Understanding Akka's Complexity in Request-Response Patterns
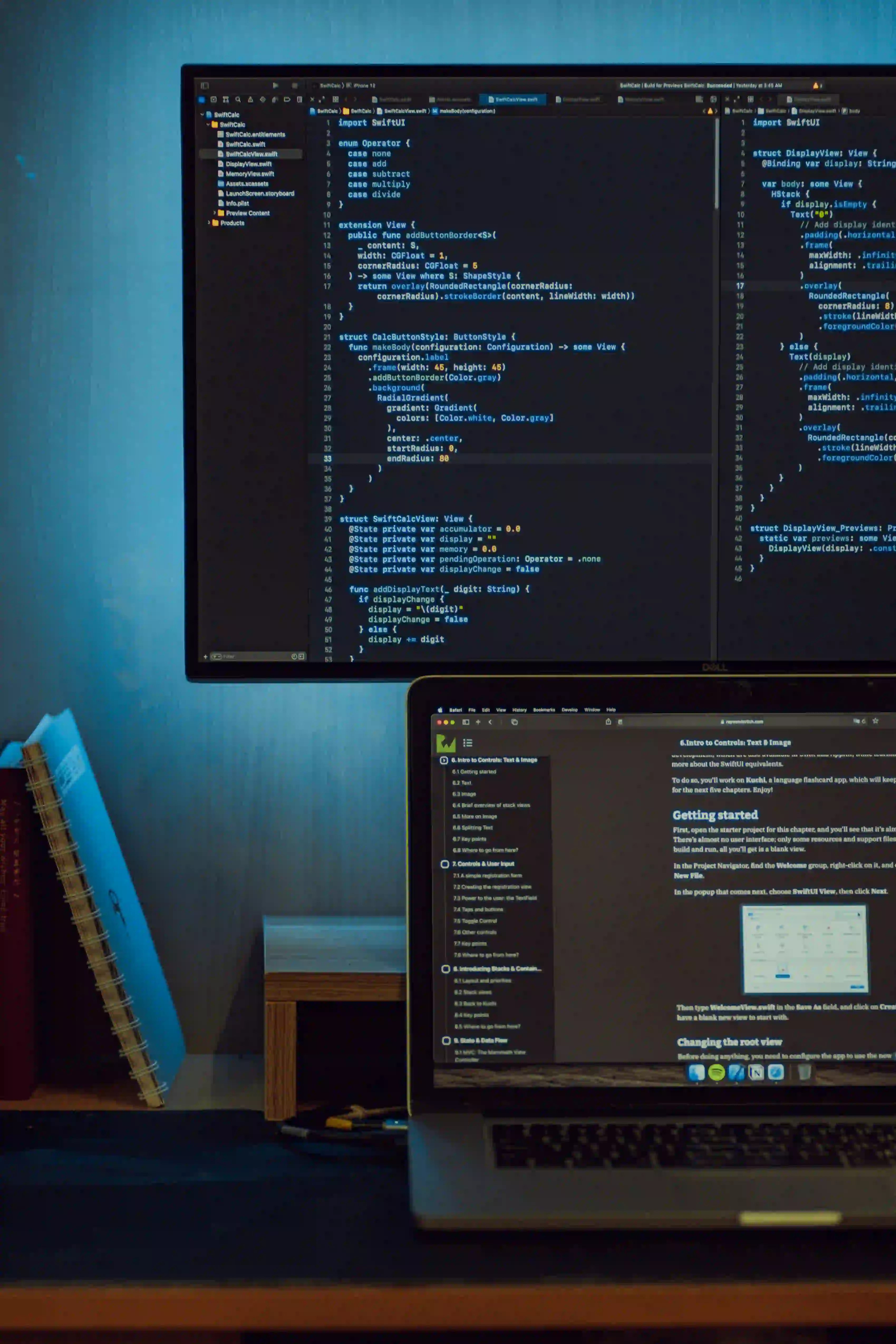
Understanding Akka’s Complexity in Request-Response Patterns
Akka is a powerful toolkit for building concurrent applications in Java and Scala. But like any robust framework, it comes with its complexities, especially when dealing with request-response patterns. In this post, we will deconstruct Akka's approach to handling such patterns, elaborating on its utility, discussing its challenges, and offering code snippets to illustrate key concepts.
What is Akka?
Akka leverages the Actor model, allowing developers to write highly scalable and resilient applications. The core of Akka's design is its ability to handle asynchronous communication, enabling the execution of multiple tasks concurrently without blocking the main thread.
To harness Akka effectively, you must familiarize yourself with the concepts of actors, messages, and the asynchronous nature of actor communication. This is particularly essential when you're implementing request-response patterns, which are ubiquitous in today's software applications.
The Basics of Request-Response in Akka
A request-response pattern facilitates synchronous communication between different parts of the system. In Akka, this typically involves an actor sending a message and waiting for a response.
Why Use Request-Response?
- Clarity: It provides a clear path for communication.
- Control: You can effectively manage the flow of the application.
- Tight Coupling: It allows direct interaction between components.
However, these benefits come with caveats, especially regarding complexity and error handling.
