Protecting Your Java App from Injection Attacks
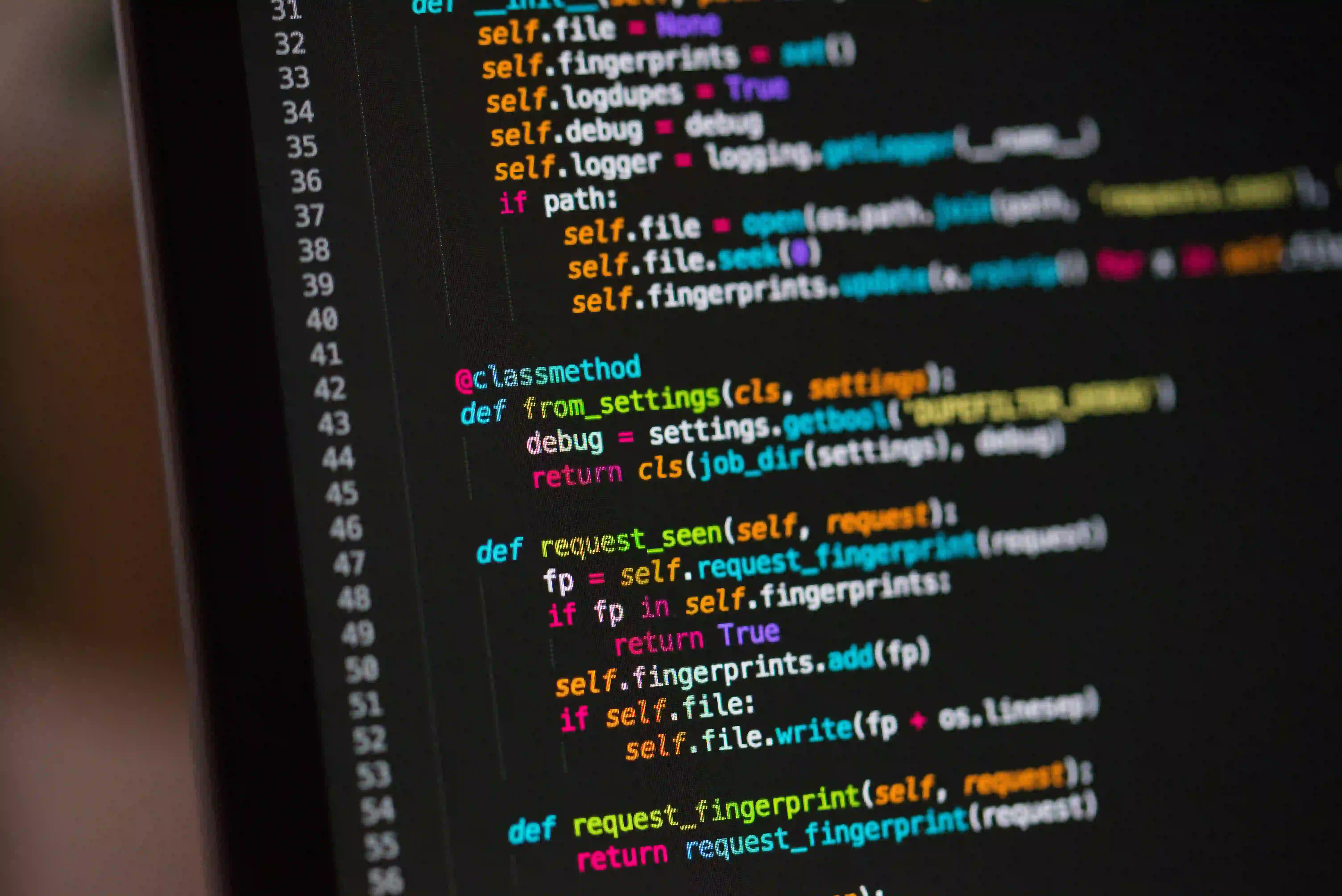
Protecting Your Java App from Injection Attacks
Injection attacks remain one of the most prevalent cybersecurity threats facing web applications today. Among the types of injection attacks, SQL injection is perhaps the most notorious, but there are also command injection, XML injection, and more. Given Java's widespread use in enterprise applications, protecting Java apps from these vulnerabilities is crucial. In this blog post, we will explore various forms of injection attacks, how they operate, and, most importantly, how to secure your Java applications against them.
