Mastering Struts2: Top Interview Questions You Must Know
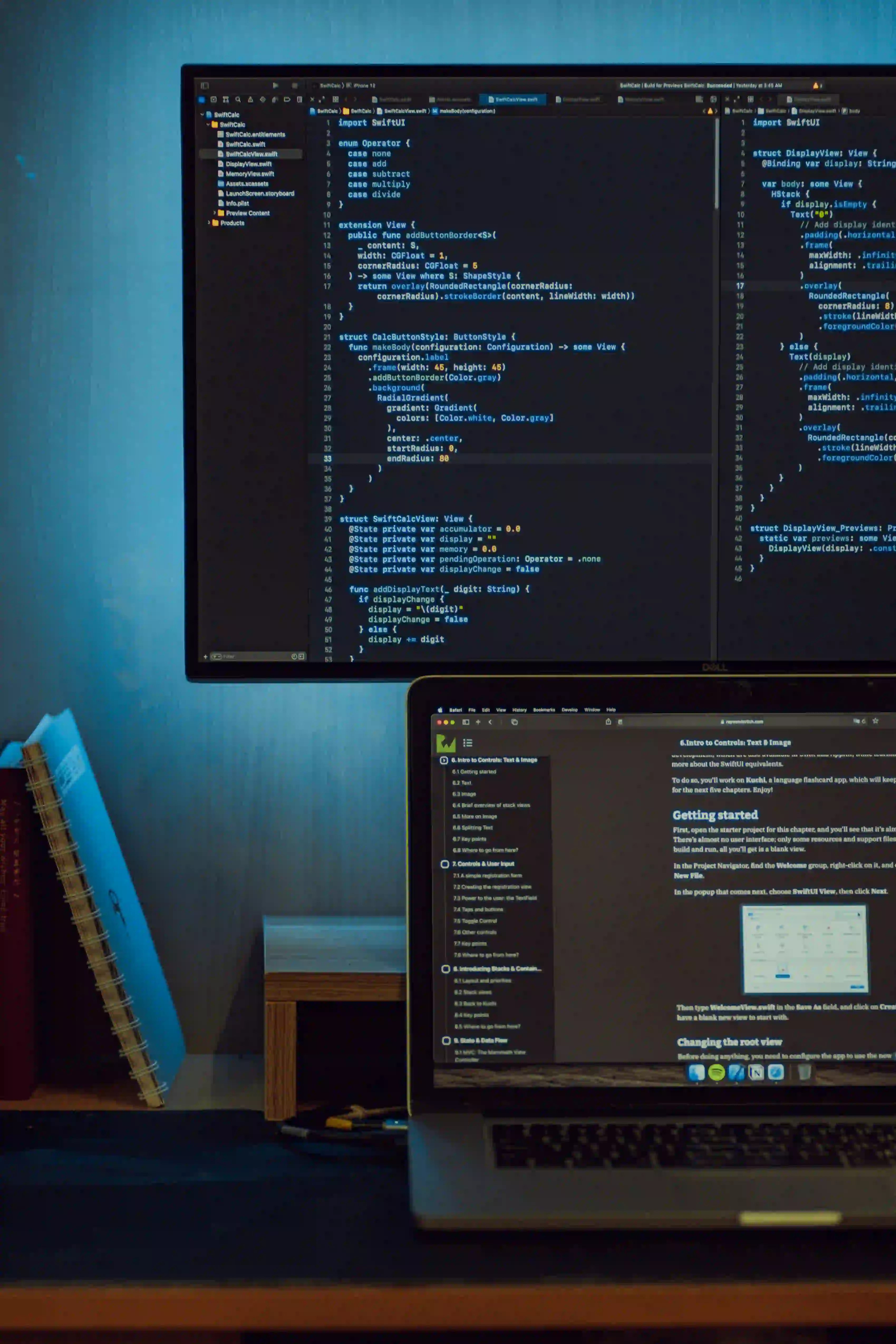
Mastering Struts2: Top Interview Questions You Must Know
Struts2 is a robust framework used primarily for developing Java web applications. Its popularity springs from its ability to provide an elegant way to build maintainable applications while improving productivity. If you're preparing for an interview related to Struts2, it's crucial to understand not only the framework’s technical aspects but also its design principles and best practices. Here, we present some of the top interview questions related to Struts2, along with their answers and context.
1. What is Struts2?
Struts2 is an open-source web application framework based on the MVC (Model-View-Controller) architecture. It uses various technologies such as JSP, JavaBeans, and XML, which allows developers to build applications that are scalable, flexible, and maintainable. Unlike its predecessor Struts1, Struts2 incorporates conventions over configuration, making development more streamlined and efficient.
Key Features:
- MVC architecture: Separates application logic, user interface, and input handling.
- Rich plugin architecture: You can easily extend functionalities.
- Support for multiple view technologies: Works with JSP, FreeMarker, etc.
- Built-in support for AJAX: Enhances user experience with asynchronous calls.
