Common Pitfalls When Using Hamcrest Matchers in JUnit
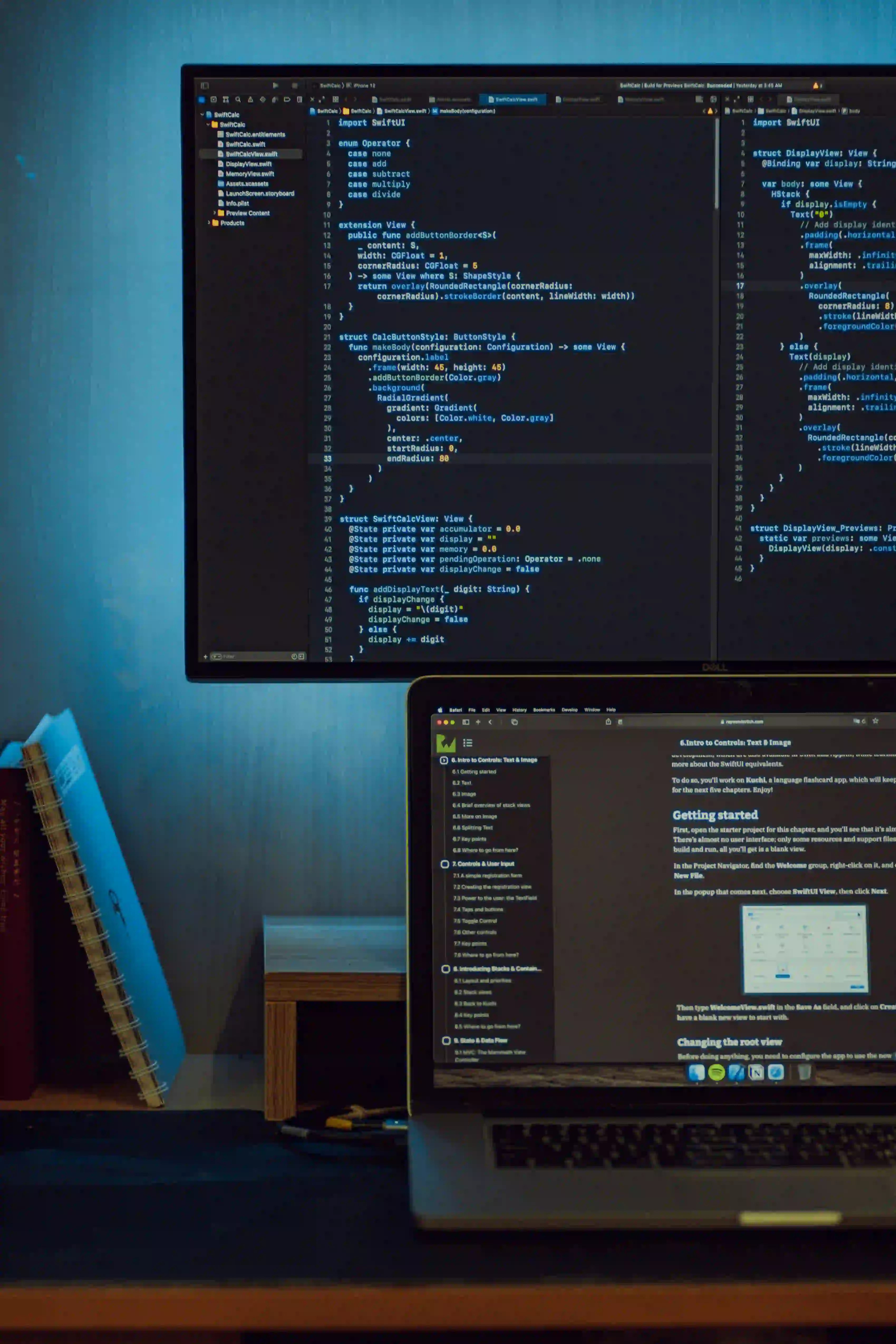
Common Pitfalls When Using Hamcrest Matchers in JUnit
In modern Java development, unit testing is a critical component of software quality assurance. One of the libraries that has risen in popularity for making assertions easier and more readable in unit tests is Hamcrest. When used with JUnit, it provides a more expressive way to validate test conditions. However, even experienced developers can make mistakes when using Hamcrest matchers.
In this post, we'll explore some common pitfalls that developers encounter when using Hamcrest matchers with JUnit, along with practical solutions and best practices.
