Navigating High Concurrency Issues in JVM HTTP Clients
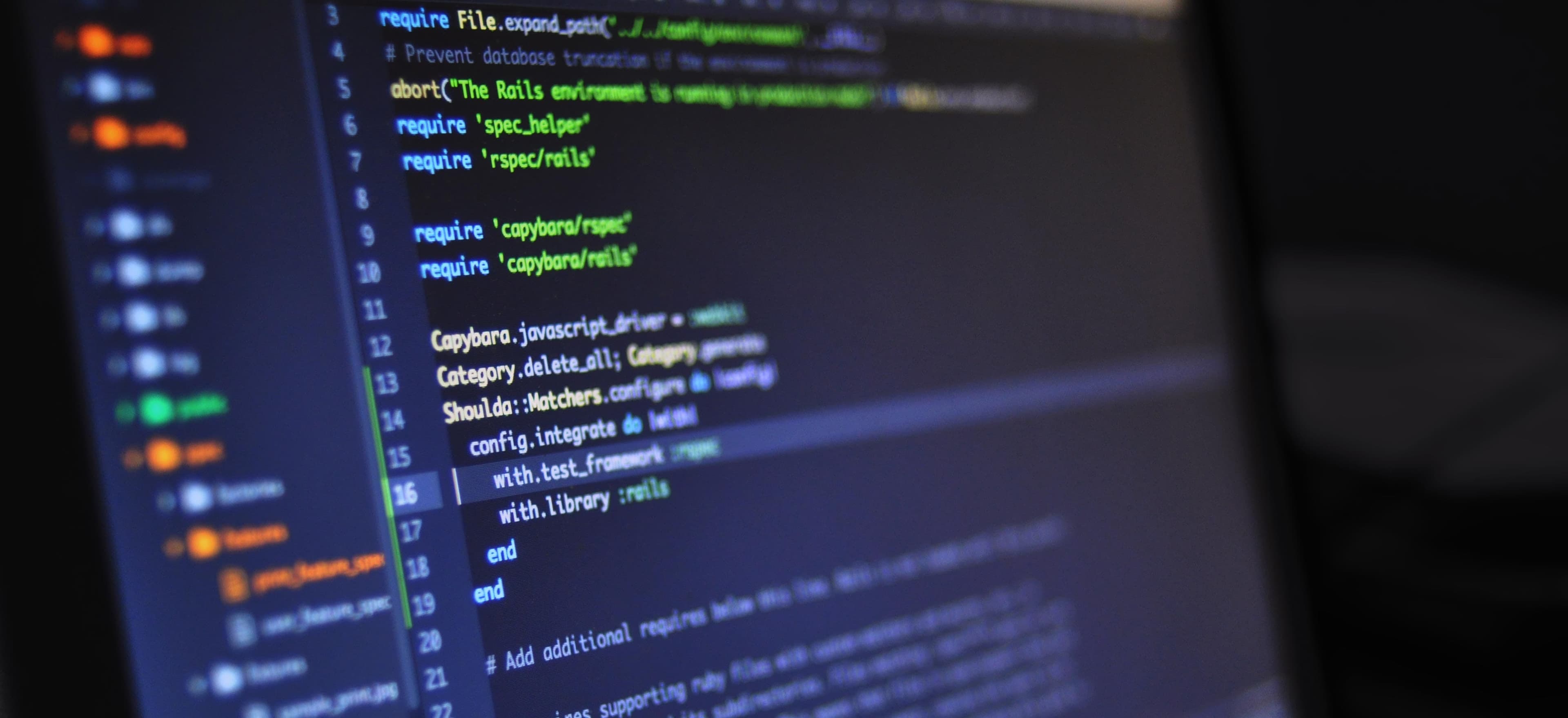
- Published on
Navigating High Concurrency Issues in JVM HTTP Clients
Concurrency is a critical aspect when working with HTTP clients in Java Virtual Machine (JVM) environments. As applications scale to handle multiple simultaneous requests, developers face challenges related to resource contention, thread management, and maintaining optimal performance. In this blog post, we'll explore high concurrency issues commonly encountered when using JVM HTTP clients and provide insights on how to effectively manage these challenges.
Understanding the Concurrency Landscape
Concurrency refers to the ability of a system to handle multiple operations at the same time. In the context of HTTP clients, it means dealing with several requests simultaneously. Java's multithreading capabilities facilitate this, but high concurrency can introduce several complications:
- Resource Contention: Multiple threads may compete for the same resources, leading to performance bottlenecks.
- Scaling Limitations: Applications can become less responsive or even crash under high load if not managed properly.
