Mastering Java's Varargs: Handle Primitive Arrays with Ease
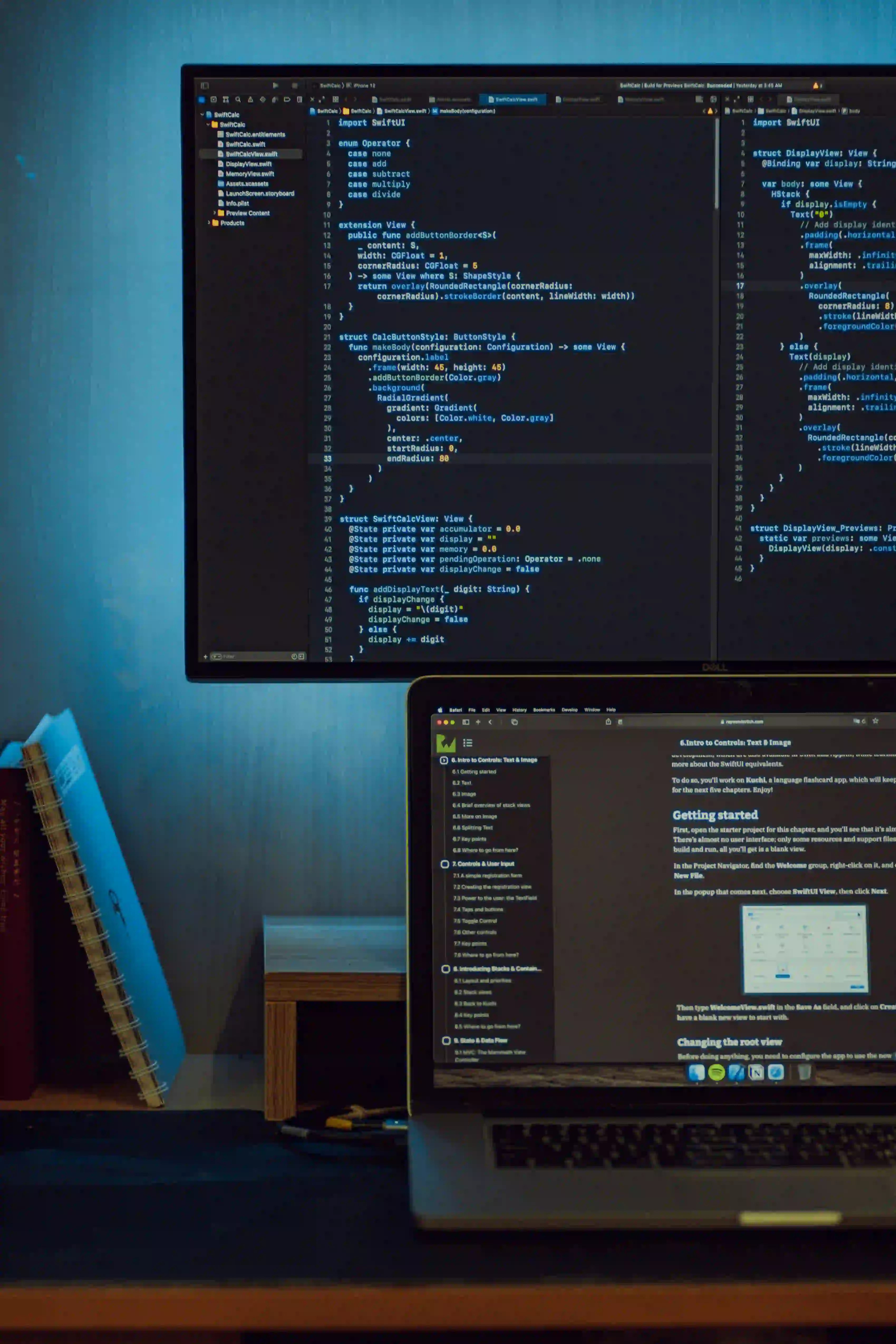
Mastering Java's Varargs: Handle Primitive Arrays with Ease
In the realm of Java programming, the term "Varargs" is a convenient shorthand for variable-length arguments. Introduced in Java 5, it allows developers to pass a variable number of arguments to methods. This feature streamlines code, enhances readability, and offers flexibility when dealing with arrays. In this blog post, we will dive deep into the concept of Varargs, focusing on how to handle primitive arrays effectively.
