Mastering Spring: Top Interview Questions You Can't Ignore
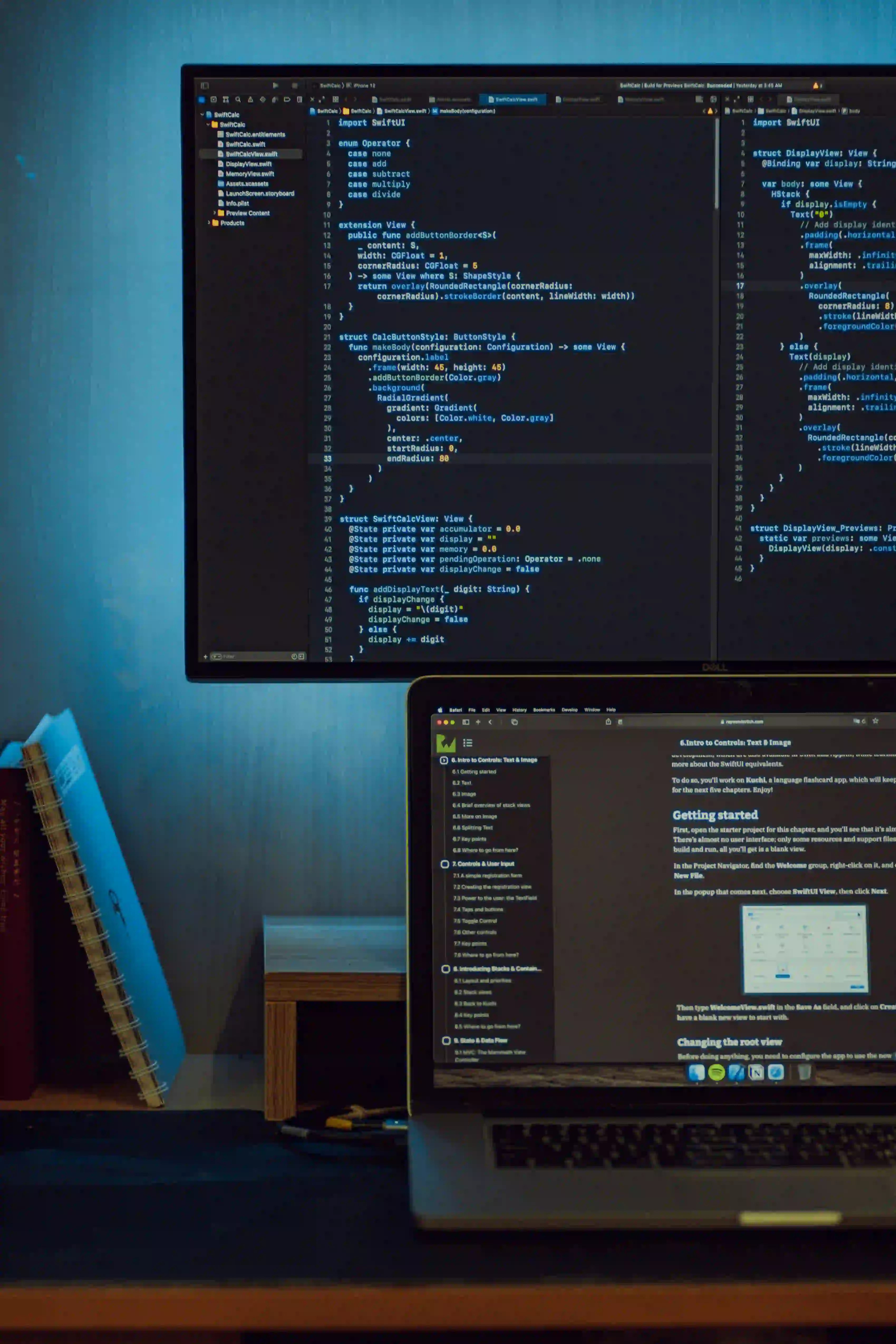
Mastering Spring: Top Interview Questions You Can't Ignore
Spring Framework has become a cornerstone in Java development, especially for building enterprise applications. Its versatility, ease of integration, and powerful features make it a popular choice among developers and companies alike. As you prepare for your next interview, it is crucial to familiarize yourself with common Spring-related questions. In this blog post, we will explore some of the most important Spring interview questions and their answers, enabling you to shine in your upcoming interviews.
1. What is Spring Framework?
The Spring Framework is an open-source application framework for the Java platform. It provides comprehensive infrastructure support for developing Java applications. Spring facilitates the development of Java applications while promoting good design practices.
Key Features of Spring:
- Inversion of Control (IoC): It allows the program to separate the creation of objects from their usage.
- Aspect-Oriented Programming (AOP): This helps in modularizing cross-cutting concerns, such as transaction management.
- Data Access: Spring simplifies data access with its JDBC and ORM integration.
- Transaction Management: The framework provides a consistent programming model for transaction management.
