Avoiding Common Pitfalls When Shutting Down Java GUI Apps
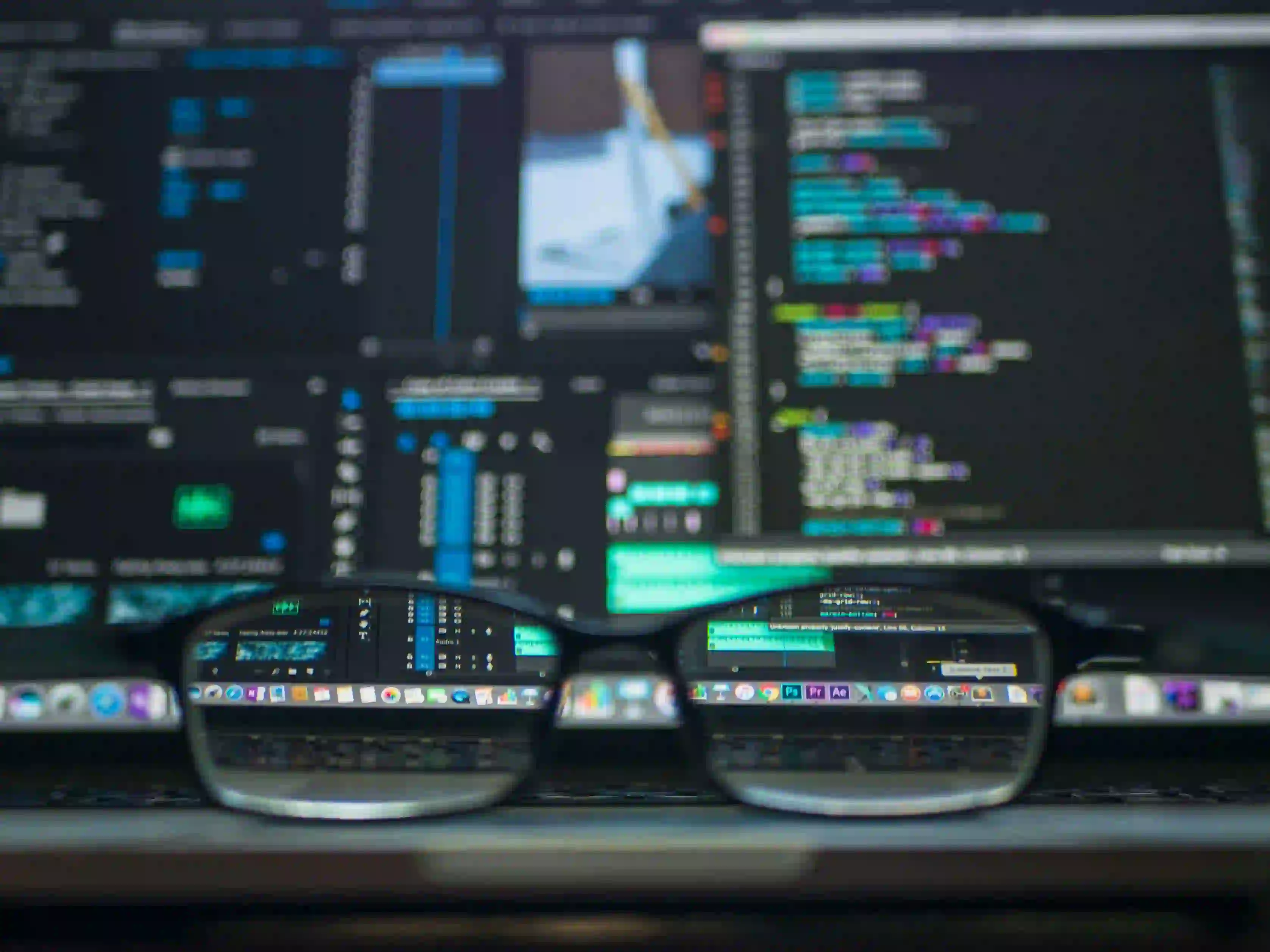
Avoiding Common Pitfalls When Shutting Down Java GUI Apps
Java provides a robust framework for developing graphical user interface (GUI) applications. However, one of the frequently overlooked aspects of this process is correctly shutting down these applications. Improper shutdowns can lead to resource leaks, incomplete operations, and data corruption. In this blog post, we'll discuss common pitfalls associated with shutting down Java GUI apps and how to avoid them.
Understanding GUI Lifecycle
Before diving into the shutdown process, it’s essential to understand the lifecycle of a Java GUI application. Java's Swing framework, which is widely used for developing GUIs, operates on a single thread called the Event Dispatch Thread (EDT). All GUI tasks should be performed on this thread to prevent freezing and ensure a responsive interface.