Understanding Injection Attacks
What is an Injection Attack?
An injection attack occurs when an attacker is able to send untrusted data to an interpreter, causing unintended commands or queries to be executed. This often leads to unauthorized access or data manipulation.
For example, in SQL injection, malicious SQL statements are inserted into a query, potentially revealing sensitive data or even allowing the attacker to modify the database.
Common Types of Injection Attacks
-
SQL Injection: Targeting databases, attackers can manipulate SQL queries by injecting malicious SQL code.
-
Command Injection: The attacker executes arbitrary commands on the server through an application.
-
XML Injection: This involves injecting malicious XML into a web service or an application that consumes XML data.
-
LDAP Injection: Attackers exploit vulnerabilities in LDAP queries, allowing unauthorized data access.
To effectively defend your Java applications, it's essential to understand these attack vectors and how they manifest.
Key Techniques to Prevent Injection Attacks
1. Use Prepared Statements
One of the most effective defenses against SQL injection in Java applications is the use of prepared statements. Prepared statements separate SQL logic and data, preventing manipulation.
Example Code
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
public class UserDAO {
public User getUserByUsername(String username) {
User user = null;
String sql = "SELECT * FROM users WHERE username = ?";
try (Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "user", "pass");
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setString(1, username); // Bind the username parameter
try (ResultSet rs = pstmt.executeQuery()) {
if (rs.next()) {
user = new User(rs.getString("username"), rs.getString("password"));
}
}
} catch (Exception e) {
e.printStackTrace();
}
return user;
}
}
Why Use Prepared Statements? In the code example above, the SQL command and the data are defined separately. This means that even if a malicious username is provided, it will not be executed as SQL code but simply treated as a string. As a result, the attack is thwarted. The prepared statement method helps in preventing SQL injections effectively.
2. Input Validation
Input validation is another key strategy for preventing injection attacks. Ensure that all user inputs are strictly validated and sanitized both client-side and server-side.
Example Code
import java.util.regex.Pattern;
public class Validator {
private static final String USERNAME_PATTERN = "^[a-zA-Z0-9]{3,20}$"; // Only allows alphanumeric usernames
public static boolean isValidUsername(String username) {
return Pattern.matches(USERNAME_PATTERN, username);
}
}
Why is Input Validation Important? In the example, the username must match specific alphanumeric criteria. This limitation ensures that only valid data is processed further in the application, reducing the risk of the attacker crafting harmful input.
3. Escaping User Input
For situations where parameterized queries are not an option, escaping user input can serve as an additional layer of protection against injection attacks. However, it should not be the sole line of defense.
4. Use ORM Frameworks
Using Object-Relational Mapping (ORM) frameworks, such as Hibernate, can help mitigate risks by abstracting direct SQL command execution. These frameworks often implement built-in protections against injection attacks.
Example Code
import org.hibernate.Session;
import org.hibernate.Transaction;
public class UserService {
public User getUser(String username) {
Transaction transaction = null;
User user = null;
try (Session session = HibernateUtil.getSessionFactory().openSession()) {
transaction = session.beginTransaction();
String hql = "FROM User u WHERE u.username = :username";
user = session.createQuery(hql, User.class)
.setParameter("username", username)
.uniqueResult();
transaction.commit();
} catch (Exception e) {
if (transaction != null) {
transaction.rollback();
}
e.printStackTrace();
}
return user;
}
}
Why Choose ORM Frameworks? In this Hibernate example, the potential for code injection is minimized by providing query parameters in a secure manner. The ORM handles input binding, which limits the chance of SQL injection.
5. Use the Principle of Least Privilege
Ensure that the database user roles have the minimum necessary privileges for application functions. Limiting access can help minimize damage in the event of an injection attack. Explore more on the Principle of Least Privilege.
The Closing Argument
Injection attacks pose a significant threat to Java applications, but with the right strategies in place, you can effectively defend your systems. Utilizing prepared statements, validating input, escaping user input, and leveraging ORM frameworks are key techniques to create a robust security posture.
Remember, no single solution will provide complete protection. It is essential to employ multi-layered defense strategies and always stay informed about evolving best practices in web security.
For further reading on securing Java applications, check out OWASP Java Code Guidelines and explore effective strategies for preventing various types of attacks.
By adopting these practices, developers can fortify their Java applications against potential breaches, thus safeguarding sensitive information and maintaining the trust of their users.