Common Errors in BIRT Reports and How to Fix Them
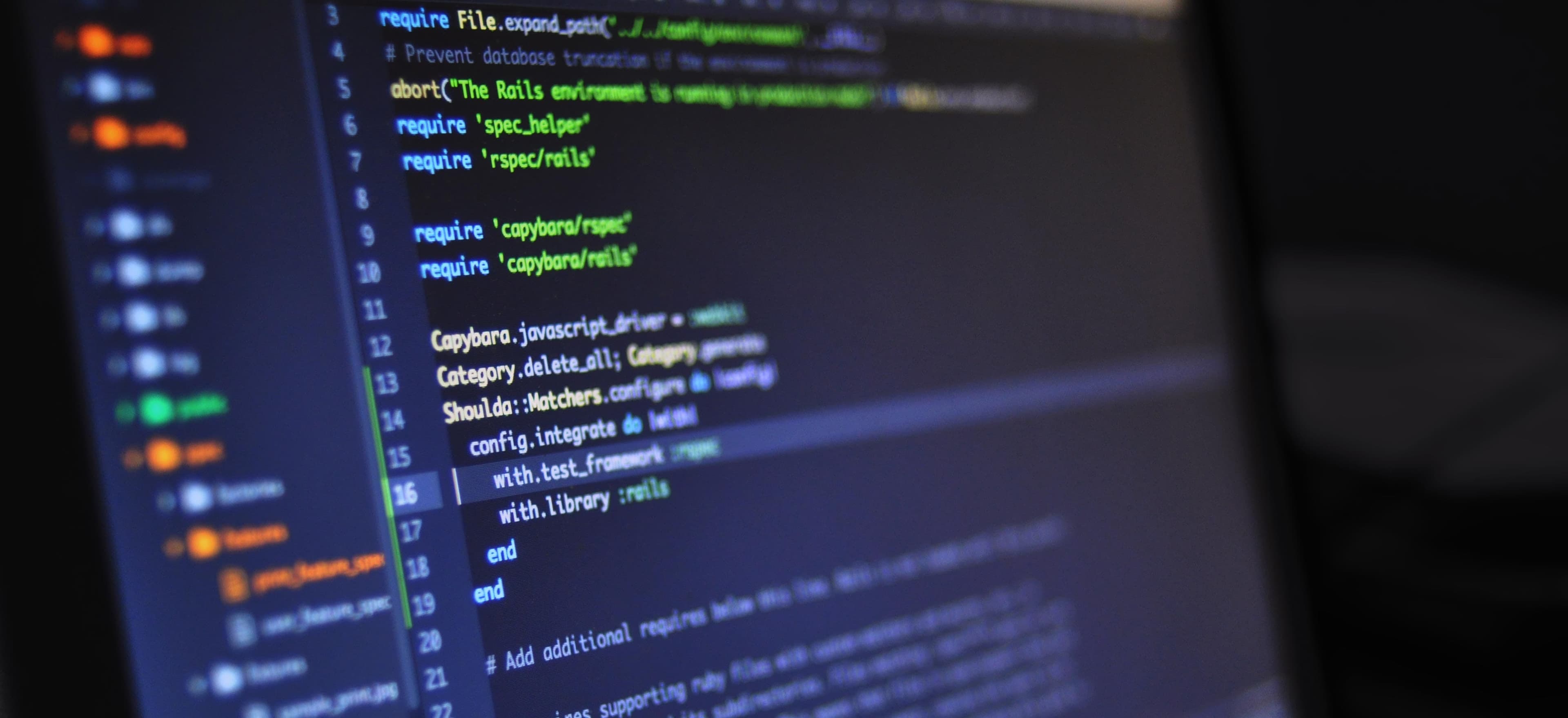
- Published on
Common Errors in BIRT Reports and How to Fix Them
Business Intelligence and Reporting Tools (BIRT) is a powerful open-source reporting system that can extract, manipulate, and present data in various formats. Despite its extensive capabilities, users often encounter a few common errors when working with BIRT reports. This blog post aims to identify those errors, explain their causes, and provide step-by-step solutions to rectify them.
Understanding BIRT: A Quick Overview
BIRT is predominantly used for reporting in Java EE applications, allowing users to create compelling reports using an intuitive interface. It supports various data sources, such as SQL databases, XML files, and Web Services, making it a versatile choice in many environments. However, even experienced users can face hurdles, largely due to misconfigurations, coding mistakes, and environmental setups.
