Common Caching Issues in Spring 3.1 and Their Solutions
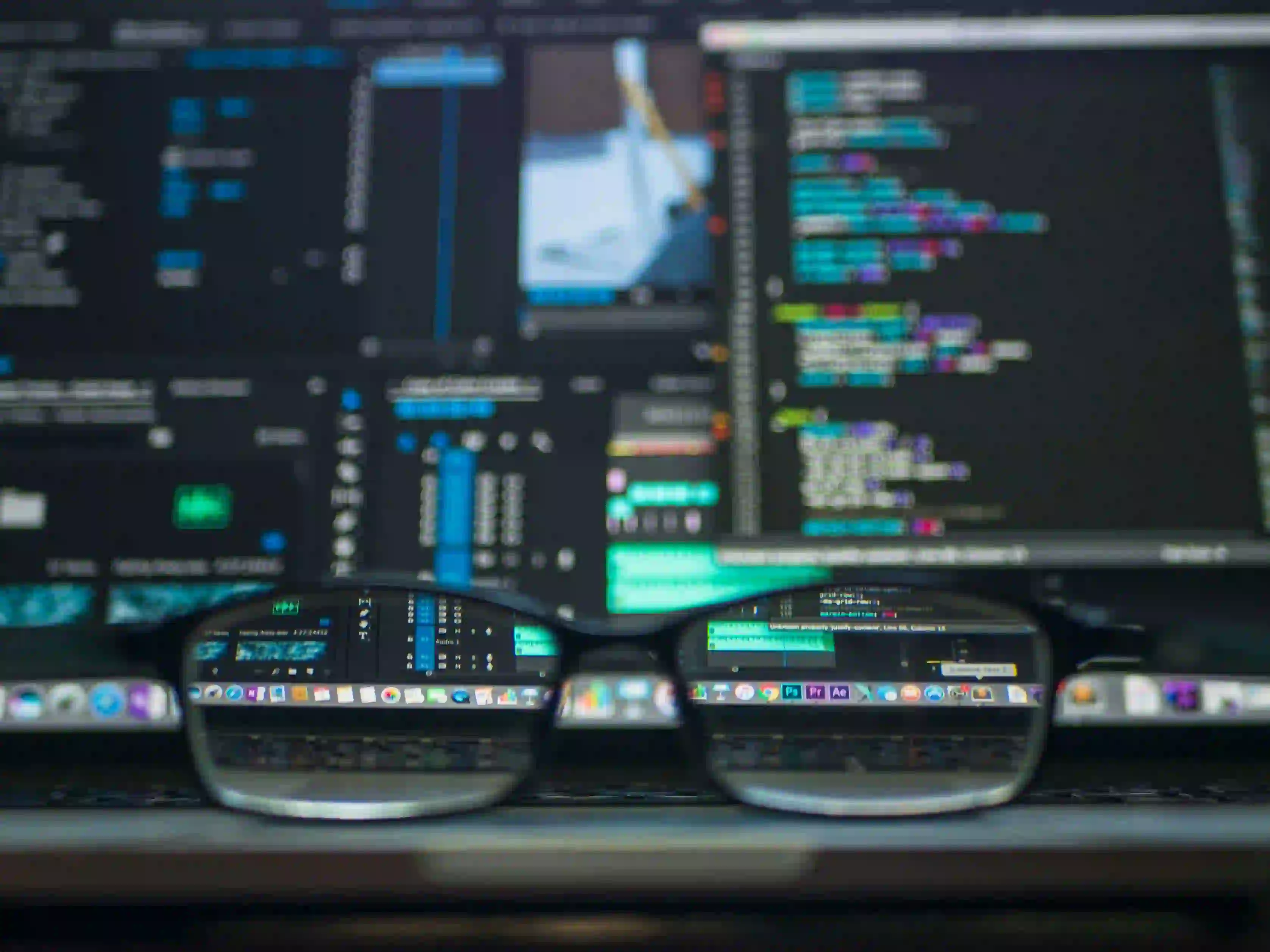
Common Caching Issues in Spring 3.1 and Their Solutions
Caching is a powerful technique used in application development to enhance performance, reduce latency, and improve scalability. In the Spring Framework, caching is supported as part of its core abstractions, which helps in managing caching operations effectively. However, while using caching in Spring 3.1, developers may encounter several common issues. This blog post will discuss these issues and provide solutions along with relevant code examples to help you implement caching effectively in your Spring applications.
What is Caching?