2. What is Dependency Injection in Spring?
Dependency Injection (DI) is a design pattern used to implement IoC, allowing a class to receive its dependencies from external sources rather than creating them itself.
Example of Dependency Injection:
@Component
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
}
In this example, UserService
receives an instance of UserRepository
through constructor injection. This approach promotes loose coupling and enhances testability, as UserService
does not control its dependencies.
3. What are the different types of dependency injection in Spring?
Spring supports three primary types of dependency injection:
-
Constructor Injection: Dependencies are provided through a class constructor.
-
Setter Injection: Dependencies are set through setter methods.
-
Field Injection: Dependencies are injected directly into fields (less preferred due to testability).
Example of Setter Injection:
@Component
public class UserService {
private UserRepository userRepository;
@Autowired
public void setUserRepository(UserRepository userRepository) {
this.userRepository = userRepository;
}
}
In setter injection, we set the dependency after creating the object, providing flexibility to change the dependency at runtime.
4. Explain the Spring Bean Lifecycle.
Spring beans go through several stages in their lifecycle. Understanding these stages is crucial for effective resource management.
Lifecycle Stages:
- Instantiation: The Spring container instantiates the bean.
- Populate properties: Spring populates the properties of the bean.
- Set Bean Name: The bean's name is set if it implements the
BeanNameAware
interface. - Set Bean Factory: The bean factory is set if it implements the
BeanFactoryAware
interface. - Post-process before Initialization: The
BeanPostProcessor
can perform operations before initialization. - Initialize: The bean is initialized by invoking its initialization callback (if any).
- Post-process after Initialization: Another chance for
BeanPostProcessor
to perform operations after initialization. - Ready for use: The bean is ready for use by the application.
- Destruction: The bean is destroyed when the application context is closed (if it implements
DisposableBean
).
!Spring Bean Lifecycle
5. What is Aspect-Oriented Programming (AOP)?
Aspect-Oriented Programming (AOP) allows you to separate cross-cutting concerns (like logging, security, etc.) from business logic. This helps to keep the code cleaner and more manageable.
Key Components of AOP:
- Aspect: A class containing cross-cutting concerns.
- Join Point: A point during execution (e.g., method execution) where an aspect can be applied.
- Advice: Code that is executed at a certain join point.
- Pointcut: An expression that selects join points.
Example of AOP in Spring:
@Aspect
@Component
public class LoggingAspect {
@Before("execution(* com.example.service.*.*(..))")
public void logBefore(JoinPoint joinPoint) {
System.out.println("Executing: " + joinPoint.getSignature().getName());
}
}
In this example, the logBefore
method is executed before any method in the service
package. This gives you powerful logging without cluttering your business code.
6. Explain the difference between @Component, @Service, @Repository, and @Controller annotations.
In Spring, these annotations are used to define beans, but they serve different purposes:
- @Component: A generic stereotype for any Spring-managed component.
- @Service: A specialization of
@Component
, used for service-layer components. - @Repository: A specialization of
@Component
, used for Data Access Objects (DAOs). It also enables exception translation. - @Controller: A specialization of
@Component
, used for web controllers.
These annotations improve the semantics of the code, making it easier to understand the role of each component.
7. What is Spring Boot, and how is it different from Spring Framework?
Spring Boot is an extension of the Spring Framework designed to simplify the setup and development of new Spring applications. It minimizes the amount of configuration needed.
Key Differences:
- Configuration: Spring Boot uses a convention-over-configuration approach to reduce boilerplate code.
- Standalone Apps: Spring Boot applications are standalone, with an embedded web server, eliminating the need for deployment to an external server.
- Auto-Configuration: It provides auto-configuration options that intelligently configure Spring applications based on the dependencies present.
Example of Spring Boot application setup:
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
With @SpringBootApplication
, multiple configurations are handled automatically, simplifying the bootstrapping process.
8. Explain the role of Spring Data and its repository abstraction.
Spring Data provides an easier way to access data storage by minimizing boilerplate code in data access layers. Its repository abstraction manages common CRUD operations through a simple interface, offering flexible methods.
Example of Spring Data Repository:
public interface UserRepository extends JpaRepository<User, Long> {
User findByUsername(String username);
}
Here, UserRepository
extends JpaRepository
, giving you built-in methods such as save()
, findAll()
, and customizable query methods for complex queries.
Closing the Chapter
In this blog post, we have highlighted some essential Spring interview questions, helping you to prepare effectively. Mastering Spring Framework concepts is invaluable not just for interviews, but also for your overall development career. As you prepare, make sure that you not only understand the "how" but also comprehend the "why" behind Spring's design choices.
To enhance your knowledge further, consider reading official Spring documentation at Spring Framework Documentation.
By exploring these fundamental topics, you can position yourself as a knowledgeable candidate ready to tackle Spring-related challenges in your next job interview. Happy coding!