A Simple Actor Example
Let’s start with a basic actor setup that demonstrates a request-response scenario.
import akka.actor.AbstractActor;
import akka.actor.ActorRef;
import akka.actor.ActorSystem;
import akka.actor.Props;
import akka.pattern.Patterns;
import akka.util.Timeout;
import scala.concurrent.Await;
import scala.concurrent.Future;
import scala.concurrent.duration.Duration;
import java.util.concurrent.CompletableFuture;
public class RequestResponseExample {
static class RequestActor extends AbstractActor {
@Override
public Receive createReceive() {
return receiveBuilder()
.matchEquals("getTimestamp", msg -> {
// Responds with the current timestamp.
getSender().tell(System.currentTimeMillis(), getSelf());
})
.build();
}
}
public static void main(String[] args) throws Exception {
ActorSystem actorSystem = ActorSystem.create("RequestResponseSystem");
ActorRef requestActor = actorSystem.actorOf(Props.create(RequestActor.class), "requestActor");
// Define the timeout for the future response
Timeout timeout = new Timeout(Duration.create(5, "seconds"));
Future<Object> future = Patterns.ask(requestActor, "getTimestamp", timeout);
// Await the response
Long timestamp = (Long) Await.result(future, timeout.duration());
System.out.println("Received Timestamp: " + timestamp);
actorSystem.terminate();
}
}
Breakdown of the Code
- Actor Creation: We set up a straightforward
RequestActor
that listens for messages. When it receives "getTimestamp", it responds with the current timestamp. - Future with Patterns.ask: The main method uses the
Patterns.ask
method, which sends a message to the actor and returns aFuture<Object>
. This represents the eventual result of the asynchronous request. - Await for Response: We use
Await.result
to block until the response is received. While this is useful for demonstration, blocking is generally discouraged in Akka.
Understanding the Complexity
Using the Actor model provides numerous advantages, notably scalability and responsiveness. However, when implementing request-response patterns, certain complexities arise.
- Error Handling: Unlike traditional synchronous calls, managing exceptions in asynchronous calls can be challenging. Actors can fail silently if not configured properly.
- Timeouts: If a request takes too long, it may lead to stuck actors or unresponsive applications. You should always define timeouts.
- Message Size: Large messages can bottleneck the system. Keep communication concise.
Alternatives to Request-Response
Sometimes request-response is not the optimal approach. In such cases, here are some alternatives:
- Fire-and-Forget: This pattern allows sending messages without waiting for a response. It promotes some level of decoupling but at the expense of knowing the state of the process.
- Pub/Sub Model: Instead of one-to-one communication, you can use a publish-subscribe model to notify multiple subscribers of changes.
Both methods reduce coupling but require careful planning to ensure activities are coordinated effectively.
Advanced Request-Response Patterns
In elaborating on Akka's request-response capabilities, we encounter scenarios where advanced patterns can be beneficial—like Circuit Breakers or using Event Sourcing to manage state.
Incorporating Circuit Breakers
Using Circuit Breakers can help manage failures more gracefully. This is particularly useful when dealing with external HTTP services or databases.
Here’s how you can apply a circuit breaker pattern in your Akka application:
import akka.actor.AbstractActor;
import akka.actor.ActorRef;
import akka.actor.ActorSystem;
import akka.actor.Props;
import akka.pattern.CircuitBreaker;
import scala.concurrent.duration.Duration;
import scala.concurrent.Future;
import java.util.concurrent.CompletableFuture;
public class CircuitBreakerExample {
static class ServiceActor extends AbstractActor {
private final CircuitBreaker circuitBreaker;
public ServiceActor(CircuitBreaker circuitBreaker) {
this.circuitBreaker = circuitBreaker;
}
@Override
public Receive createReceive() {
return receiveBuilder()
.match(String.class, msg -> {
// Simulate a risky call
Future<Object> future = circuitBreaker.callWithCircuitBreaker(() -> CompletableFuture.completedFuture("Data received"));
getSender().tell(future, getSelf());
})
.build();
}
}
public static void main(String[] args) {
ActorSystem system = ActorSystem.create("CircuitBreakerSystem");
CircuitBreaker circuitBreaker = new CircuitBreaker(system.scheduler(),
akka.dispatch.ExecutionContexts.global(), 5, // max failures
Duration.create(1, "minute"), // reset timeout
Duration.create(10, "seconds")); // call timeout
ActorRef serviceActor = system.actorOf(Props.create(ServiceActor.class, circuitBreaker), "serviceActor");
// Future implementation to send messages and receive responses
// (Similar to the previous example)
system.terminate();
}
}
Commentary
In this code snippet, we create a ServiceActor that employs a CircuitBreaker for managing service stability. If an operation fails too many times, the circuit breaker will trip, preventing further calls until it’s safe to continue.
Why Use Circuit Breakers?
- Fault Tolerance: Avoid overwhelming services that are failing.
- Better Resource Management: Free up resources when they are not available.
- User Experience: Improve responsiveness; users are less likely to wait indefinitely for failed requests.
Exploring Further
To deepen your understanding of Akka and its messaging patterns, consider reviewing Akka Documentation and the Actor Model explanation by Martin Fowler. Both resources provide invaluable insights into the underlying mechanics of the framework and its applications.
In Conclusion, Here is What Matters
Akka’s complexity in request-response patterns is a double-edged sword. While it offers powerful mechanisms for building resilient systems, it also demands thorough planning and design considerations.
By leveraging the true potential of Akka while being cognizant of its complexities, you can build scalable, efficient applications. Whether through simple request-response or more intricate patterns like Circuit Breakers, mastering these concepts will significantly enhance your development capabilities. Happy coding!