Uncovering the Surprising Impact of SpotBugs on Code Quality
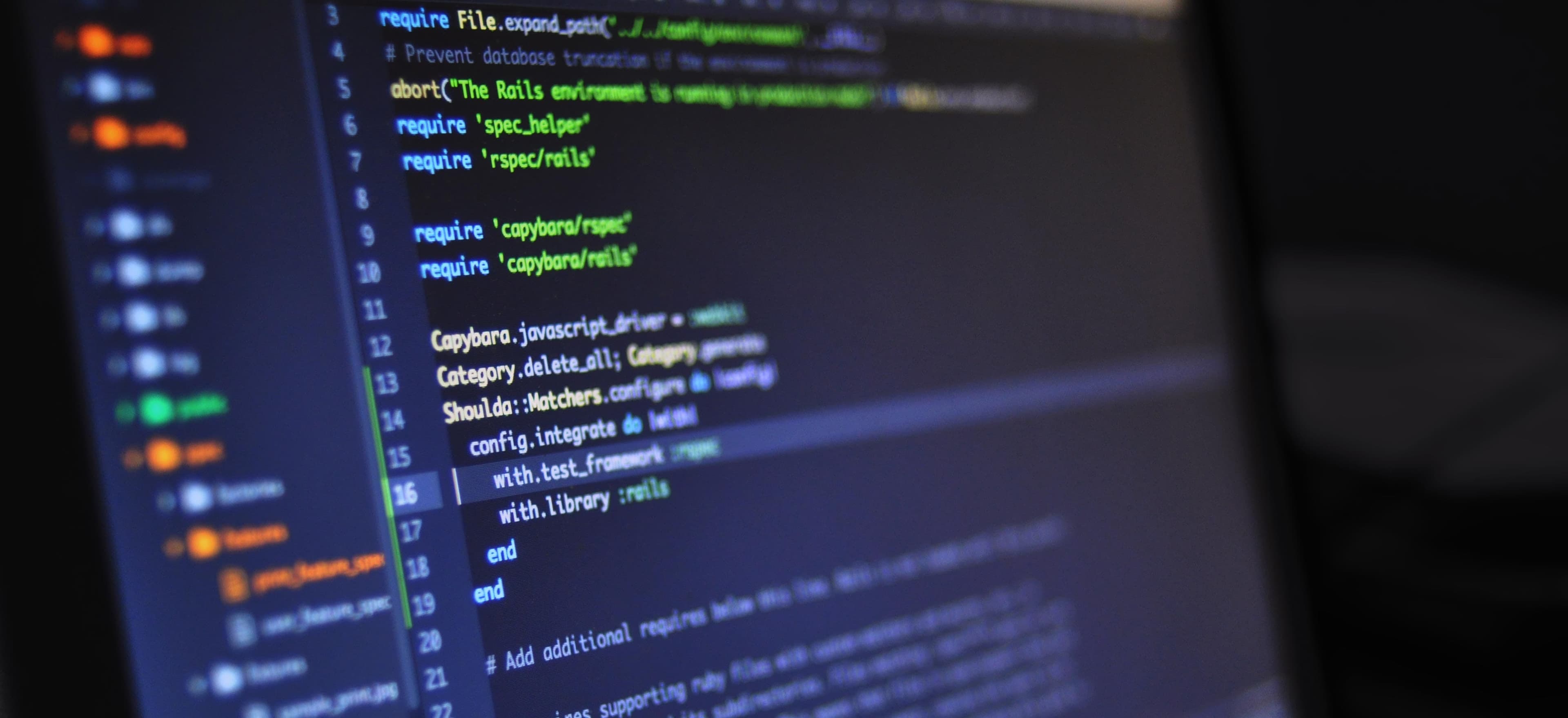
- Published on
Uncovering the Surprising Impact of SpotBugs on Code Quality
In the modern landscape of Java software development, maintaining high code quality is paramount. One of the tools that stand out for improving code quality is SpotBugs. This powerful static analysis tool has gained significant attention for its ability to detect potential bugs in Java code. In this blog post, we will delve into SpotBugs, its functionalities, and the surprising impact it can have on your code quality.
What is SpotBugs?
SpotBugs is an open-source tool used for static analysis of Java bytecode. It identifies potential bugs in Java programs by analyzing the underlying bytecode instead of the Java source code. This approach allows it to find a wider range of issues, including performance bottlenecks, non-compliant coding standards, and potential defects that could lead to runtime errors.
For those looking to integrate SpotBugs into their development workflow, the official SpotBugs GitHub repository offers a wealth of information and resources.
Why Use SpotBugs?
Using SpotBugs can greatly enhance the quality of Java applications. Here are some core reasons:
- Early Detection of Bugs
- SpotBugs detects bugs during the development process, allowing developers to address issues before deployment. This early detection saves time and effort in the long run.