Caching is a process that stores frequently accessed data in a location that can be quickly retrieved. This helps in improving application performance as it reduces the time taken to fetch data. In Java and specifically in Spring applications, caching can be configured to store the results of method calls, data from databases, or expensive computations.
Getting Started with Spring Caching
To use caching in Spring, you need to enable it by adding the @EnableCaching
annotation on your configuration class. Below is a simple example of how to set it up:
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.context.annotation.Configuration;
@Configuration
@EnableCaching
public class CacheConfig {
}
This configuration allows Spring to manage caching using annotations like @Cacheable
, @CachePut
, and @CacheEvict
.
Common Caching Issues in Spring 3.1
1. Cache Not Being Hit
One common issue developers face is that the cache is not hit, meaning the method is always executed instead of returning cached results. This could happen due to several reasons:
-
Key Generation: By default, the cache key is generated based on the method parameters. If the parameters are not passed correctly, the cache will not pick the expected result.
Solution: Explicitly define the key using the
key
attribute.☕snippet.java@Cacheable(value = "books", key = "#isbn") public Book findBookByIsbn(String isbn) { // Expensive call to fetch the book }
Here, using
key = "#isbn"
ensures that theisbn
parameter is effectively utilized for caching.
2. Multi-parameter Methods
When working with methods that have multiple parameters, it can be tricky to generate the cache key correctly. If the method parameters are ignored, the cache won’t work as intended.
Solution: Define a custom key generator or use SpEL (Spring Expression Language) to define the key.
@Cacheable(value = "books", key = "#author + '_' + #title")
public Book findByAuthorAndTitle(String author, String title) {
// Fetch the book by author and title
}
This concatenates the author and title to create a unique key.
3. Cache Eviction Issues
When dealing with updating or removing items from the cache, developers may notice that old data persists longer than expected. This is often caused by improper use of the @CacheEvict
annotation.
Solution: Utilize @CacheEvict
correctly.
@CacheEvict(value = "books", key = "#isbn")
public void removeBook(String isbn) {
// Logic to remove the book from the database
}
Using this annotation will remove the cache entry for the specified isbn
, ensuring that future method calls will fetch updated data.
4. Cache Settings Misconfiguration
Improper cache configuration can lead to severe performance issues. For instance, setting up expiration policies incorrectly, such as using very short TTL (Time to Live) for cache entries, may lead to frequent cache misses.
Solution: Access appropriate cache configuration settings.
Here’s an example of configuring a simple cache manager:
import org.springframework.cache.CacheManager;
import org.springframework.cache.concurrent.ConcurrentMapCacheManager;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class CacheConfig {
@Bean
public CacheManager cacheManager() {
return new ConcurrentMapCacheManager("books");
}
}
With this configuration, make sure to define adequate TTL settings based on your needs.
5. Inconsistent State Between Cache and Database
If the cache does not accurately reflect the current state of the database, this can lead to discrepancies and bugs in your application.
Solution: Use both @CachePut
and @CacheEvict
strategically to maintain synchronization between the cache and the data source.
For example:
@CachePut(value = "books", key = "#book.isbn")
public Book saveBook(Book book) {
// Save the book to the database
return book;
}
@CacheEvict(value = "books", key = "#isbn")
public void removeBook(String isbn) {
// Logic to remove the book from the database
}
Here, the cache is updated every time a book is saved.
6. Transactional Caching Problems
Spring's caching and transaction management can present challenges. If a transactional method is marked with @Cacheable
, the result may not be cached if the transaction fails.
Solution: Consider the implications of transactional caching.
@Transactional
@Cacheable(value = "books", key = "#isbn")
public Book findBook(String isbn) {
// Repository call that can fail
}
Be cautious with mixing the two unless you precisely control the transaction boundaries.
7. Debugging Caching Issues
Debugging cache-related issues can sometimes be challenging. It is beneficial to enable logging for the caching layer to gain insights.
Solution: Configure logging properties in your application.properties
.
logging.level.org.springframework.cache=DEBUG
This configuration will enable detailed logs regarding cache operations.
Closing Remarks
Caching in Spring 3.1 is a robust feature that can significantly boost application performance. However, like any feature, it has its share of challenges. By understanding the common caching issues and their solutions discussed in this post, you can effectively implement caching strategies that optimize your Spring application.
For more detail on caching in Spring, check the Spring Documentation on Caching.
By being proactive about caching issues, you can ensure your application runs smoothly while reaping the benefits of reduced latency and improved user experience.