What is Hamcrest?
Hamcrest is a library that provides a framework for writing matcher objects. You can think of matchers as an API for making assertions in a more readable format. When combined with JUnit, it allows you to construct assertions that read more like natural language. For example, instead of writing:
assertTrue(myList.size() == 3);
You can express this more clearly using Hamcrest matchers:
assertThat(myList, hasSize(3));
While this is a more readable approach, it’s essential to be aware of common missteps when implementing Hamcrest matchers in your tests.
1. Overusing is()
The Pitfall
A common mistake is overusing the is()
matcher. While it's perfectly valid to use is()
, it doesn't always add value. For example:
assertThat(myString, is(equalTo("hello")));
The Fix
Instead, you can simplify:
assertThat(myString, equalTo("hello"));
Using is()
in this context doesn’t provide additional clarity and can make your test code unnecessarily verbose. Use is()
sparingly for improved readability only when required.
2. Ignoring Type Safety
The Pitfall
Hamcrest matchers can sometimes lead to type safety issues when generic types are not well-defined. For instance, if you are using a raw type, you might experience ClassCastException at runtime:
List<String> myList = new ArrayList<>();
myList.add("hello");
myList.add("world");
assertThat(myList, contains("hello", "world")); // No type safety
The Fix
Make sure to use generics properly:
List<String> myList = new ArrayList<>();
myList.add("hello");
myList.add("world");
assertThat(myList, contains("hello", "world")); // This is safe but ensure type is correct
By defaulting on using generic lists, you mitigate the risk of runtime exceptions. Moreover, ensure your matchers are appropriately typed to avoid any ClassCastException
.
3. Misusing anything()
The Pitfall
Another common pitfall is misusing the anything()
matcher, which can lead to tests that are too broad and might not catch errors. For instance:
assertThat(myObject, is(not(anything())));
The Fix
Using anything()
can make your tests less meaningful as they allow any value through. Instead, strive for specific matchers that clarify your expectations:
assertThat(myObject, is(not(nullValue())));
This way, you're ensuring that myObject
is not null, making your tests more precise rather than allowing anything. The clarity of intention improves code maintainability.
4. Not Utilizing Matchers
Effectively
The Pitfall
Developers new to Hamcrest often stick to basic matchers, limiting their capabilities to validate assertions effectively. For example, using only simple matchers like equalTo
without leveraging the full power of Hamcrest can lead to inadequate tests.
The Fix
Explore and use various matchers. Instead of verifying string equality like so:
assertThat(myString, equalTo("stringValue"));
You can expand on this with matchers for strings:
assertThat(myString, startsWith("str"));
assertThat(myString, containsString("ing"));
By utilizing combinations of matchers, you can create more meaningful assertions, and your tests will cover multiple aspects of the expected outcome.
5. Failure to Understand Error Messages
The Pitfall
Hamcrest provides descriptive error messages when tests fail, but developers sometimes ignore these messages, leading to frustration during debugging.
The Fix
Read the failure messages carefully. For instance, if you see:
Expected: a string that starts with "str"
but: was "hello"
This gives you critical information about what went wrong. Always inspect the output carefully, and don't ignore the stack trace. It often provides context crucial for resolving issues.
6. Confusing Boosted Matchers
The Pitfall
Using boosted matchers can lead to confusion, especially when the matcher cannot find what it is looking for. For instance, applying multiple matchers incorrectly can provide incorrect test outcomes:
assertThat(myList, both(hasSize(3)).and(contains("hello")));
The Fix
Make sure that both conditions are clear and that the test context directly relates to both assertions. Use comments or break down complex assertions into separate statements to make them easier to read and debug.
assertThat(myList, hasSize(3)); // Assert size first
assertThat(myList, contains("hello")); // Assert for content next
Breaking assertions into separate statements can enhance clarity, making it easier to identify which condition fails.
Key Takeaways
Using Hamcrest matchers in JUnit offers a powerful way to write expressive and readable tests. However, developers must be mindful of common pitfalls to get the most out of this library. By avoiding issues such as overusing is()
, ignoring type safety, misusing anything()
, and not utilizing matchers effectively, you can develop cleaner, more maintainable tests.
Harnessing the power of Hamcrest will not only improve the readability of your tests but also lead to more robust and descriptive assertions. Refer to the official Hamcrest documentation for more insights and best practices.
Happy testing!