- Latency Issues: High contention can result in increased response times for users.
To mitigate these issues, understanding the threading model of your HTTP client is essential.
Key HTTP Client Options in Java
1. Apache HttpClient
Apache HttpClient is a robust choice for many developers. It supports various connection pools and allows fine-tuned configurations.
Code Example:
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.impl.conn.PoolingHttpClientConnectionManager;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
public class HttpClientExample {
private static final int MAX_TOTAL_CONNECTIONS = 100;
private static final int MAX_CONNECTION_PER_ROUTE = 10;
public static void main(String[] args) throws Exception {
PoolingHttpClientConnectionManager connectionManager = new PoolingHttpClientConnectionManager();
connectionManager.setMaxTotal(MAX_TOTAL_CONNECTIONS);
connectionManager.setDefaultMaxPerRoute(MAX_CONNECTION_PER_ROUTE);
try (CloseableHttpClient httpClient = HttpClients.custom()
.setConnectionManager(connectionManager)
.build()) {
HttpGet request = new HttpGet("http://example.com");
try (CloseableHttpResponse response = httpClient.execute(request)) {
// Process the response...
}
}
}
}
Commentary:
-
PoolingHttpClientConnectionManager: By utilizing a connection pool, you can manage multiple connections efficiently. The parameters
MAX_TOTAL_CONNECTIONS
andMAX_CONNECTION_PER_ROUTE
control the number of concurrent connections. -
Memory Management: With pooled connections, your application can handle multiple requests without creating excessive overhead.
2. OkHttp
OkHttp is another popular choice known for its performance and ease of use. It provides built-in support for connection pooling and HTTP/2.
Code Example:
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
public class OkHttpClientExample {
private static final OkHttpClient client = new OkHttpClient();
public static void main(String[] args) throws Exception {
Request request = new Request.Builder()
.url("http://example.com")
.build();
try (Response response = client.newCall(request).execute()) {
// Process the response...
}
}
}
Commentary:
-
Connection Pooling: By default, OkHttp manages a shared pool of connections. This feature greatly reduces latency and resource consumption.
-
Simplicity: The API is straightforward, allowing for fast adaptation and implementation.
Best Practices for High Concurrency
When dealing with high concurrency issues in JVM HTTP clients, follow these best practices:
1. Use Connection Pools Wisely
Employ connection pooling effectively to handle multiple requests efficiently. This minimizes the overhead of establishing new connections and optimizes the use of system resources.
2. Tune Pool Parameters
Always configure the parameters based on your application's needs. Monitor your application's performance and adjust parameters like maximum total connections and maximum connections per route based on observed traffic.
3. Asynchronous Calls
Consider using asynchronous HTTP clients to avoid blocking threads, which can lead to resource contention. For example, using libraries like CompletableFuture or reactive libraries like RxJava and Project Reactor can be beneficial.
Example with CompletableFuture:
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.util.concurrent.CompletableFuture;
public class AsyncHttpClientExample {
private static final HttpClient client = HttpClient.newHttpClient();
public static void main(String[] args) {
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("http://example.com"))
.build();
CompletableFuture<HttpResponse<String>> responseFuture = client.sendAsync(request, HttpResponse.BodyHandlers.ofString());
responseFuture.thenAccept(response -> {
// Process the response...
}).join();
}
}
Commentary:
-
Non-blocking Calls: Using
CompletableFuture
allows multiple requests without blocking threads, thus enhancing performance. -
Error Handling: Make sure to handle exceptions that may arise from asynchronous calls to ensure a robust application.
4. Load Testing
Perform load testing on your applications to identify bottlenecks and performance issues under high concurrency. Tools like Apache JMeter or Gatling can be utilized to simulate a high load on your application.
5. Monitor and Profile Your Application
Regularly monitor the performance metrics of your HTTP clients. Profiling your application will help you identify potential bottlenecks and optimize performance settings dynamically.
Final Thoughts
Navigating high concurrency issues in JVM HTTP clients is not without its challenges. However, with a thorough understanding of HTTP client options, proper configuration, and adherence to best practices, you can significantly enhance the performance and reliability of your applications.
Always keep an eye on load testing and monitoring your application's performance to adapt to changing traffic conditions. By prioritizing these practices, you can ensure your applications maintain performance even as you scale to meet user demand.
For further reading on this subject, consider checking out the Apache HttpClient documentation or OkHttp’s official site. These resources will provide you with deeper insights and advanced features that can help you in refining your HTTP client implementation.
By understanding and implementing these strategies, you'll create a more resilient and scalable Java application that performs exceptionally under high concurrency conditions.
Checkout our other articles