Mastering Java 8 Streams: Filter with Complex Conditions
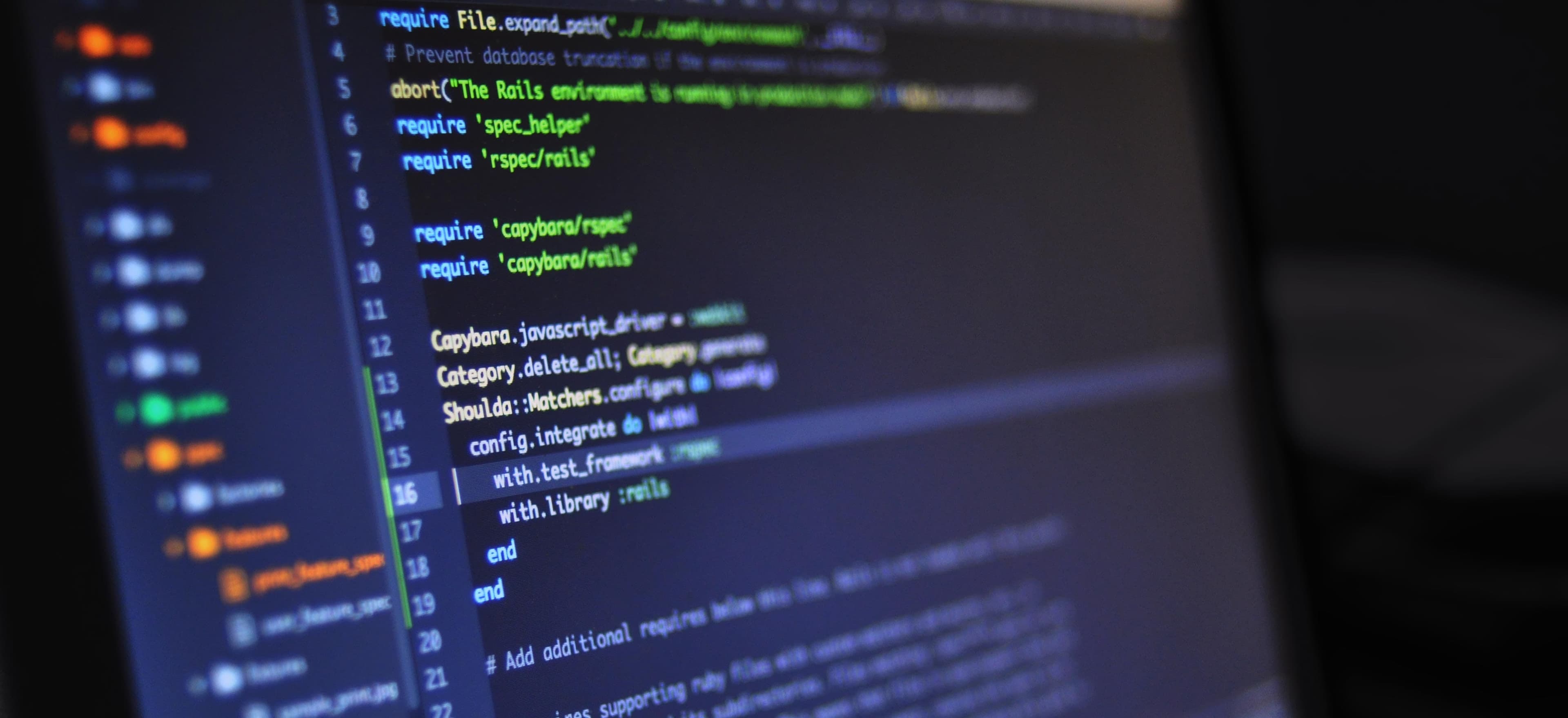
- Published on
Mastering Java 8 Streams: Filter with Complex Conditions
Java 8 introduced a plethora of features that significantly transformed how developers write code. One of the most notable additions is the Streams API, which allows for functional-style operations on collections of data. Among them, the filter
operation is a powerful feature for sifting through data based on specific criteria.
In this blog post, we will explore how to use Java 8 Streams to filter collections with complex conditions. We'll dive into examples, providing code snippets with detailed explanations on why each approach works. By the end of this post, you'll be equipped with the knowledge to use Streams effectively and efficiently in your Java applications.
What is the Streams API?
