Avoiding SQL Injection: Key Mistakes Java Developers Make
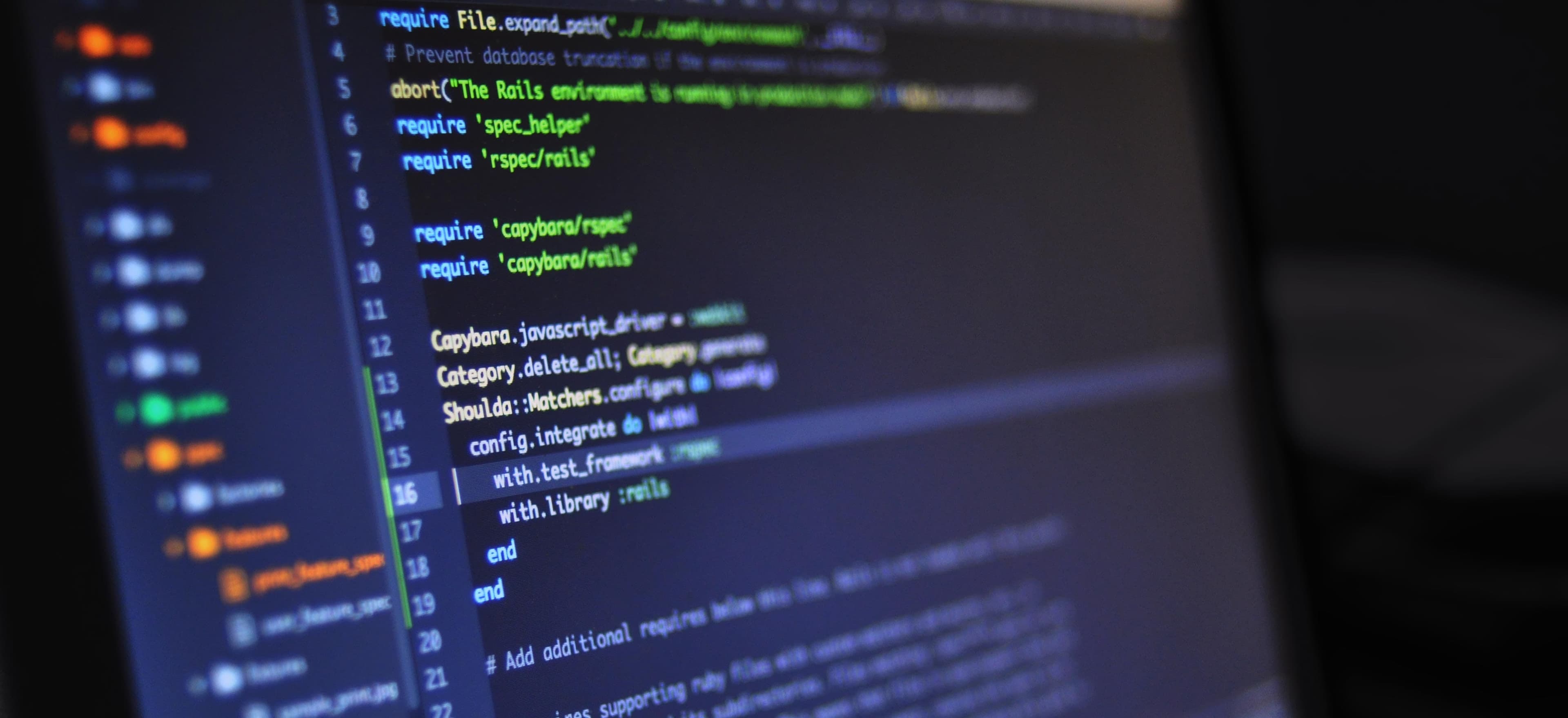
- Published on
Avoiding SQL Injection: Key Mistakes Java Developers Make
SQL injection remains one of the most significant threats to application security. For Java developers, understanding common pitfalls is vital to safeguarding applications against these attacks. This post will explore key mistakes made by Java developers in preventing SQL injection and how to avoid them effectively, with examples, explanations, and best practices.
Understanding SQL Injection
SQL injection occurs when an attacker manipulates SQL queries by injecting malicious SQL code through input fields. This can lead to unauthorized data access, data loss, and compromised application integrity.
Common SQL Injection Examples:
- Retrieving Sensitive Data: Attackers can retrieve usernames and passwords using injected queries.
- Modifying Data: Attackers can modify existing data, such as changing a user's password.
- Database Configuration Changes: Attackers can execute destructive commands that affect database configuration and management.
