Decrypting Crypto Quirks: JDK Cipher Streams Exposed!
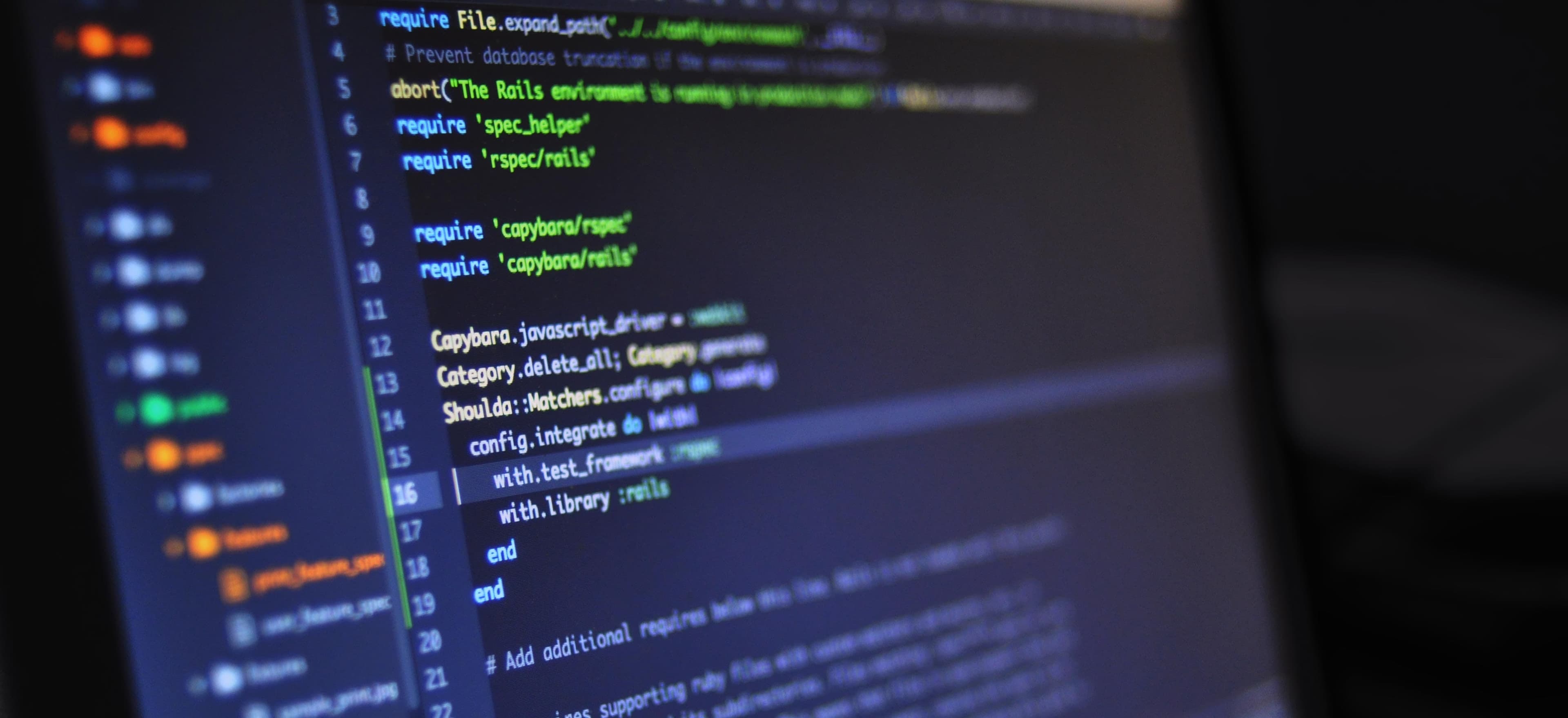
- Published on
Decrypting Crypto Quirks: JDK Cipher Streams Exposed!
Encryption and decryption are critical aspects of data security in today's digital age. Java provides robust libraries for cryptography, allowing developers to implement secure data transmission. In this post, we will dive deep into Java's JDK Cipher Streams, understanding how they work, their inner workings, and best practices to employ them in your applications.
Understanding Cipher Streams
Before we get into the code, let’s clarify what Cipher Streams are. In Java, the javax.crypto
package offers a straightforward way to encrypt and decrypt data using streams. This functionality is vital for applications that deal with sensitive information, such as online banking, personal messages, or confidential documents.
The major classes within this package are Cipher
, CipherInputStream
, and CipherOutputStream
. These classes come together to create a fluid and efficient cryptographic experience.
What is a Cipher?
The Cipher
class is a centerpiece of the cryptographic architecture in Java. It provides the means to encrypt and decrypt data based on various algorithms like AES, DES, RSA, and more.
Example: Initializing a Cipher
Here’s how you can initialize a cipher for AES encryption:
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import java.security.NoSuchAlgorithmException;
public class CipherExample {
public static void main(String[] args) throws Exception {
// Initialize the cipher to AES algorithm
Cipher cipher = Cipher.getInstance("AES");
// Generate a secret key
KeyGenerator keyGen = KeyGenerator.getInstance("AES");
keyGen.init(128); // Specify key size
SecretKey secretKey = keyGen.generateKey();
// Initialize cipher for encryption
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
// Now, it's ready to encrypt data!