- Wide Coverage of Bug Patterns
- The tool identifies numerous bug patterns ranging from simple issues like unused variables to complex problems like potential thread-safety issues.
- Improved Code Maintainability
- By adhering to best practices highlighted by SpotBugs, the maintainability of the codebase improves, making it easier to understand and modify in the future.
- Community and Support
- As an open-source project, SpotBugs has a supportive community. Developers can report issues, suggest improvements, and contribute to the project.
Getting Started with SpotBugs
Installation
To install SpotBugs, you can either download it directly or integrate it into your build tool. Here, we will outline how to add SpotBugs to Maven and Gradle.
For Maven:
Add the SpotBugs plugin to your pom.xml
:
<build>
<plugins>
<plugin>
<groupId>com.github.spotbugs</groupId>
<artifactId>spotbugs-maven-plugin</artifactId>
<version>4.6.0</version>
<configuration>
<effort>Max</effort>
<reportLevel>Low</reportLevel>
</configuration>
<executions>
<execution>
<goals>
<goal>check</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
This configuration tells Maven to use SpotBugs with the maximum effort level to check for bugs.
For Gradle:
Include the SpotBugs plugin in your build.gradle
file:
plugins {
id 'com.github.spotbugs' version '4.6.0'
}
spotbugs {
effort = 'max'
reportLevel = 'low'
}
Running SpotBugs
After installation, running SpotBugs is straightforward. Simply execute the following command in your project directory:
For Maven:
mvn spotbugs:check
For Gradle:
./gradlew spotbugsMain
Upon completion, you will obtain a report highlighting various potential issues in your code.
Results and Analysis
Once you run SpotBugs, it generates a report that details various issues identified in your code. Let's analyze a few potential results and understand their implications.
Example Bug Report
Consider the following snippet:
public class Example {
public void doSomething() {
String str = null;
int length = str.length(); // This will cause a NullPointerException
}
}
Running SpotBugs on the above code might yield an error like "NullPointerException" detected at the line where str.length()
is called.
Why it Matters
Detecting this potential issue is crucial. A NullPointerException at runtime could lead to application crashes, a poor user experience, and ultimately, loss of revenue. By catching such errors early, SpotBugs enables developers to create more robust applications.
Exploring Common Bug Patterns
SpotBugs identifies various types of bugs, and recognizing some common patterns will aid developers in addressing issues effectively:
- Null Dereference
- SpotBugs can identify areas in the code where an object may be uninitialized before use.
- Infinite Recursion
- When a method calls itself indefinitely, SpotBugs can detect this pattern and notify the developer.
- Resource Leaks
- The tool watches for inputs and outputs where resources (like sockets and database connections) are not closed properly.
- Concurrency Issues
- SpotBugs provides insights into possible data races that can occur in multi-threaded environments.
For a comprehensive list of bug patterns, refer to the SpotBugs documentation.
How SpotBugs Improves Code Quality
1. Encouraging Best Practices
SpotBugs highlights deviations from best practices and coding standards. For example, it may flag a method that isn't thread-safe:
public class Counter {
private int count = 0;
public void increment() {
count++; // Not thread-safe
}
}
This warning encourages developers to implement synchronization mechanisms or use atomic classes for thread-safe operations.
2. Promoting Code Reviews
SpotBugs can serve as a valuable aid during code reviews. Including SpotBugs reports in code reviews ensures potential issues are addressed collectively rather than sidelined until later stages.
3. Enhancing Team Collaboration
By using SpotBugs as a common tool across teams, every developer works towards a shared understanding of code quality. This shared responsibility encourages knowledge transfer and continuous learning among team members.
Integrating SpotBugs into CI/CD
To truly maximize the benefits of SpotBugs, integrating it into your CI/CD pipeline is essential. By automating the analysis process, you can ensure consistent code quality checks with every build.
Example CI/CD Integration
For instance, if you are using GitHub Actions, you can set up a simple workflow to run SpotBugs as part of your CI:
name: Build and Analyze
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Check out the repository
uses: actions/checkout@v2
- name: Set up JDK 11
uses: actions/setup-java@v1
with:
java-version: '11'
- name: Build with Maven
run: mvn clean install
- name: Run SpotBugs
run: mvn spotbugs:check
This configuration checks out the repository, sets up Java, builds the project with Maven, and executes SpotBugs to detect potential issues.
The Bottom Line
SpotBugs has proven to be an indispensable tool for Java developers aiming to enhance code quality. With its ability to detect a wide array of potential issues early in the development process, it empowers developers to write cleaner, more maintainable, and reliable code.
By integrating SpotBugs into your development and CI/CD processes, you will not only elevate the quality of your codebase but also foster a culture of quality among your development team. Whether you are a solo developer or part of a larger organization, the impact of SpotBugs on code quality is clear and well-positioned to surprise you.
Explore more about static analysis tools and their significant benefits here.
Happy coding!