Key Points to Consider:
- Thread Safety: Always interact with GUI components from the EDT.
- Event Listeners: Ensure that listeners are properly deregistered before shutdown.
- Resource Management: Always release resources for smooth operations.
Common Pitfalls
Here are the most common pitfalls developers face when shutting down Java GUI applications:
1. Ignoring the EDT
When shutting down a Java GUI application, it's crucial to ensure that the EDT processes any pending events before the application terminates. Failing to wait for the EDT can leave the application in an inconsistent state.
Example of Proper Shutdown Using SwingUtilities
:
import javax.swing.SwingUtilities;
public static void shutdownApplication() {
SwingUtilities.invokeLater(() -> {
// Perform cleanup tasks here
System.exit(0); // Exits the application
});
}
Why Use SwingUtilities.invokeLater
?
This method places the provided runnable on the EDT, ensuring that all pending events are processed before a shutdown. This is crucial because it allows the user interface to gracefully respond to the shutdown signal, thus preventing any inconsistencies.
2. Not Handling Window Closing Events
When the user closes a window, the default behavior of Java applications might not involve cleanup processes like saving data or releasing resources.
Example of Adding a WindowListener:
import javax.swing.*;
import java.awt.event.*;
public class MyApp extends JFrame {
public MyApp() {
setTitle("My Application");
setDefaultCloseOperation(JFrame.DO_NOTHING_ON_CLOSE);
addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
confirmExit();
}
});
setSize(400, 300);
setVisible(true);
}
private void confirmExit() {
int confirm = JOptionPane.showConfirmDialog(this,
"Are you sure you want to exit?", "Confirm Exit",
JOptionPane.YES_NO_OPTION);
if (confirm == JOptionPane.YES_OPTION) {
dispose(); // Close the frame
System.exit(0); // Exit the application
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(MyApp::new);
}
}
Why Use windowClosing
?
By utilizing WindowListener
, you can intercept the closing event of your application. This allows you to confirm with the user before proceeding with shutdown, ensuring that any critical tasks like saving unsaved work can be handled smoothly.
3. Resource Leakage
Java applications often utilize resources such as database connections, file handles, and network sockets. Neglecting to close these connections before shutdown can lead to resource leakage issues.
Example of Closing Resources:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseManager {
private Connection connection;
public DatabaseManager() {
try {
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "user", "password");
} catch (SQLException e) {
e.printStackTrace();
}
}
public void closeConnection() {
if (connection != null) {
try {
connection.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
// Call this method during application shutdown
public static void shutdown(DatabaseManager dbManager) {
dbManager.closeConnection();
System.exit(0);
}
}
Why is Closing Resources Essential?
Closing resources ensures that all database connections, file streams, or other resources are released and ready for garbage collection. This is essential in preventing potential memory leaks, which may degrade performance and lead to system crashes.
4. Ignoring Thread Interruption
If your GUI application has background threads (for example, for long-running operations), you must ensure they are gracefully interrupted or stopped before shutting down the application.
Example of Thread Management:
class LongRunningTask extends Thread {
private volatile boolean running = true;
public void run() {
while (running) {
// Perform long-running task
}
}
public void stopTask() {
running = false;
}
public static void shutdown(LongRunningTask task) {
task.stopTask(); // Stop the long-running task
System.exit(0); // Exit the application
}
}
Why Use a Volatile Flag?
The volatile
keyword allows the running
variable to be modified by multiple threads safely, ensuring that changes made in one thread are visible to others. This allows the long-running task to stop cleanly, preventing the application from hanging during shutdown.
Best Practices for Clean Shutdown
To ensure a smooth and efficient shutdown of a Java GUI application, adhere to the following best practices:
- Centralized Shutdown Method: Create a method that handles all cleanup tasks consistently across your application.
- User Confirmation: Always confirm with users before closing the application to avoid accidental data loss.
- Scheduled Tasks: Utilize
ScheduledExecutorService
for periodic tasks, ensuring they can also be shut down gracefully. - Proper Resource Management: Always ensure all resources are properly released before shutdown.
In Conclusion, Here is What Matters
Shutting down Java GUI applications may seem trivial, but it is an area that requires careful attention and consideration. By avoiding the common pitfalls discussed and adhering to best practices, you can ensure a smooth and clean shutdown process, thereby enhancing the user experience and maintaining application integrity.
If you want to dive deeper into Java Swing applications and their lifecycle management, consider reading Java’s Official Documentation on Swing for a more comprehensive understanding.
Additionally, you may want to explore Java Concurrency in Practice for more insights on managing threads effectively in your applications.
Implementing a robust shutdown process is not just about exiting the application; it is about respecting the user's data and ensuring the application's reliability. Take the time needed to structure your shutdown process correctly, and you will save yourself and your users from future headaches.