2. Explain the MVC architecture in Struts2.
The Model-View-Controller (MVC) design pattern is central to Struts2. Here's how it breaks down:
-
Model: Represents the data of the application. In Struts2, POJOs (Plain Old Java Objects) often serve as the model.
-
View: Represents the user interface. In Struts2, JSPs, Freemarker templates, or other view technologies act as the view layer.
-
Controller: Handles user requests and determines which model and view to use. In Struts2, the
Action
classes serve as controllers.
By separating these concerns, Struts2 enhances maintainability and scalability.
Code Snippet Example:
public class UserAction extends ActionSupport {
private String name;
public String execute() {
if (name != null) {
return SUCCESS;
}
return ERROR;
}
// Getter and Setter for name
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Why?: In this UserAction
class, the execute method controls the flow of the application based on user input. If the user’s name is provided, the method returns a success message. This demonstrates how actions act as controllers to handle user interaction.
3. What are Action classes in Struts2?
Action classes in Struts2 are fundamental components that handle requests. They encapsulate the business logic of your application. When a request is sent, Struts2 creates an instance of the specified action class and invokes its execute method. Action classes can be simple Java beans or they might contain complex business logic.
Elements of Action classes:
-
Stateful or stateless: Defines whether they maintain state across requests.
-
Implementation of interfaces: Actions can implement
Action
,ActionSupport
, orModelDriven
.
Code Snippet Example for Action Interface:
public class RegistrationAction implements Action {
@Override
public String execute() {
// Perform registration logic
return SUCCESS;
}
}
Why?: Implementing the Action
interface provides access to built-in functionalities such as internationalization and error handling. By returning SUCCESS, we specify the outcome of the action.
4. What is the purpose of the struts.xml file?
The struts.xml
file is the core configuration file in Struts2 applications. It defines the relationship between the actions and the result types. This file uses XML for configuration and supports various settings such as interceptors, action mappings, and result types.
Key Tags in struts.xml:
- action: Maps the URL to the action class.
- result: Specifies what should happen when an action is executed.
- interceptor: Configures interceptors which can pre-process or post-process request handling.
Code Snippet Example:
<struts>
<package name="default" extends="struts-default">
<action name="register" class="com.example.RegistrationAction">
<result name="success">/welcome.jsp</result>
<result name="error">/error.jsp</result>
</action>
</package>
</struts>
Why?: This configuration maps the register
URL to the RegistrationAction
class. Depending on the outcome of the execution, it will navigate dynamically to either "welcome.jsp" for success or "error.jsp" for failure.
5. Explain the role of Interceptors in Struts2.
Interceptors in Struts2 are used to process a request before it reaches the action class and after the action has been executed. They allow for cross-cutting concerns such as logging, authentication, and validation without code duplication.
Important Built-in Interceptors:
- Parameter Validation Interceptor: Validates incoming parameters.
- Logging Interceptor: Logs all the actions that are invoked.
- Execution Time Interceptor: Measures the execution time of actions.
Code Snippet Example:
<interceptor-stack name="myStack">
<interceptor-ref name="logging">
<interceptor-ref name="basicStack"/>
</interceptor-stack>
<action name="register" class="com.example.RegistrationAction">
<interceptor-ref name="myStack"/>
<result name="success">/welcome.jsp</result>
</action>
Why?: Defining a custom interceptor stack allows for reusability. Here, we're logging each action's execution while processing the request, ensuring that we have oversight throughout the application flow.
6. What is the difference between ActionSupport and the Action interface?
The ActionSupport
class is an implementation of the Action
interface, designed to make implementing action classes easier. Here are the differences:
-
Action Interface: Requires you to implement all methods manually. It's lighter but may lead to repetition of code.
-
ActionSupport Class: Provides default implementations for various methods and includes additional functionality, like validation, internationalization, and error-handling capabilities.
Code Snippet Comparison:
Using Action:
public class UserAction implements Action {
public String execute() {
return SUCCESS;
}
}
Using ActionSupport:
public class UserAction extends ActionSupport {
public String execute() {
return SUCCESS; // Availability of predefined constants like SUCCESS, ERROR
}
}
Why?: By extending ActionSupport
, you gain access to a wealth of built-in functionalities that simplify the action class implementation, making it more concise and less error-prone.
7. How do you handle exceptions in Struts2?
Struts2 provides several methods for exception handling. You can use global exception mapping in struts.xml
, specific actions, or interceptor approach.
Example of Global Exception Mapping:
<global-exception-mapping exception="java.lang.Exception" result="error" />
Why?: This mapping allows all unhandled exceptions to navigate to a unified error page, improving user experience and keeping the application flow manageable.
To Wrap Things Up
Understanding Struts2 thoroughly can significantly enhance your ability to handle Java web application development. This guide outlined some of the crucial interview questions you may encounter as you prepare for your next job interview. Each element of Struts2, from MVC architecture to action classes to interceptors, contributes to a smooth and effective application design.
For additional reading, refer to the Struts2 official documentation as well as resources like Java Brains for deeper insights into Java frameworks.
Master these concepts, practice with code, and you’ll stand a much better chance of acing your Struts2-related interviews. Good luck!