TestNG vs JUnit: Choosing the Right Framework for You
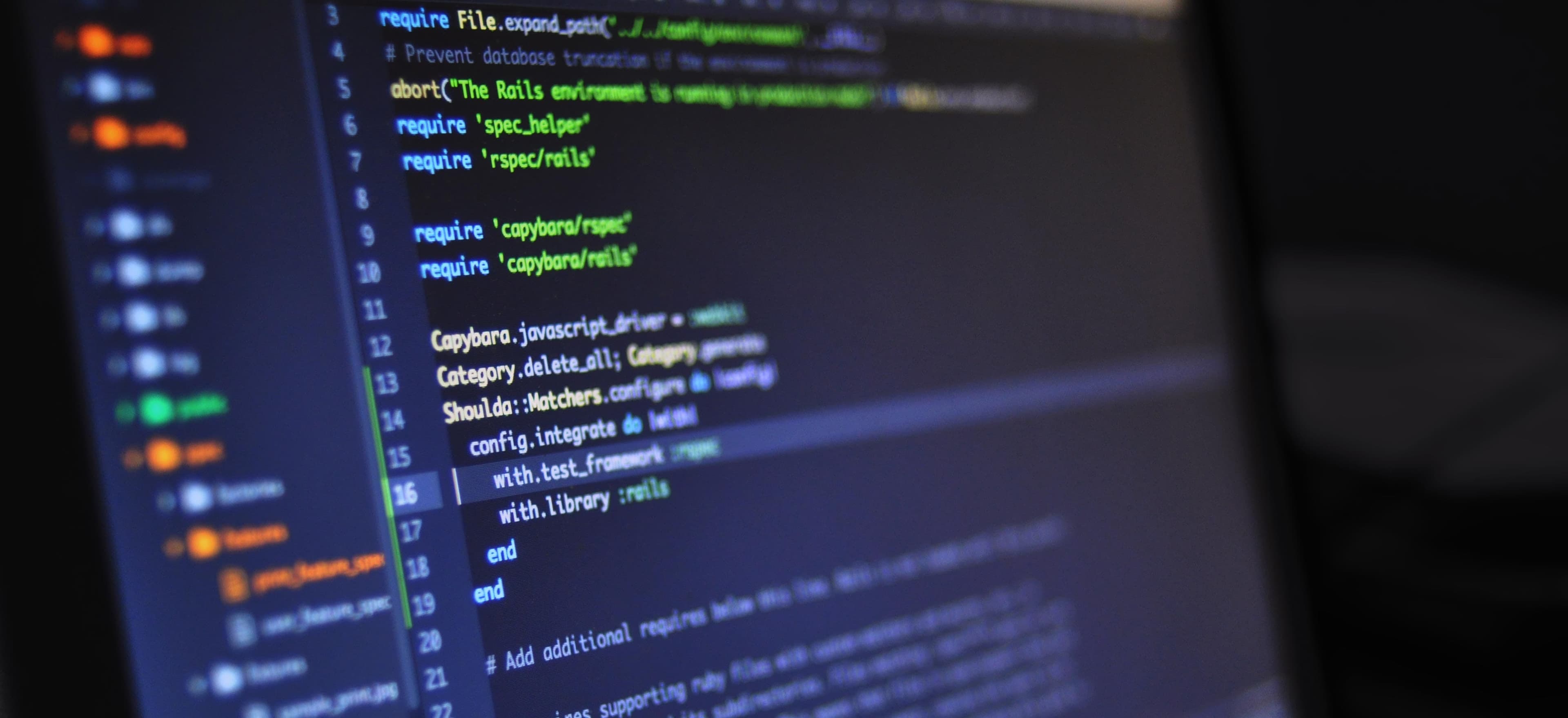
- Published on
TestNG vs JUnit: Choosing the Right Framework for You
When embarking on the journey of unit testing in Java, developers often encounter a crucial decision: which testing framework to choose? Among the plethora of available options, TestNG and JUnit stand out as two of the most popular frameworks. Each has its unique strengths, making the choice dependent on various factors including project requirements, team experience, and integration needs. In this article, we will delve into the distinctions, features, and use cases of both TestNG and JUnit to help you make an informed decision.
Overview of JUnit
JUnit is one of the oldest and most widely used testing frameworks in the Java ecosystem. Its simplicity and robustness have made it a cornerstone of Java unit testing.