What Are Varargs?
Varargs simplifies method definitions that require a variable number of parameters. With the use of an ellipsis (...) in your method signature, you can accept zero or more arguments of the same type. For example, if you want to create a method that calculates the sum of numbers without having to overload it for different numbers of parameters, Varargs can do the job effortlessly.
Example of a Varargs Method
Here's a simple illustration of how a Varargs method can be defined:
public class VarargsExample {
public static int sum(int... numbers) {
int total = 0;
for (int number : numbers) {
total += number;
}
return total;
}
}
In this example, the sum
method can take any number of int
arguments. The method iterates over each number in the numbers
array and calculates the total.
Why Use Varargs?
- Code Simplification: Instead of creating multiple overloaded methods for different numbers of parameters, you can create a single Varargs method.
- Improved Readability: The method signature can remain clean and understandable, making the code easier to read and maintain.
Primitive Arrays and Varargs
While Varargs can handle wrapper types seamlessly, managing primitive data types is where developers may face challenges. Understanding how to deal with primitive arrays while utilizing Varargs is pivotal.
Creating Primitive Arrays with Varargs
Using Varargs can lead you to think that the method automatically converts primitive types into arrays for you. Let's break this down with a practical example:
public class VarargsWithPrimitives {
public static void printNumbers(int... numbers) {
for (int number : numbers) {
System.out.println(number);
}
}
public static void main(String[] args) {
printNumbers(1, 2, 3, 4, 5);
// It will display:
// 1
// 2
// 3
// 4
// 5
}
}
Why This Works
When invoking printNumbers(1, 2, 3, 4, 5);
, the integers are treated as a primitive array behind the scenes. Java automatically creates an array of int
types to accommodate the Varargs feature in this context.
Key Considerations
Though Varargs is a powerful tool, it's important to understand its limitations and nuances:
- Single Varargs Parameter: A method can only have one Varargs parameter, and it must be the last one in the parameter list. For example:
public void exampleMethod(String str, int... numbers) {
// valid
}
public void exampleMethod(int... numbers, String str) {
// invalid - Varargs must be the last parameter
}
-
Array of Varargs: Inside a method, the Varargs parameter is treated as an array. This means you can use it similarly to any normal array in Java, such as iterating over it or utilizing its length property.
-
Non-Primitives: Varargs can seamlessly handle both primitive and wrapper types. For example, Integer, String, and Double are all valid Varargs types.
Example Application
Here is an example of how you can leverage Varargs in a practical scenario. Let’s create a method that finds the maximum value from a list of integers using Varargs.
public class MaxFinder {
public static int findMax(int... numbers) {
if (numbers.length == 0) {
throw new IllegalArgumentException("At least one number is required");
}
int max = numbers[0];
for (int number : numbers) {
if (number > max) {
max = number;
}
}
return max;
}
public static void main(String[] args) {
int max = findMax(5, 12, 3, 67, 25);
System.out.println("Max value: " + max);
// Output: Max value: 67
}
}
Why This Code Matters
This findMax
method adheres to the principle of clean code by following a straightforward approach with the Varargs feature. The explicit check for an empty input array ensures robustness.
Advantages of Using Varargs
- Increased Flexibility: Varargs promotes code that adapts to calling conventions without strict requirements on input.
- Simplicity in Syntax: Reduces clutter with multiple overloaded methods, making the method easier to use and remembering it simpler.
Best Practices
- Use for Natural Grouping: Varargs is best utilized when you logically group similar parameters together.
- Document Your Methods: Always document methods using Varargs clearly so other developers understand what they expect.
- Consider Performance: Though Varargs is convenient, creating a new array each time can introduce overhead. Use it selectively where clarity and ease outweigh performance concerns.
Final Considerations
Mastering Java's Varargs feature opens up new horizons in writing efficient and readable code. By effectively managing primitive arrays through Varargs, you can create clearer, more maintainable solutions to everyday programming challenges.
For further reading on this topic, refer to the official Java Documentation.
Keep experimenting, keep coding, and let Java's Varargs simplify your programming endeavors!