = 5;
console.log(myVar); // This logs 5
Here’s what happens under the hood:
- JavaScript creates a variable
myVar
in memory and sets its initial value toundefined
. - The code then assigns
5
tomyVar
.
Hoisting of Functions
Function declarations are also hoisted. The following example illustrates that we can call a function even before it is declared.
sayHello(); // This works
function sayHello() {
console.log("Hello, World!");
}
In this case, the function sayHello
is hoisted, allowing it to be called before its declaration.
Hoisting's Impact on Java Integrations
Now that we understand hoisting, let’s explore its implications in Java environments, specifically when integrating JavaScript within Java applications. Frameworks such as Node.js may run JavaScript for server-side operations, or we may embed JavaScript within Java web applications using technologies such as JSP or Servlets.
Unpredictable Behavior
When JavaScript hoisting occurs within a script that is integrated into a Java application, it can lead to unpredictable behavior. For instance:
function calculateTotal() {
console.log(total); // logs undefined
var total = 100;
return total;
}
calculateTotal(); // This returns 100
Even though total
is defined later in the function, it’s hoisted, and calling it before assigning it a value will yield undefined
.
Error Potentials
When Java interacts with JavaScript, developers may unintentionally create scenarios where the timing of variable initialization leads to bugs. If a JavaScript module is executed from a Java backend and relies on hoisted variables, it may cause issues that are tough to debug.
For instance, consider a JavaScript file that is called in a Java backend application:
function getUser() {
console.log(user); // This logs undefined
var user = { name: "Alice" };
return user;
}
If this file is invoked under conditions in a Java application, the logged output can be misleading, potentially affecting how the Java application processes data.
Null Safety
In Java, null safety is a significant feature that prevents null pointer exceptions. However, in mixed environments utilizing JavaScript, one may encounter variables that appear initialized but are, in fact, still undefined due to hoisting.
Best Practices for Avoiding Hoisting Issues
Integrating JavaScript code into Java applications can introduce complexities. Here are some best practices to avoid hoisting issues:
- Use
let
andconst
: Unlikevar
, which is hoisted,let
andconst
are block-scoped and do not allow access to variables before they're declared.
console.log(myNewVar); // ReferenceError: Cannot access 'myNewVar' before initialization
let myNewVar = 5;
-
Design with Hoisting in Mind: Always declare your variables at the top of their scope. This will help in avoiding the confusion that hoisting introduces.
-
Avoid Function Declarations in the Middle of Blocks: Prefer function expressions (including arrow functions) instead of declarations inside blocks.
// Avoid this
if (true) {
function innerFunction() {
console.log("Hello");
}
}
// Prefer this
const innerFunction = () => {
console.log("Hello");
};
-
Use Linting Tools: Tools like ESLint can provide warnings about possible hoisting-related issues in your code, allowing you to address potential problems before they become errors.
-
Unit Testing: Implement unit tests that check for unintended side effects in your JavaScript code. This will help you catch hoisting issues early in the development process.
Lessons Learned
As we have explored, JavaScript hoisting can have significant effects when integrated with Java applications. Understanding how hoisting works helps developers write cleaner, less error-prone code, especially in scenarios where JavaScript code interacts with Java components. By adhering to best practices and remaining cautious of the nuances of variable declarations and scope, one can avoid many of the pitfalls associated with hoisting.
For a deeper dive into hoisting-specific issues in JavaScript, check out the detailed article Why JavaScript Hoisting Can Break Your Code: A Deep Dive.
In the rapidly evolving landscape of technology, being aware of these interactions will make you a more effective developer, ready to harness the strengths of both Java and JavaScript. Happy coding!