Debugging Common Dropwizard and MongoDB Integration Issues
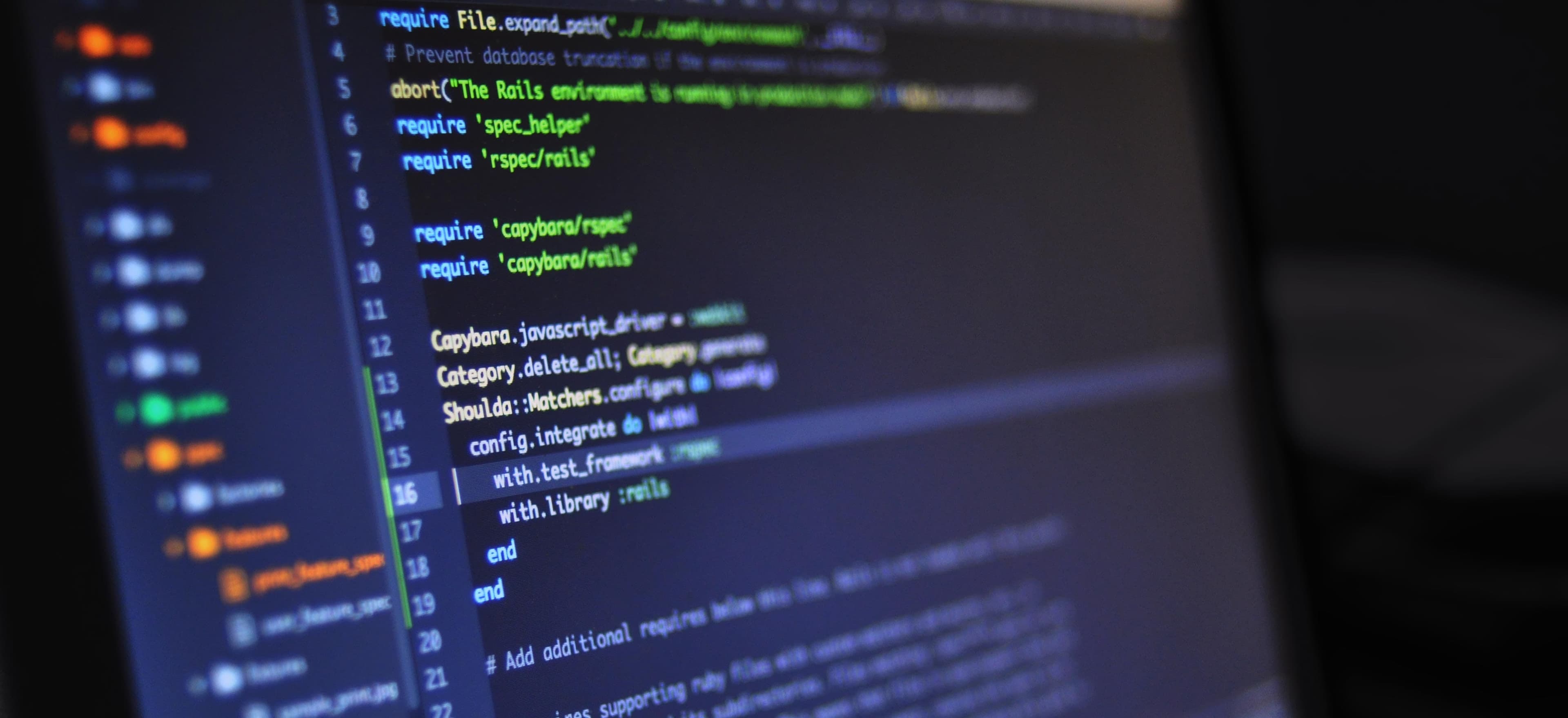
- Published on
Debugging Common Dropwizard and MongoDB Integration Issues
Dropwizard is a powerful framework for building RESTful web services in Java. Coupled with MongoDB, a popular NoSQL database, it provides a robust platform for developing modern applications. However, integrating these two technologies might come with its own set of challenges. In this blog post, we will explore common issues faced during Dropwizard and MongoDB integration and offer effective debugging techniques to resolve them.