The Streams API is a part of the Java Collections Framework and is included in Java 8 and later versions. It allows developers to process sequences of elements using functional-style operations, enabling a more declarative approach to data processing. Here’s a brief overview of some key features:
- Laziness: Streams are evaluated lazily, meaning computations are only performed when needed.
- Parallelism: Streams can be processed in parallel for improved performance.
- Functional-style: This makes it easier to read and write complex data processing tasks.
Filtering Basics
The filter
method is used to filter elements based on a predicate (a function that returns a boolean). The typical structure of using filter
looks like this:
list.stream()
.filter(element -> element.meetsCondition())
.collect(Collectors.toList());
This concise syntax enables us to express complex logic in a clear manner. Let's move on to some practical examples.
Example: Simple Filtering
Consider a scenario where we have a list of integers and we want to filter out the even numbers:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class SimpleFilterExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
List<Integer> evenNumbers = numbers.stream()
.filter(num -> num % 2 == 0)
.collect(Collectors.toList());
System.out.println("Even Numbers: " + evenNumbers);
}
}
Why this Works
- Stream Creation: We first convert our list of integers into a Stream.
- Predicate: The
filter
method applies the predicatenum -> num % 2 == 0
to each element, keeping only those that are even. - Collect: Finally,
collect(Collectors.toList())
gathers the filtered elements back into a list.
This example demonstrates how straightforward filtering can be when the condition is simple. However, what if our condition is more complex?
Filtering with Complex Conditions
Let’s explore filtering a list of objects with more intricate conditions. Assume we have a Person
class with attributes like name
, age
, and city
.
Define the Person Class
public class Person {
private String name;
private int age;
private String city;
public Person(String name, int age, String city) {
this.name = name;
this.age = age;
this.city = city;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public String getCity() {
return city;
}
}
More Complex Filtering Example
In this example, we'll filter:
- Persons who are older than 25 and live in "New York".
- Persons whose names start with "A".
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class ComplexFilterExample {
public static void main(String[] args) {
List<Person> people = Arrays.asList(
new Person("Alice", 30, "New York"),
new Person("Bob", 22, "Los Angeles"),
new Person("Anne", 27, "New York"),
new Person("Jason", 32, "Chicago"),
new Person("Maria", 24, "New York")
);
List<Person> filteredPeople = people.stream()
.filter(person -> person.getAge() > 25 && "New York".equals(person.getCity()))
.filter(person -> person.getName().startsWith("A"))
.collect(Collectors.toList());
filteredPeople.forEach(p ->
System.out.println("Filtered Person: " + p.getName()));
}
}
Explanation of the Filtering Conditions
- Chaining Filters: You can chain multiple
filter
calls. Each call processes the previously filtered stream. - Predicate Logic: The first filter checks if the person is older than 25 and lives in "New York". The second filter checks if their name starts with "A".
This flexibility allows for more granular control over which items to keep in your data streams.
Using Predicates for Complex Conditions
If you find yourself repeating predicates across various filtering operations, consider defining a Predicate
interface. This enhances readability and reusability.
import java.util.function.Predicate;
public class ComplexFilterWithPredicate {
public static void main(String[] args) {
// Predicate for age and city
Predicate<Person> ageAndCityPredicate =
person -> person.getAge() > 25 && "New York".equals(person.getCity());
// Predicate for name
Predicate<Person> namePredicate =
person -> person.getName().startsWith("A");
List<Person> filteredPeople = people.stream()
.filter(ageAndCityPredicate)
.filter(namePredicate)
.collect(Collectors.toList());
filteredPeople.forEach(p ->
System.out.println("Filtered Person: " + p.getName()));
}
}
Why Use Predicates?
- Code Clarity: By defining predicates, we make the filter conditions reusable and our code cleaner.
- Easier Debugging: It becomes easier to debug filters since they have clear, separate definitions.
Additional Resources
For those interested in mastering the Streams API in Java, here are some additional resources that provide deeper insights and more examples:
The Bottom Line
Java 8 Streams provide an elegant way to process collections of data with greater expressiveness. Whether you are working with simple or complex filtering conditions, the ability to chain filters and utilize predicates will enhance the quality of your code.
As you continue to master Java Streams, remember to focus on writing clean, maintainable code. The power of the Streams API lies not only in its features but also in how you can leverage those features to solve real-world problems efficiently.
Happy coding!
Checkout our other articles