}
}
Commentary: Why Use This Code Snippet?
-
Cipher Initialization: The
Cipher.getInstance()
method takes a transformation string that specifies the algorithm. In this case, we are using AES due to its security strengths. -
Key Generation: Generating a secret key is critical for the encryption process. A strong key, specified here as 128 bits, ensures the security of your encrypted data.
-
Mode Setting: The
init
method allows you to set the cipher mode (encryption or decryption) along with the secret key. This setup is crucial for processing data securely.
CipherInputStream and CipherOutputStream
With our cipher initialized, we can proceed to the CipherInputStream
and CipherOutputStream
. These classes are used to stream data through the cipher, allowing you to encrypt and decrypt data incrementally rather than loading entire files into memory.
Example: Writing Encrypted Data
Here’s an example that shows how to write data to a file using CipherOutputStream
:
import javax.crypto.CipherOutputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class EncryptFileExample {
public static void main(String[] args) {
try {
SecretKey secretKey = generateSecretKey();
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
try (FileOutputStream fileOut = new FileOutputStream("encryptedData.dat");
CipherOutputStream cipherOut = new CipherOutputStream(fileOut, cipher)) {
String data = "Sensitive information here";
cipherOut.write(data.getBytes());
}
} catch (Exception e) {
e.printStackTrace();
}
}
private static SecretKey generateSecretKey() throws NoSuchAlgorithmException {
KeyGenerator keyGen = KeyGenerator.getInstance("AES");
keyGen.init(128); // Specify key size
return keyGen.generateKey();
}
}
Commentary: Why Use Cipher Output Stream?
-
Efficient Writing: Using
CipherOutputStream
, we can encrypt data as it is being written to a file. This is memory-efficient for large data sets. -
File Handling: The try-with-resources statement ensures that resources are properly closed, preventing resource leaks.
-
Data Integrity: Encrypting data before saving adds a layer of security, making it difficult for unauthorized entities to read the information.
Decrypting Data
Decryption follows a similar structure as encryption but requires the same secret key used for encryption. Below is an example of how to read encrypted data using CipherInputStream
.
Example: Reading Decrypted Data
import javax.crypto.CipherInputStream;
import java.io.FileInputStream;
import java.io.IOException;
public class DecryptFileExample {
public static void main(String[] args) {
try {
SecretKey secretKey = retrieveSecretKey(); // Ensure retrieval of the same secret key
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.DECRYPT_MODE, secretKey);
try (FileInputStream fileIn = new FileInputStream("encryptedData.dat");
CipherInputStream cipherIn = new CipherInputStream(fileIn, cipher)) {
byte[] buffer = new byte[256];
int numBytesRead;
StringBuilder data = new StringBuilder();
while ((numBytesRead = cipherIn.read(buffer)) != -1) {
data.append(new String(buffer, 0, numBytesRead));
}
System.out.println("Decrypted Data: " + data.toString());
}
} catch (Exception e) {
e.printStackTrace();
}
}
private static SecretKey retrieveSecretKey() {
// Logic to retrieve the secret key should be added here
return null; // Replace null with actual key retrieval code
}
}
Commentary: Why Use Cipher Input Stream?
-
Real-time Decryption: Decrypting data on-the-fly as it is read helps minimize memory usage and makes it easier to handle large files.
-
Robust Implementation: By using a buffer, we read chunks of data from the input stream and decrypt them incrementally, which is efficient and prevents potential data overflow.
-
Secure Key Handling: Always ensure secure handling of your keys. Consider using a secure vault or storing keys in an environment variable.
Best Practices for Using JDK Cipher Streams
-
Use Strong Key Sizes: Always opt for secure key lengths, typically at least 128 bits for standard tasks. Enhance your security by considering 256-bit keys if your platform supports it.
-
Manage Keys Securely: Never hard-code your keys in the source code. Use secure storage mechanisms like AWS KMS, HashiCorp Vault, or similar tools.
-
Use IVs for Symmetric Algorithms: When using modes like AES in CBC mode, always initialize the cipher with an Initialization Vector (IV) to ensure that identical plaintexts produce different ciphertexts each time.
-
Regularly Update Dependencies: Keep libraries and dependencies updated to ensure you are protected against known vulnerabilities.
-
Catch Exceptions: Implement thorough exception-handling mechanisms. This will not only help in debugging but also protect your application from unexpected behavior.
The Last Word
Java’s JDK provides a powerful set of tools for encryption and decryption using Cipher Streams. By understanding how to leverage Cipher
, CipherInputStream
, and CipherOutputStream
, you can implement secure data handling in your applications effectively.
As you work with encryption, always remember to maintain best practices for security. If you want to dive deeper, consider reading the Java Cryptography Architecture documentation for comprehensive insights.
By prioritizing data security through proper encryption mechanisms, you can significantly bolster the protection of sensitive information. In an era where data breaches are rampant, the importance of implementing robust cryptography cannot be overstated!
Now that we have uncovered the native capabilities of Java's Cipher Streams, your applications are one step closer to being fortified against unwanted access. Happy coding!
Checkout our other articles