Importance of Debugging in BIRT
Debugging is an essential part of the development process. Understanding how to diagnose and fix errors in BIRT can save time and reduce frustration. BIRT provides several tools, including logs and a preview mode, to aid developers and users in identifying issues.
Common BIRT Errors and Solutions
1. Data Source Connection Errors
Description: Often, reports fail to generate due to incorrect data source configurations.
Causes:
- Incorrect Database URL
- Missing drivers
- Invalid database credentials
Solution: To resolve connection issues, double-check your data source settings.
- Open your report in the BIRT Report Designer.
- Navigate to the "Data Explorer" pane and expand "Data Sources."
- Right-click your data source and select "Edit."
- Verify the Database URL, username, and password.
Here’s a basic example of how to set up a JDBC connection for a MySQL database.
// Example JDBC Connection
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "username";
String password = "password";
Connection connection = null;
try {
Class.forName("com.mysql.cj.jdbc.Driver");
connection = DriverManager.getConnection(url, user, password);
// Connection successful, proceed with queries
} catch(SQLException e) {
System.err.println("Connection failed: " + e.getMessage());
} finally {
if(connection != null){
try {
connection.close();
} catch(SQLException e) {
System.err.println("Failed to close connection: " + e.getMessage());
}
}
}
2. Report Preview Issues
Description: When trying to preview the report in the BIRT Viewer, users often see blank pages or errors.
Causes:
- Empty data set
- Incorrect report parameter values
Solution: To troubleshoot preview issues:
- Check if the report dataset is correctly populated.
- Ensure that all report parameters are set with valid values.
When working with parameters, it is crucial to validate them using expressions. For instance:
// Example of a report parameter validation
if (reportContext.getParameterValue("startDate") == null) {
throw new Exception("Start Date cannot be null");
}
3. Rendering Errors
Description: Rendering errors might occur due to incompatible elements or formats during export.
Causes:
- Using unsupported features in PDF/HTML exports
- Incorrect report design layouts
Solution: Check your report elements:
- Avoid complex nested tables or high-resolution images, which can lead to rendering issues.
- Test the report by exporting to different formats to identify the problematic element.
To ensure compatibility, keep the report layout simple. Here’s an example of a basic table setup.
<Table>
<TableHeader>
<TableRow>
<TableCell>Header 1</TableCell>
<TableCell>Header 2</TableCell>
</TableRow>
</TableHeader>
<TableDetail>
<DataSetRow dataSet="myDataSet">
<TableRow>
<TableCell><dataColumn1/></TableCell>
<TableCell><dataColumn2/></TableCell>
</TableRow>
</DataSetRow>
</TableDetail>
</Table>
4. Parameter Passing Errors
Description: Parameters may not work as expected when integrated with Java applications.
Causes:
- Incorrect parameter binding in the calling Java code
- Missing parameter definitions in the report
Solution: Ensure parameters are correctly defined and passed from your Java code.
Here’s an example of binding parameters in Java:
// Example of passing parameters
ReportEngine reportEngine = new ReportEngine(configuration);
IReportRunnable design = reportEngine.openReportDesign("path/to/report.rptdesign");
IRunAndRenderTask task = reportEngine.createRunAndRenderTask(design);
// Set parameters
task.setParameterValue("param1", value1);
task.setParameterValue("param2", value2);
// Execute report
task.run(params);
5. Performance Issues
Description: Large datasets may lead to significant performance degradation when generating reports.
Causes:
- Inefficient queries
- Large number of report elements
Solution: To enhance performance:
- Optimize SQL queries to fetch only necessary data.
- Limit the number of data elements displayed on the report.
- Utilize pagination to handle large datasets.
Consider implementing a SQL query like this:
SELECT id, name FROM users WHERE active = 1 LIMIT 1000
Using pagination techniques and batching data can drastically improve report generation times.
In Conclusion, Here is What Matters
BIRT is a robust tool for creating standardized reports, but like any technology, it comes with its own set of challenges. By understanding the common errors, their causes, and the potential solutions detailed in this blog post, you can navigate BIRT with increased confidence.
For further reading and resources:
- BIRT Official Documentation
- Common BIRT Report Types
- Debugging BIRT Reports
Armed with this information, you can troubleshoot your BIRT reports effectively and optimize your reporting processes for better end-user experiences.