Key Mistakes Java Developers Make
1. Using Dynamic Queries Without Parameterization
One common mistake among Java developers is using dynamic SQL queries without parameterization. Developers often concatenate strings to form SQL commands, exposing the application to SQL injection.
Example of Bad Practice
String username = request.getParameter("username");
String password = request.getParameter("password");
String sql = "SELECT * FROM users WHERE username = '" + username + "' AND password = '" + password + "'";
// Execute SQL query
Why This is Risky:
In the above example, if an attacker inputs admin'--
as the username, the query becomes:
SELECT * FROM users WHERE username = 'admin'--' AND password = ''
The double dash (--
) comments out the remainder of the SQL command, potentially granting access to the admin account.
Best Practice
Instead, use prepared statements with parameterized queries:
String sql = "SELECT * FROM users WHERE username = ? AND password = ?";
try (PreparedStatement pstmt = connection.prepareStatement(sql)) {
pstmt.setString(1, username);
pstmt.setString(2, password);
ResultSet rs = pstmt.executeQuery();
// Process result set
}
Why This Works: Prepared statements ensure that user input is treated as data, not as executable code, preventing injection attacks.
2. Neglecting Input Validation
Another crucial mistake is neglecting input validation. Developers often assume that user input is safe without validating it against the expected format.
Example of Bad Practice
String id = request.getParameter("id");
String sql = "SELECT * FROM products WHERE id = " + id;
// Execute SQL query
Why This is Risky: If an attacker inputs a non-numeric value, it could alter the intended query.
Best Practice
Validate that input meets expected criteria before executing SQL queries:
String idStr = request.getParameter("id");
if (idStr.matches("\\d+")) { // Checks if the ID contains only digits
int id = Integer.parseInt(idStr);
String sql = "SELECT * FROM products WHERE id = ?";
try (PreparedStatement pstmt = connection.prepareStatement(sql)) {
pstmt.setInt(1, id);
ResultSet rs = pstmt.executeQuery();
// Process result set
}
} else {
throw new IllegalArgumentException("Invalid ID format.");
}
Why This Works: Input validation eliminates potentially malicious data before it can affect the SQL command, reducing the risk of SQL injection.
3. Exposing Database Errors
Database error messages can reveal sensitive information about the database structure or queries, giving attackers clues for performing SQL injection attacks. Developers often neglect to handle exceptions properly.
Example of Bad Practice
try {
// Execute SQL query
} catch (SQLException e) {
e.printStackTrace(); // Exposing detailed error message
}
Why This is Risky: The detailed stack trace may show SQL syntax or structural information that aids attackers.
Best Practice
Log errors without revealing detailed information to the end user:
try {
// Execute SQL query
} catch (SQLException e) {
// Log error with general message
logger.error("Database access error occurred. Please try again later.");
}
Why This Works: By hiding the details of the error from users, you limit the information available to potential attackers.
4. Failing to Use ORM Frameworks
Some developers opt to write raw SQL queries rather than utilizing Object-Relational Mapping (ORM) frameworks, which often have built-in protections against SQL injection.
Example of Bad Practice
public List<User> getUsersByRole(String role) {
String sql = "SELECT * FROM users WHERE role = '" + role + "'"; // Raw query
// Execute SQL query
}
Why This is Risky: This approach is prone to SQL injection, especially if user input isn't validated.
Best Practice
Use an ORM framework like Hibernate or JPA:
public List<User> getUsersByRole(String role) {
String hql = "FROM User WHERE role = :role"; // Parameterized query
Query<User> query = session.createQuery(hql);
query.setParameter("role", role);
return query.list();
}
Why This Works: ORM frameworks ensure that user input is handled in a safe manner, reducing the risk of SQL injection while also improving code quality.
5. Not Updating Dependencies
Many security vulnerabilities arise from outdated libraries and frameworks. Developers often overlook important security updates, leaving them vulnerable to SQL injection and other threats.
Best Practice
Regularly update dependencies and monitor security advisories. Tools like OWASP Dependency-Check can help manage dependency vulnerabilities.
Closing Remarks
SQL injection remains a prevalent threat, but Java developers can take proactive steps to avoid common pitfalls. Use prepared statements, validate input, hide error messages, utilize ORM frameworks, and keep dependencies updated. By adhering to these best practices, developers can significantly enhance the security posture of their Java applications.
References
- OWASP SQL Injection
- OWASP Dependency-Check
In summary, understanding and mitigating the risks of SQL injection is crucial for Java developers. By avoiding these key mistakes and employing best practices, you can build secure applications that protect sensitive user data. Secure coding practices not only defend against attacks but also promote trust and reliability in your software. Start implementing these strategies today to safeguard your applications!