Key Features of JUnit
- Annotations: JUnit uses annotations to define test methods. Common annotations include:
@Test
: Marks a method as a test case.@BeforeEach
: Indicates a method that runs before each test execution.@AfterEach
: Used for defining cleanup logic after each test.
- Assertion Methods: JUnit provides various assertion methods, such as:
assertTrue()
assertEquals()
assertThrows()
Example JUnit Test Case
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
class CalculatorTest {
private Calculator calculator;
@BeforeEach
void setUp() {
calculator = new Calculator();
}
@Test
void testAdd() {
// Testing the add method
assertEquals(4, calculator.add(2, 2), "2 + 2 should equal 4");
}
}
Why this structure?
Here, we instantiate the Calculator
class in the @BeforeEach
method, ensuring a fresh instance is available before each test. This helps prevent state leaks between tests, a common pitfall in unit testing.
Overview of TestNG
TestNG is a more advanced testing framework inspired by JUnit but designed to address its limitations. It's particularly noted for its powerful features that cater to a wide range of testing needs, from unit testing to integration and functional testing.
Key Features of TestNG
-
Annotations: Similar to JUnit but offers more flexibility:
@Test
: Used for test methods.@BeforeClass
and@AfterClass
: Methods that run once for the entire class.
-
Dependency Testing: TestNG allows you to define dependencies between tests easily, enabling better management of test execution order.
-
Data-Driven Testing: TestNG supports data providers that help run the same test method multiple times with different data sets.
Example TestNG Test Case
import org.testng.annotations.BeforeClass;
import org.testng.annotations.DataProvider;
import org.testng.annotations.Test;
import static org.testng.Assert.*;
public class CalculatorTest {
private Calculator calculator;
@BeforeClass
public void setUp() {
calculator = new Calculator();
}
@DataProvider(name = "additionData")
public Object[][] additionData() {
return new Object[][] {
{1, 1, 2},
{2, 2, 4},
{3, 3, 6}
};
}
@Test(dataProvider = "additionData")
public void testAdd(int a, int b, int expected) {
assertEquals(calculator.add(a, b), expected, "Sum should be correct");
}
}
Why using a DataProvider?
In the above example, the @DataProvider
annotation allows running the same test with various inputs, improving test coverage and ensuring that the add
method behaves correctly for multiple inputs.
Key Differences Between JUnit and TestNG
While both frameworks aim to simplify the testing process, their differences cater to different scenarios.
-
Test Structure:
- JUnit focuses on simplicity. Its structure is straightforward, making it ideal for beginners.
- TestNG's intricate structure accommodates complex testing requirements through features like test grouping and dependency management.
-
Test Execution:
- JUnit executes tests in a single-threaded environment.
- TestNG supports parallel test execution, allowing for faster test completion, which can be critical in large projects.
-
Configurability:
- JUnit configurations are primarily annotations-based.
- TestNG provides an XML configuration file option for customizing test execution, a significant advantage when dealing with intricate test scenarios.
-
Integration with Build Systems:
- Both frameworks integrate smoothly with Maven and Gradle, but TestNG offers additional flexibility in XML configuration.
When to Choose JUnit
JUnit is highly suitable for projects requiring classic unit tests that are relatively simple. For teams new to testing or those working on smaller codebases, JUnit's ease of use and straightforward approach makes it an excellent choice.
-
Pros:
- Beginner-friendly syntax
- Impressive maturity and community support
- Seamless integration with CI/CD systems
-
Cons:
- Limited support for complex testing needs
- No built-in support for parallel execution (prior to JUnit 5)
When to Choose TestNG
On the other hand, TestNG shines in complex testing scenarios that demand a robust framework. It is particularly beneficial for projects that require a combination of unit testing, integration testing, and functional testing within the same framework.
-
Pros:
- Handles dependency testing and parallel execution seamlessly
- Data-driven testing capabilities
- XML configuration for customized setups
-
Cons:
- Slightly steeper learning curve
- Heavier for smaller projects with simple testing needs
Lessons Learned
Choosing between TestNG and JUnit boils down to understanding your project requirements and team familiarity with testing frameworks. JUnit provides simplicity and ease of use, which is perfect for beginners or smaller applications. TestNG offers rich features that cater to advanced testing needs, making it favorable for larger, more complex projects.
Ultimately, both JUnit and TestNG are powerful tools in a Java developer's arsenal. Knowing their strengths and weaknesses allows developers to harness the power of unit testing effectively, contributing to improved code quality and system reliability.
As you consider your options, take into account the development environment, project scale, and specific testing requirements before making a decision. Happy testing!
Checkout our other articles