Understanding Dropwizard and MongoDB Integration
Before we dive into the debugging process, it’s essential to understand how Dropwizard and MongoDB interact. The integration typically involves:
- Dropwizard: A Java framework that simplifies the development of RESTful web services.
- MongoDB: A NoSQL database that stores data in flexible, JSON-like documents.
When configuring Dropwizard to work with MongoDB, you typically add a MongoDB bundle and then specify your database connection parameters in the config.yml
file.
Example Configuration in config.yml
mongodb:
uri: mongodb://localhost:27017/mydatabase
In the above configuration, we define the URI for connecting to a local MongoDB instance.
Common Integration Issues
1. Connection Issues
One of the most frequent issues developers encounter is failing to connect to MongoDB. This can stem from various factors such as incorrect URI, network issues, or even firewall settings.
Solution Steps:
-
Check the Connection String: Ensure that your URI is properly formatted and your database is running.
-
Firewall and Network Settings: Make sure no firewall rules are blocking the connection to your MongoDB instance.
Code Snippet
Here is an example of how you can check if the connection is successful:
import com.mongodb.MongoClient;
import com.mongodb.MongoClientURI;
public class MongoConnectionTest {
public static void main(String[] args) {
MongoClientURI uri = new MongoClientURI("mongodb://localhost:27017/mydatabase");
try (MongoClient mongoClient = new MongoClient(uri)) {
// The `getDatabase()` method retrieves the database
mongoClient.getDatabase("mydatabase");
System.out.println("Connection to MongoDB was successful.");
} catch (Exception e) {
System.err.println("Connection failed: " + e.getMessage());
}
}
}
2. Configuration Issues in Dropwizard
Incorrect configurations in your config.yml
can prevent your application from starting.
Solution Steps:
-
Validate YAML Syntax: Ensure that your configuration file adheres to proper YAML indentation and syntax.
-
Inspect Logs: Dropwizard provides detailed logs. Look for any errors during startup related to configuration.
3. Data Serialization Issues
When working with MongoDB, a common concern is the conversion of Java objects to BSON (Binary JSON) and vice versa. If your class does not match the expected structure of the MongoDB document, you may run into various errors.
Solution Steps:
-
Use Annotations: Consider adding annotations to your Java classes for better serialization.
-
Implement Custom Serializers/Deserializers: If the default conversion doesn’t suffice, you can implement custom logic.
Example Class with Annotations
import org.bson.codecs.EncodeIntoDocument;
import org.bson.codecs.pojo.annotations.BsonProperty;
public class User {
@BsonProperty(value = "user_id")
private String userId;
@BsonProperty(value = "name")
private String name;
// Constructor, getters, and setters
}
4. Reactive Queries and Performance Issues
Queries that are slow or unresponsive can lead to poor application performance. This may not be an outright error, but it can cause significant slowdowns.
Solution Steps:
-
Normalize Your Data Structure: MongoDB allows you to store nested documents, but sometimes this can lead to complexities that slow down your queries.
-
Indexing: Create appropriate indexes on your MongoDB collections for faster query performance.
Example of Creating an Index
import com.mongodb.client.MongoCollection;
import org.bson.Document;
MongoCollection<Document> collection = mongoClient.getDatabase("mydatabase").getCollection("users");
collection.createIndex(new Document("user_id", 1));
This index creation establishes a performance improvement for queries that use the user_id
field.
5. Dependency Version Conflicts
Sometimes, using incompatible versions of dependencies can lead to absurd runtime errors. For Dropwizard and MongoDB, check their official documentation for compatibility guidelines.
Solution Steps:
-
Review Your Maven/Gradle Dependencies: Ensure you have the correct versions specified.
-
Read Release Notes: Whenever you upgrade, review the release notes for breaking changes.
Additional Resources
For deeper insights into debugging and enhancing your Dropwizard and MongoDB integration, consider visiting:
Key Takeaways
Integrating Dropwizard with MongoDB can take your Java application to the next level of functionality and performance. However, it is not without its challenges. By systematically examining connection settings, configuration files, data serialization, performance metrics, and dependency versions, you can troubleshoot and resolve most issues.
By leveraging the tips and techniques discussed in this blog post, you'll be empowered to create a smoothly running application that effectively utilizes both Dropwizard and MongoDB. Happy coding!
Checkout our other articles